Node.js Event Loop Explained
Summary
TLDRThis video dives into the Node.js event loop, explaining its various stages and how they interact to handle asynchronous operations. It starts by detailing how the event loop runs continuously when a server is active, handling timers, callbacks, and other tasks. The video also covers the differences between microtasks and macrotasks, and their priorities in the loop. The creator emphasizes the importance of understanding these details for optimizing Node.js applications, and hints at future videos on improving performance and avoiding event loop blocking.
Takeaways
- 🔄 Node.js has an event loop that operates continuously, especially when running processes like a web server.
- ⏳ The first phase of the event loop is called 'timers', where functions like `setTimeout` and `setInterval` are checked and executed.
- 📋 Microtasks, such as `process.nextTick()` and promises, have higher precedence than macrotasks like timers and I/O operations.
- ⚙️ The V8 engine, which powers Node.js and browsers, separates tasks into microtasks and macrotasks, with microtasks running first.
- ⏱️ Inside the timers phase, microtasks and macrotasks run with their own order of precedence.
- ⏲️ The 'poll' phase of the event loop is crucial for handling incoming I/O operations such as network requests and file reads.
- 💻 Understanding the internal workings of the event loop helps in optimizing Node.js applications for performance.
- 🕒 The 'check' phase is specifically for handling `setImmediate`, which executes with higher precedence over other timers.
- ❌ The last phase, 'close callbacks', handles events like socket closures and cleanup operations.
- 📊 Optimizing Node.js performance involves reducing event loop lag and avoiding blocking operations, with strategies like using worker threads.
Q & A
What is the event loop in Node.js and when does it start?
-The event loop in Node.js starts as soon as you run a Node.js script (e.g., `node index.js`). It is responsible for handling asynchronous operations, and it keeps running as long as there are tasks, such as web servers, waiting for events like incoming network connections.
What happens in the 'timers' phase of the Node.js event loop?
-In the 'timers' phase, Node.js checks for and processes any scheduled functions that were set using `setTimeout` or `setInterval`. These are executed in the order they were set, based on their delay times.
What are microtasks and how do they differ from macrotasks in Node.js?
-Microtasks have a higher priority than macrotasks and are executed before macrotasks in the event loop. Examples of microtasks include promise callbacks, `process.nextTick`, and `queueMicrotask`. Macrotasks include `setTimeout`, `setInterval`, and I/O operations like network requests.
What is the priority order for tasks within the 'timers' phase?
-The priority order is: synchronous code, `process.nextTick` (next tick queue), microtasks (e.g., promise callbacks), and finally macrotasks (timers like `setTimeout` and `setInterval`).
What role do 'pending callbacks' play in the Node.js event loop?
-The 'pending callbacks' phase handles callbacks from I/O operations that were deferred in the previous cycle of the event loop. These callbacks are executed in this phase after all timers and microtasks are processed.
What is the 'poll' phase and why is it considered the most important part of the event loop?
-The 'poll' phase is where most of the asynchronous operations happen, such as incoming connections (HTTP, TCP) and file I/O. It checks for new events and handles them, making it critical for performance in a Node.js application.
What is the significance of 'setImmediate' in Node.js?
-`setImmediate` is a special function in Node.js that schedules code to run at the next iteration of the event loop. It has a higher precedence than normal timers (`setTimeout`) and is executed in the 'check' phase.
What is the 'close callbacks' phase in the Node.js event loop?
-The 'close callbacks' phase is the final step of the event loop, where it handles callbacks for closing events, such as when a TCP connection or file stream is closed.
Why is understanding the event loop important for Node.js developers?
-Understanding the event loop helps developers write more efficient and optimized code by knowing how asynchronous tasks are handled, how to prevent blocking the event loop, and when to use certain functions like `setImmediate`, `process.nextTick`, and microtask queues.
What is 'event loop lag' and how can it affect the performance of a Node.js application?
-Event loop lag occurs when long-running operations or synchronous code block the event loop, preventing it from handling other tasks. This can degrade the performance of a Node.js application, especially in cases involving high concurrency or heavy I/O.
Outlines
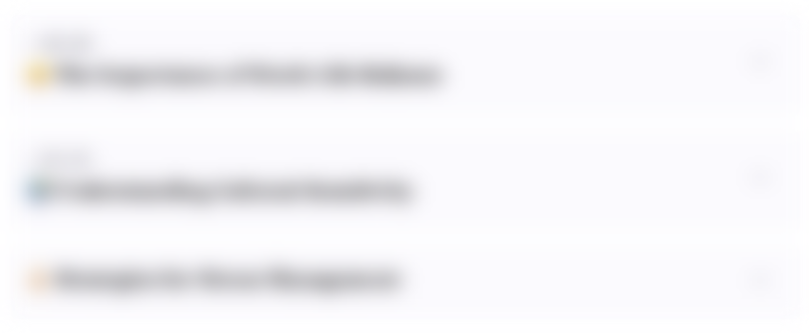
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
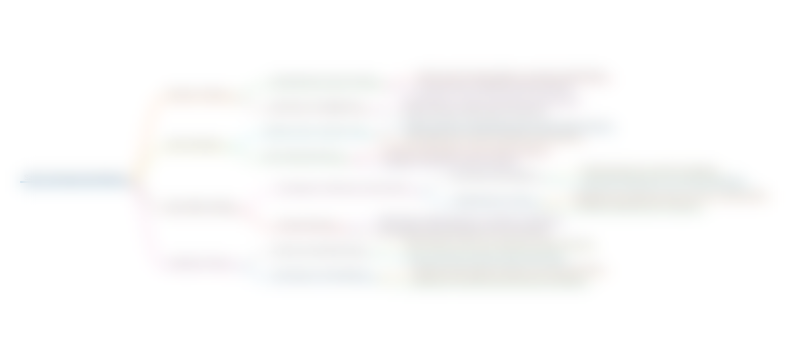
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
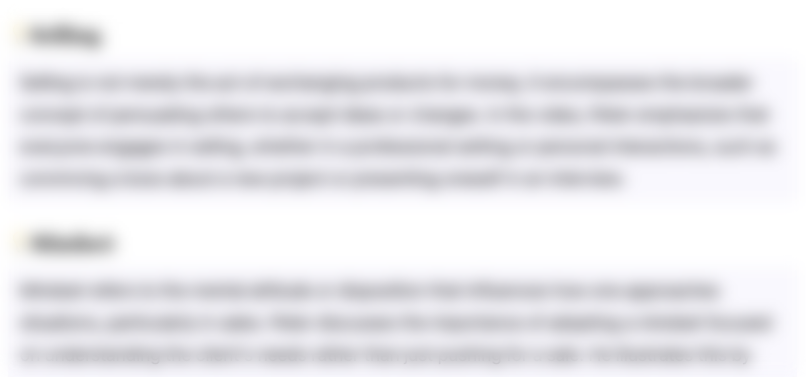
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
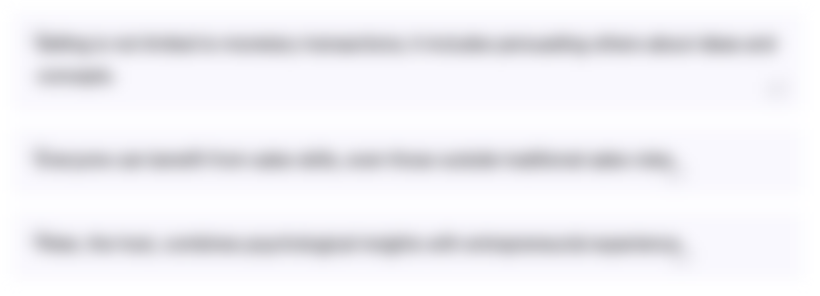
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
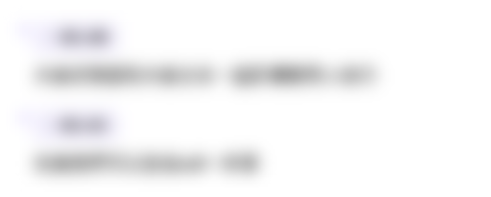
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
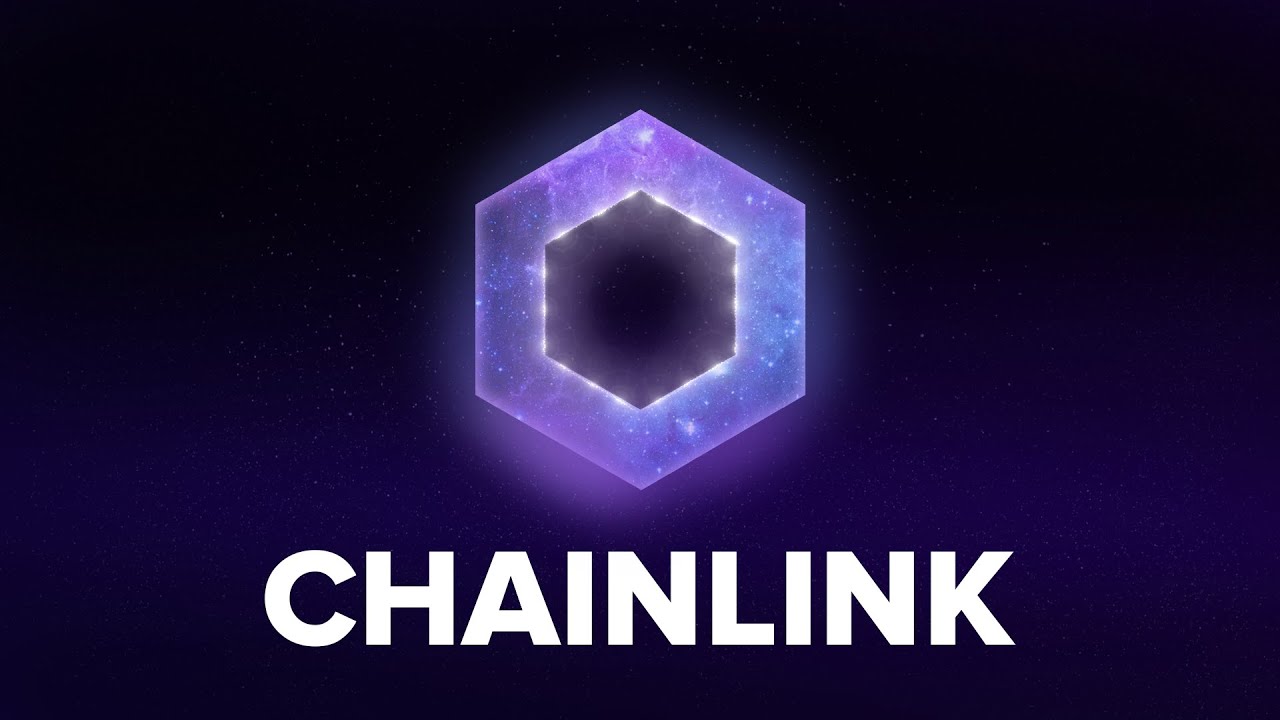
What is Chainlink? LINK Explained with Animations (Price Prediction)
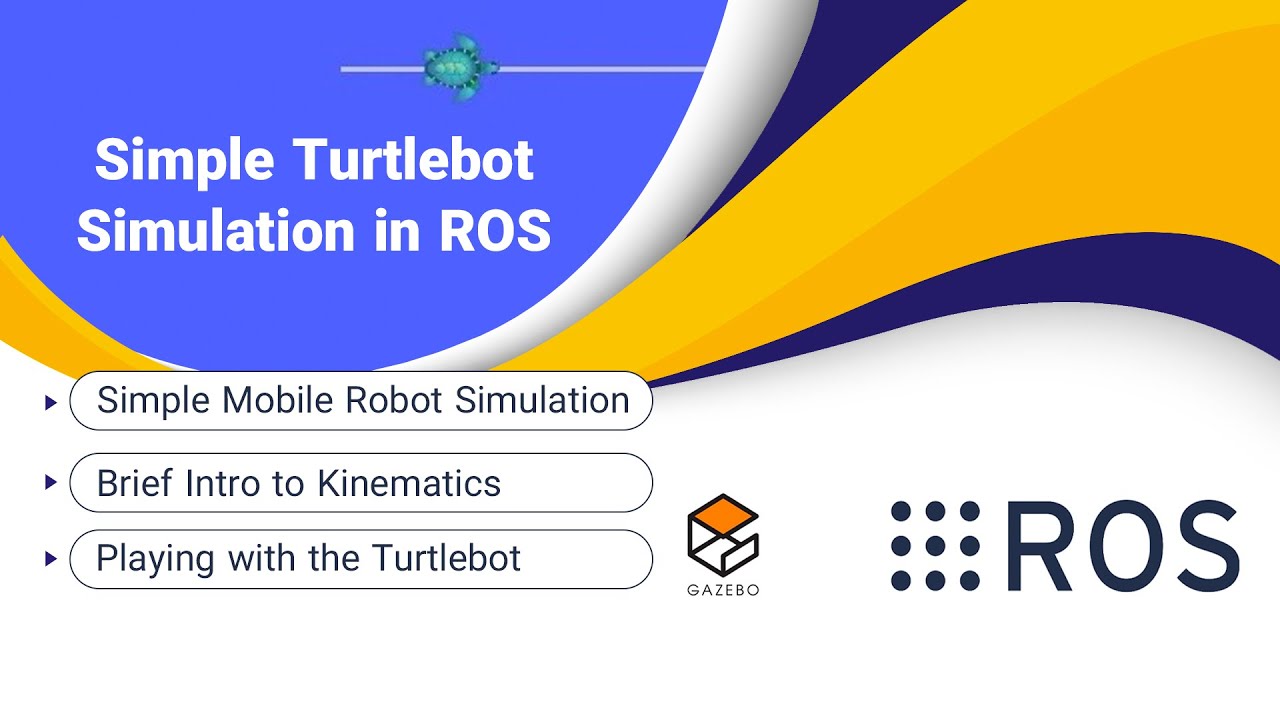
Simple Turtlebot Simulation in ROS | ROS 101 | ROS Tutorials for Beginners | Lesson 3
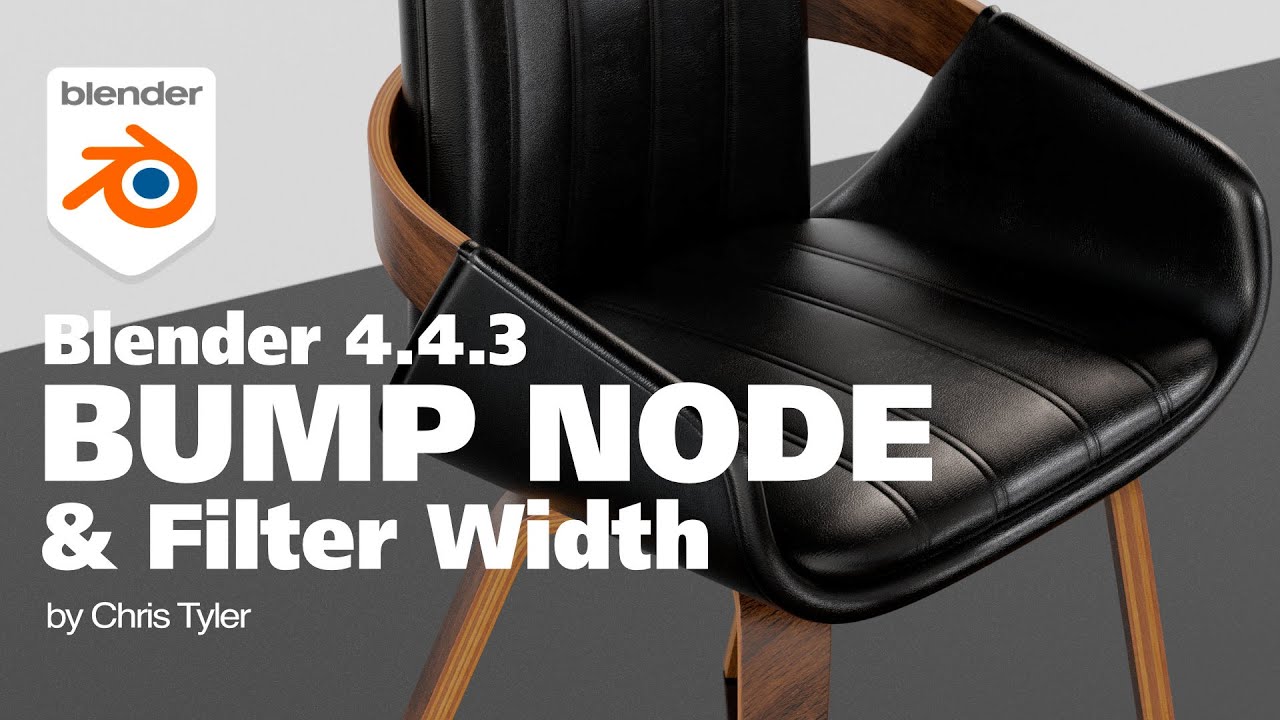
Bump Node & Filter Width, what you need to know.
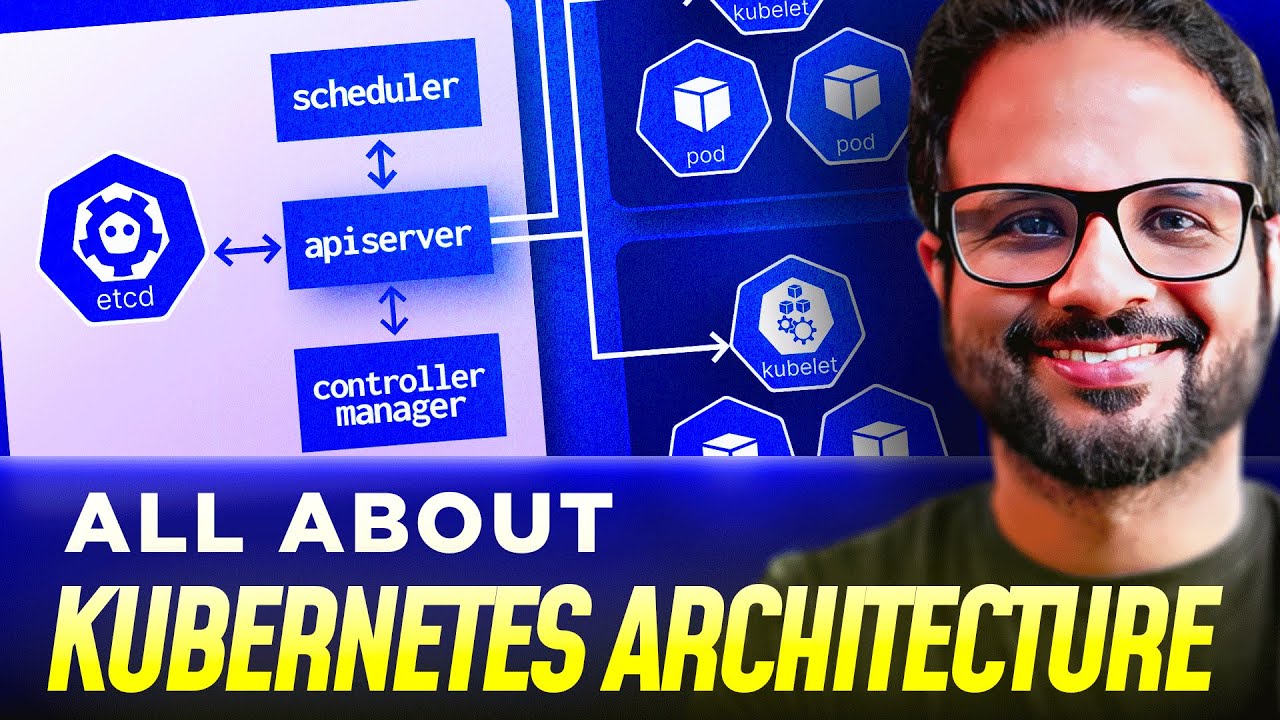
Day 5/40 - What is Kubernetes - Kubernetes Architecture Explained

Exit Nodes | Tailscale Explained
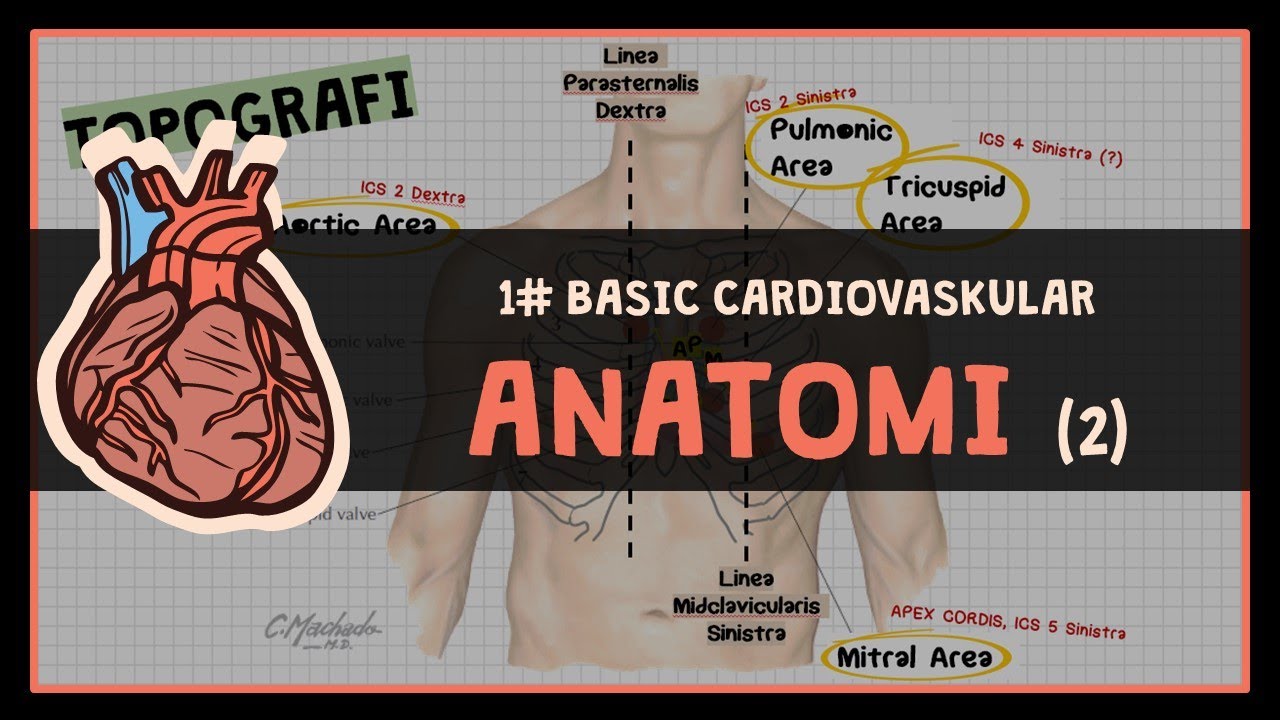
(2/2) Anatomi Sistem Konduksi & Vaskular Jantung dan Topografi : #1 BASIC CARDIOVASKULAR

Lec-101: Insertion in B-Tree with example in Hindi
5.0 / 5 (0 votes)