excep7 writing your own exception classes
Summary
TLDRThis video explains key Java concepts related to exceptions, focusing on the difference between the 'throws' and 'throw' keywords. It walks through how to declare exceptions in method signatures using 'throws' and how to generate exceptions within methods using 'throw.' The video also dives into custom exception handling, showcasing an example of a 'NoStockException' and 'PurchasingException,' and how to handle checked vs. unchecked exceptions. It concludes by demonstrating exception propagation and how to partially process exceptions before re-throwing them in more complex systems.
Takeaways
- ๐ The 'throw' keyword is used to actually throw an exception within a method, while 'throws' is used in the method signature to declare potential exceptions.
- ๐ The 'throws' keyword can specify multiple exception types, separated by commas.
- ๐ The 'throw' keyword creates an exception instance, and it must be used within a method body.
- ๐งต 'NoStockException' is an example of an unchecked exception, extending 'RuntimeException'.
- ๐๏ธ To create custom exceptions, extend either 'Exception' (for checked exceptions) or 'RuntimeException' (for unchecked exceptions).
- ๐จ Checked exceptions need to be either caught or declared in the method signature using 'throws'.
- ๐ก Catching an exception within the same method that throws it is usually pointless unless specific processing is required.
- ๐ Exceptions can be partially processed and re-thrown to the caller, often encapsulating a different custom exception.
- ๐ฆ The purchasing department class handles exceptions from an external vendor and converts them to a 'PurchasingException' before propagating them.
- ๐ When using try-catch blocks, printing the stack trace directly to the console isn't recommended for production code; it's better to log or handle the message appropriately.
Q & A
What is the primary difference between the 'throws' and 'throw' keywords in Java?
-The 'throws' keyword is used in a method signature to declare that the method might throw certain exceptions, while the 'throw' keyword is used within a method to actually throw an instance of an exception.
How does the 'parseInt' method from the Integer class in Java handle exceptions?
-The 'parseInt' method checks if the input string is null. If it is, it uses the 'throw' keyword to create and throw a new 'NumberFormatException'. The 'throws' keyword is used in the method signature to indicate that 'NumberFormatException' might be thrown.
What is exception propagation in Java?
-Exception propagation refers to the process where an exception thrown in a method is passed up the call stack until it's caught by a surrounding try-catch block or terminates the program.
How can you create a custom unchecked exception in Java?
-To create a custom unchecked exception, you extend 'RuntimeException'. In the provided example, a 'NoStockException' is created as an unchecked exception by extending 'RuntimeException' and adding a constructor that takes a message.
What happens when the 'NoStockException' is thrown by the 'orderComputer' method in the external vendor class?
-When 'NoStockException' is thrown by the 'orderComputer' method, it is not caught within the method. The exception propagates back to the 'purchaseComputer' method of the purchasing department, and then further to the main method where it is caught in a try-catch block.
Why doesn't it make sense to throw and catch an exception in the same method?
-Throwing and catching an exception in the same method is redundant because the purpose of throwing an exception is to notify the caller about a problem. Catching it in the same method defeats this purpose since no one else gets notified about the issue.
What is the purpose of logging an exception and then re-throwing a different one?
-Logging an exception and then re-throwing a different one allows you to handle and document the specific error while re-throwing a more general or relevant exception for the higher-level caller to process. This approach is often used when creating domain-specific exceptions like 'PurchasingException'.
What is the difference between checked and unchecked exceptions in Java?
-Checked exceptions must be either caught or declared to be thrown in the method signature. Unchecked exceptions, which extend 'RuntimeException', don't require handling or declaration. In the script, 'NoStockException' is changed from an unchecked exception to a checked exception to demonstrate this difference.
What happens if a method that throws a checked exception doesn't declare it in its method signature?
-If a method that throws a checked exception doesn't declare it in the method signature, the program will fail to compile. The exception must either be caught within the method or declared in the method signature using the 'throws' keyword.
How can you propagate an exception after partially processing it?
-You can catch an exception, process it (e.g., log it), and then propagate a new exception by throwing a different exception with a relevant message. For example, in the purchasing department class, a 'NoStockException' is caught, and a new 'PurchasingException' is thrown with an updated message.
Outlines
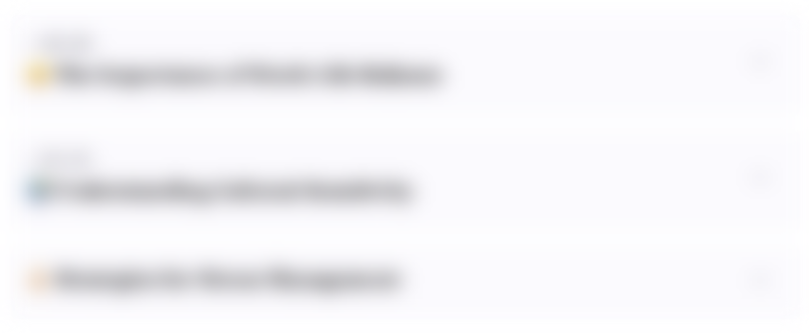
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
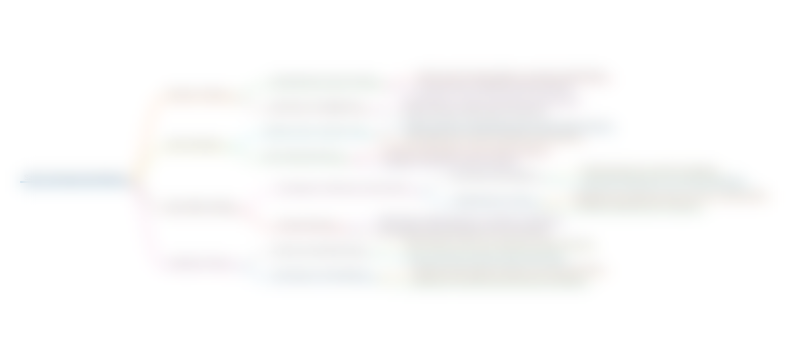
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
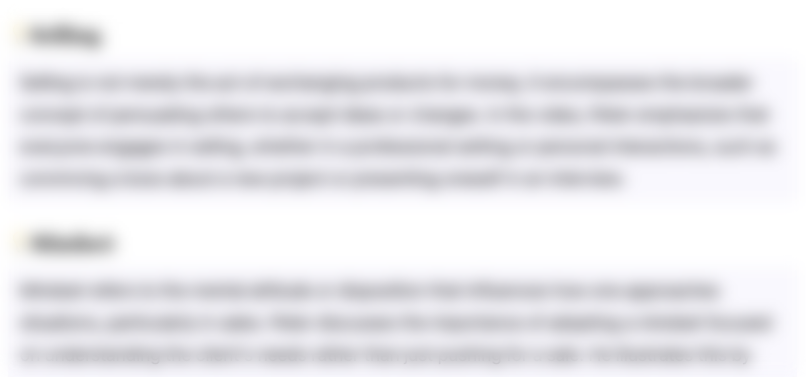
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
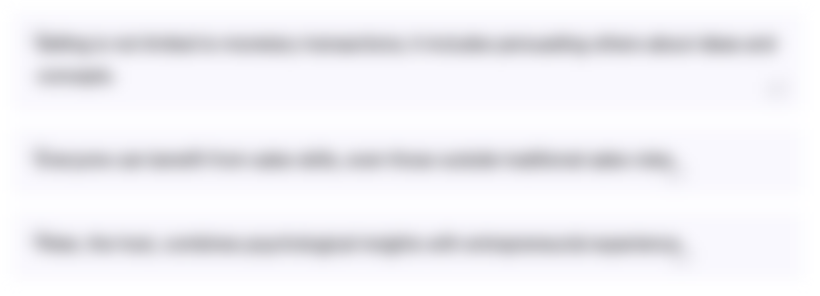
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
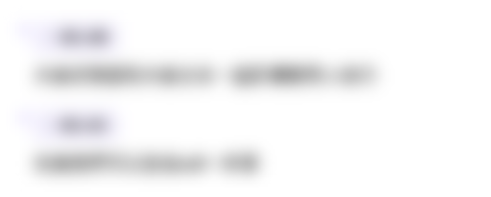
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

JAVA TECHNICAL QUESTION AND ANSWERS FOR INTERVIEW PART III (Classes & Objects)
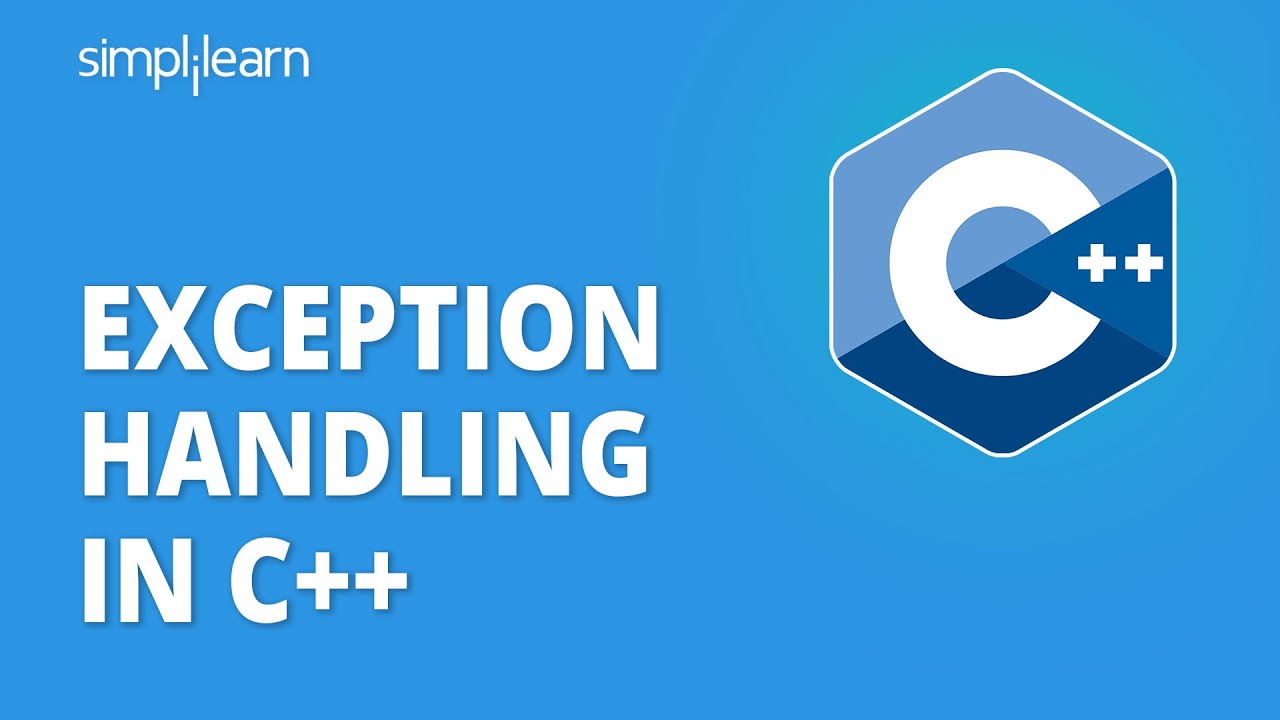
Exception Handling In C++ | What Is Exception Handling In C++ | C++ Programming | Simplilearn
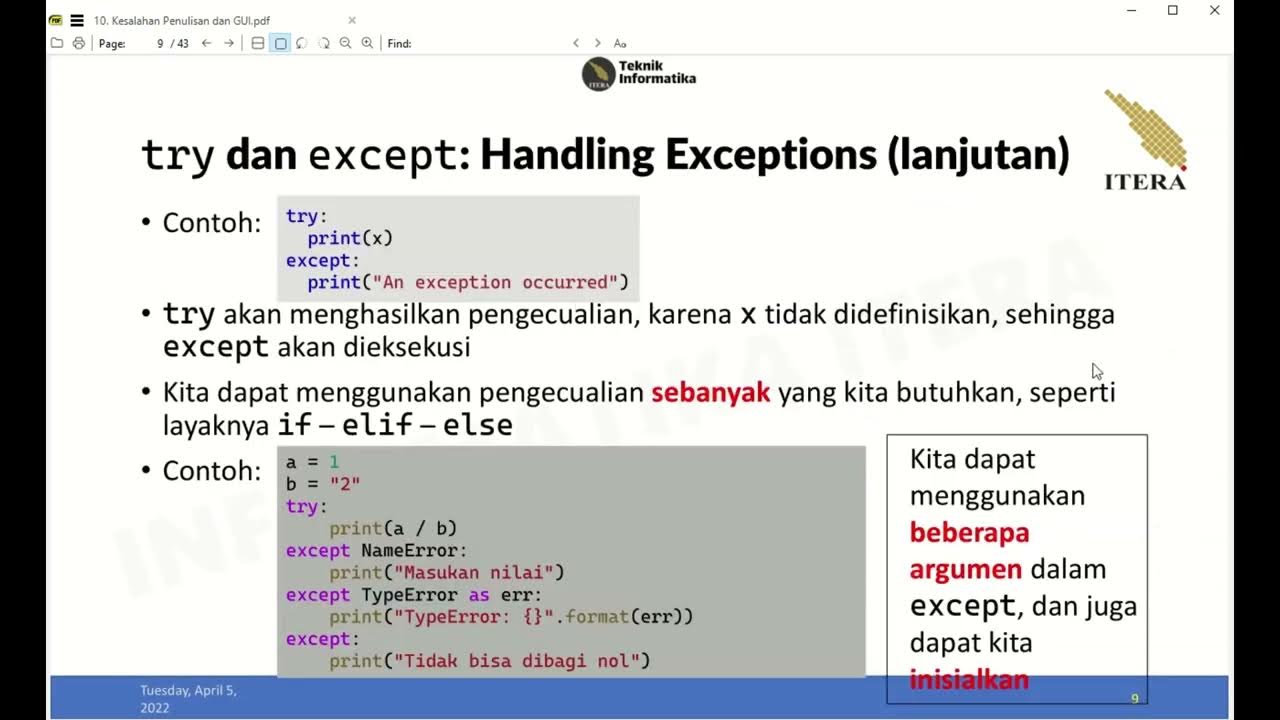
Exception pada Pemrograman Berorientasi Objek - Python

java microservice telephonic interview of 10 years experienced
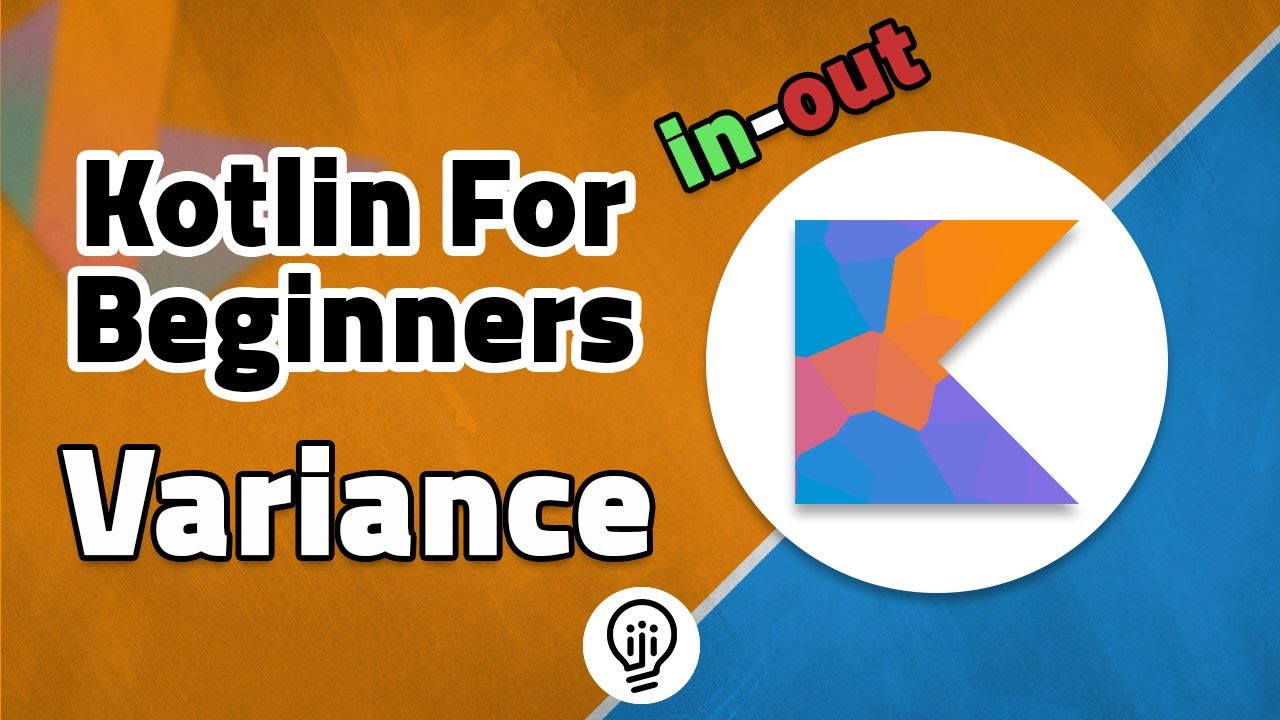
Kotlin For Beginners - Variance, Covariance, Contravariance and Type erasure

Affix, Root, Stem, Base
5.0 / 5 (0 votes)