Tracing function calls | Intro to CS - Python | Khan Academy
Summary
TLDRThe video explains the detailed process of how a computer executes a function call in Python. It walks through how the program reads the code, stores function definitions in memory, and then creates a separate stack frame for each function call to isolate its execution. The instructor highlights the importance of simplifying expressions before passing arguments, how return values are processed, and how the memory is managed between global and function-specific contexts. This clear breakdown helps viewers understand the mechanics of function calls and memory management in Python programming.
Takeaways
- 🖥️ The computer reads and processes the program line by line, starting at the top.
- 📜 When encountering a `def` keyword, the computer stores the function's body in memory, but does not execute it yet.
- 📂 The function name and number of parameters are tagged and saved for later use in memory.
- 💾 Variable assignments store values in memory, and expressions are simplified before they are stored.
- 🧮 Function calls are simplified down to single values before being executed.
- 🧱 A new stack frame is created for each function call to isolate the function's memory from the main program.
- 🔗 Parameters are passed into the stack frame, and the function executes within its own isolated memory space.
- 🛑 The `return` statement ends the function, returns the computed value, and clears the function's stack frame.
- 🚫 If a function call's return value isn't stored, it's lost once the function completes.
- 📋 The program clears all memory, including user-defined functions, at the end of execution.
Q & A
What happens when the computer encounters a function definition?
-When the computer sees the 'def' keyword, it defines the function in memory, tagging the location with the function name and the number of parameters. The function itself is not executed at this point, just stored for later use.
Does the computer execute the function code when it first reads the definition?
-No, the computer does not execute the function code when it first encounters the definition. It only stores the function and its parameters in memory, waiting for the function to be called later in the program.
What is the purpose of a stack frame when a function is called?
-A stack frame is created when a function is called to isolate the function's memory from the global memory. It helps ensure that the function operates in its own memory space without affecting or accessing the rest of the program.
How does the computer handle function parameters during a function call?
-The computer copies the argument values passed during the function call into new locations within the stack frame. These values are tagged with the corresponding parameter names from the function definition.
What happens after the function completes its execution?
-Once the function completes its execution, the return value is calculated, and the stack frame for the function is cleared. The return value is passed back to the point in the code where the function was called.
What happens to a function’s return value if it is not used?
-If a function’s return value is not assigned or used, it is essentially lost. The value is discarded after the function call is completed.
Why is it important to avoid unnecessary function calls in a program?
-Unnecessary function calls waste the computer’s processing time by calculating values that are not used. It’s better to only call functions when the results are needed.
What is the significance of indentation in Python functions?
-In Python, indentation is critical for defining the scope of the function body. The lines of code that are indented after the function header are part of the function, and this structure must be maintained.
Does Python retain user-defined functions between program executions?
-No, Python does not retain user-defined functions between program executions. The function definition must be included each time the program is run to 'teach' the computer how to execute that function.
What happens when a return statement is reached in a function?
-When a return statement is reached, the function execution is terminated, and the return value is passed back to the point where the function was called. The stack frame used by the function is cleared.
Outlines
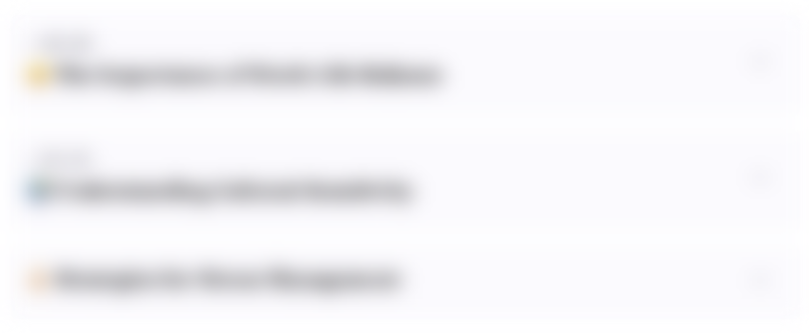
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
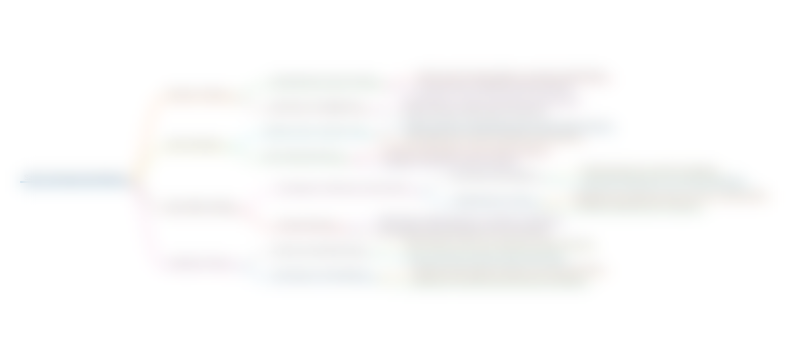
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
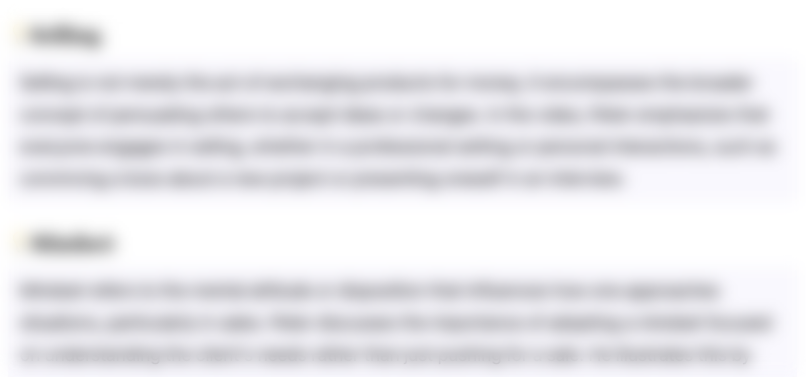
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
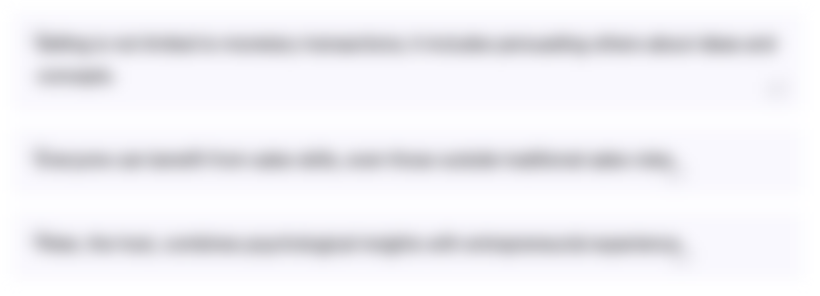
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
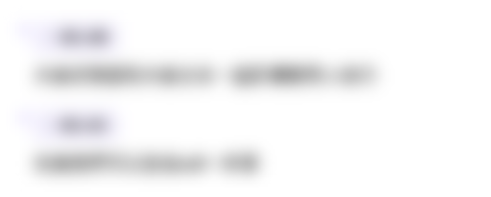
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
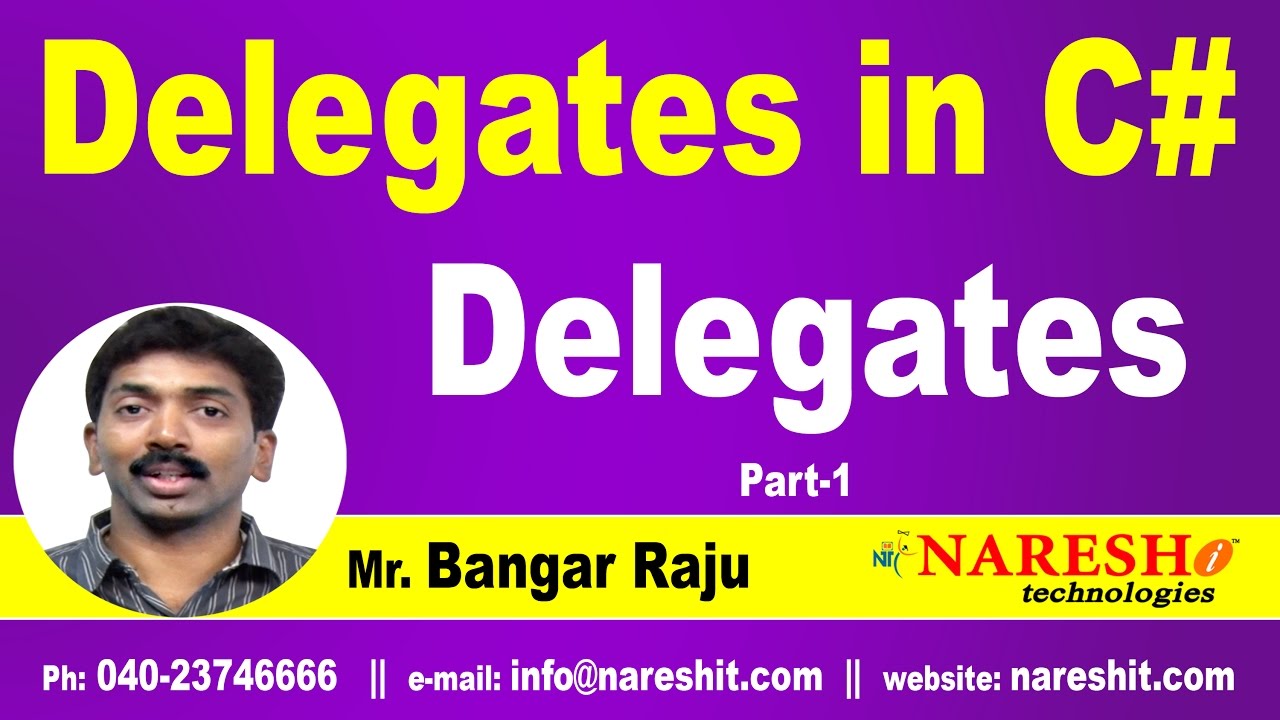
Delegates in C# | Delegates Part 1 | C#.NET Tutorial | Mr. Bangar Raju
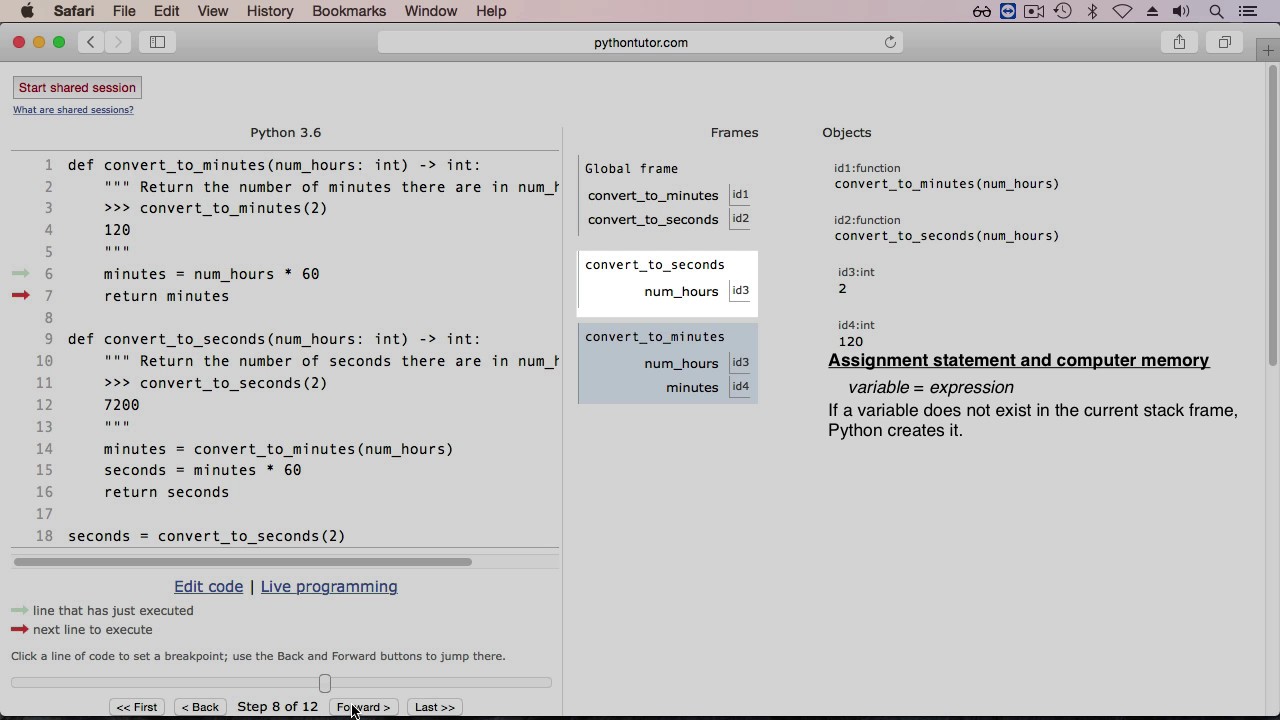
3scopeintro
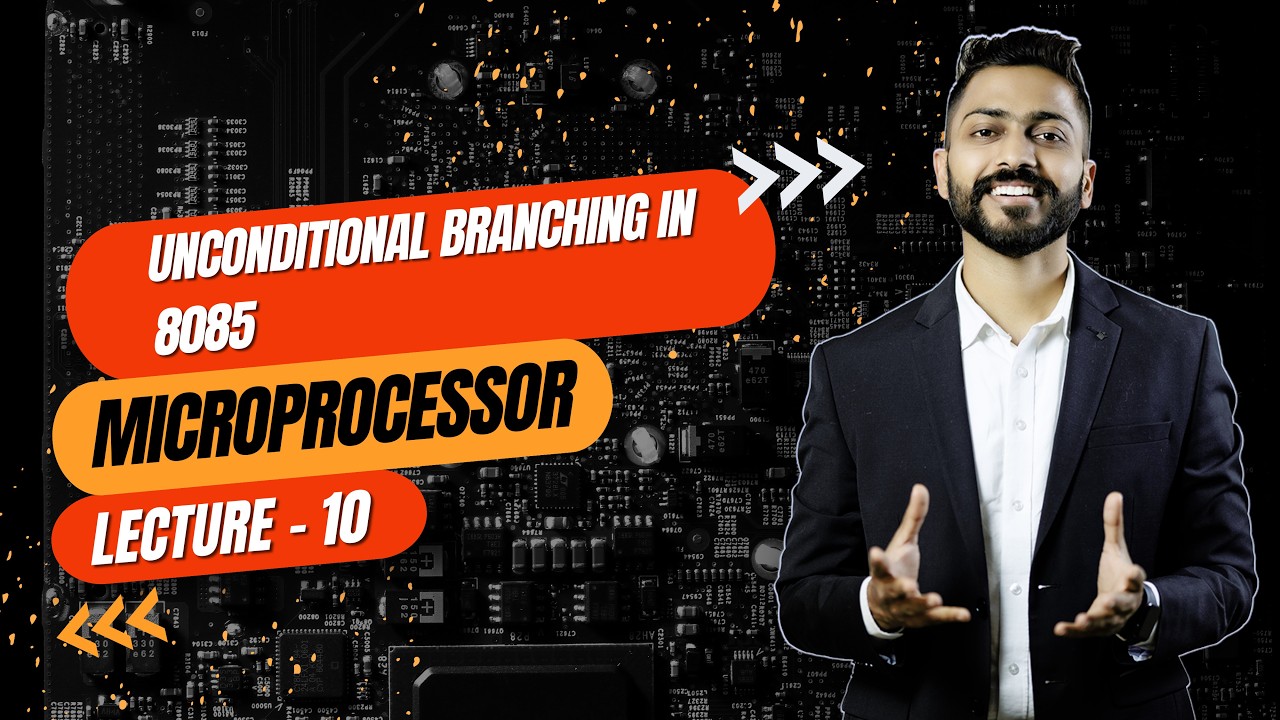
Lec-10: Unconditional Branching in 8085 | Microprocessor
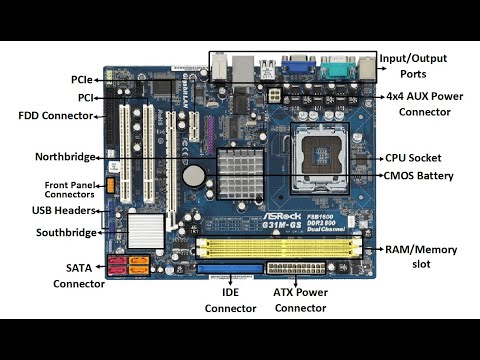
How to Disassemble/Assemble a Computer System Unit? - Basic Parts and Function

JavaScript - Throttle (節流) 常見的面試問題 (Scrollbar, Infinite Scroll) (前端優化)

Cara Kerja CPU Dalam Komputer Secara Aritmatika dan Logika
5.0 / 5 (0 votes)