Learn CORS In 6 Minutes
Summary
TLDRThis video tutorial explains how to resolve CORS errors in web development. It demonstrates using a simple script and server setup with Express, illustrating how to fix CORS by setting the 'Access-Control-Allow-Origin' header. The video shows how to use the 'cors' library in Express to allow requests from specific origins or any origin. It also covers handling preflight 'OPTIONS' requests for methods like PUT and DELETE, and how to enable the sending of cookies with CORS by setting the 'Access-Control-Allow-Credentials' header.
Takeaways
- π οΈ CORS stands for Cross-Origin Resource Sharing, and it's a mechanism that uses additional HTTP headers to tell browsers to allow a web application to request resources from a different domain than the one from which the first resource was served.
- π« A common CORS error occurs when a web application tries to fetch data from a server on a different port or domain, and the server does not have the appropriate CORS headers set.
- π§ To fix CORS errors, you need to set the 'Access-Control-Allow-Origin' header on the server to specify which origins are allowed to access the server's resources.
- π The script example in the video demonstrates a simple Express server setup that listens on port 3000 and how to configure it to allow requests from a client running on port 5500.
- π By default, browsers only allow requests to the same origin. If you need to make requests to a different origin, you must specify the allowed origins using the CORS headers.
- π The 'cors' library in Node.js is used to simplify the process of setting CORS headers in Express applications.
- π When making requests like PUT or DELETE, browsers send an 'OPTIONS' preflight request first to check if the actual request method is allowed by the server.
- π The 'cors' library allows you to specify which methods are allowed by setting the 'methods' property in the configuration.
- πͺ If you need to send cookies with a request (include credentials), you must set the 'Access-Control-Allow-Credentials' header to 'true' on the server.
- π The video provides a comprehensive guide to handling CORS errors and securing web applications by controlling which origins and methods are allowed.
- π For further reading and related security videos, resources are provided in the video description.
Q & A
What is the main issue the video script is addressing?
-The video script is addressing the issue of CORS (Cross-Origin Resource Sharing) errors that occur when trying to make requests from one origin to another, and how to fix them.
What is CORS and why is it important?
-CORS is a security feature implemented by browsers to restrict web pages from making requests to a different domain than the one that served the web page. It's important for security reasons to prevent malicious websites from making unauthorized requests to other domains.
What is the 'Access-Control-Allow-Origin' header and why is it necessary?
-The 'Access-Control-Allow-Origin' header is an HTTP response header that specifies which origins are permitted to read the response. It is necessary to allow requests from different origins to access the resources on the server.
How does the video demonstrate a CORS error?
-The video demonstrates a CORS error by showing an attempt to fetch data from localhost:3000 from a client running on localhost:5500, resulting in an error message stating that 'No 'Access-Control-Allow-Origin' header is present on the requested resource.'
What library is suggested to handle CORS in an Express server?
-The library suggested to handle CORS in an Express server is 'cors', which can be installed using npm.
What is the purpose of the 'methods' option in the 'cors' library?
-The 'methods' option in the 'cors' library is used to specify an array of HTTP methods that are allowed when making requests from different origins.
What is an 'OPTIONS' request and why is it used?
-An 'OPTIONS' request is a preflight request used by the browser to determine whether the actual request is allowed by the server. It is used for methods like PUT or DELETE to check if the server allows these types of requests.
How can you allow any origin to access your server resources using the 'cors' library?
-You can allow any origin to access your server resources by setting the 'origin' parameter in the 'cors' library to '*'.
What does the 'include' option do when set to 'credentials'?
-The 'include' option, when set to 'credentials', allows the request to include credentials such as cookies, authorization headers, or TLS client certificates.
How do you handle CORS when dealing with cookies?
-To handle CORS with cookies, you need to set the 'Access-Control-Allow-Credentials' header to 'true' on the server side, and include the 'credentials' option in the 'cors' library configuration.
What additional resources are provided for further learning about CORS?
-The video offers links to more security-related videos and a full written version of the video in the description below for further learning about CORS.
Outlines
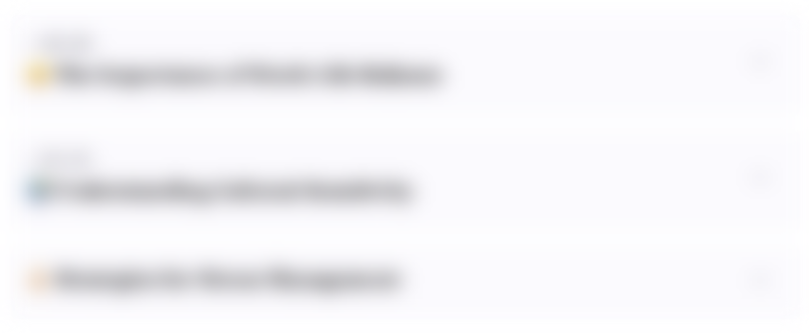
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
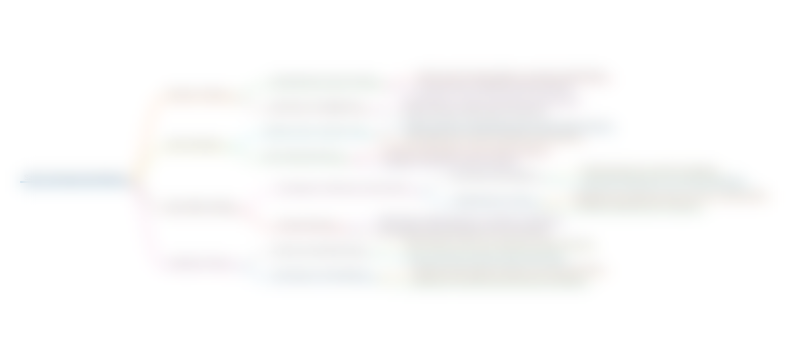
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
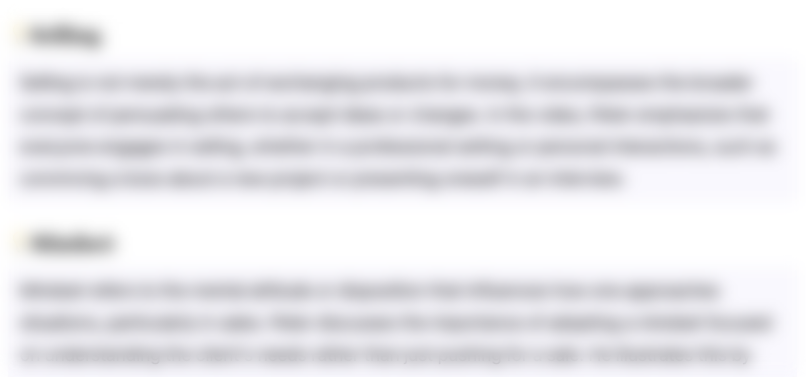
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
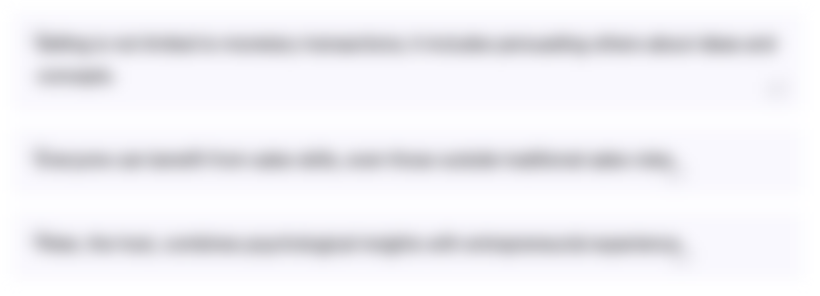
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
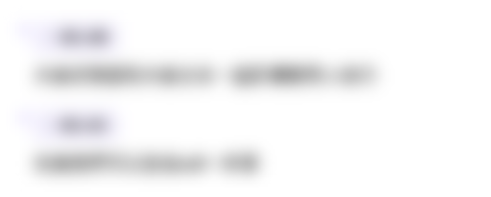
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
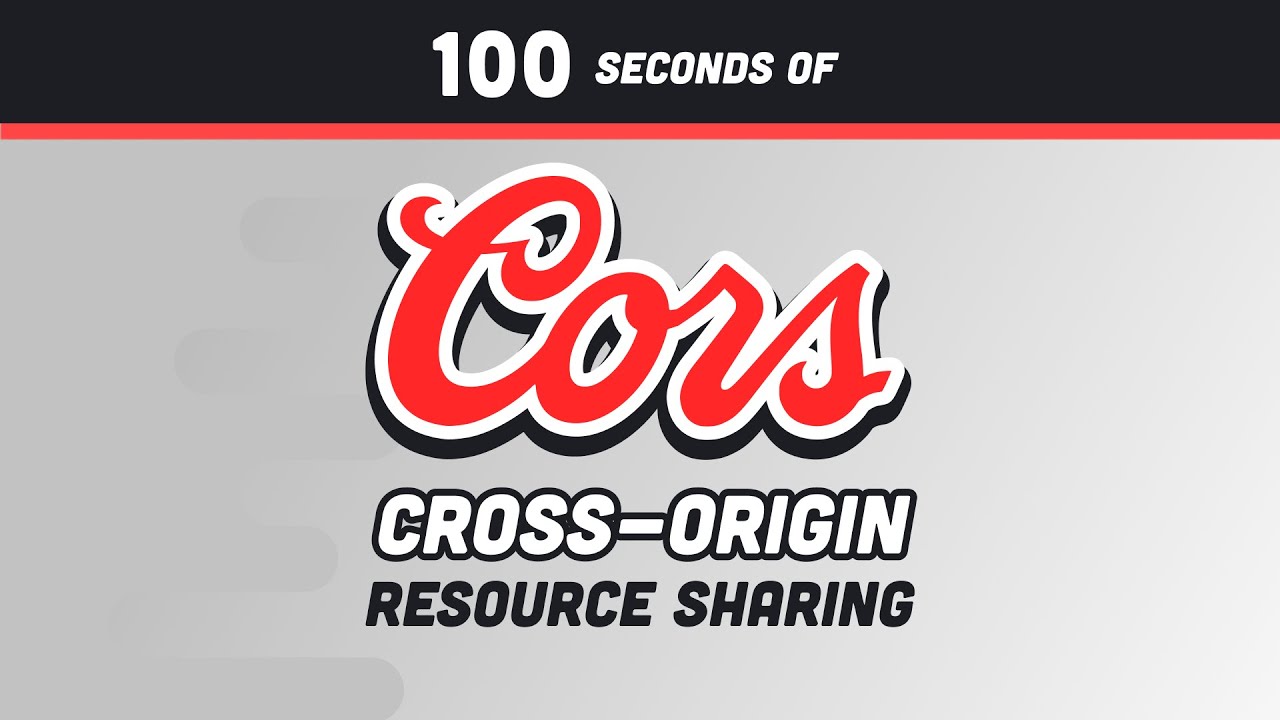
CORS in 100 Seconds
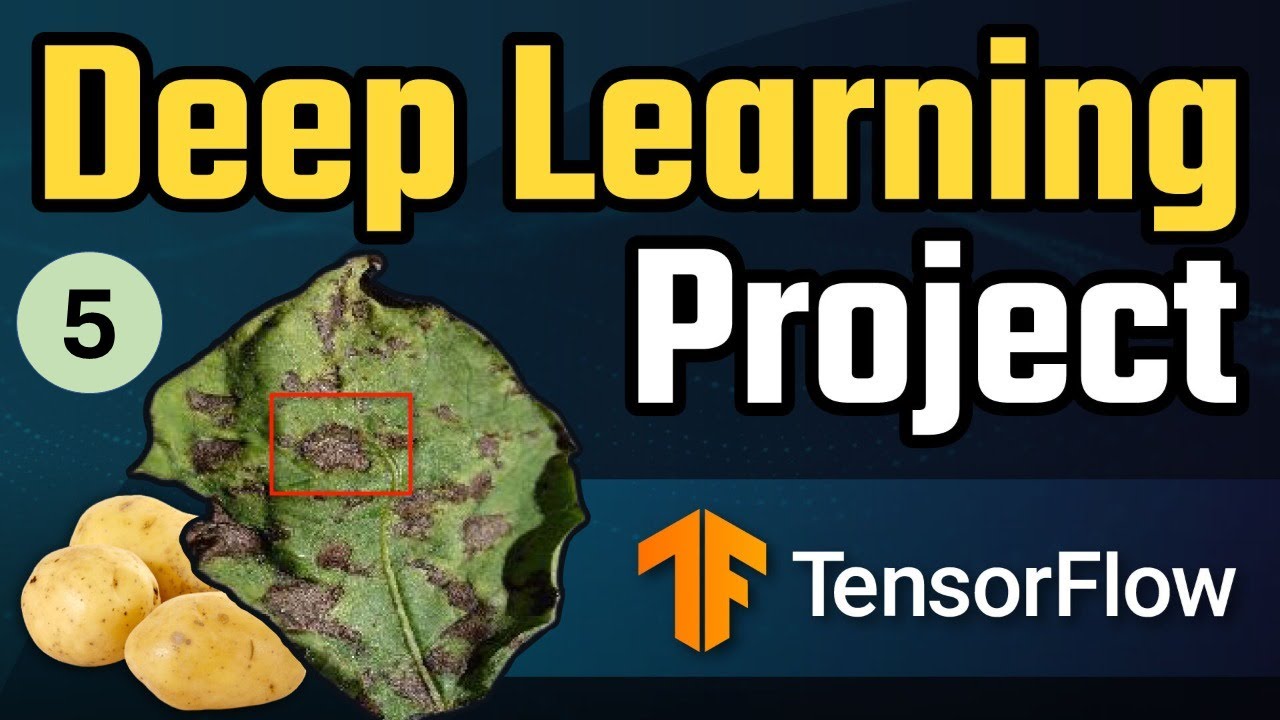
Deep learning project end to end | Potato Disease Classification - 5 : Website (In React JS)
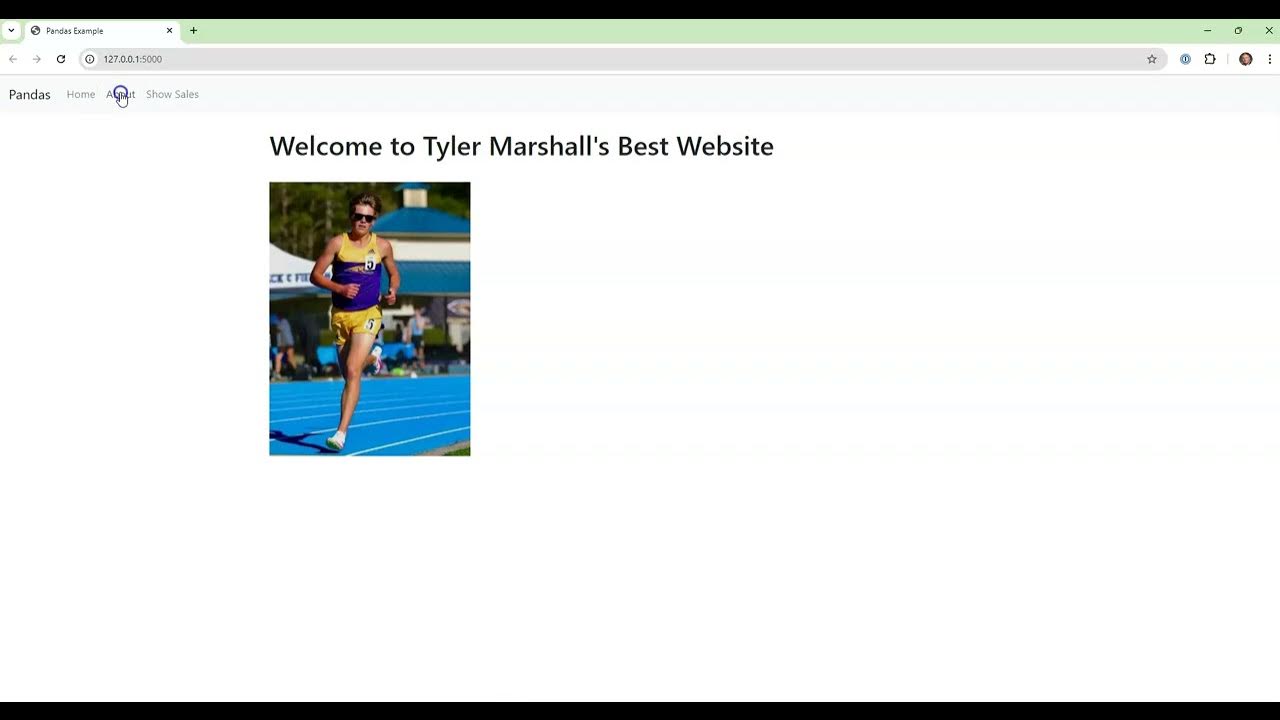
Python Module 6 (Part 1) Using Python Libraries Like Pandas for Displaying Data

#26: Connect React with NodeJS & MongoDB | Stored Registration Form Data in Database in MERN
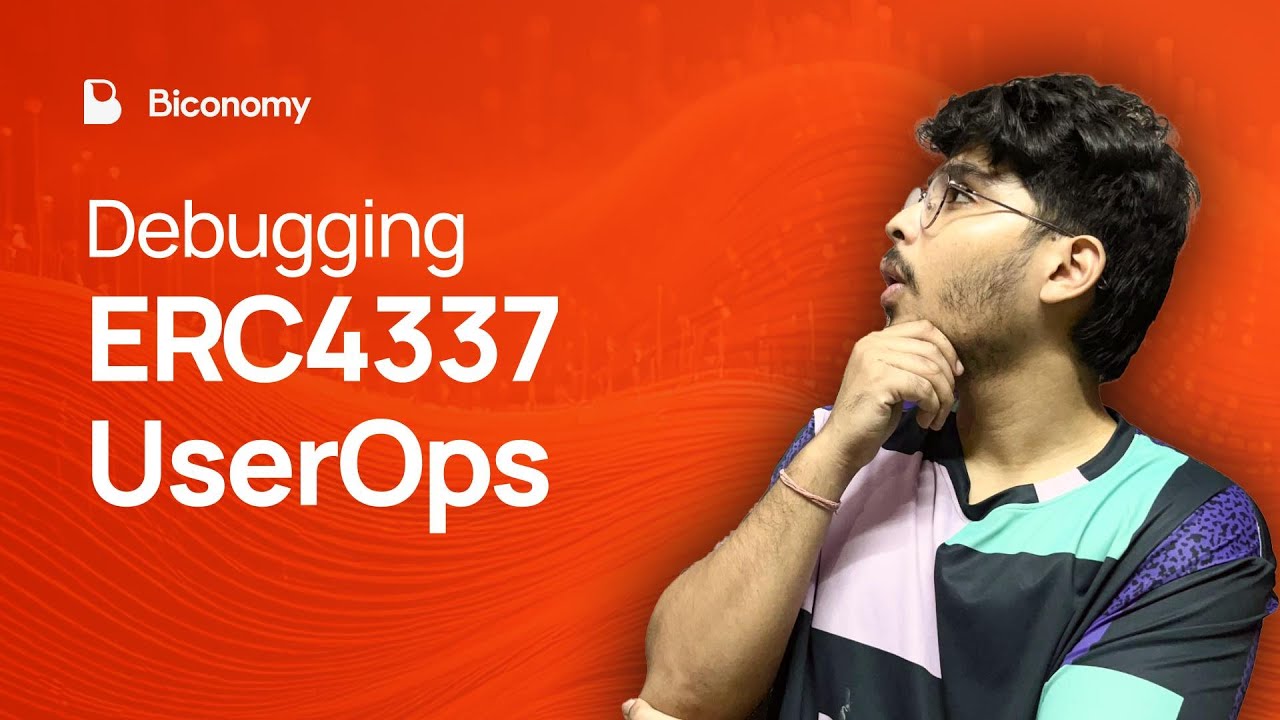
Debugging ERC4337 UserOps using Tenderly

How to Handle SSL Certificate in selenium Webdriver || Ganesh Jadhav Automation Studio
5.0 / 5 (0 votes)