Without delegate this won't be possible
Summary
TLDRThis video explains the importance of delegates in C# programming, particularly in scenarios where certain functionalities cannot be achieved without them. It demonstrates how delegates allow developers to introduce additional logic and handle specific tasks dynamically. Using a simple example of generating GUIDs, the video shows how a delegate can trigger a method when a condition is met (e.g., GUID starting with 'A'). The video emphasizes the critical role of delegates in enhancing flexibility and extending functionality in third-party software applications.
Takeaways
- 😀 Delegates in C# are crucial for handling functionality that cannot be achieved otherwise.
- 🛠️ Delegates are often used to integrate additional logic or functionality into third-party software.
- 📜 Example given: A simple software is created to generate GUIDs, and a delegate is introduced to add more flexibility.
- 🔗 Delegates allow passing methods as arguments, enabling more dynamic and flexible code.
- 🔍 In the example, a `GenerateGID` method generates a list of GUIDs, but delegates allow specific actions based on certain conditions (e.g., if the GUID starts with a specific character).
- 🖥️ The process involves creating a delegate `public delegate void SpecificGUID(string currentGUID)` and linking it to the GUID generation method.
- 🎯 The delegate is triggered when certain conditions are met, allowing for custom logic execution.
- 📥 A delegate method `PrintCurrentGUID` is defined and passed as a parameter, printing GUIDs that start with 'A'.
- 📊 Without delegates, it would be more difficult to inject this kind of dynamic functionality into existing code, particularly when working with third-party libraries.
- 🤔 Delegates are essential when you need to add extra logic, especially for event handling or callbacks.
Q & A
What is a delegate in C#?
-A delegate in C# is a type that defines a method signature, allowing methods to be passed as parameters to other methods. It helps in designing flexible and reusable code by enabling the addition of custom logic to existing methods.
Why are delegates essential in C#?
-Delegates are essential in C# because they allow the programmer to encapsulate methods and pass them as arguments to other methods. This is especially useful when adding new logic to existing functionality or when handling events.
How is the delegate used in the given code example?
-In the example, the delegate is used to create a specific functionality where the system checks if a generated GUID starts with the letter 'A'. If it does, the delegate is invoked to execute the associated method.
What is the purpose of the `generateGID` method in the code example?
-The `generateGID` method generates a specified number of unique GUIDs and returns them as a list of strings. It loops through the count, generates a GUID, and formats it before adding it to the result list.
How does the delegate enhance the functionality of the third-party software in the example?
-The delegate enhances functionality by allowing the client to add custom logic (like printing GUIDs starting with 'A') without modifying the core method. This decouples the main functionality from specific behaviors.
What is the signature of the delegate in the example?
-The signature of the delegate in the example is `public delegate void specificGuid(string currentGuid);`, which accepts a string parameter representing the current GUID and returns void.
What method satisfies the delegate signature in the client code?
-The `printCurrentGUID` method satisfies the delegate signature. It accepts a string parameter (the current GUID) and writes a message if the GUID starts with the letter 'A'.
What happens if the generated GUID starts with the letter 'A'?
-If the generated GUID starts with the letter 'A', the delegate is invoked, and the associated method (in this case `printCurrentGUID`) is executed to print a message indicating the GUID starts with 'A'.
How would the functionality be limited without the use of a delegate?
-Without the delegate, it would be difficult to extend or modify the functionality of the third-party software without directly altering its code. The delegate allows the client to add custom behavior dynamically.
Can you pass multiple methods to a delegate?
-Yes, you can pass multiple methods to a delegate in C#. This is done by combining delegate instances using the `+` operator or the `Delegate.Combine` method. When the delegate is invoked, all the methods will be called in the order they were added.
Outlines
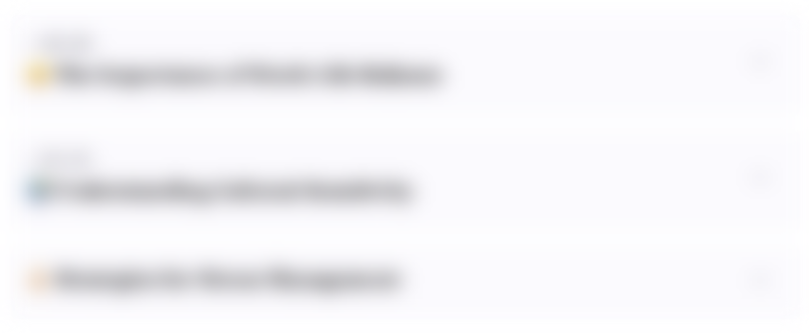
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
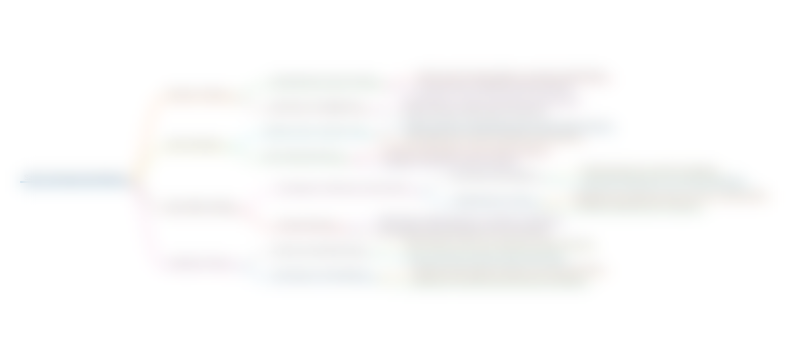
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
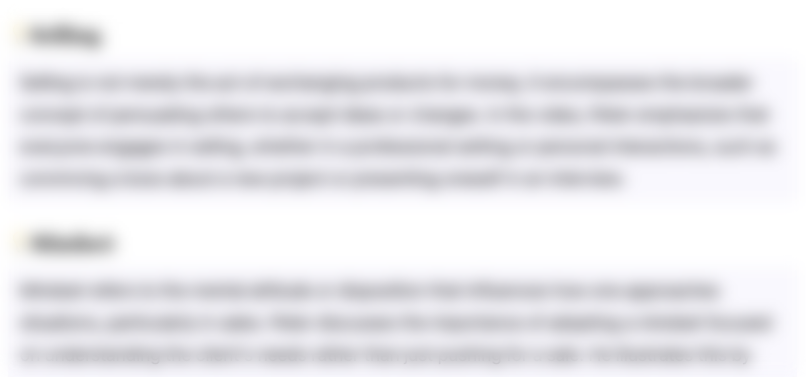
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
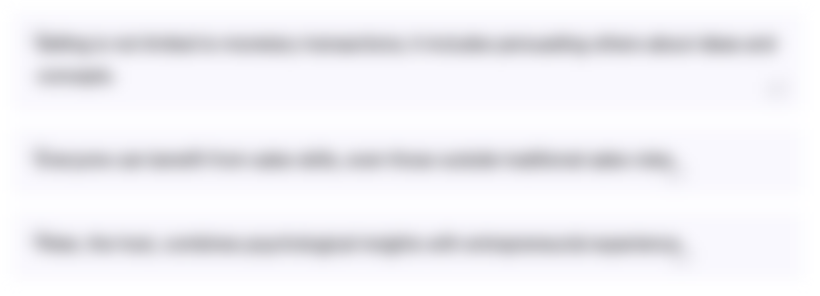
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
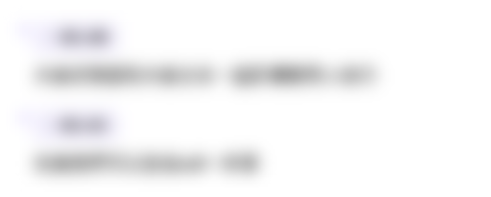
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
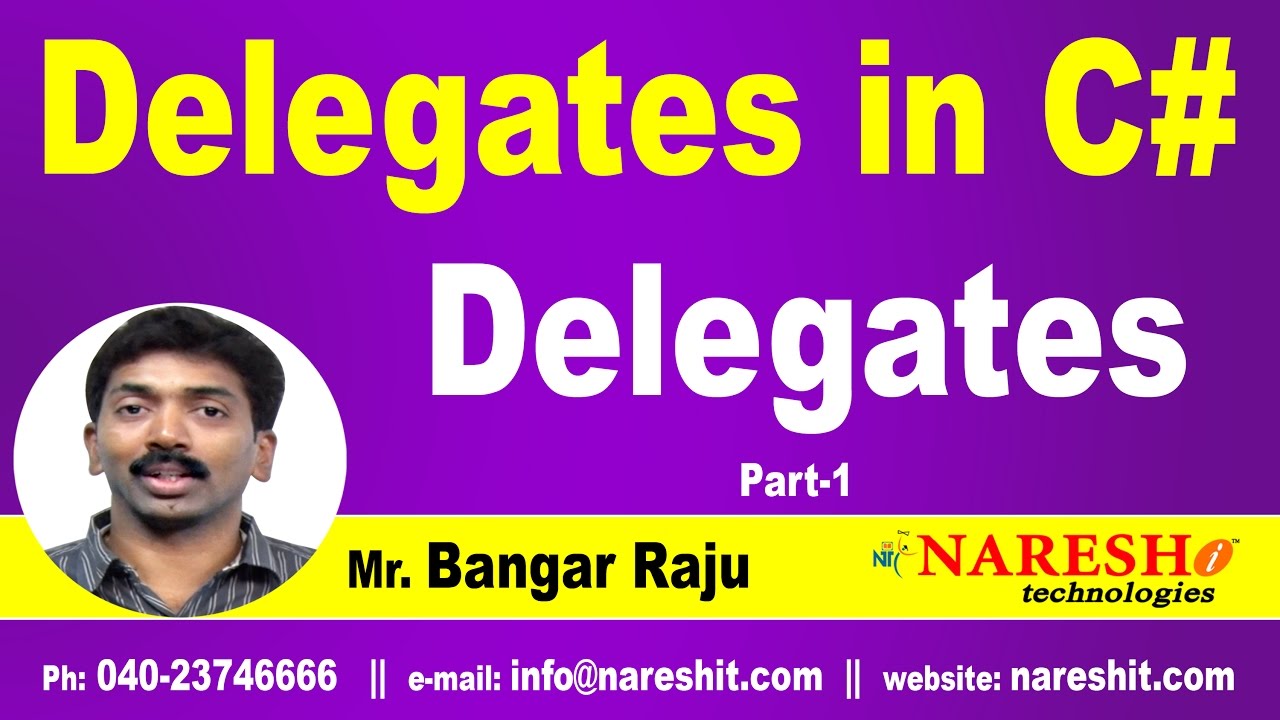
Delegates in C# | Delegates Part 1 | C#.NET Tutorial | Mr. Bangar Raju
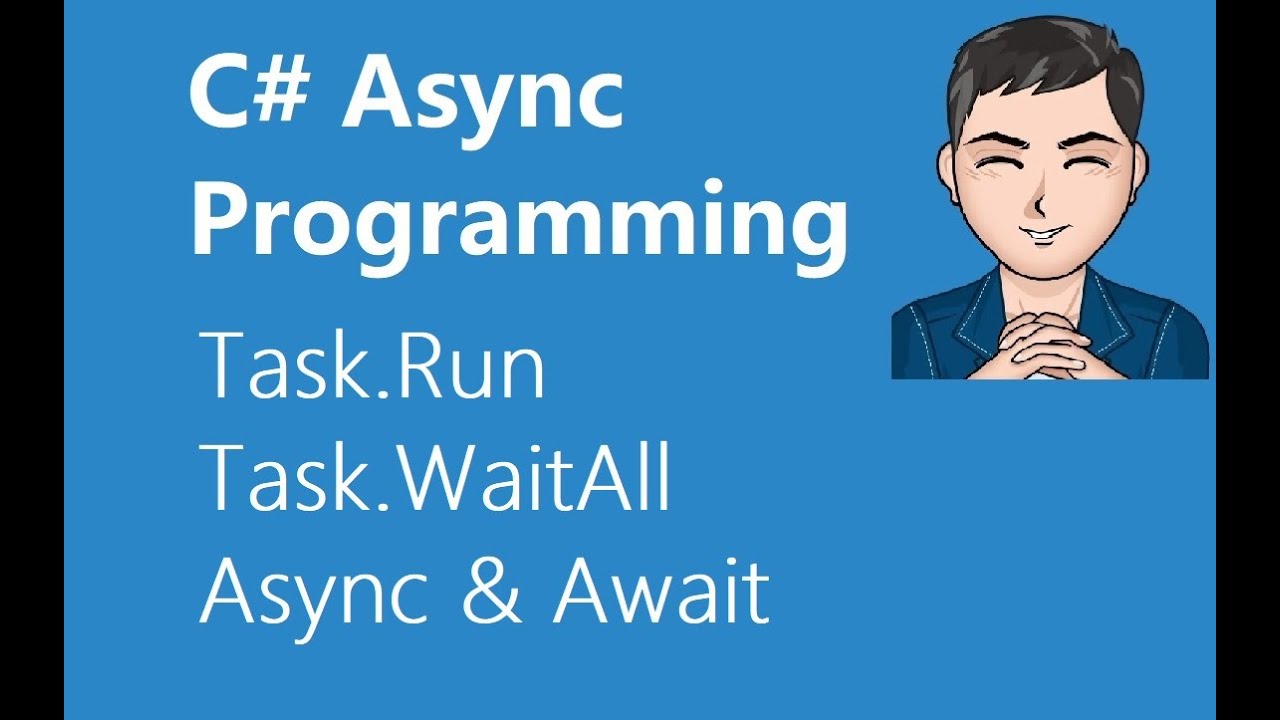
Asynchronous Programming in C# Explained (Task.Run, Task.WaitAll, Async and Await)

Part 1 Introduction || C#.Net Tutorials For Beginners & Experienced || @NehanthWorld
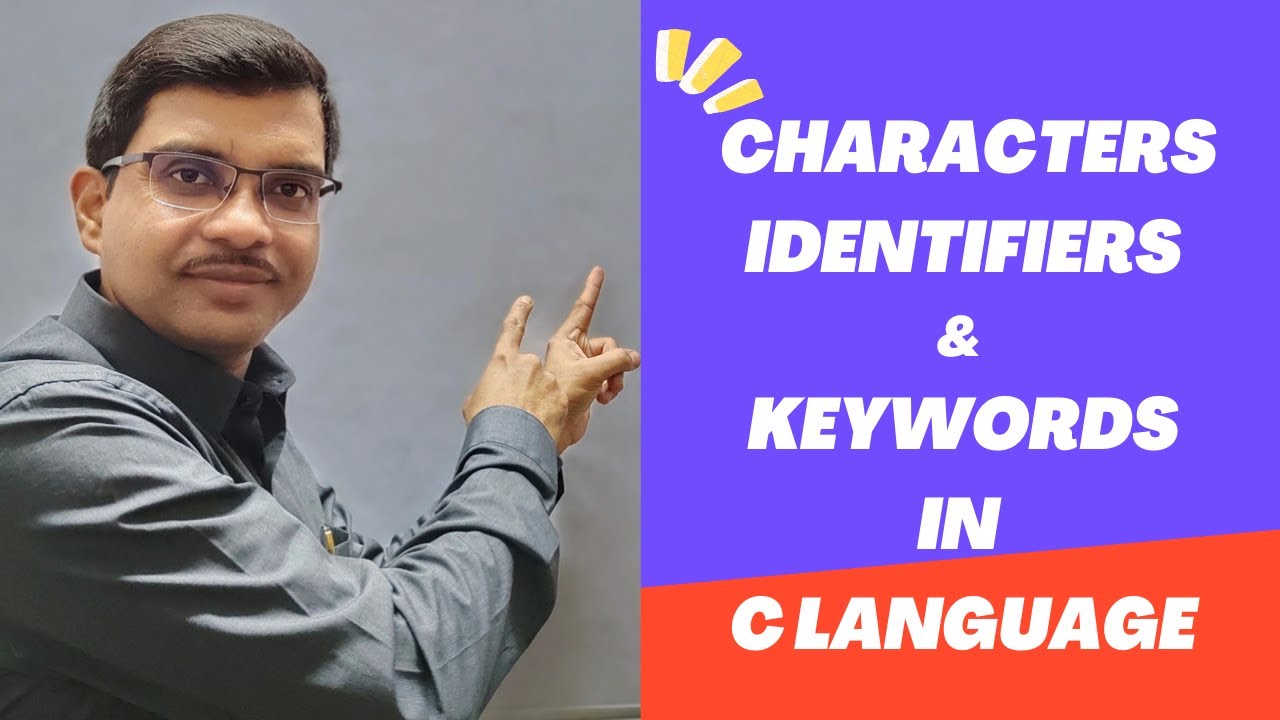
C_08 Characters | Identifiers & Keywords in C Language | C Programming Tutorials
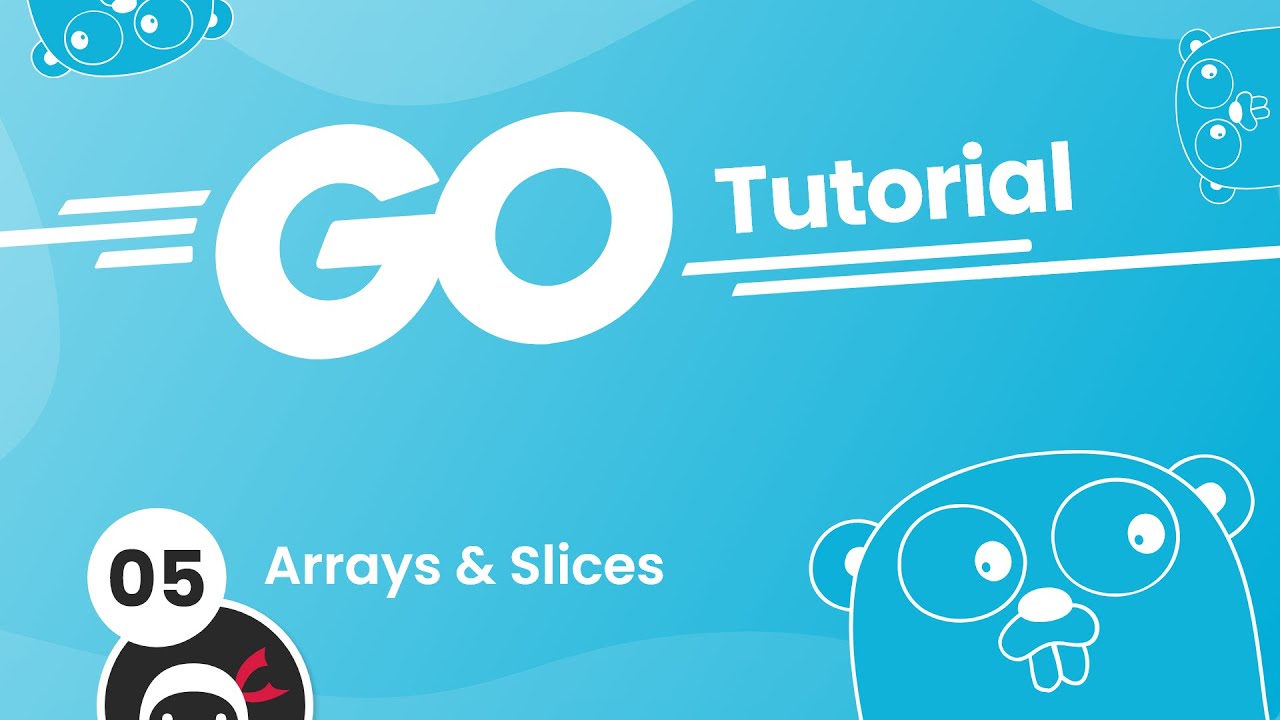
Go (Golang) Tutorial #5 - Arrays & Slices
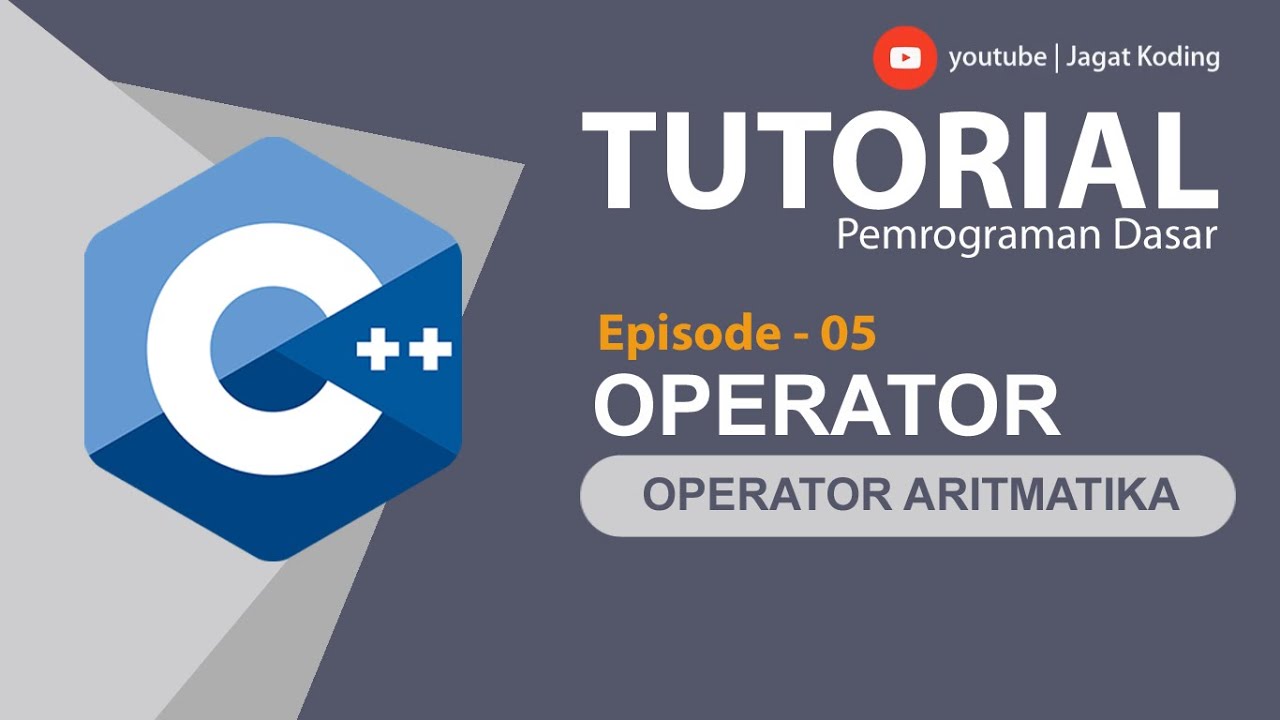
C++ 05 | Operator Aritmatika | Tutorial Dev C++ Indonesia
5.0 / 5 (0 votes)