OOP Principles: Composition vs Inheritance
Summary
TLDRThis course video discusses object-oriented design, focusing on inheritance and composition in Python. It explains that while inheritance is useful for creating subclasses, it can lead to problems when duplicating methods across classes. Composition, however, offers a more flexible solution by building classes through reusable components. The video walks through examples using animals and robots, illustrating how composition can simplify design, allowing for greater modularity and fewer issues compared to inheritance. The key takeaway is to favor composition over inheritance when possible for more maintainable and scalable code.
Takeaways
- ๐ง **Inheritance vs. Composition**: The script discusses the difference between using inheritance and composition in object-oriented design, suggesting that while inheritance is a common approach, composition might often be a better strategy.
- ๐ถ **Starting with Simple Classes**: It begins with creating simple classes for dogs and cats that can bark and meow, respectively, to illustrate the basics of object-oriented programming.
- ๐ฝ๏ธ **Adding Functionality**: The script then addresses the challenge of adding a new method ('eat') to existing classes, which leads to duplication of code and potential for errors.
- ๐ **Inheritance as a Solution**: To solve the duplication issue, inheritance is introduced as a way to create a superclass ('Animal') with a shared method ('eat'), which is inherited by subclasses ('Dog' and 'Cat').
- ๐ค **Expanding to More Complex Classes**: The concept is further expanded to include more complex classes like robots with methods for 'clean' and 'cook', and the same inheritance pattern is applied.
- ๐ง **Inheritance Limitations**: The script highlights the limitations of inheritance, such as the difficulty of managing a class that needs to inherit multiple behaviors (like a robot that needs to 'clean', 'move', 'bark', and 'play games').
- ๐ **Composition to the Rescue**: Composition is introduced as a solution to the limitations of inheritance, allowing a class to include objects from other classes to gain their behaviors.
- ๐พ **Creating a 'Super Bot'**: An example is given of creating a 'Super Bot' class that includes objects from 'Robot', 'Dog', and 'Clean Robot' classes to achieve multiple behaviors without inheritance.
- ๐ ๏ธ **Flexibility in Design**: The script emphasizes the importance of choosing the right design model based on the problem at hand, suggesting that composition provides more flexibility and should be the default choice when possible.
- โ๏ธ **Balance Between Inheritance and Composition**: It concludes with the advice that while inheritance has its place, it should be used judiciously due to its potential to introduce complexity and maintenance challenges.
Q & A
What is the main focus of the video transcript?
-The main focus of the video transcript is on object-oriented design, specifically comparing the use of inheritance and composition in designing software applications.
Why might inheritance not be an ideal design model in some cases?
-Inheritance might not be ideal because it can lead to duplication of methods, and making changes in one subclass could result in inconsistencies or errors in others. It also forces subclasses to inherit everything from the superclass, which may not always be desirable.
What problem does the video illustrate using the example of dogs and cats?
-The video illustrates the problem of method duplication in the example of dogs and cats. Initially, both dog and cat classes have separate methods for eating, barking, and meowing, which leads to duplicated code and potential errors if the methods become out of sync.
How is inheritance used to solve the problem of duplicated methods in the dog and cat example?
-Inheritance is used by creating a superclass called 'Animal' that contains the common 'eat' method. Dog and Cat then become subclasses that inherit the 'eat' method from Animal, avoiding duplication.
What issue arises when designing robots with the inheritance model?
-When adding new behaviors like 'move' to multiple robot classes (such as cook robot and clean robot), the same problem of method duplication occurs. Each robot class needs to implement the 'move' method, which leads to redundancy.
How does the video suggest resolving the robot design problem using inheritance?
-The video suggests creating a superclass called 'Robot' that contains the common 'move' method. Subclasses like cook robot and clean robot inherit the 'move' method from the Robot superclass, eliminating method duplication.
What challenge does the creation of a personal robot pose in the inheritance model?
-The personal robot needs to combine behaviors from different classes, such as moving, cleaning, barking, and playing games. This makes the inheritance model difficult to use effectively because it requires mixing multiple behaviors that don't fit neatly into a single inheritance hierarchy.
What is composition, and how is it different from inheritance?
-Composition is a design model where objects are composed of other objects to share behaviors, rather than inheriting them from a superclass. It is based on a 'has a' relationship, unlike inheritance, which is based on an 'is a' relationship. Composition allows more flexibility by assembling objects with desired behaviors.
How is composition applied to solve the problem of the personal robot in the video?
-Composition is applied by creating individual classes for each behavior, such as barking, moving, and cleaning. These behaviors are then combined in a 'super bot' class, where each method (e.g., move, bark) refers to the corresponding behavior from its respective class, avoiding the rigidity of inheritance.
What is the general advice given about when to use inheritance versus composition?
-The general advice is to use composition when possible and inheritance only when necessary. Composition tends to be simpler and less prone to problems, while inheritance can lead to unwanted dependencies and complexities due to forced inheritance of all behaviors from the superclass.
Outlines
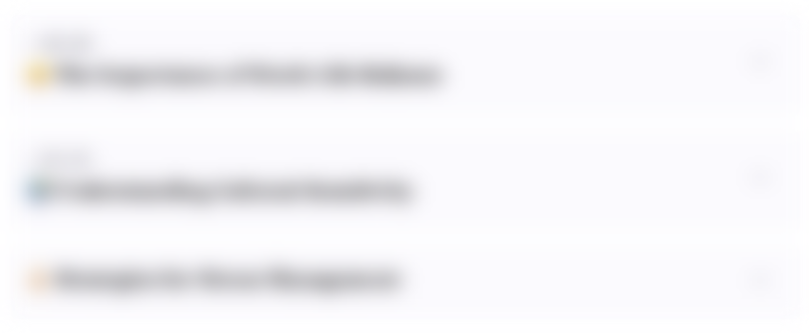
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
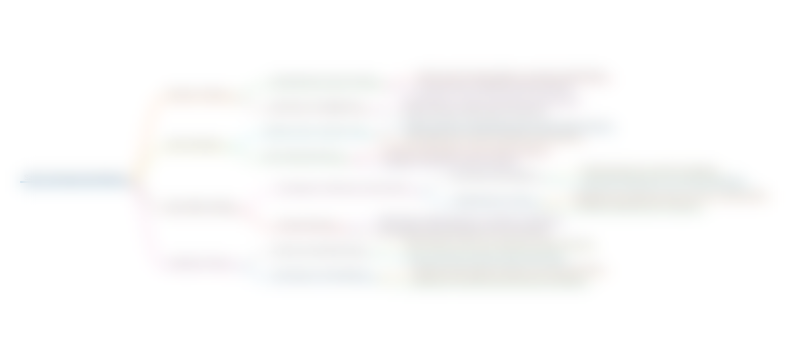
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
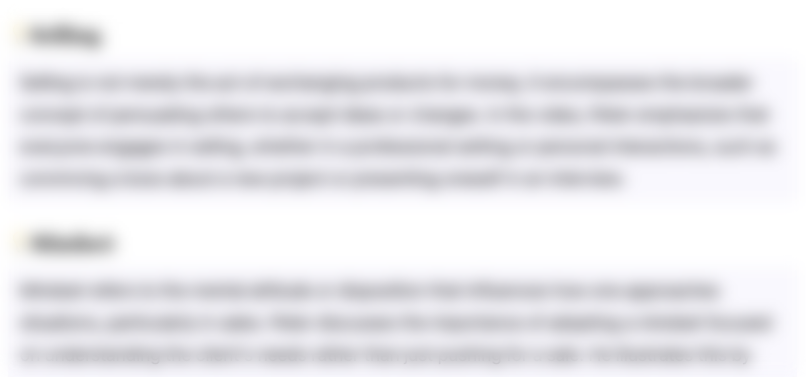
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
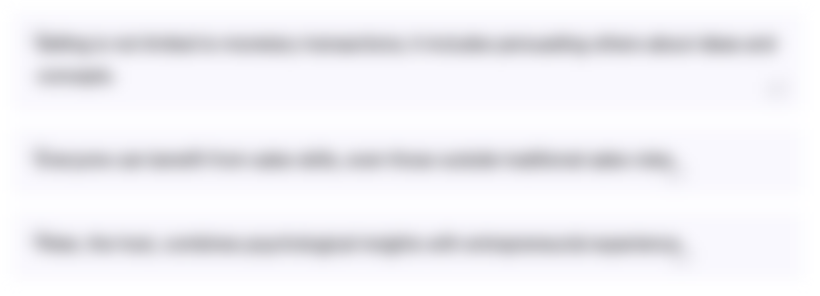
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
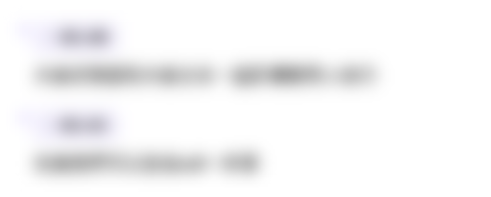
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
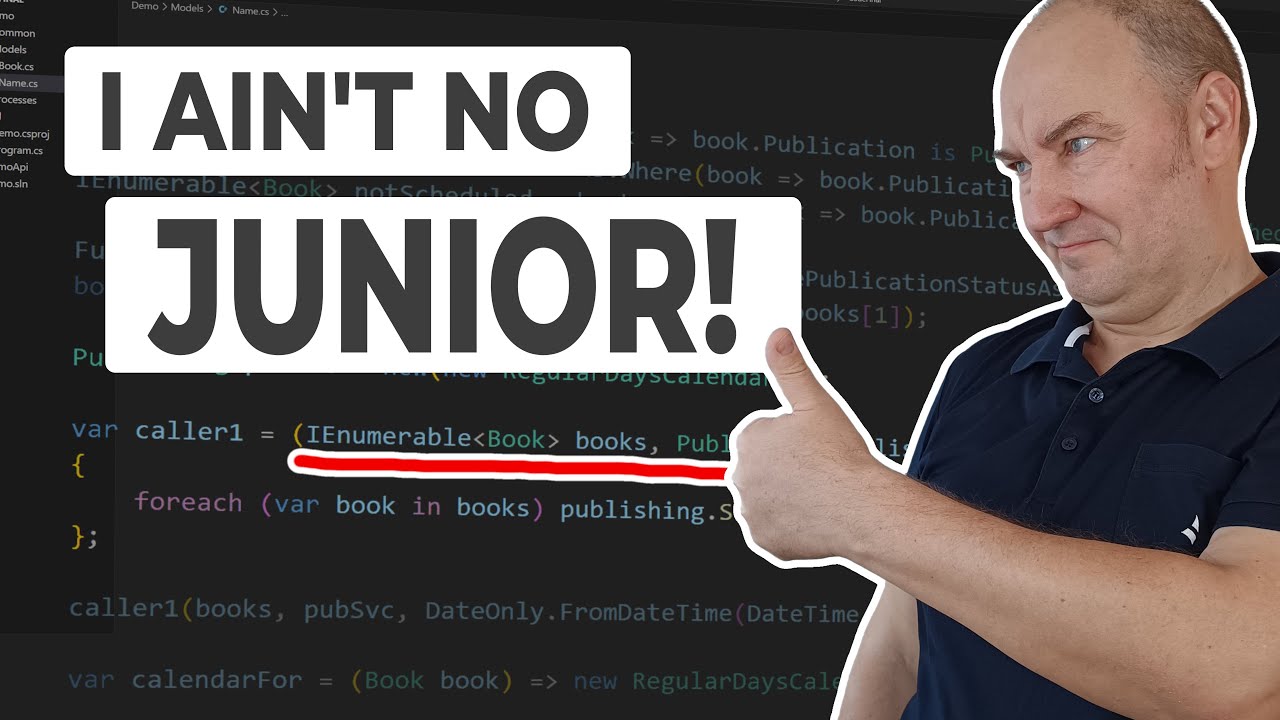
5 Sure Signs You're No Longer Junior Programmer

Fundamental Concepts of Object Oriented Programming
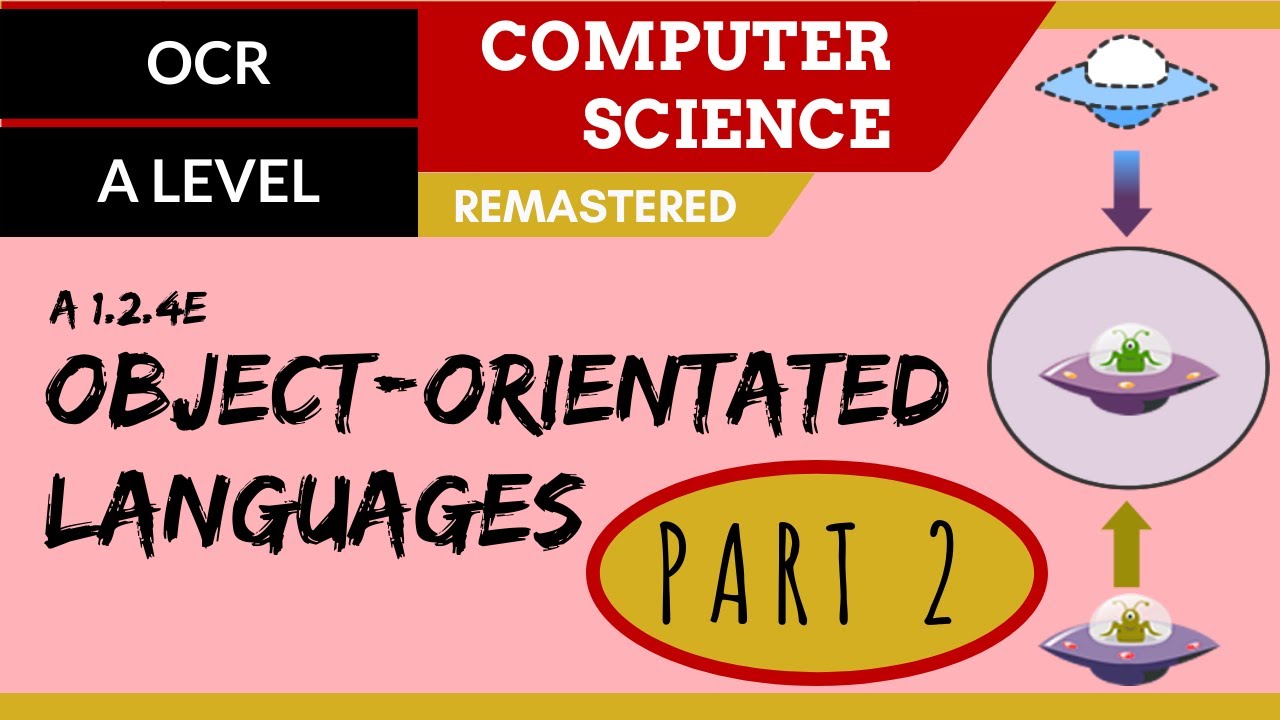
37. OCR A Level (H446) SLR7 - 1.2 Object-oriented languages part 2
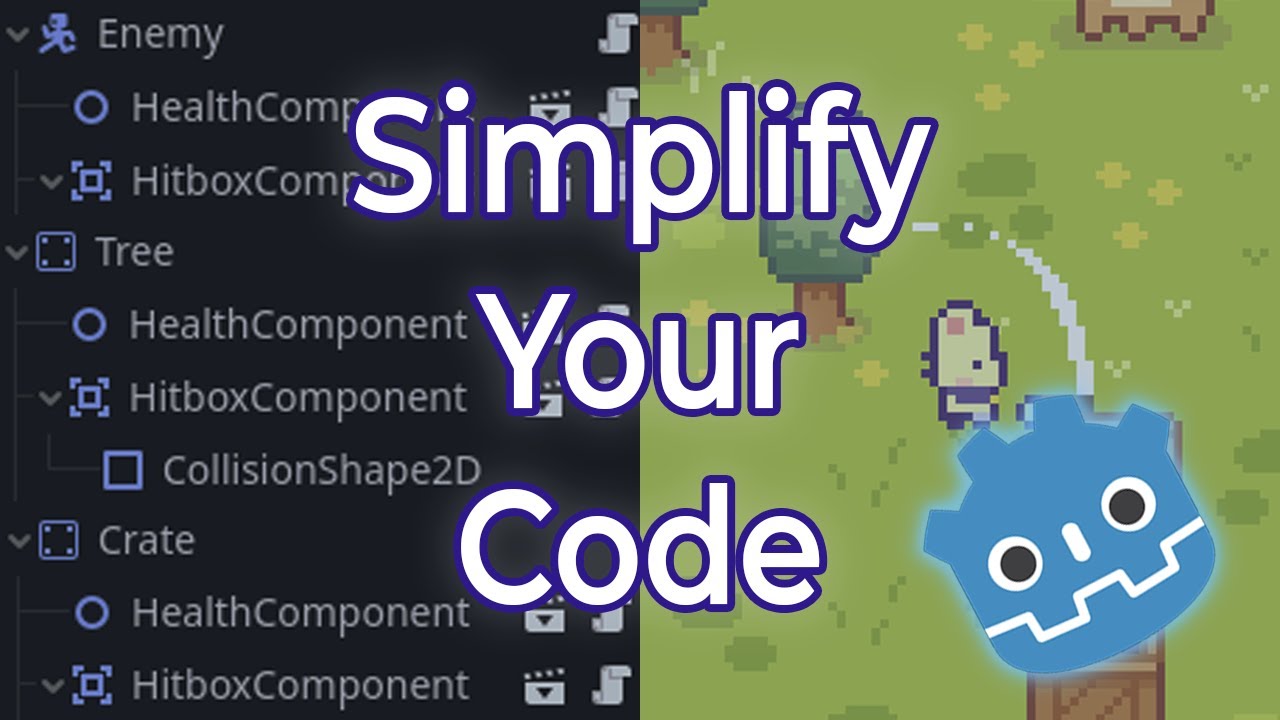
How You Can Easily Make Your Code Simpler in Godot 4
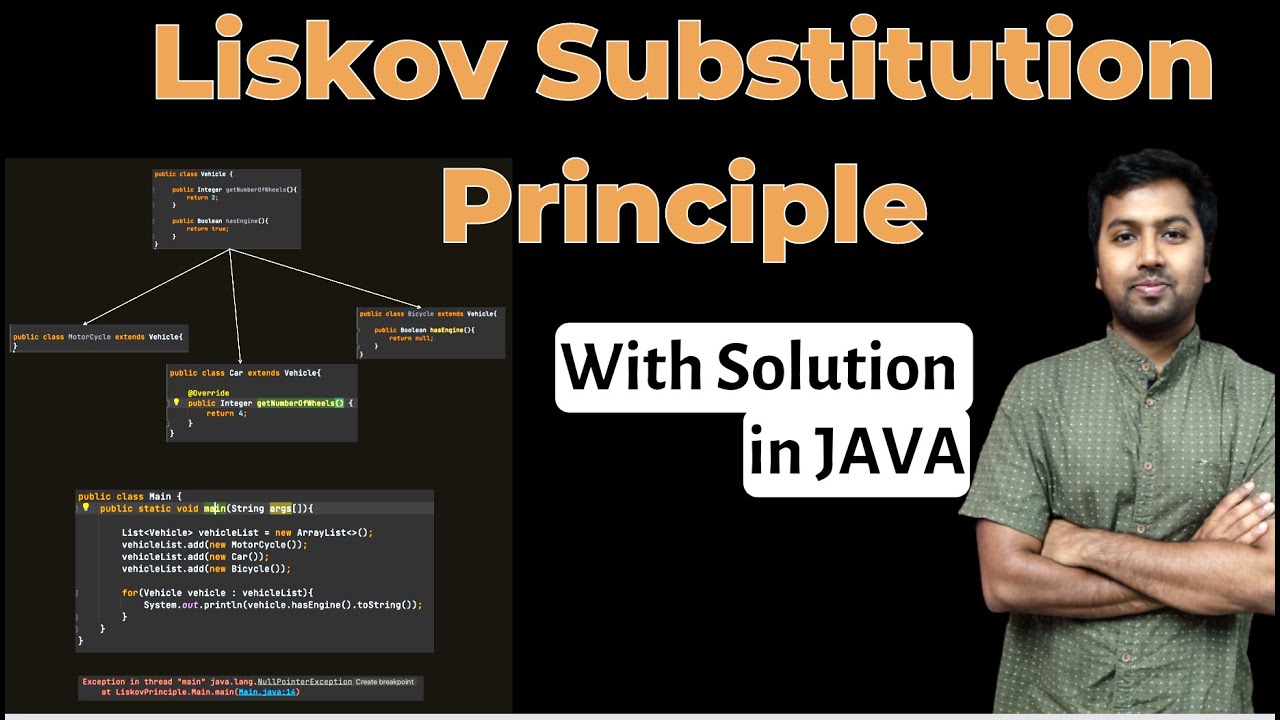
1.1 Liskov Substitution Principle (LSP) with Solution in Java - SOLID Principles of Low Level Design

Object Oriented Programming Using Python [With Examples] - Part 2 | The Knowledge Academy
5.0 / 5 (0 votes)