How To Master React In 2024 (Complete Roadmap)
Summary
TLDRThis comprehensive video offers an exhaustive roadmap for mastering React, guiding viewers through key concepts, from understanding the DOM and dynamic websites to components, state management, hooks, and performance optimization. While acknowledging React's declining popularity for website development, replaced by frameworks like Next.js, the video positions React as a prerequisite for learning Next.js and React Native for mobile app development. It covers essential topics like routing, styling, tooling, forms management, data fetching, and testing, providing a solid foundation for those embarking on the React journey.
Takeaways
- π Understand the DOM and dynamic websites to appreciate the value React brings for building modern web applications.
- π§ Comprehend React's underlying reconciliation process and how it works under the hood to manage dynamic user interfaces.
- π Start with creating basic React apps (like a todo list or calculator) to understand how React components wrap HTML, CSS, and JavaScript.
- π§± Master React fundamentals: components, state, rendering, and props - the core building blocks of React applications.
- π Learn React hooks (useState, useEffect, useMemo, useCallback, useRef) to manage state and side effects in functional components.
- π§° Create custom hooks to encapsulate reusable stateful logic and separate concerns in your codebase.
- π Grasp concepts like prop drilling, context API, and suspense API to manage and optimize state across components.
- π¦ Explore state management tools like Redux, Recoil, or Zustand for better state management in larger applications.
- π Implement routing in your React apps to handle multiple pages and views using libraries like React Router.
- π Learn styling techniques and libraries like Tailwind CSS, Material UI, or Styled Components to build visually appealing UIs.
Q & A
What is the purpose of this video?
-The purpose of this video is to provide a comprehensive roadmap for learning React, covering various topics that are essential for getting started with React development.
Why is it important to understand the DOM and dynamic websites before learning React?
-Understanding the DOM and dynamic websites is crucial because it helps you grasp the challenges and problems that React solves, and why it was adopted so quickly. It also provides an intuition for the difficulties of manipulating the DOM directly, which React aims to simplify.
What is reconciliation, and why is it important to understand how React works under the hood?
-Reconciliation is the process by which React updates the DOM efficiently. Understanding how React works under the hood, including reconciliation, is important because it helps you comprehend the principles and mechanisms behind React's performance and functionality.
What is the significance of building a basic React application and analyzing the output?
-Building a basic React application and analyzing the output helps you understand that React is ultimately generating HTML, CSS, and JavaScript code, similar to what you would write manually. It reinforces the concept that React is a tool to simplify and streamline the process of creating dynamic web applications.
What are the four key concepts in React that need to be understood?
-The four key concepts in React that need to be understood are components, state, rendering, and props. Understanding these concepts is crucial for creating React applications and grasping the power and principles behind React.
What is the purpose of hooks in React, and why are they important?
-Hooks in React provide a way to tap into lifecycle events and handle side effects in functional components. They are important because they allow developers to write more concise and reusable code, and enable the use of state and other React features in functional components.
What is prop drilling, and how can it be addressed in React applications?
-Prop drilling is an anti-pattern in React where state is passed down through multiple levels of components. It can be addressed by using the Context API provided by React or by implementing a state management solution like Redux or Recoil.
Why is routing important in React applications, and what are some popular routing libraries?
-Routing is important in React applications because it allows for handling multiple pages or views within a single-page application. Some popular routing libraries for React include React Router, Next.js (which includes routing), and Reach Router.
What are some popular styling libraries for React applications?
-Some popular styling libraries for React applications include Tailwind CSS, Material-UI, Styled Components, Emotion, and Chakra UI.
What are some performance improvement techniques and libraries mentioned in the video?
-The video mentions using libraries like React Hook Form and Formik for optimizing form management, libraries like SWR and React Query for efficient data fetching, and potentially writing tests for improved code quality and maintainability.
Outlines
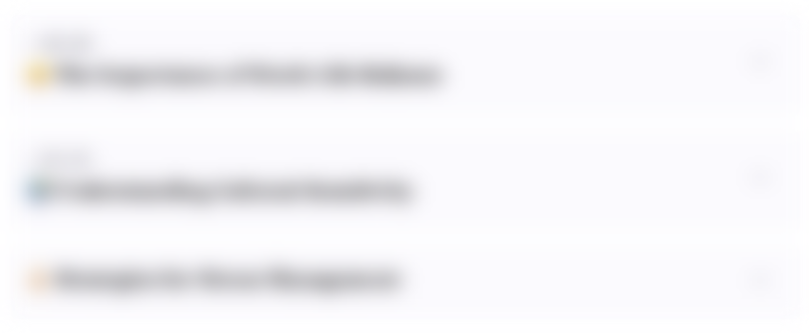
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
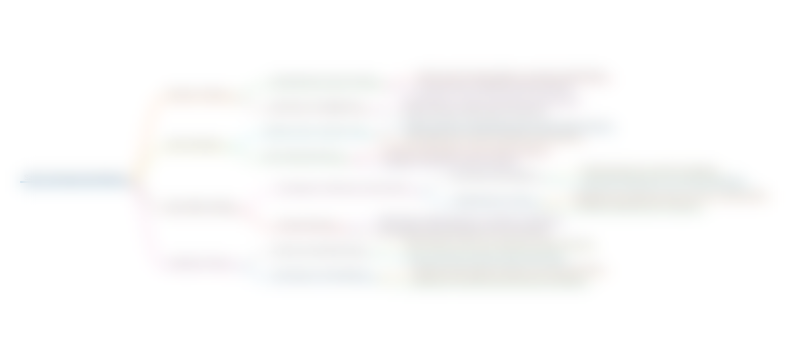
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
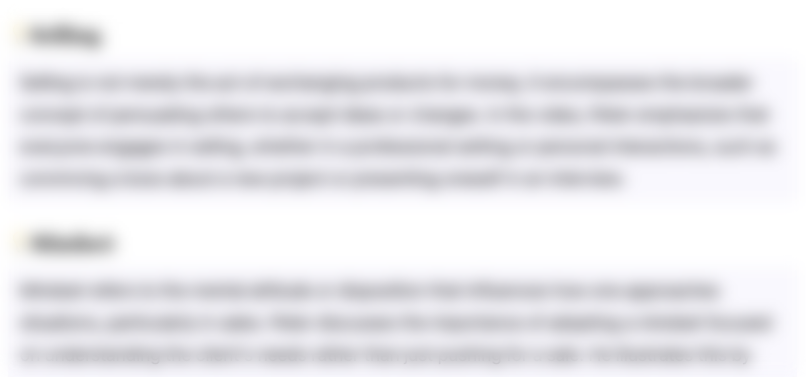
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
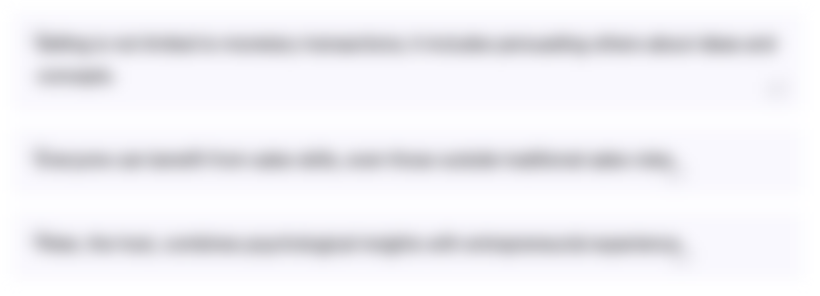
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
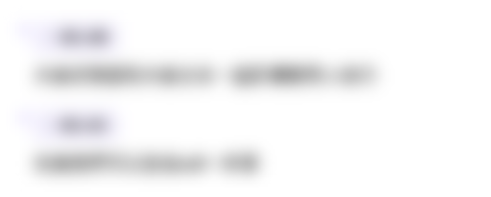
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)