How Rendering Works: Overview | Lecture 123 | React.JS π₯
Summary
TLDRThis video script provides an insightful overview of how React renders applications behind the scenes. It delves into the three phases involved in displaying components on the screen: the render phase, where React calls component functions and figures out how to update the DOM; the commit phase, where React actually updates the DOM; and the browser repaint phase, where visual changes are displayed. The script clarifies the distinction between React's definition of 'rendering' and the traditional understanding, emphasizing that rendering in React is an internal process without visual changes. It also explores how renders are triggered and the importance of understanding the entire rendering process for a comprehensive grasp of React's inner workings.
Takeaways
- π§© React applications are built with components, which can be nested and instantiated multiple times.
- π JSX code in components produces React.createElement calls, which create React elements representing the UI.
- π React elements are eventually transformed into DOM elements and rendered on the screen.
- π React's rendering process is triggered by state updates or the initial application load.
- π The rendering process has three phases: render, commit, and browser repaint.
- π¨ In the render phase, React calls component functions to determine how to update the DOM, but doesn't actually update it.
- βοΈ In the commit phase, React updates the DOM to reflect the latest state changes.
- π» The browser then repaints the screen to display the visual changes.
- β±οΈ Renders are not triggered immediately after state updates, but are scheduled when the JavaScript engine has free time.
- π In some cases, like multiple setState calls in the same function, renders are batched together.
Q & A
What is the main purpose of this lecture series?
-This lecture series aims to provide an in-depth understanding of how React renders applications behind the scenes. It is divided into three parts, with this video serving as an overview and the next two videos going into the React internals in greater detail.
What is the difference between a component and a component instance in React?
-A component is a reusable piece of code that defines the structure and behavior of a part of the user interface. A component instance is the actual physical representation of a component in the application, holding its own state and props.
What role do React elements play in the rendering process?
-React elements are the output of the JSX code, produced by calling the React.createElement function for each component instance. These React elements are then transformed into DOM elements and displayed as the user interface on the screen.
What is the purpose of the render phase in React?
-In the render phase, React calls the component functions and figures out how to update the DOM to reflect the latest state changes. However, it does not actually update the DOM during this phase.
How does React's definition of rendering differ from the traditional understanding?
-In React, rendering refers to the internal process of calling component functions and determining DOM updates, not the actual updating of the DOM or displaying elements on the screen. This differs from the traditional understanding of rendering as displaying elements visually.
When does the actual DOM update happen in React?
-The actual DOM update happens in the commit phase, after the render phase. During the commit phase, new elements may be added to the DOM, existing elements may be updated, or elements may be removed to reflect the current state of the application.
What are the two ways in which a render can be triggered in React?
-A render can be triggered in React in two ways: the initial render when the application first runs, and a re-render triggered by a state update in one or more component instances.
Does React trigger a render immediately after a state update?
-No, React does not trigger a render immediately after a state update. Instead, the render is scheduled to occur when the JavaScript engine has some free time, usually just a few milliseconds later.
What is the significance of batching renders in React?
-In some situations, such as multiple setState calls in the same function, React will batch renders together. This means that instead of triggering a render for each state update, React will combine them into a single render, improving performance.
What is the key takeaway from this lecture?
-The key takeaway from this lecture is that the rendering process in React is more complex than it initially appears. It involves multiple phases, including the render phase, where component functions are called and DOM updates are determined, and the commit phase, where the actual DOM updates occur.
Outlines
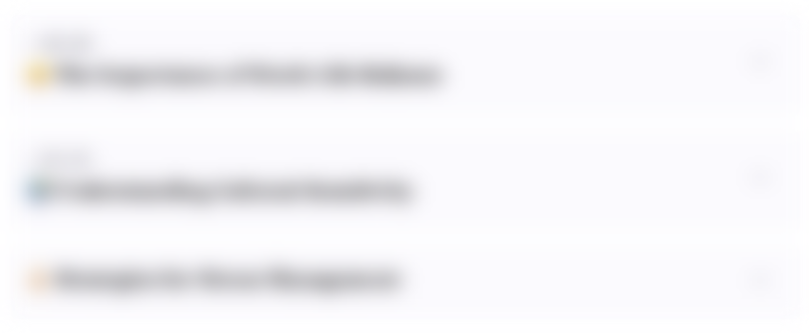
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
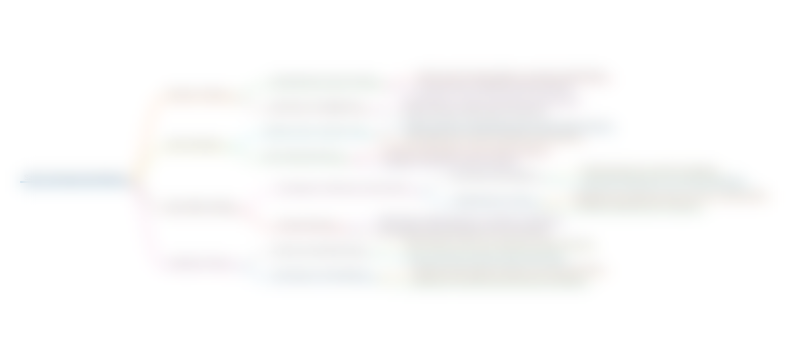
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
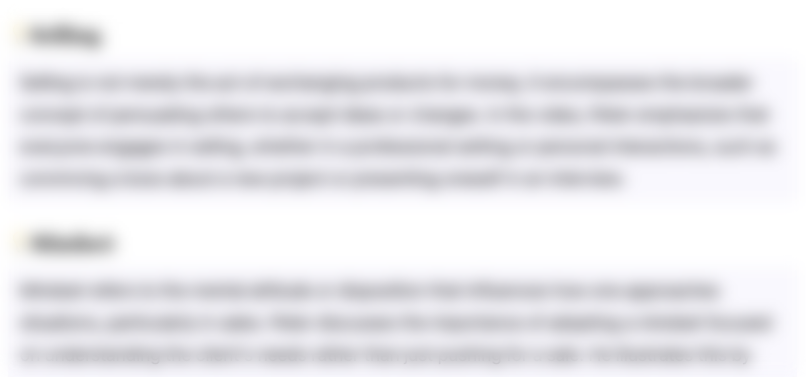
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
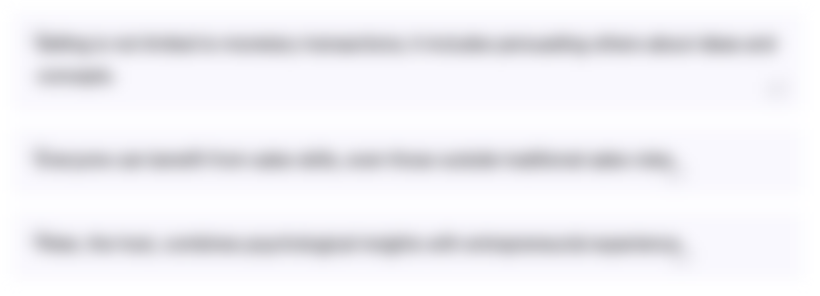
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
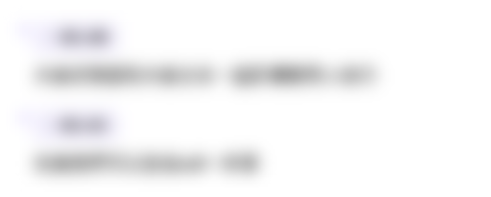
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

How Rendering Works: The Render Phase | Lecture 124 | React.JS π₯

Components, Instances, and Elements | Lecture 121 | React.JS π₯

Instances and Elements in Practice | Lecture 122 | React.JS π₯

Performance Optimization and Wasted Renders | Lecture 239 | React.JS π₯

How Events Work in React | Lecture 134 | React.JS π₯
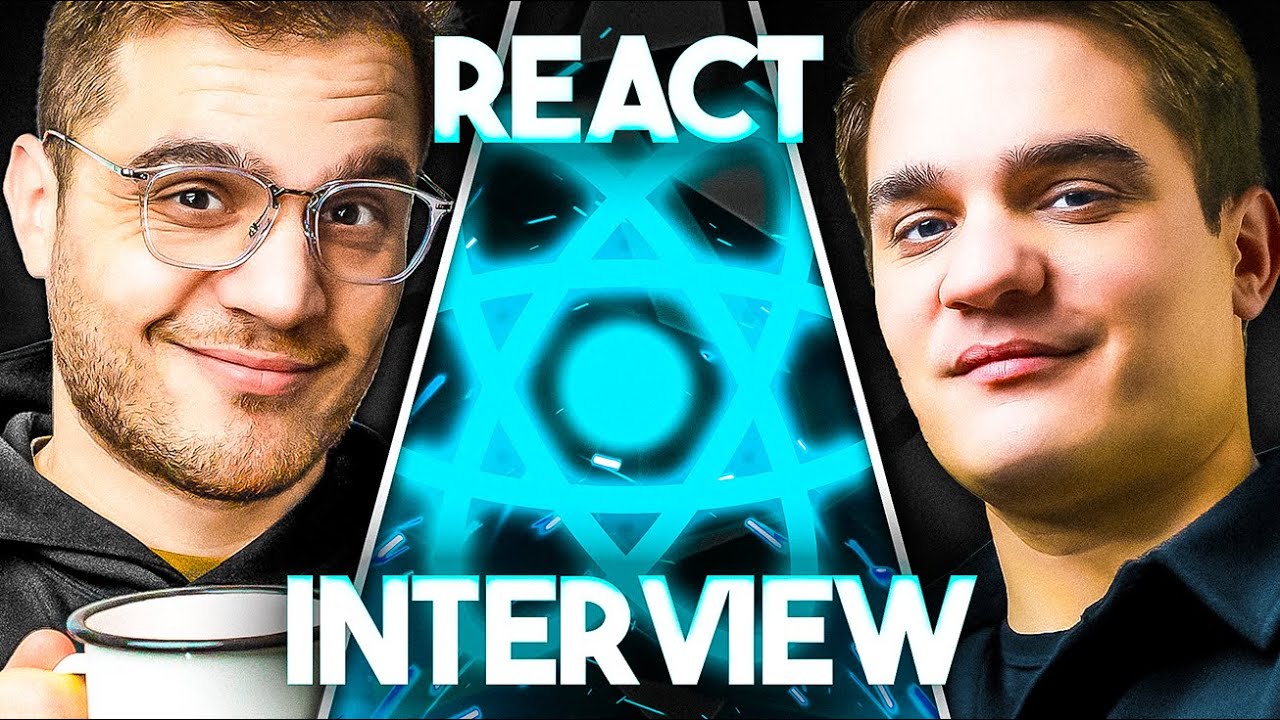
React Interview Questions Senior Level (React Fiber, Reconciliation, Virtual DOM)
5.0 / 5 (0 votes)