The Ultimate API Showdown: Server Actions, tRPC, GraphQL, and REST Compared!
Summary
TLDRThis video provides a comprehensive comparison of four different paradigms for handling server-side operations in web development: Server Actions, tRPC (TypeSafe Remote Procedure Calls), GraphQL, and REST. The video analyzes various aspects, including ease of setup, ease of use, handling mutations and queries, type safety, compatibility with other clients, and the ability to work without JavaScript enabled. It offers insights into the advantages and disadvantages of each approach, helping developers choose the most suitable option based on their project's requirements. The video ultimately recommends Server Actions for simple web applications, tRPC for supporting mobile or desktop clients, and GraphQL as a viable choice for internal applications, while cautioning against exposing it to the open internet due to potential denial-of-service vulnerabilities.
Takeaways
- 🔑 Server Actions, tRPC, GraphQL, and REST are four different paradigms for handling client-server communication.
- 🚀 Server Actions are incredibly easy to set up and use in Next.js 14, but lack robust query capabilities.
- 🔐 tRPC and GraphQL excel at handling queries, with tRPC being easier to set up and GraphQL providing better type safety through introspection.
- 📝 Mutations are straightforward with Server Actions, tRPC, and GraphQL, while REST allows more flexibility but lacks standardization.
- 🧩 Server Actions have limited compatibility with non-web clients, while tRPC and GraphQL offer better interoperability.
- 🔌 Only Server Actions can work without JavaScript enabled on the client.
- 🏆 For simple web apps, a combination of Server Actions and React Server Components is recommended.
- 📱 For apps with mobile or desktop clients, tRPC or GraphQL (for internal apps) are better options.
- ⚠️ GraphQL has potential for Denial of Service issues and should be used cautiously on the open internet.
- 🤔 The choice between these paradigms depends on factors like app complexity, client types, and security requirements.
Q & A
What is the purpose of this video script?
-The purpose of this video script is to compare different paradigms for server-client communication, such as server actions, tRPC, GraphQL, and REST, and provide insights into which might be the best choice for a particular project.
What are server actions?
-Server actions are functions defined on the server side that can be invoked from the client. In Next.js 14, they are defined using the `use server` pragma, and the framework manages the communication between the client and server.
What is tRPC (TypeSafe Remote Procedure Calls)?
-tRPC is a protocol that allows defining remote procedure calls that can be exposed by the server. It automatically creates query hooks and handles the marshalling between the API endpoint and the function, sitting on top of HTTP and JSON.
What is GraphQL?
-GraphQL is a query language created by Meta (formerly Facebook) that sits on top of HTTP and JSON. It allows specifying queries or mutations using a well-defined syntax, and the server returns the requested data in JSON format.
What is REST (Representational State Transfer)?
-REST is an architectural style where entities are defined in a hierarchical structure, but it doesn't have strict standards or formality. It relies on different styles and formats for APIs, with tools like OpenAPI and Swagger providing some standardization.
Which paradigm is the easiest to set up?
-According to the script, server actions are the easiest to set up, as they are built into Next.js 14 and require no additional configuration.
Which paradigm is the most type-safe?
-The script suggests that GraphQL has an advantage in terms of type safety due to its introspection capability, which allows clients to request the schema from the server and generate types accordingly.
What is the potential issue with GraphQL in terms of queries?
-The script mentions that with GraphQL, clients can make up any query against the server, which can lead to a denial of service attack if a malicious API request consumes excessive database or server resources.
Which paradigm is the most compatible with different clients (e.g., mobile, desktop)?
-According to the script, GraphQL is the most compatible paradigm due to its standardized format, which allows libraries and clients in different languages and platforms to consume GraphQL APIs efficiently.
What is the recommendation for simple Next.js web applications?
-For simple Next.js web applications, the script recommends using a combination of queries on the server in React Server Components and server actions, as it is the easiest way to go.
Outlines
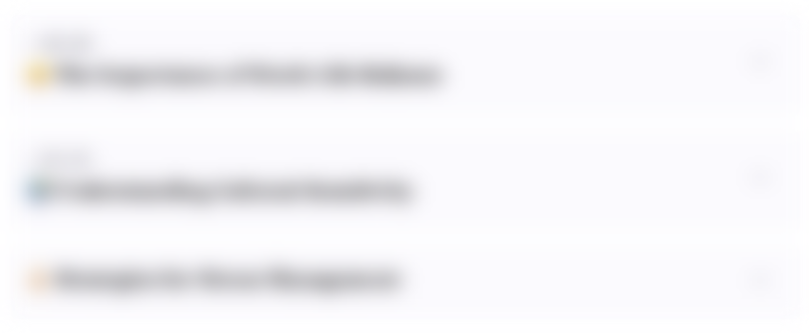
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
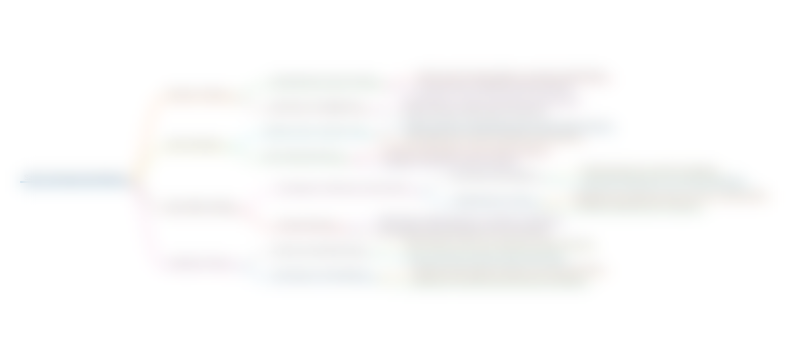
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
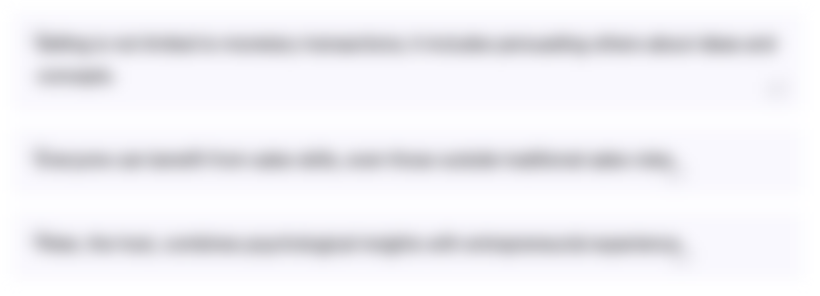
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
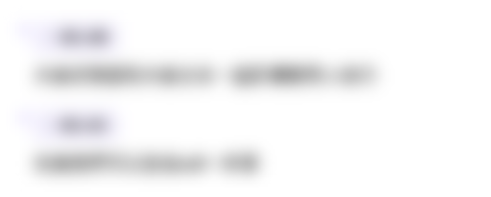
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)