Svelte Tutorial - 10 - List Rendering
Summary
TLDRThis video script teaches how to display lists in web applications using Svelte's 'each' block. It demonstrates iterating over arrays of strings and objects to render HTML elements dynamically. The script covers basic syntax, using aliases for current items, and optionally displaying indices. It also touches on iterating over arrays of objects, accessing properties like first and last names, and hints at the next topic: using keys for lists in Svelte.
Takeaways
- 😀 Building web applications often requires displaying lists of items, such as names, products, or courses.
- 🔄 In Svelte, the 'each' block is used to repeat HTML for each item in a list.
- 📝 The syntax for an 'each' block involves using curly braces, a hash symbol, and the block name, followed by an alias for the current item.
- 💡 To display a list of names, define an array in the script section and use the 'each' block to iterate over it, binding each name to the HTML.
- 🔑 The 'each' block syntax includes an alias for the current item and optionally the index, which can be used for additional context.
- 🌐 When rendering a list, the 'each' block will iterate as many times as there are items in the array, binding the current item to the HTML each time.
- 📑 If inspecting the rendered HTML, you'll see the element repeated for each item in the array, with the inner text reflecting the current item.
- 🔢 For lists with an index, the index is provided by Svelte and can be displayed alongside the item.
- 🏷 Iterating over an array of objects is similar, but you access properties like 'first' and 'last' names using dot notation on the alias.
- 🔑 The 'each' block can also include a 'key' expression for more efficient re-rendering of lists with dynamic content.
Q & A
What is the purpose of using the 'each' block in Svelte?
-The 'each' block in Svelte is used to iterate over a list of items, such as an array, and repeat a block of HTML for each item in the list.
How do you define an array of strings in Svelte's script section?
-You define an array of strings in Svelte's script section by using the `const` keyword followed by the array name and its values enclosed in square brackets, e.g., `const names = ['Bruce', 'Clark', 'Diana'];`.
What is the syntax for starting an 'each' block in Svelte?
-The syntax for starting an 'each' block in Svelte is `{#each arrayName as alias}` where `arrayName` is the name of the array you want to iterate over and `alias` is the variable to refer to the current item in the loop.
How do you end an 'each' block in Svelte?
-An 'each' block in Svelte is ended with `{/each}` followed by the name of the block if necessary.
What does the 'as' keyword do in the 'each' block syntax?
-The 'as' keyword in the 'each' block syntax is used to create an alias for the current item in the array during each iteration of the loop.
How can you display the items of an array in the UI using Svelte?
-You can display the items of an array in the UI by binding the alias to the HTML elements within the 'each' block, allowing the same HTML structure to be repeated for each item.
Can you access the index of the current item in an 'each' block iteration?
-Yes, in Svelte, you can access the index of the current item by using the syntax `{#each arrayName as item, index}`.
What happens if you want to iterate over an array of objects in Svelte?
-When iterating over an array of objects, you can access the properties of each object using the alias and the property names, e.g., `{#each fullNames as name (index)}` where `name.first` and `name.last` can be used to access the first name and last name properties.
How can you display the index of items in an 'each' block iteration?
-You can display the index of items in an 'each' block iteration by binding the index to an HTML element within the 'each' block, e.g., `{#each names as name (index)}` and then using `{index + 1}` to display the index starting from 1.
What is the key expression in the 'each' block, and what is its purpose?
-The key expression in the 'each' block is used to provide a unique identifier for each item in the array, which helps Svelte efficiently update the DOM when the list changes. It is mentioned as a topic for the next video in the script.
Outlines
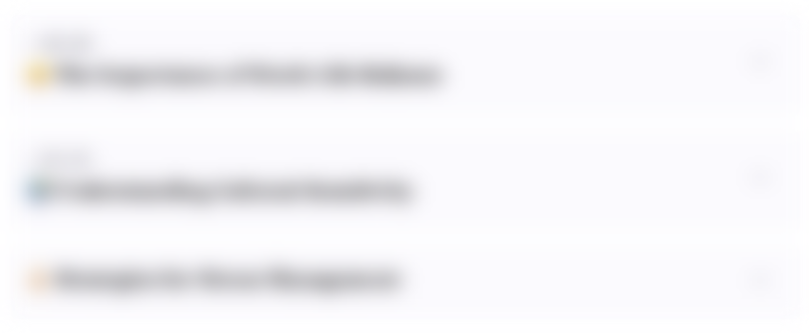
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
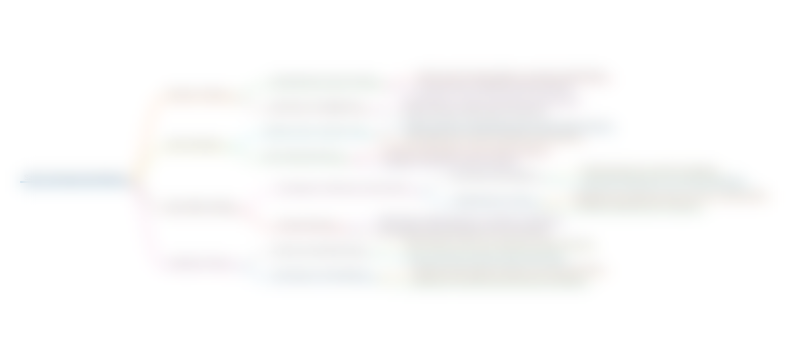
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
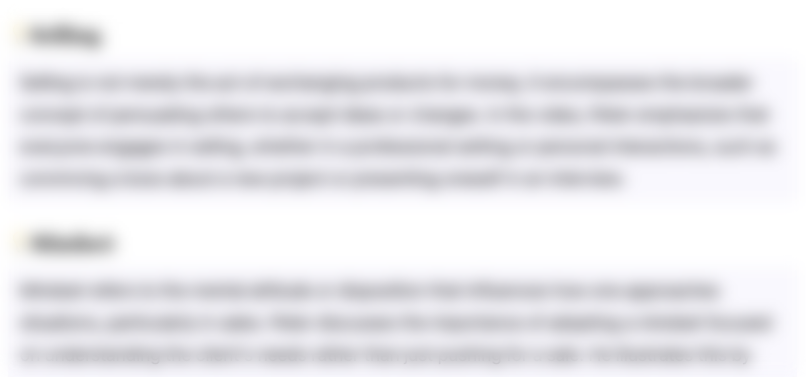
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
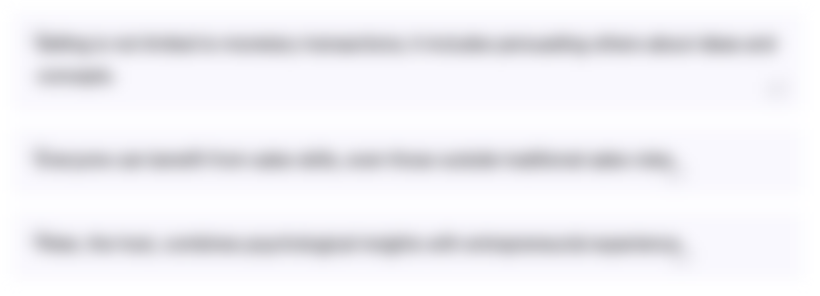
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
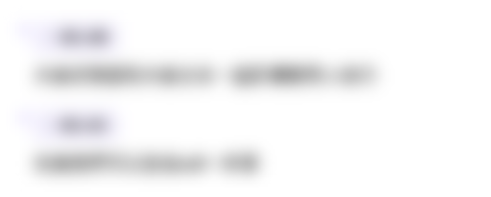
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

CSS Display Property | Sigma Web Development Course - Tutorial #23
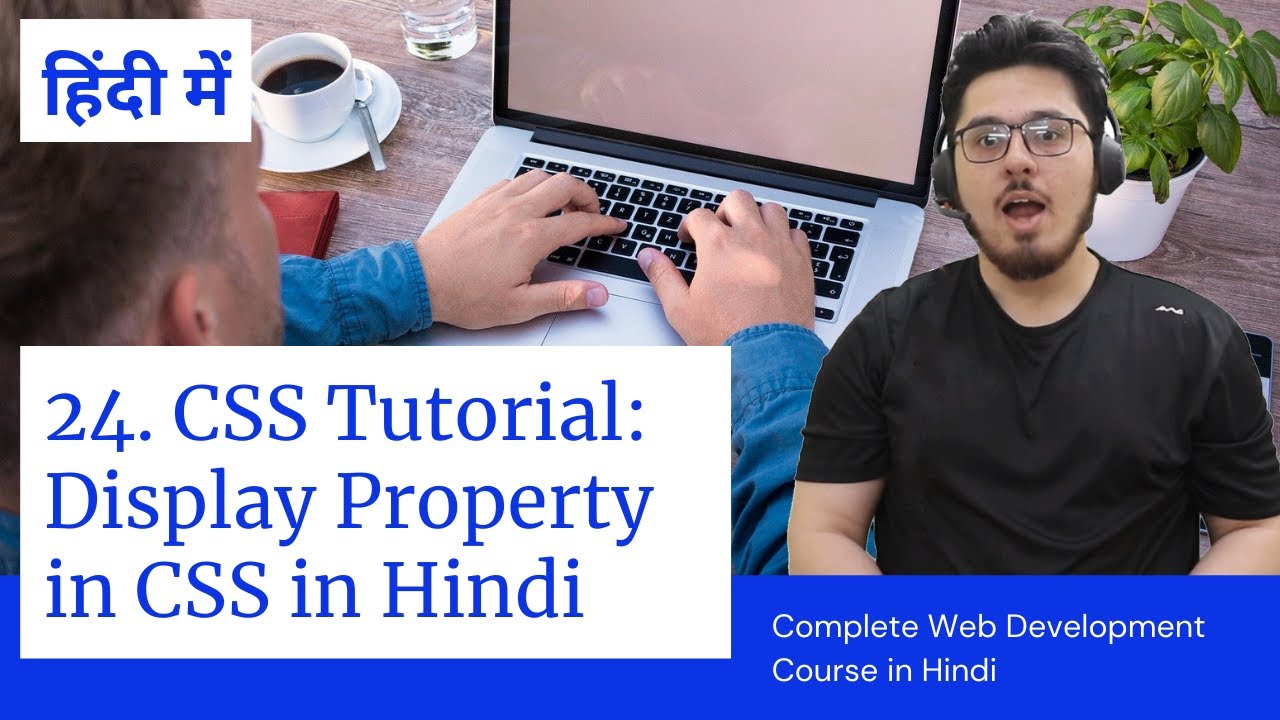
CSS Tutorial: CSS Display Property | Web Development Tutorials #24
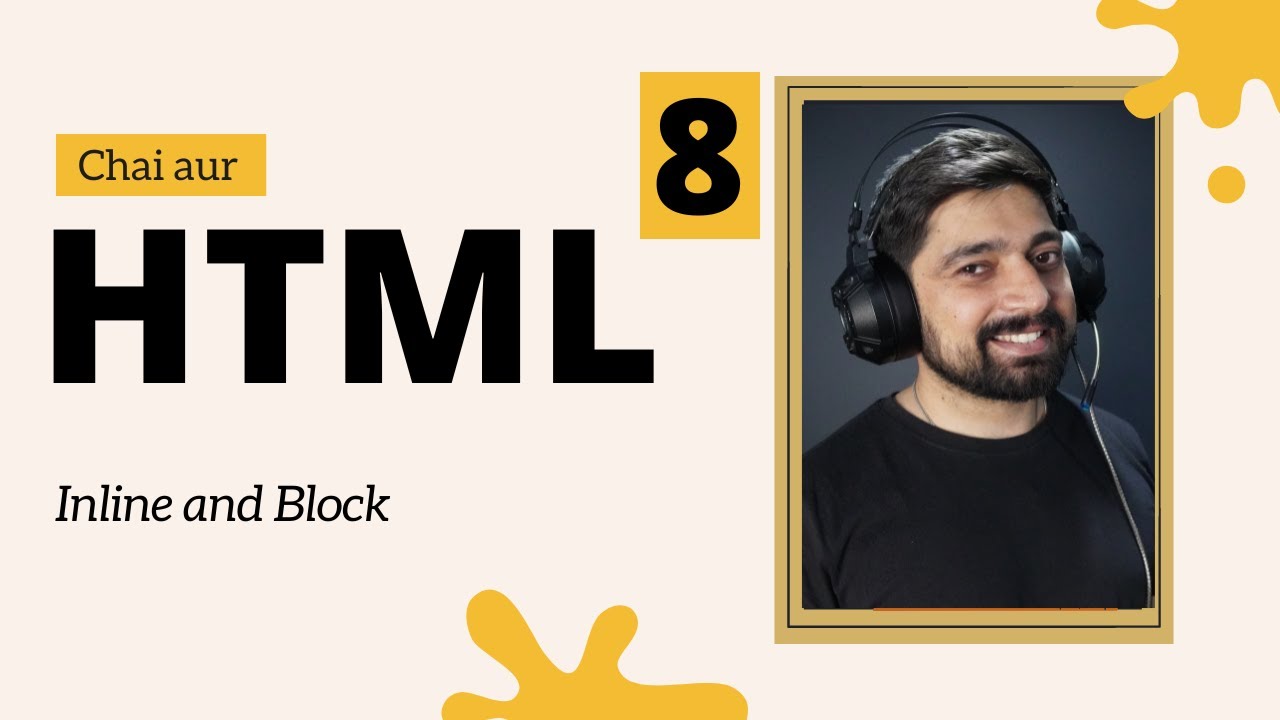
List, inline and Block element

Curso HTML Completo: Aula 10 - Listas

Mengkonfigurasi Sistem Keamanan Jaringan - Teknik Komputer dan Jaringan
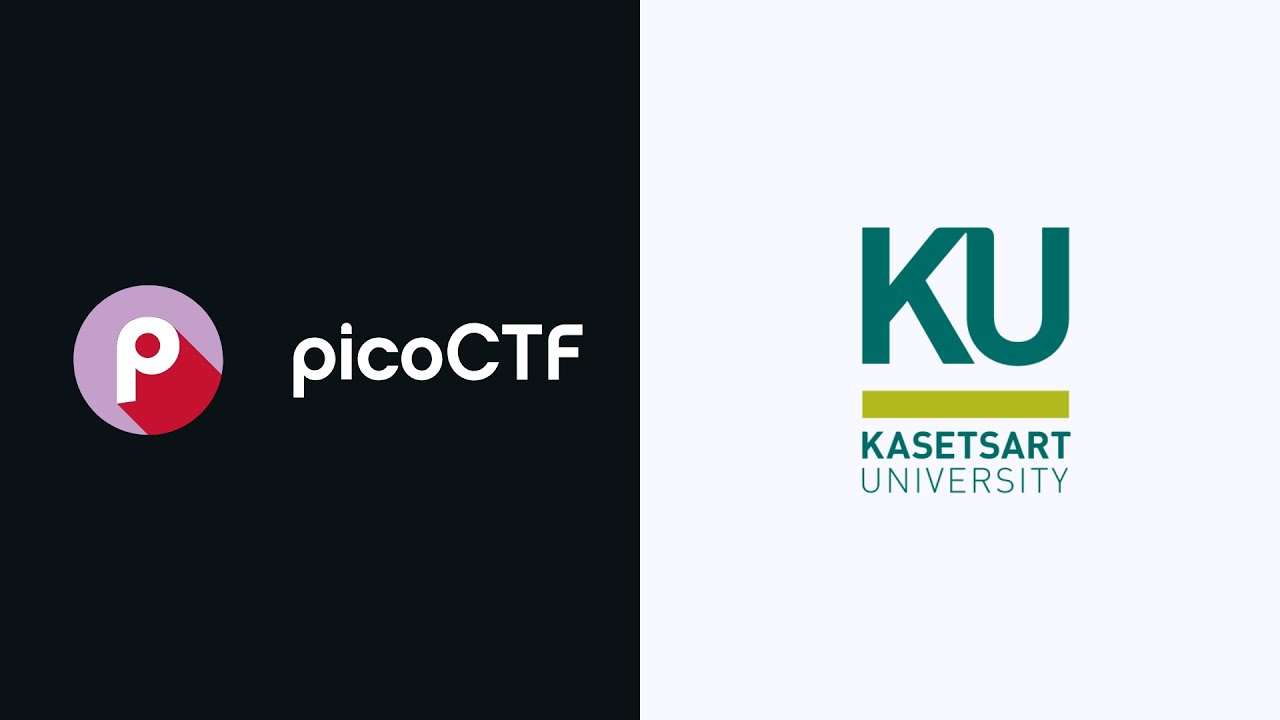
พาตะลุยโจทย์ picoCTF หมวดหมู่ Web Exploitation
5.0 / 5 (0 votes)