Arithmetic Overflow and Underflow | Hack Solidity (0.6)
Summary
TLDRThis video tutorial delves into the concept of overflow and underflow in smart contracts, particularly using the example of a time-locked contract vulnerable to such issues. It demonstrates how overflow can allow unauthorized early withdrawal by manipulating contract time, and introduces the Safe Math library by OpenZeppelin to prevent such exploits, showcasing its implementation to safeguard against numeric vulnerabilities.
Takeaways
- 📚 Overflow and underflow are vulnerabilities in smart contracts that occur when numbers exceed their maximum or minimum range, causing unexpected results.
- 🔢 In Solidity, the 'uint256' data type can represent numbers from 0 to 2^256 - 1, and exceeding this range leads to overflow.
- ⏱ Overflow can be exploited to manipulate the 'lock time' in a smart contract, allowing immediate withdrawal instead of waiting for the set period.
- 💡 The script demonstrates a smart contract called 'TimeLock' that is vulnerable to overflow, allowing attackers to bypass the intended waiting period.
- 🛠 To exploit the vulnerability, an 'attack' contract is created, which sends a specific (overflow-inducing) value to the 'TimeLock' contract to reset the lock time to zero.
- 🔄 The overflow is achieved by calculating a value that, when added to the current lock time, equals 2^256, causing the lock time to wrap around to zero.
- 🛡 To prevent overflow and underflow vulnerabilities, the script recommends using the 'SafeMath' library, which checks for arithmetic operations that could lead to overflow.
- 📝 The 'SafeMath' library adds functions like 'add', 'sub', 'mul', and 'div' to the 'uint256' type, ensuring that arithmetic operations are safe from overflow.
- 🔬 The script includes a practical demonstration of how using 'SafeMath' can prevent the overflow exploit, making the 'TimeLock' contract secure against such attacks.
- 🔗 The video provides a link to the code in the description for further exploration and learning about overflow, underflow, and smart contract security.
Q & A
What is an overflow in the context of the video?
-An overflow occurs when a number exceeds the maximum range it can represent, causing the excess to wrap around and start counting from zero, resulting in a smaller number than intended.
What is underflow, and how does it differ from overflow?
-Underflow is similar to overflow but happens in the opposite direction. It occurs when a number is smaller than the minimum range, causing it to count backward from the maximum number, resulting in a larger number than intended.
What is the significance of the number 2^256 in the context of unsigned integers?
-The number 2^256 represents the maximum value that an unsigned integer with 256 bits can hold. It signifies the upper limit before an overflow occurs.
How does the video demonstrate an overflow example using unsigned integers?
-The video demonstrates an overflow by showing what happens when a number exceeds the maximum value of 2^256 - 1. It explains that adding 3 to this maximum value would wrap around to 2, illustrating the overflow effect.
What is the 'TimeLock' contract mentioned in the video, and what is its purpose?
-The 'TimeLock' contract is a smart contract that allows users to deposit Ether with the condition that they must wait at least one week before they can withdraw it. It uses mappings to keep track of balances and lock times.
How can a vulnerability in the 'TimeLock' contract be exploited to bypass the one-week waiting period?
-The vulnerability can be exploited by causing an unsigned integer overflow in the lock time mapping. By depositing Ether and then increasing the lock time with a huge number that causes an overflow, the lock time can be reset to zero, allowing immediate withdrawal.
What is the 'SafeMath' library, and how does it help prevent overflow and underflow?
-The 'SafeMath' library is a collection of mathematical functions that automatically check for overflow and underflow conditions. By using this library, operations that would result in an overflow or underflow will revert, preventing the exploit.
How can the 'TimeLock' contract be modified to incorporate the 'SafeMath' library and prevent overflows?
-The 'TimeLock' contract can be modified by importing the 'SafeMath' library and using its functions for arithmetic operations, such as 'add', instead of the default unsigned integer operations. This ensures that any operation that would cause an overflow will be prevented.
What is the purpose of the 'attack' contract demonstrated in the video?
-The 'attack' contract is a demonstration of how an attacker could exploit the overflow vulnerability in the 'TimeLock' contract to withdraw Ether without waiting for the required one-week period.
How does the video show the successful prevention of the overflow attack after implementing 'SafeMath'?
-The video shows that after recompiling and deploying the 'TimeLock' contract with 'SafeMath', attempting the overflow attack with the 'attack' contract results in a transaction failure due to the 'SafeMath' library's overflow check.
What is the final recommendation of the video for preventing overflow and underflow in smart contracts?
-The final recommendation is to use the 'SafeMath' library or similar mathematical libraries that include overflow and underflow checks to secure smart contracts against such vulnerabilities.
Outlines
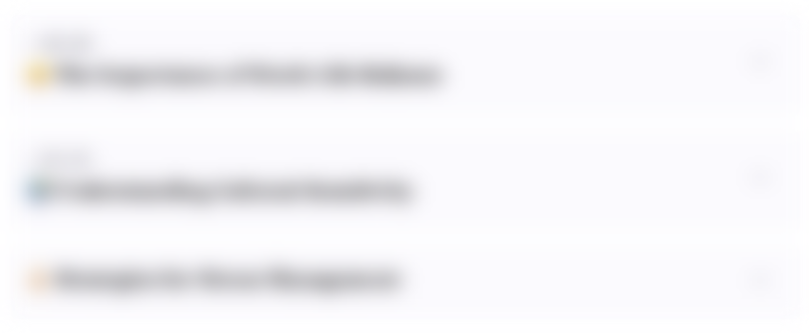
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
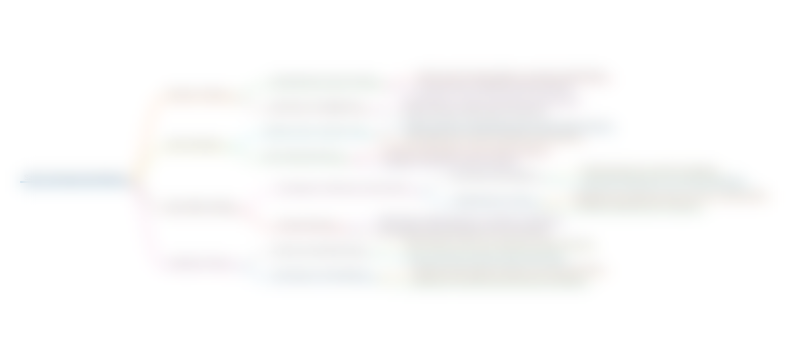
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
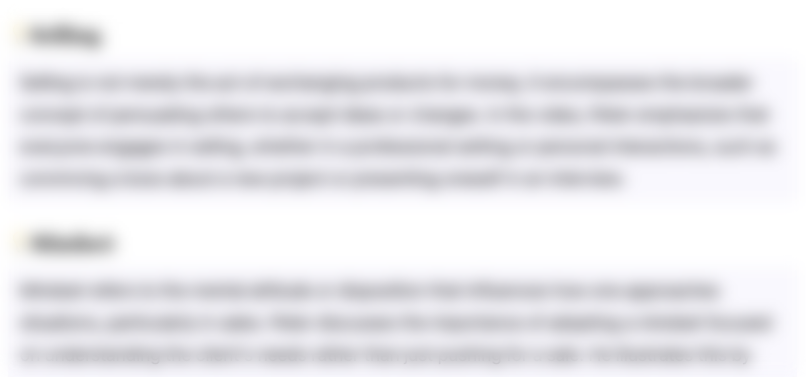
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
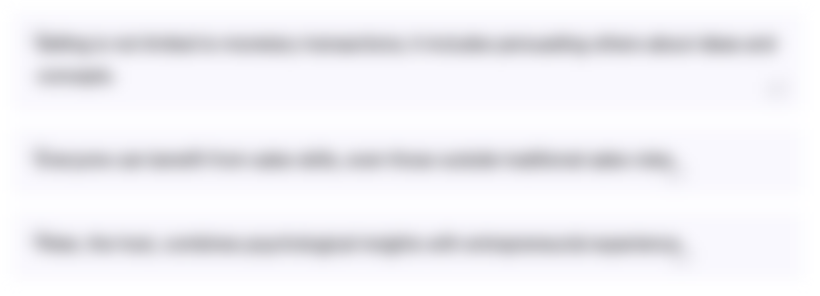
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
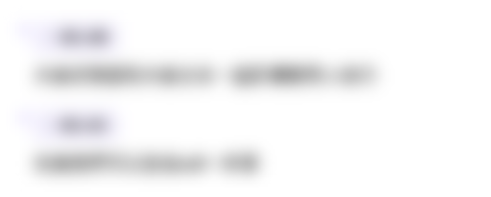
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)