How much JavaScript do you need to learn React?
Summary
TLDRThis video is designed for those planning to learn React and unsure if they have sufficient JavaScript knowledge. It outlines essential JavaScript topics to master before diving into React, such as data types, operators, loops, functions, DOM manipulation, and modern JavaScript features like ES6 syntax. By understanding these concepts first, learners can avoid the confusion of simultaneously learning JavaScript and React, making the learning journey smoother and more efficient. The video emphasizes the importance of solid JavaScript foundations for a successful React experience.
Takeaways
- 📚 Before learning React, ensure you have a solid understanding of JavaScript basics to avoid a difficult learning curve.
- 🧩 React is a JavaScript framework that simplifies development by providing ready-made features, similar to using a pre-made spice mix in cooking.
- 🔤 Start with the basics of JavaScript, including syntax, variables, data types, operators, control structures, loops, functions, arrays, and objects.
- 📊 Understanding JavaScript fundamentals like truthy and falsy values, short-circuit evaluation, strict vs. loose equality, and type coercion is crucial.
- 🔗 Grasp the concepts of pass by value vs. pass by reference and closures, as they are essential for working with functions in JavaScript.
- ⚙️ Learn about JavaScript’s DOM manipulation methods, which are important for understanding how React handles DOM elements behind the scenes.
- 📝 Modern JavaScript features like let and const, asynchronous programming, promises, async/await, sets, maps, spread/rest operators, destructuring, and modules are frequently used in React.
- 🏗️ Arrow functions are a concise way to create functions in JavaScript, especially useful for callbacks in React.
- 🔍 Familiarize yourself with event handling in JavaScript, including events, event handlers, and event propagation.
- 💡 The video recommends additional resources and a JavaScript Mastery course for those needing further guidance on these topics.
Q & A
Why is it important to learn certain JavaScript topics before learning React?
-Learning certain JavaScript topics before diving into React is important because React is built on top of JavaScript. If you don't have a strong foundation in JavaScript, you'll find it more difficult to understand and use React effectively. By learning these topics first, you can focus more on learning React without being overwhelmed by trying to learn JavaScript fundamentals simultaneously.
What is the analogy used in the script to explain the relationship between JavaScript and React?
-The script compares JavaScript and React to cooking spices. JavaScript is like the basic spices used to cook a meal, while React is like a pre-made spice mix that simplifies the cooking process. Just as the spice mix builds on the basic spices, React builds on JavaScript, providing a structure and framework that makes development more efficient.
What are the basic JavaScript topics recommended to learn before React?
-The recommended JavaScript topics include understanding the basic syntax and structure, data types, primitive data types vs. objects, operators (arithmetic, comparison, logical, assignment), control structures (if-else statements, switch statements), loops (for loop, while loop, etc.), functions, arrays, objects, and basic error handling (try-catch, custom errors).
Why is understanding the DOM important before learning React?
-Understanding the DOM is important because React automates much of the DOM manipulation, making development easier. However, knowing how the DOM works and how to manipulate it using vanilla JavaScript helps in understanding what React does under the hood, leading to better comprehension and troubleshooting skills when working with React.
What are some key modern JavaScript features that are frequently used in React?
-Some key modern JavaScript features frequently used in React include let and const for variable declarations, asynchronous programming (promises, async/await), sets and maps, the spread and rest operators, destructuring objects and arrays, the nullish coalescing operator, optional chaining, arrow functions, and modules for organizing and importing/exporting code.
What is the difference between primitive data types and objects in JavaScript?
-Primitive data types in JavaScript (such as strings, numbers, and booleans) hold simple data, while objects are more complex and can hold multiple values or properties. Understanding this difference is crucial for working effectively with JavaScript, particularly in managing data and variables.
Why is it important to understand 'pass by value' and 'pass by reference' in JavaScript?
-'Pass by value' and 'pass by reference' are important concepts because they describe how data is passed to functions in JavaScript. Primitive data types are passed by value, meaning the function works with a copy of the original value, while objects are passed by reference, meaning the function can modify the original object. This distinction is crucial to avoid unintended side effects in your code.
How does React utilize closures in its hooks?
-React uses closures in hooks like `useState` and `useEffect`. Closures allow these hooks to remember the state or values from their outer function, even after the function has executed. This is essential for maintaining state and side effects across renders in a React component.
What is short-circuit evaluation and how is it used in JavaScript and React?
-Short-circuit evaluation in JavaScript is a logical operation that returns the first truthy or falsy value it encounters. It's used in conditional rendering and assigning default values in JavaScript and React, allowing developers to write more concise and efficient code.
Why is asynchronous programming important in JavaScript, particularly in React?
-Asynchronous programming is important because it allows JavaScript to handle tasks like API requests, timeouts, and intervals without blocking the main thread. In React, asynchronous operations are common, especially when fetching data from APIs or handling user interactions, making it crucial to understand how to work with promises, async/await, and the event loop.
Outlines
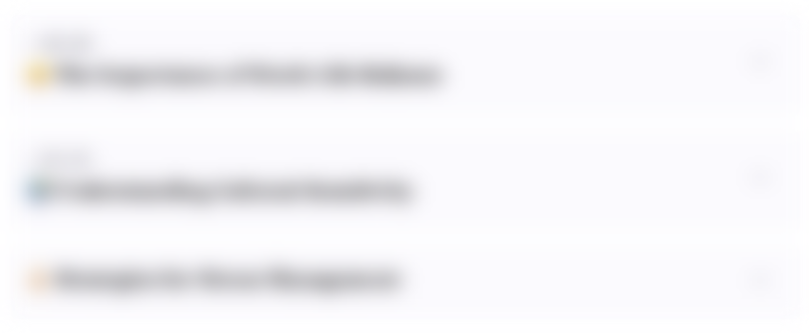
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
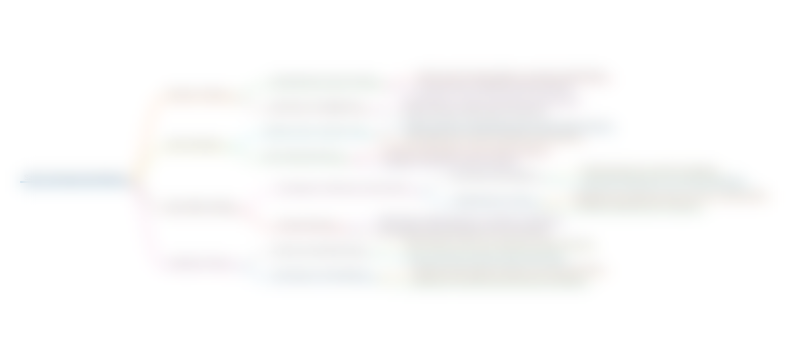
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
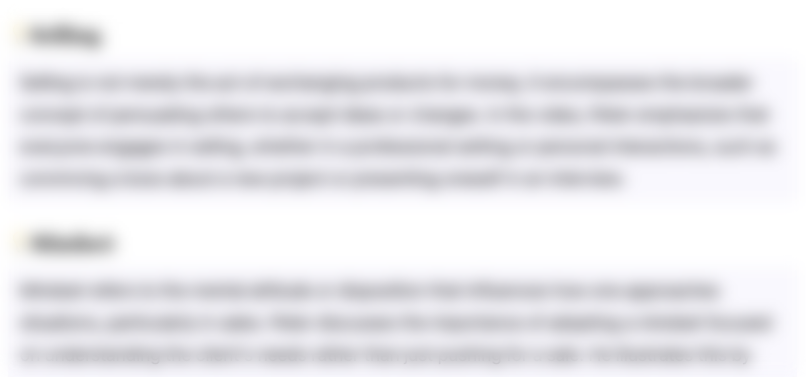
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
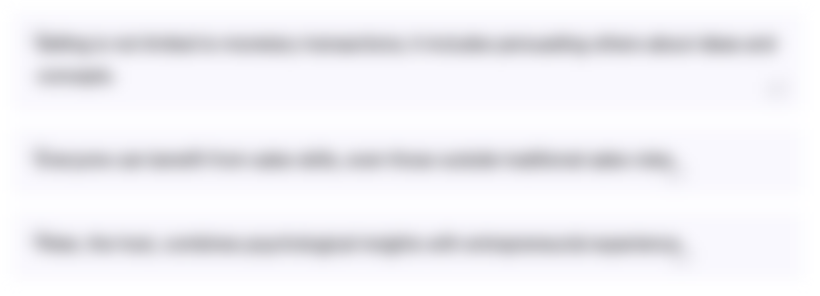
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
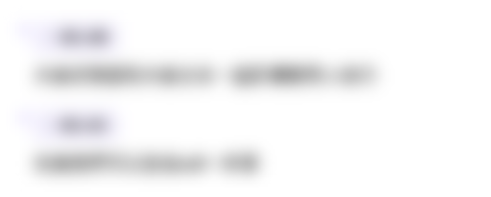
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
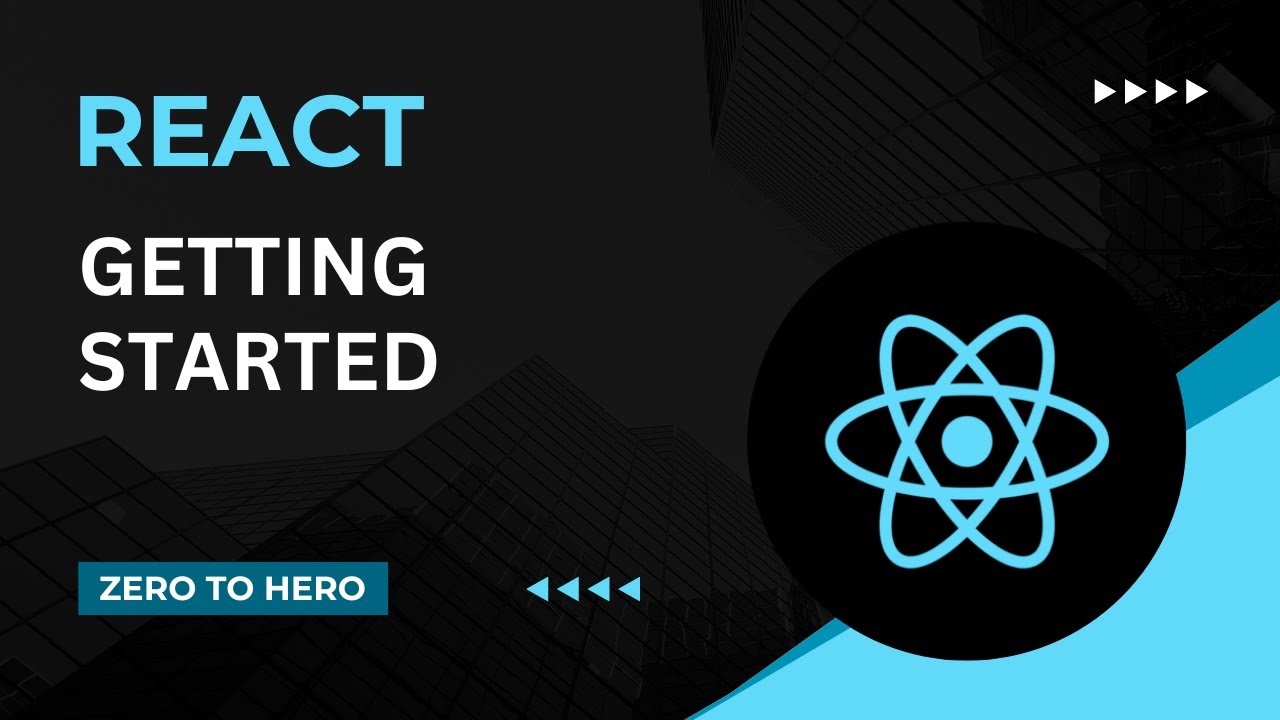
Getting Started | Mastering React: An In-Depth Zero to Hero Video Series
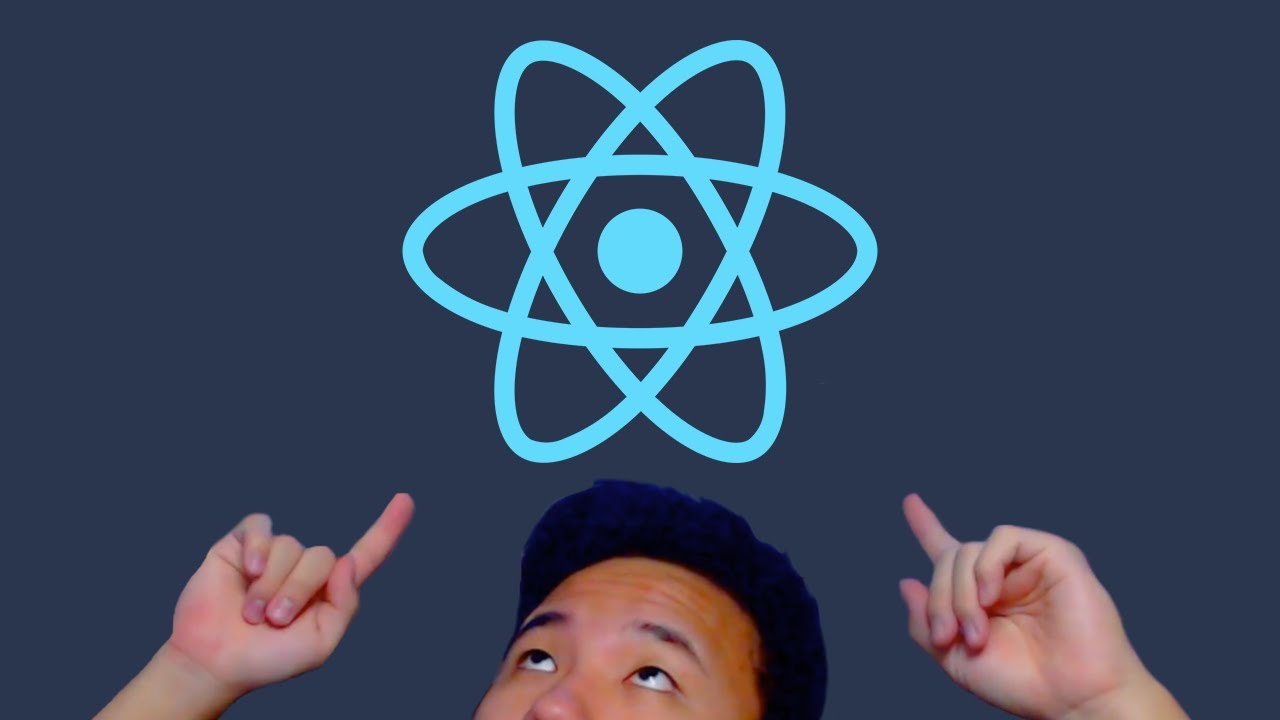
Aprende React en 45 Minutos
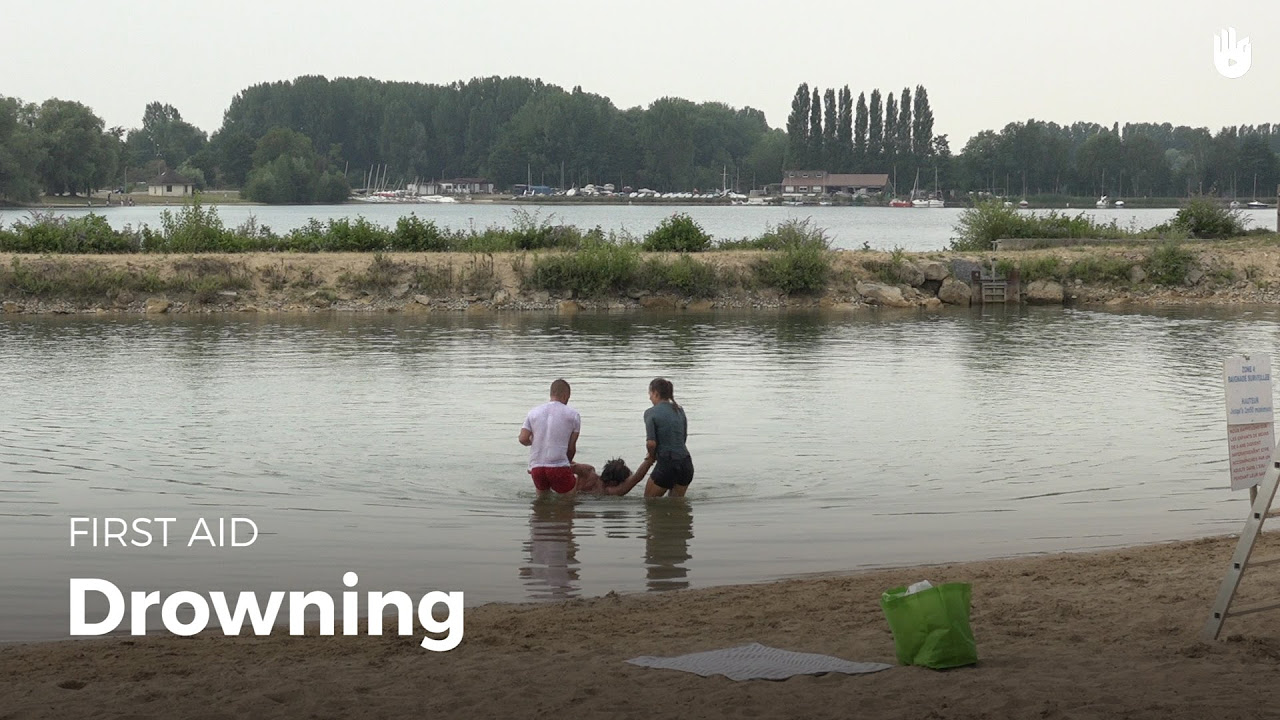
Learn first aid gestures: Drowning
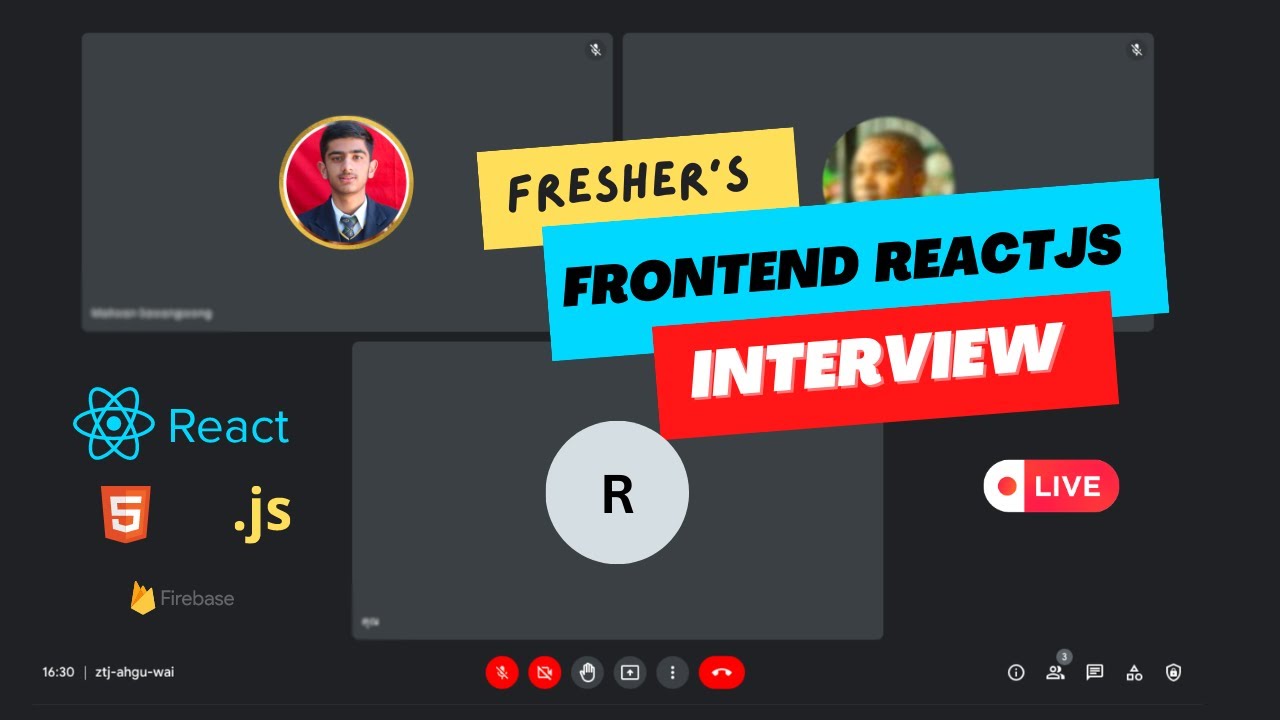
Fresher's Frontend Interview 🎉 | JavaScript | #reactjs (Mock) [Most Asked Questions -2023]
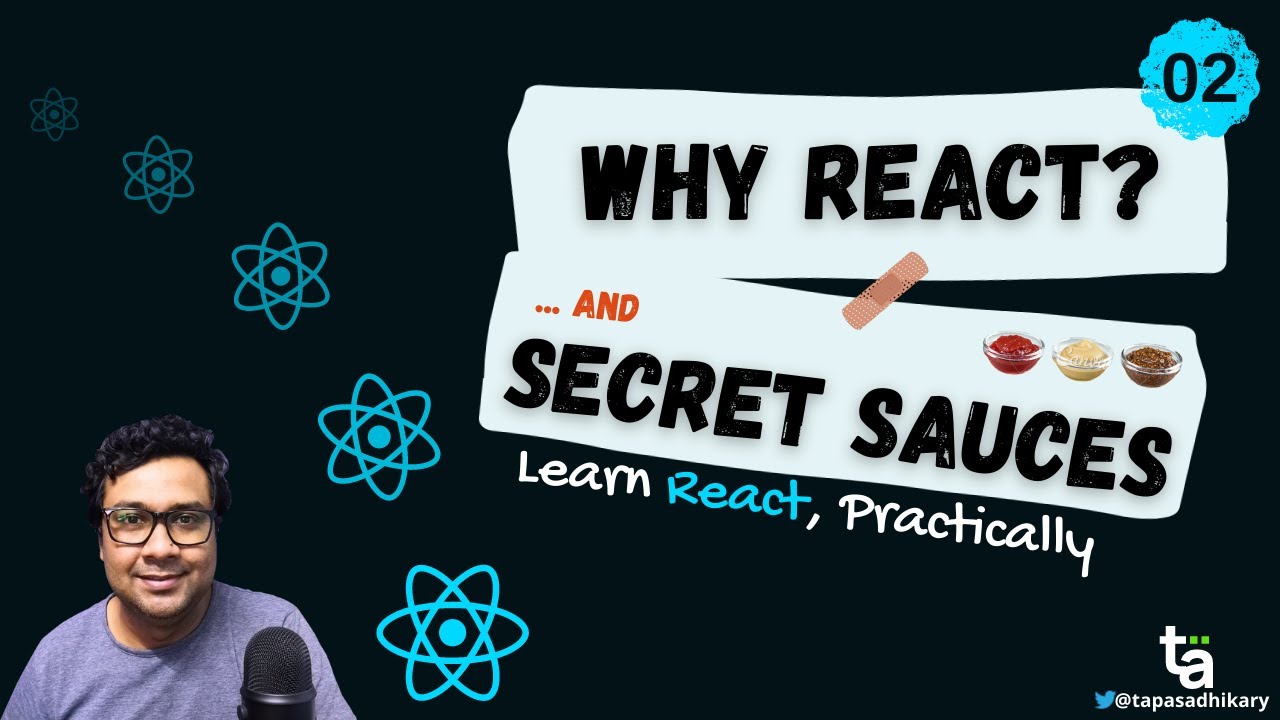
02 - Why React & Secret Sauces - React Fundamentals - Why React is a Declarative - Mastering Reactjs
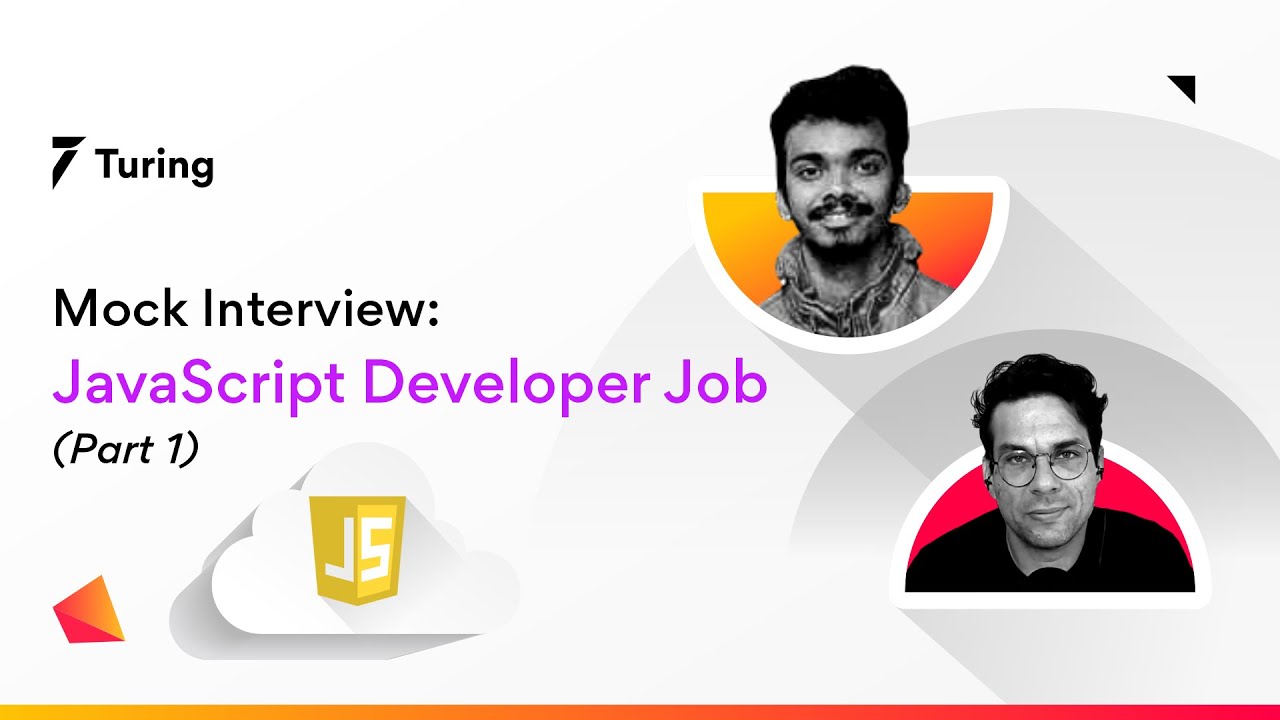
JavaScript Mock Interview | Interview Questions for Senior JavaScript Developers | Part 1
5.0 / 5 (0 votes)