Minimum Swaps to Group All 1's Together II - Leetcode 2134 - Python
Summary
TLDRIn this coding tutorial, the presenter tackles the algorithmic problem of 'minimum swaps to group all ones together.' The challenge involves rearranging an array of zeros and ones with the least number of swaps to make all ones contiguous, taking into account the array's circular nature. The video debunks a common misconception about using the minimum length window approach and introduces a more effective strategy of finding a window of the specific size that contains the maximum number of ones. The presenter also explains two methods to address the circular aspect: one using extra space and another with constant space complexity, concluding with a working solution that iterates through the array with pointers and modulus operations.
Takeaways
- 😀 The video discusses solving a coding problem involving minimum swaps to group all ones together in an array.
- 🔍 The problem is to find the minimum number of swaps required to make all ones in an array adjacent, considering the array as circular.
- 💡 A naive approach of finding the minimum length window containing all ones is introduced but later shown to be incorrect.
- 🔧 A counterexample is provided to demonstrate the flaw in the initial greedy solution approach.
- 📚 The correct approach is to find a window of size equal to the total number of ones that contains the maximum number of ones.
- 🔄 Two methods to address the circular aspect of the problem are discussed: one using extra space and another with constant space complexity.
- 📈 The video explains using a sliding window technique to find the optimal window without creating a new array.
- 👉 The 'count' function in Python is used to determine the total number of ones in the input array.
- 🔄 The sliding window technique involves adjusting a left and right pointer to find the window with the maximum ones.
- 📝 The solution involves keeping track of the number of ones in the current window and updating it as the window slides.
- 🎯 The final result is calculated by subtracting the maximum number of ones found in any window from the total number of ones.
Q & A
What is the main problem being discussed in the video?
-The main problem discussed is finding the minimum number of swaps required to group all ones together in a circular array containing only zeros and ones.
What is the significance of considering the array as circular in this problem?
-Considering the array as circular allows for the possibility that elements at the end of the array are adjacent to elements at the beginning, which affects how swaps are calculated.
What is the initial approach the speaker tried to solve the problem, and why did it fail?
-The initial approach was to find the minimum length window containing all ones. It failed because it didn't account for the possibility of making fewer swaps by rearranging ones in a way that skips holes, as demonstrated in the counterexample provided.
What alternative approach does the speaker suggest to solve the problem?
-The speaker suggests finding a window of size equal to the total number of ones in the input that contains the maximum number of ones, which can be more efficient in terms of swaps.
How does the speaker propose to handle the circular aspect of the problem?
-The speaker proposes two methods: one that requires extra space by concatenating a copy of the array, and another that uses constant space by employing a sliding window technique with modulo operation to simulate the circular nature without extra memory.
What is the sliding window technique mentioned in the script, and how is it applied here?
-The sliding window technique involves maintaining a window of a fixed size and sliding it across the data structure. In this script, it is applied by using two pointers, one representing the start and the other the end of the window, and adjusting them to find the optimal window with the maximum number of ones.
Why is the window size in the sliding window technique set to the total number of ones in the input array?
-The window size is set to the total number of ones because the goal is to find a contiguous group of ones that matches this count, which will minimize the number of swaps needed.
How does the speaker calculate the number of swaps needed using the sliding window technique?
-The speaker calculates the number of swaps needed by determining the difference between the window size (total number of ones) and the maximum number of ones found in any window of that size during the sliding window process.
What is the 'mod' operation used for in the context of this problem?
-The 'mod' operation is used to handle the circular nature of the array without creating a new array. It maps indices that would be out of bounds in a normal array to valid indices in the original array, considering the array's circular property.
What is the time complexity of the solution presented in the video?
-The time complexity of the solution is linear, as it involves a single loop that iterates over the array twice (once for counting ones and once for applying the sliding window technique).
How does the speaker ensure that the sliding window does not exceed the total number of ones?
-The speaker ensures this by checking if the length of the current window exceeds the total number of ones and, if so, adjusting the left pointer and updating the number of window ones accordingly.
Outlines
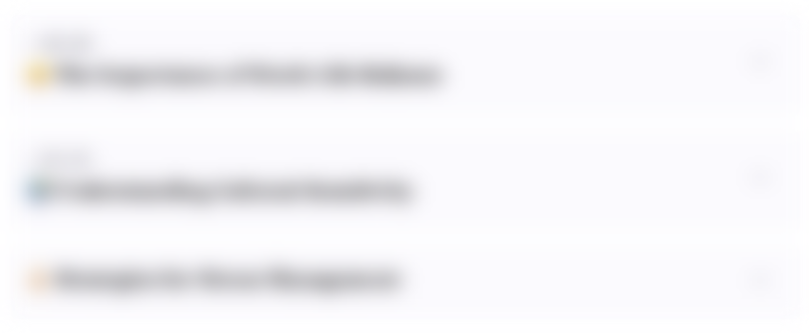
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
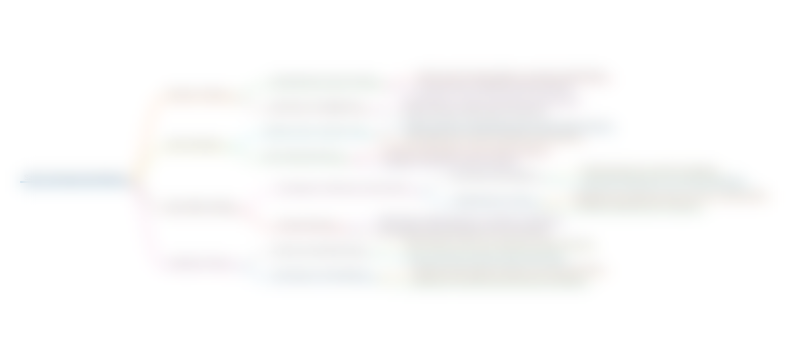
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
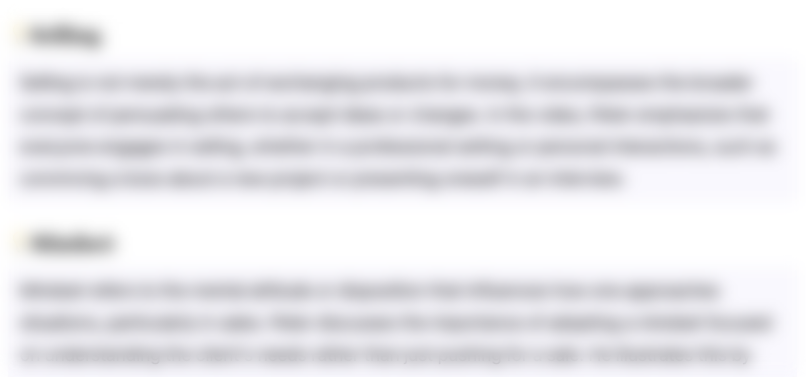
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
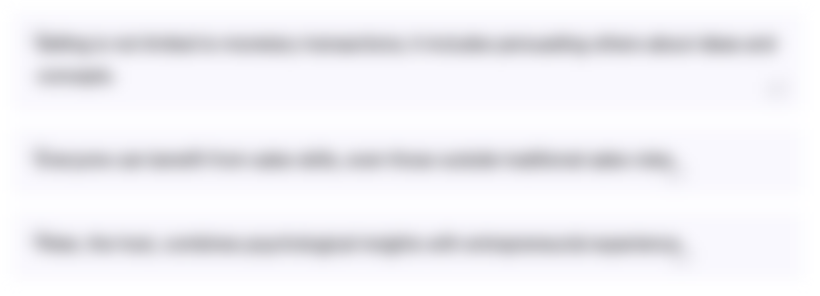
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
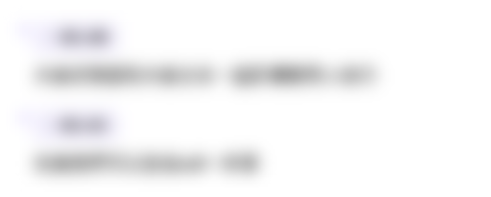
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
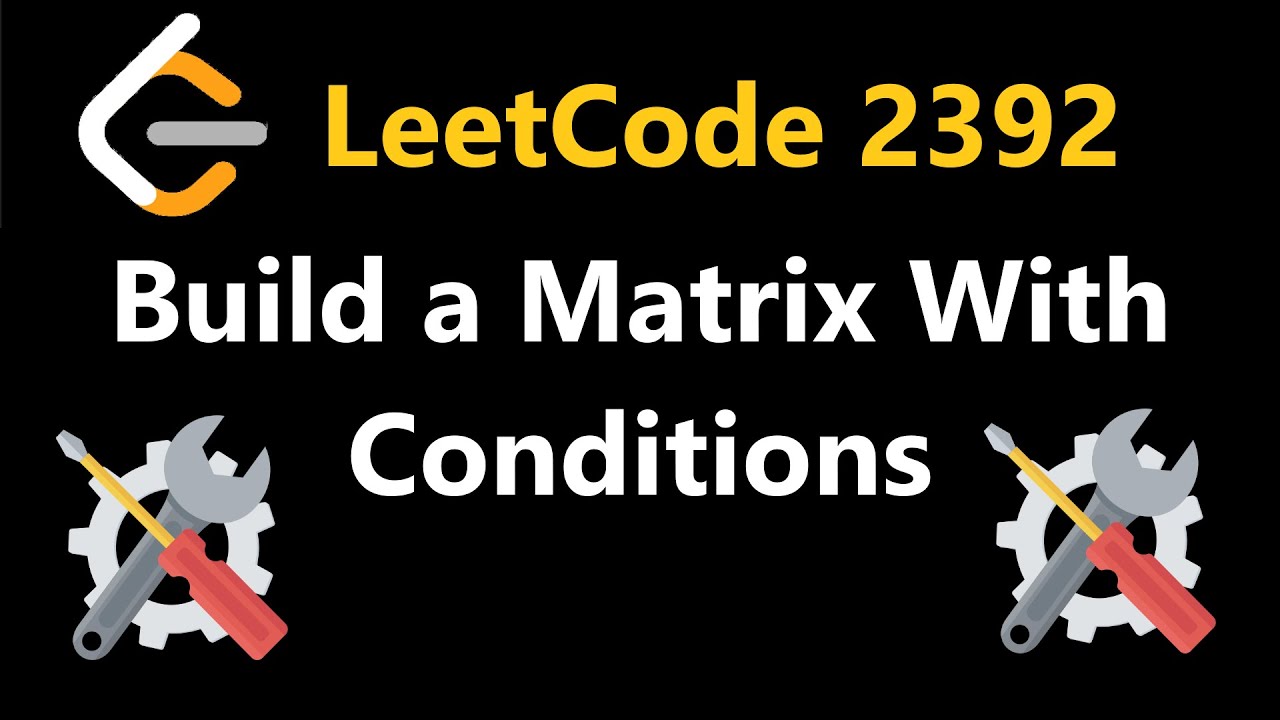
Build a Matrix With Conditions - Leetcode 2392 - Python
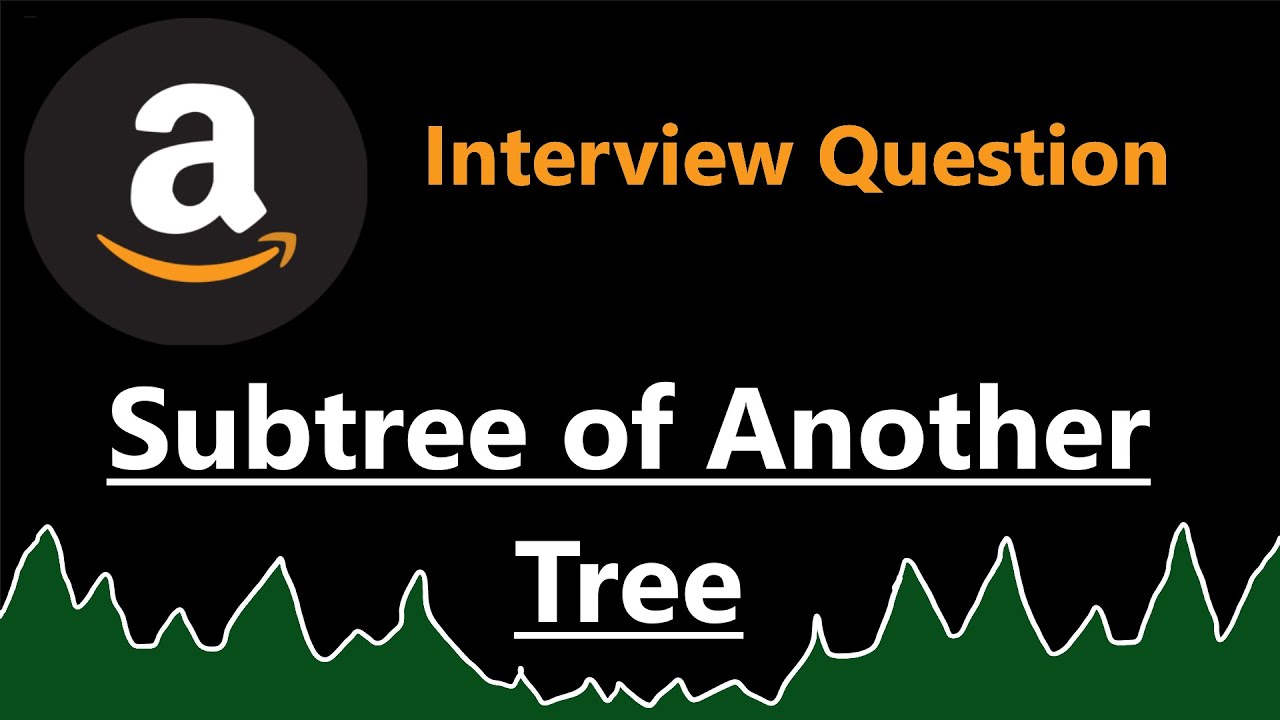
Subtree of Another Tree - Leetcode 572 - Python
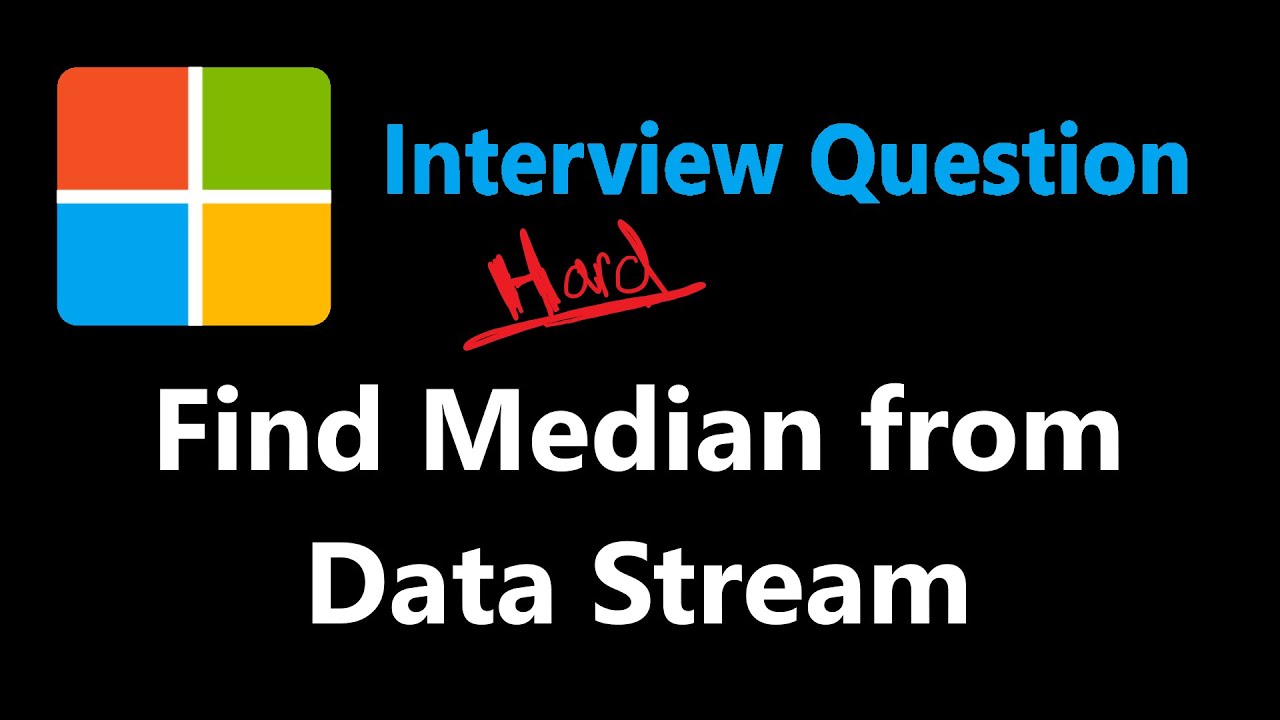
Find Median from Data Stream - Heap & Priority Queue - Leetcode 295
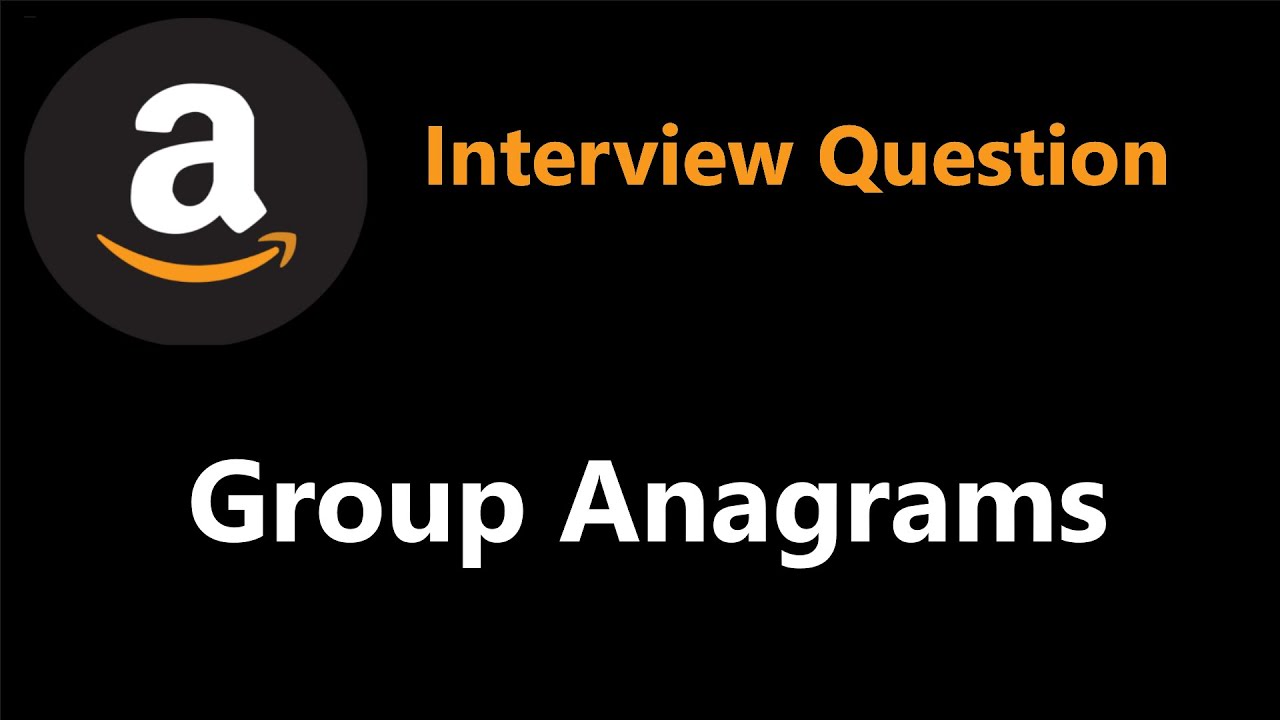
Group Anagrams - Categorize Strings by Count - Leetcode 49

Examples of Antenna Array | Solved Examples based on Parameters of Antenna Array | Engineering Funda
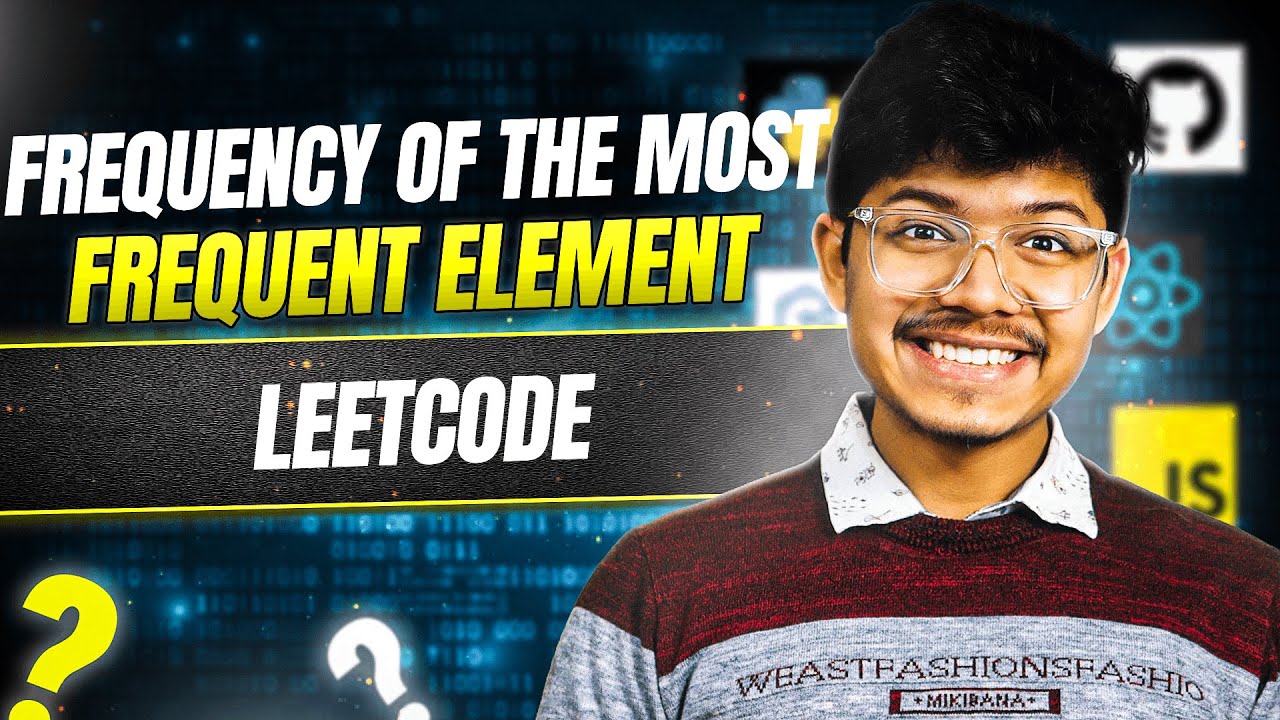
1838. Frequency of the Most Frequent Element | Sliding Window Template | Shrinking & Non-Shrinking
5.0 / 5 (0 votes)