Create A Python API in 12 Minutes
Summary
TLDRThe video explains what an API is and how to create a simple API using Python and the Flask framework. It covers API concepts like routes, HTTP methods, path parameters, and query parameters. A get route and post route are demonstrated to retrieve and create data. The video recommends using Postman to test APIs and curl requests. Additional topics mentioned are authenticating APIs and connecting them to databases for more advanced capabilities.
Takeaways
- 😀 API stands for Application Programming Interface and allows software systems to communicate
- 💡 APIs typically live on a server, manipulate a database, and handle requests and responses
- 📝 Flask is a popular Python web framework for building APIs
- 🌐 APIs have different routes that correspond to endpoints that return data
- 🔍 GET requests retrieve data, POST creates data, PUT updates data, DELETE removes data
- 📎 Path parameters allow passing dynamic values in a route URL
- 📋 Query parameters add extra values after the main route path
- 📤 POST routes receive JSON data in the request body and can return it in the response
- ✅ Postman is a useful tool for testing API requests and responses
- 🌟 Next steps are adding authentication and connecting APIs to databases
Q & A
What does API stand for and what does it allow?
-API stands for Application Programming Interface. It allows different software systems to communicate with each other through a set of rules.
Where does an API typically live and what does it manipulate?
-An API typically lives on some type of server and manipulates some kind of database.
What are the main HTTP methods used when creating API routes?
-The main HTTP methods used are GET, POST, PUT and DELETE.
How can you access path parameters and query parameters in Flask?
-Path parameters are accessed directly as function parameters. Query parameters are accessed using request.args which contains a dictionary of the parameters.
How do you return JSON data from a Flask API?
-Use the json.jsonify function to convert a Python dictionary to JSON and return it along with an appropriate status code like 200.
What tool does the speaker recommend for testing APIs?
-The speaker recommends using Postman for testing APIs.
What code do you need to create a basic Flask application?
-You need to import Flask, create an app variable set to Flask(__name__), and run the app using if __name__ == '__main__': app.run().
How do you specify which HTTP methods a route accepts?
-Pass the allowed methods like ["GET", "POST"] as the second argument to the app.route decorator.
How can you check the request method inside a route function?
-Use request.method to get the request method string like 'GET', 'POST' etc.
What is typically the next step after creating a basic API?
-The next typical steps are adding authentication and connecting the API to a database.
Outlines
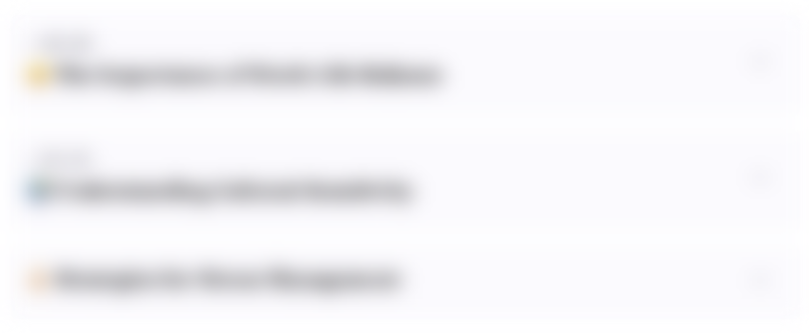
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
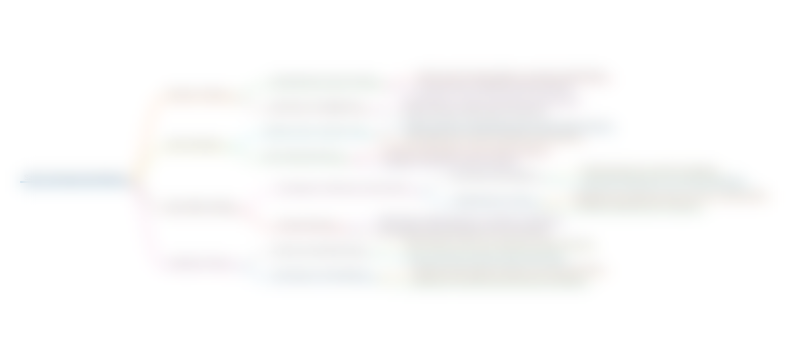
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
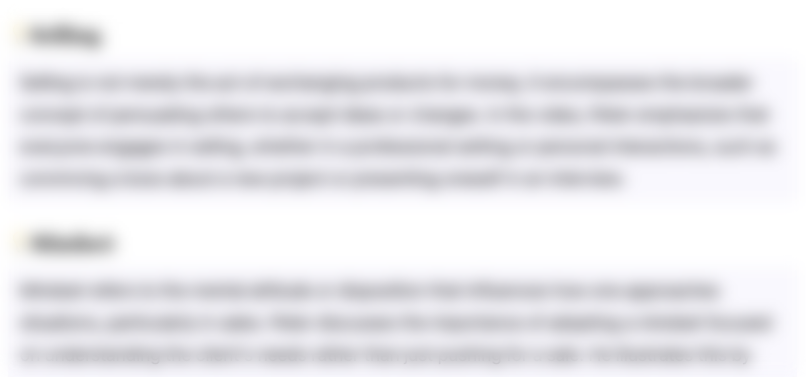
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
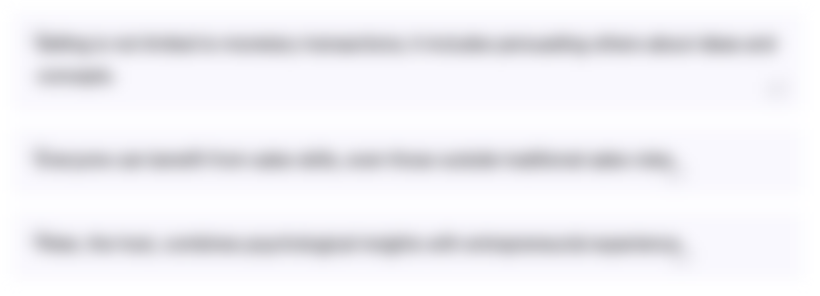
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
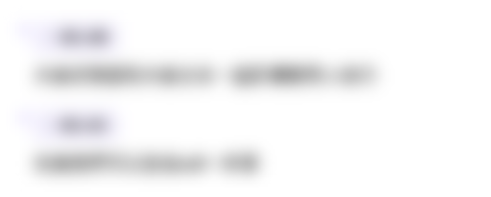
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
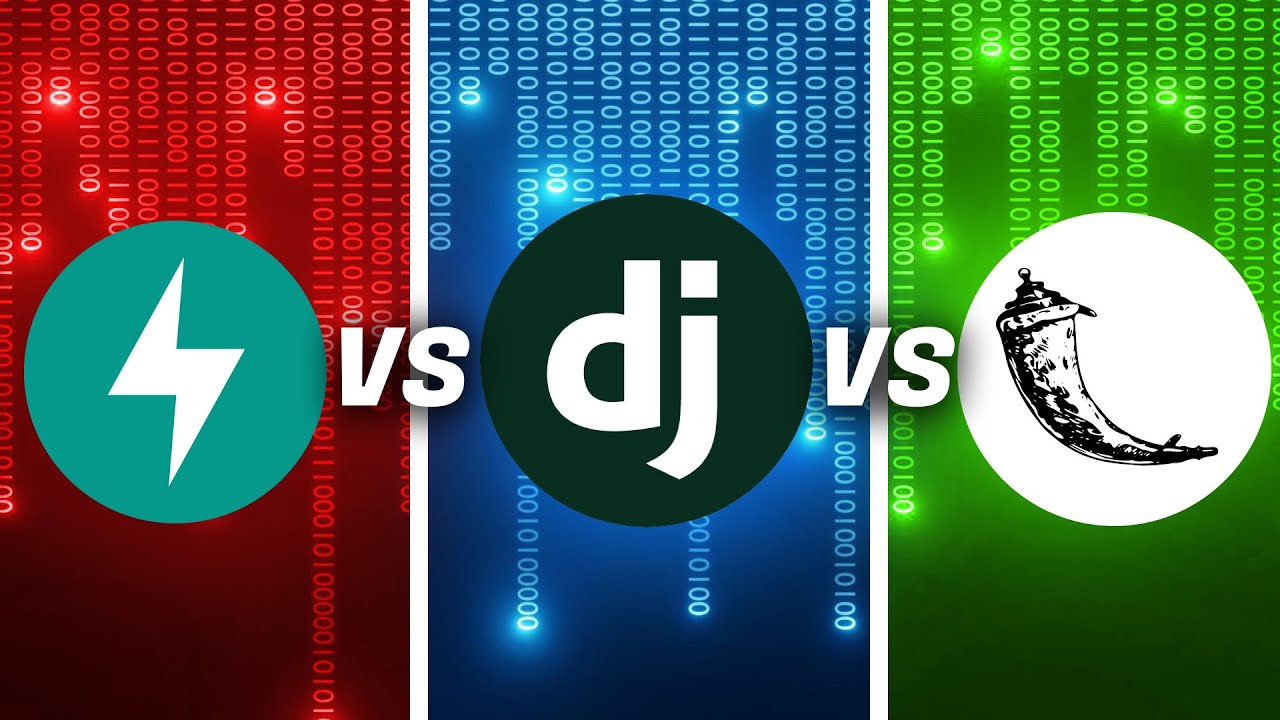
FastAPI, Flask or Django - Which Should You Use?
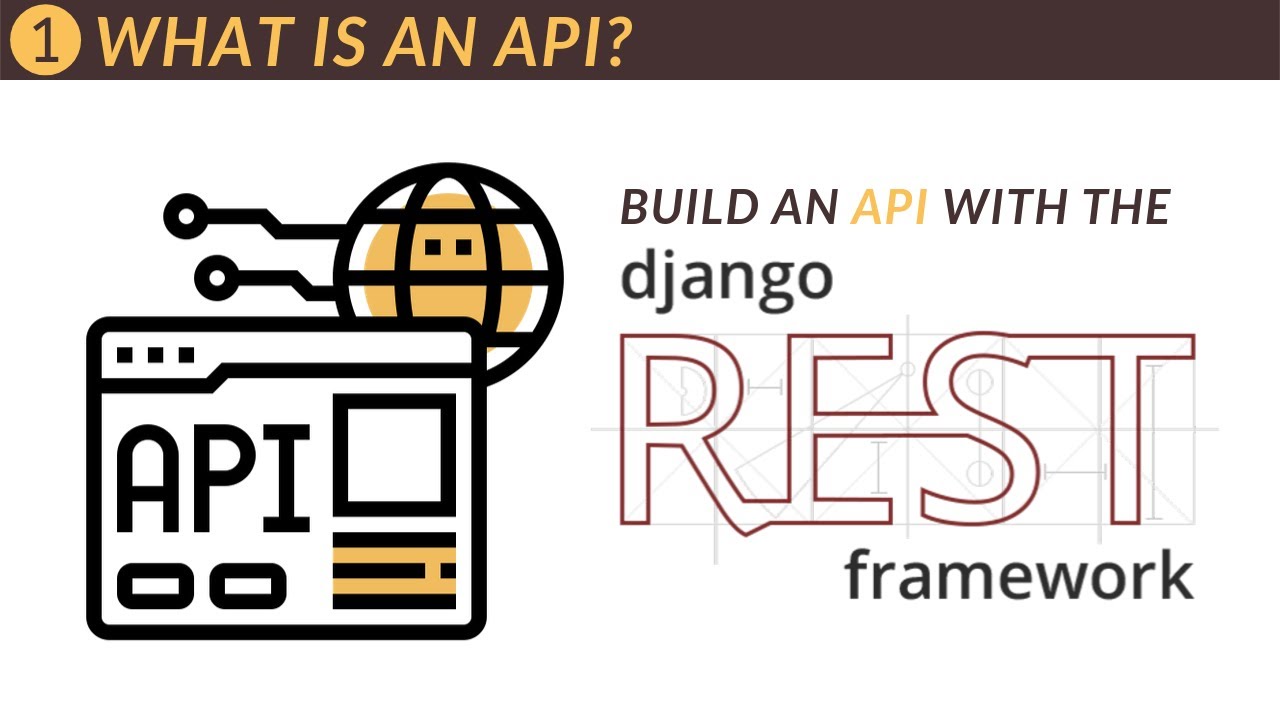
Build an API with Django // Part 1 - What is an API?
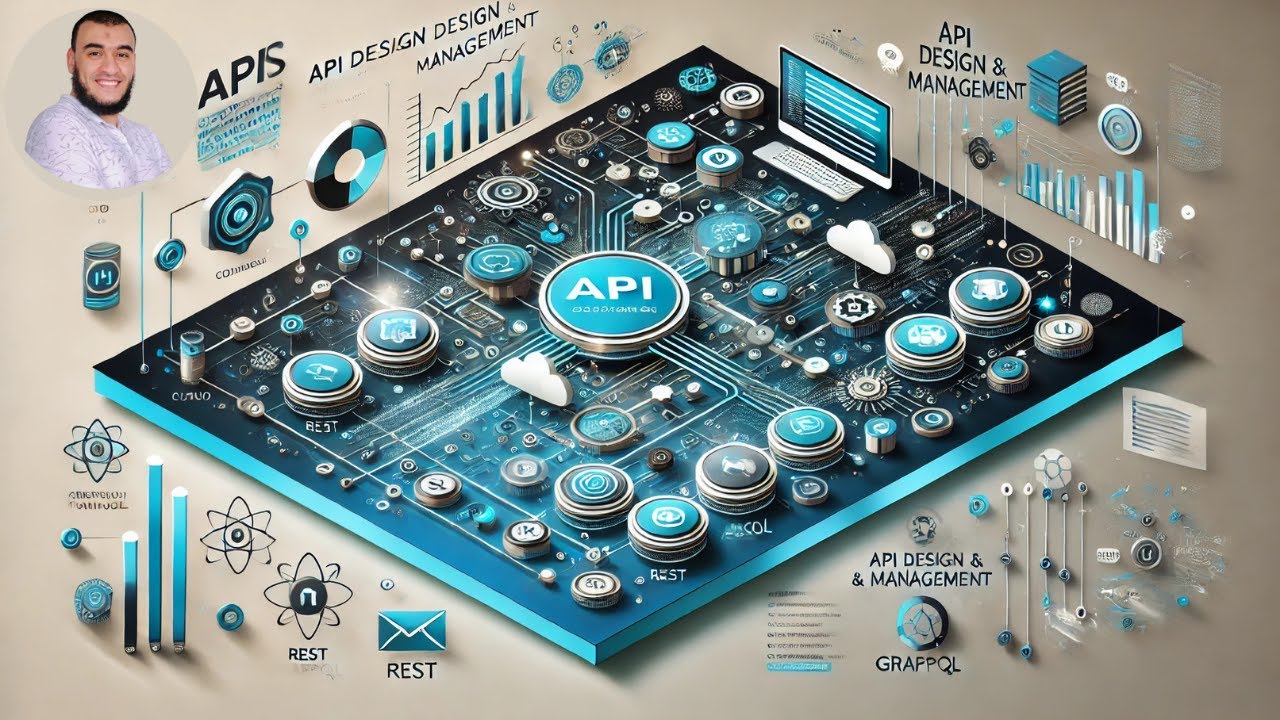
API Design and Management (Understanding API) - بالعربي
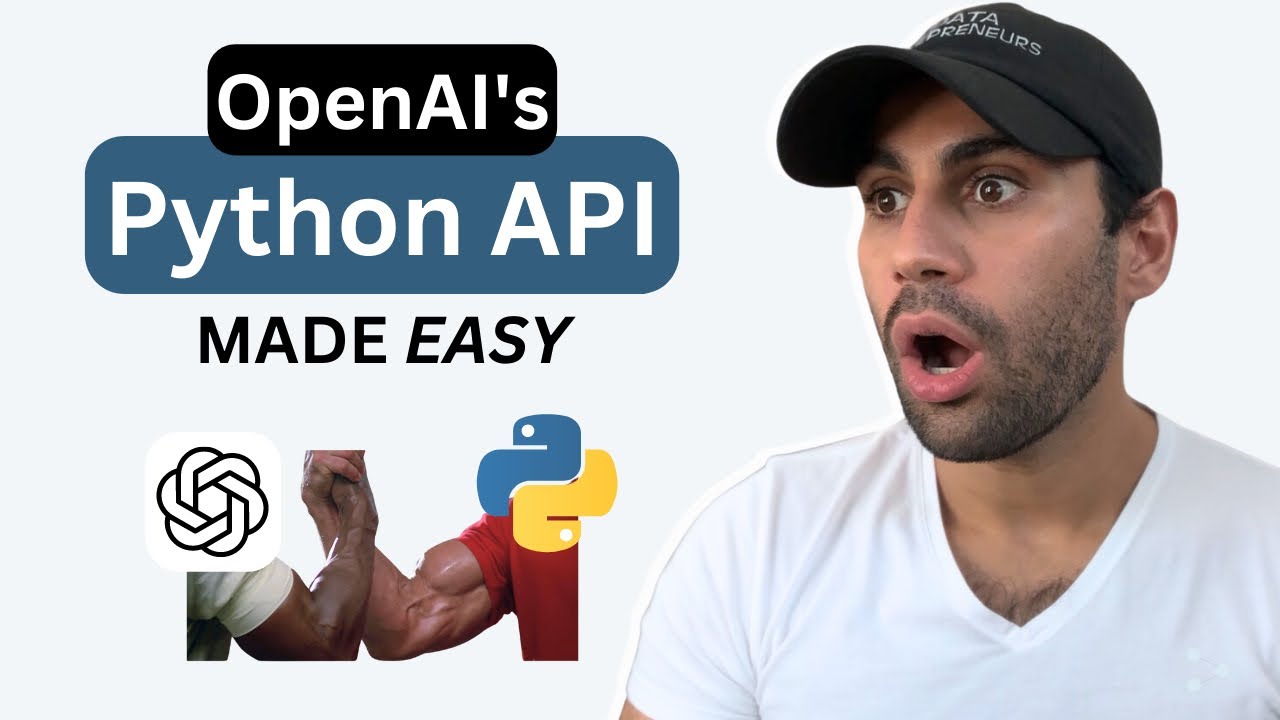
The OpenAI (Python) API | Introduction & Example Code
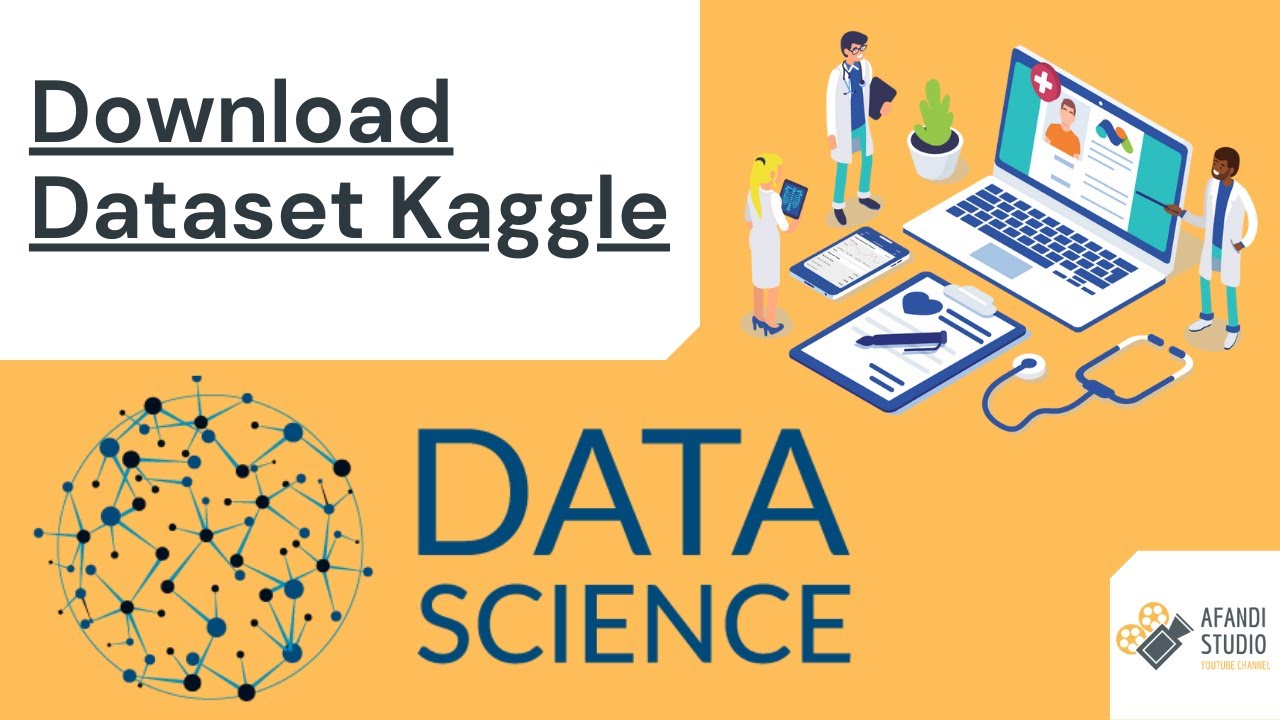
Download Dataset Kaggle dari Jupyter Notebook Menggunakan Kaggle API

【超簡単】PythonでChatGPTを使ってAIチャットボットを作ろう!ChatGPTとPythonの最強コンビ!~プログラム公開中~
5.0 / 5 (0 votes)