Lec-22: List Functions In Python 🐍 | Built in Functions Python 🐍
Summary
TLDRThis tutorial explains various Python list functions, ideal for beginners and those preparing for exams. It covers creating lists, using functions like `len`, `append`, `extend`, `insert`, and `count`, and demonstrates methods to remove, pop, and sort elements. The video also highlights how to find the smallest or largest element using `min` and `max`, as well as the `reverse` function. Special attention is given to handling duplicates, sorting in ascending/descending order, and managing lists with multiple values. The presenter emphasizes the importance of mastering these functions for Python interviews and exams.
Takeaways
- 😀 Lists are an essential data structure in Python and can be created using square brackets or the list() function.
- 😀 You can access list elements using their index, with indexing starting from 0 in Python.
- 😀 The length of a list can be obtained using the built-in `len()` function, which counts the total number of elements.
- 😀 The `append()` method adds a single element to the end of the list.
- 😀 Use `insert()` to add an element at a specific index in the list.
- 😀 The `extend()` method allows you to add multiple elements at once to the list.
- 😀 The `remove()` method removes the first occurrence of a specified element from the list.
- 😀 The `pop()` method removes an element at a given index and returns it, enabling element manipulation during list operations.
- 😀 You can sort a list in ascending or descending order using the `sort()` method, with the `reverse=True` argument for descending order.
- 😀 To reverse a list in place, use the `reverse()` method. Alternatively, `sorted()` returns a sorted copy of the list without modifying the original.
- 😀 The `count()` method counts how many times a specific element appears in the list, helping to track element frequency.
Q & A
What is a Python list and how is it created?
-A Python list is a mutable data structure that can hold multiple items. It is created by placing elements inside square brackets, separated by commas, e.g., `my_list = [10, 20, 30, 40]`.
How can you access an element from a list in Python?
-Elements in a Python list are accessed using indexing. Indexing starts from 0. For example, `my_list[0]` will return the first element of the list.
What does the `len()` function do when used with a list?
-The `len()` function returns the number of elements present in a list. For example, `len(my_list)` will return the total number of items in the list.
How can you add an element to the end of a list in Python?
-You can add an element to the end of a list using the `append()` method. For example, `my_list.append(50)` will add 50 to the end of the list.
What is the purpose of the `insert()` method in Python lists?
-The `insert()` method allows you to add an element at a specific index in a list. For example, `my_list.insert(2, 25)` inserts 25 at index 2 of the list.
How can you extend a list with another list in Python?
-You can extend a list by using the `extend()` method, which adds all elements from another list to the current list. For example, `my_list.extend([60, 70])` will add 60 and 70 to the end of `my_list`.
What is the difference between `remove()` and `pop()` methods in Python?
-`remove()` removes the first occurrence of a specified value from the list, while `pop()` removes and returns an element at a specific index or the last element by default.
How does the `sort()` method work in Python?
-The `sort()` method sorts the elements of a list in ascending order by default. For example, `my_list.sort()` will reorder the list in increasing order.
What is the difference between the `sort()` method and the `sorted()` function?
-The `sort()` method sorts a list in place, meaning it modifies the original list, while the `sorted()` function returns a new list that is sorted, leaving the original list unchanged.
How can you count the occurrences of an element in a list?
-You can count the occurrences of an element using the `count()` method. For example, `my_list.count(20)` will return the number of times 20 appears in `my_list`.
Outlines
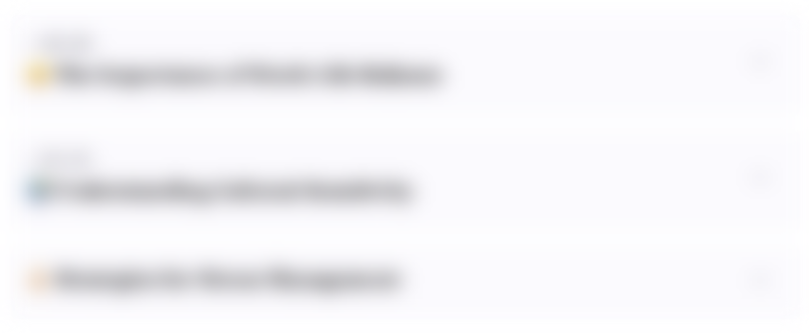
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
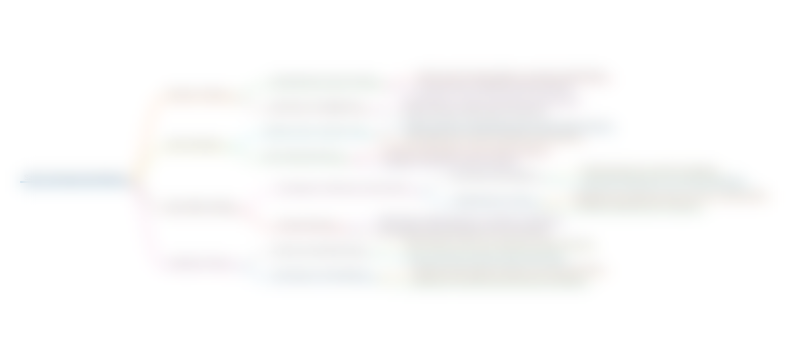
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
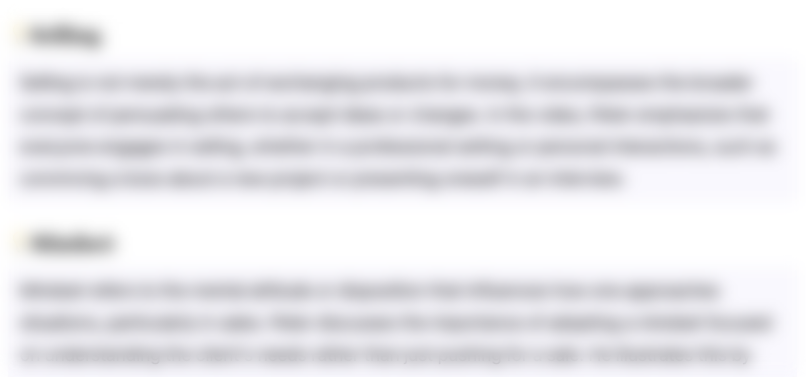
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
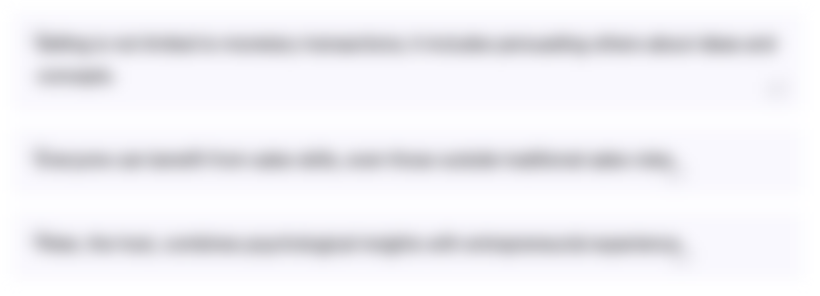
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
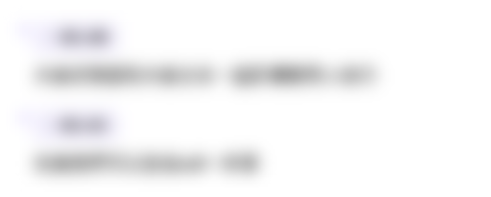
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
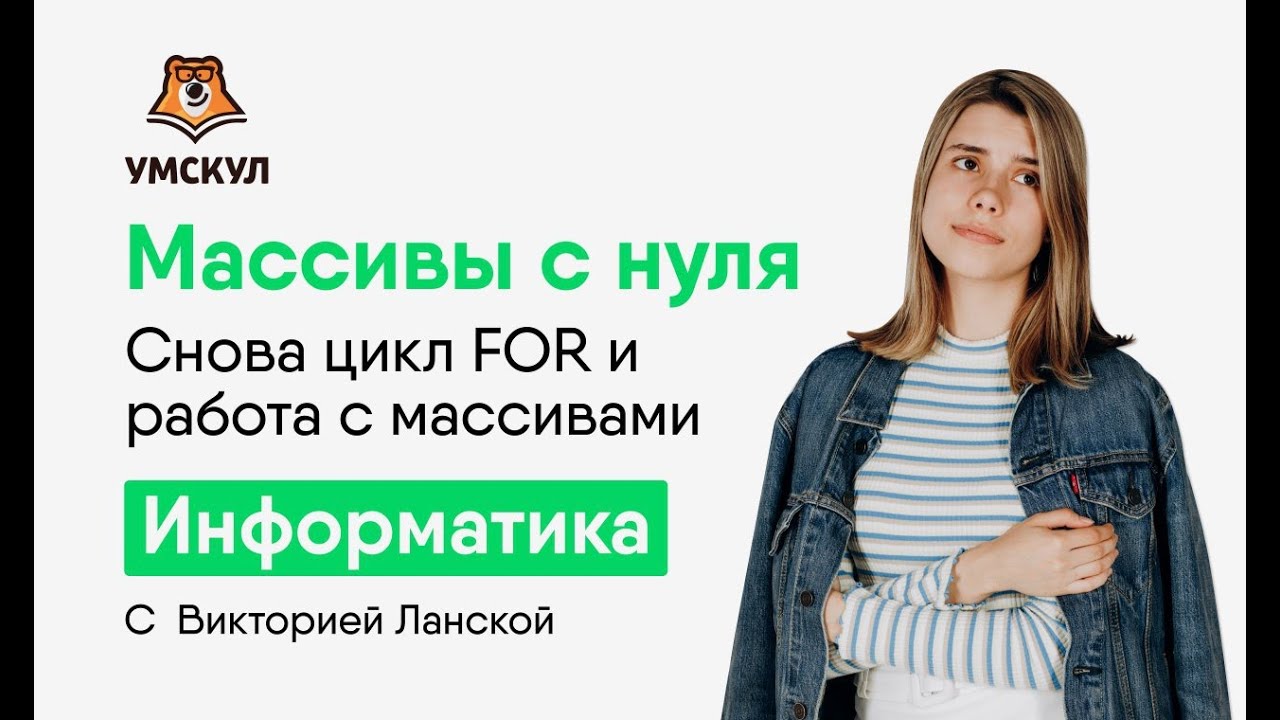
Python для ЕГЭ. Массивы с нуля. Снова цикл for и работа с массивами.
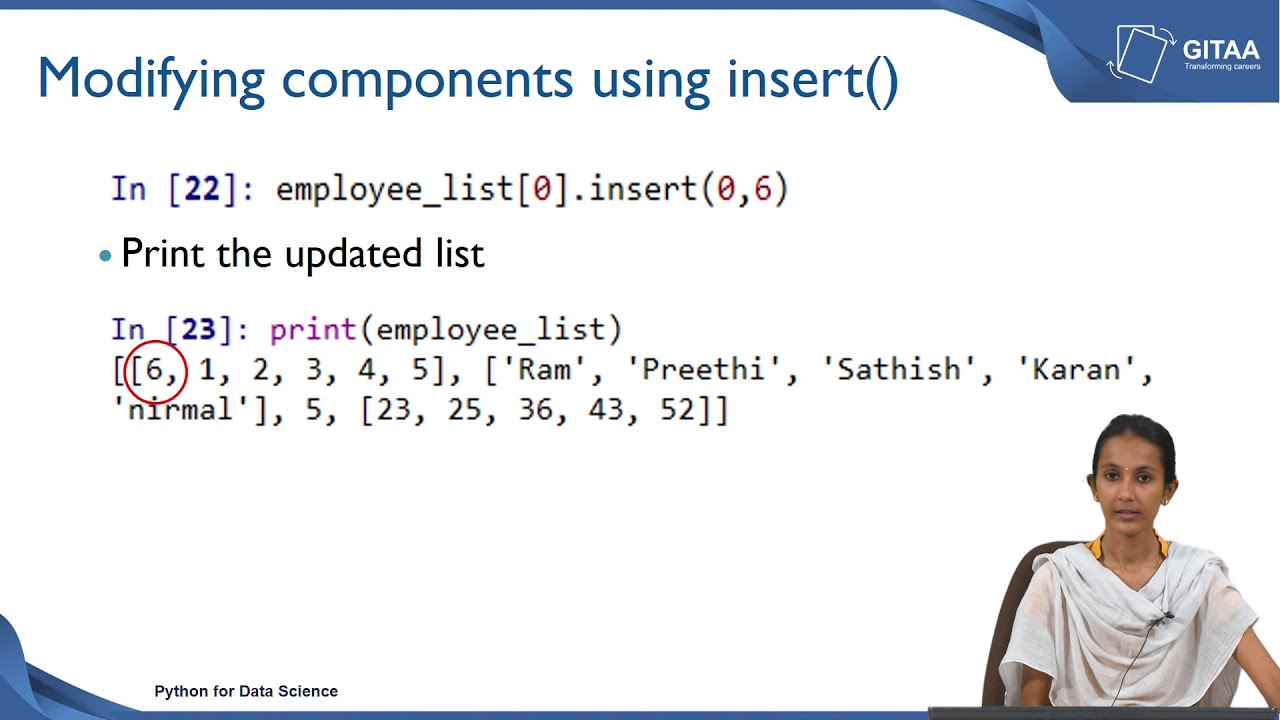
List Part 2
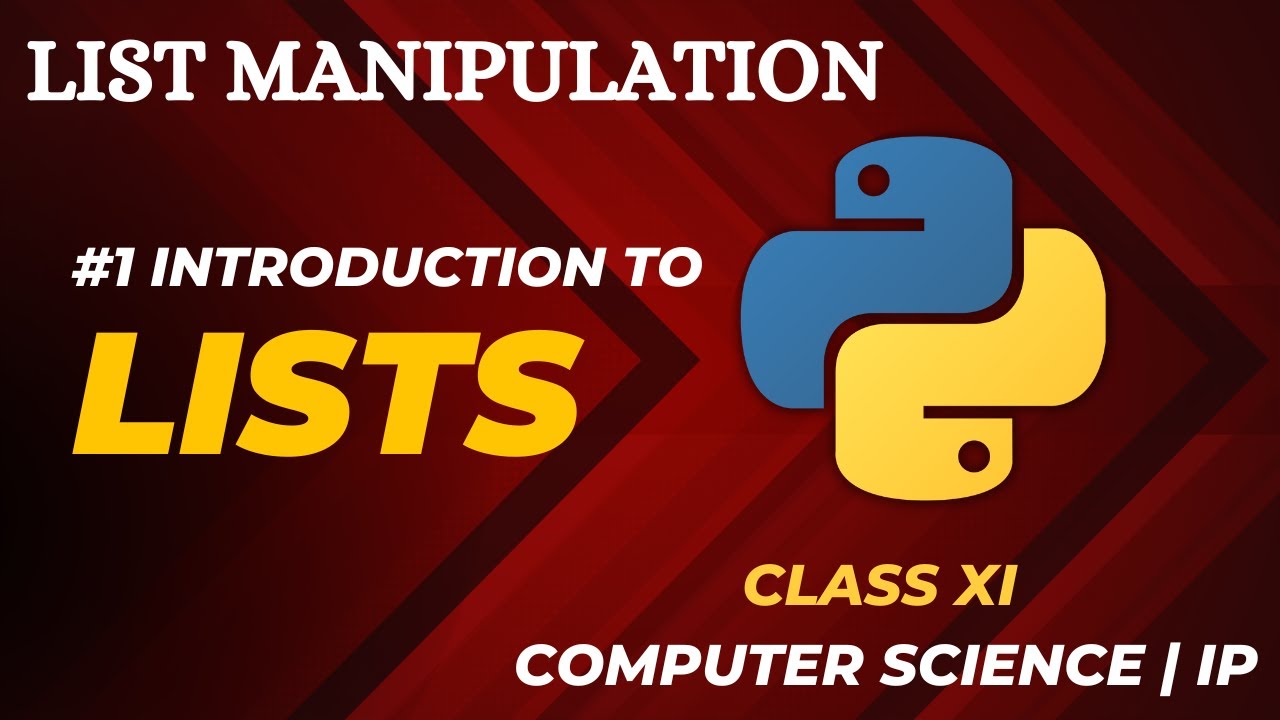
#1 Introduction to Lists | List Manipulation | Class 11 CBSE Computer Science and IP
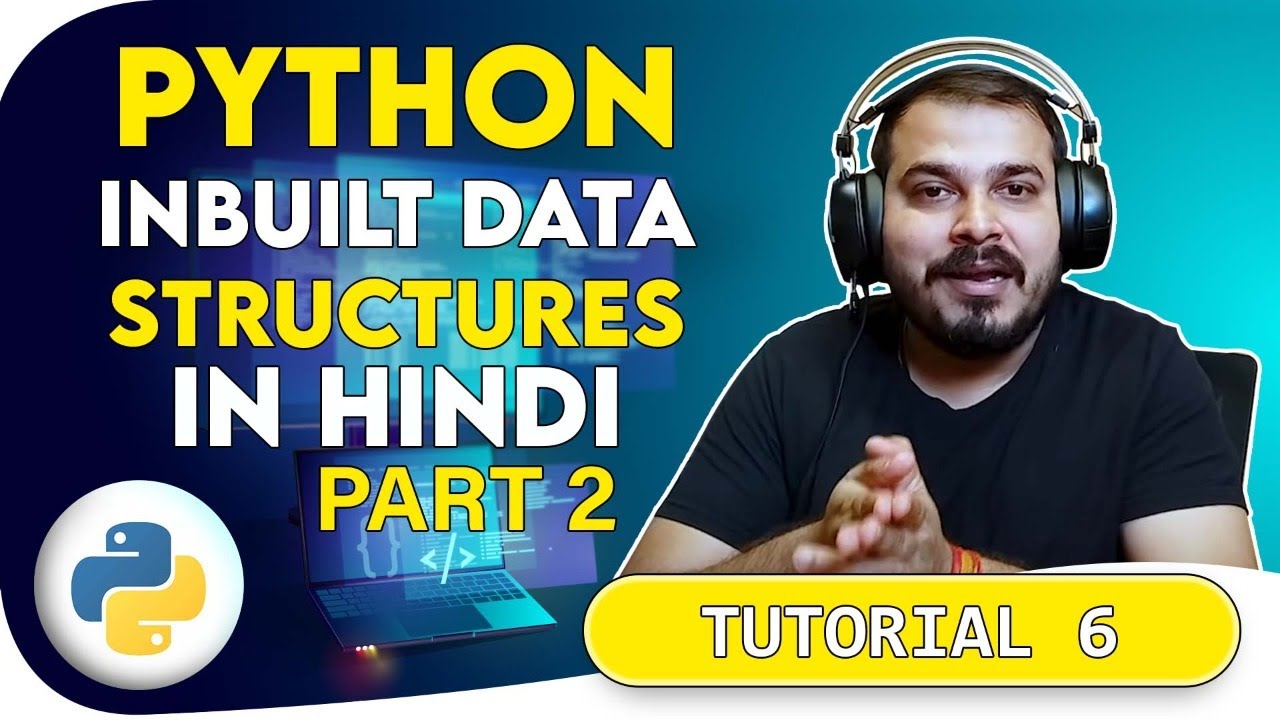
Tutorial 6- Python List And Its Inbuilt Function In Hindi
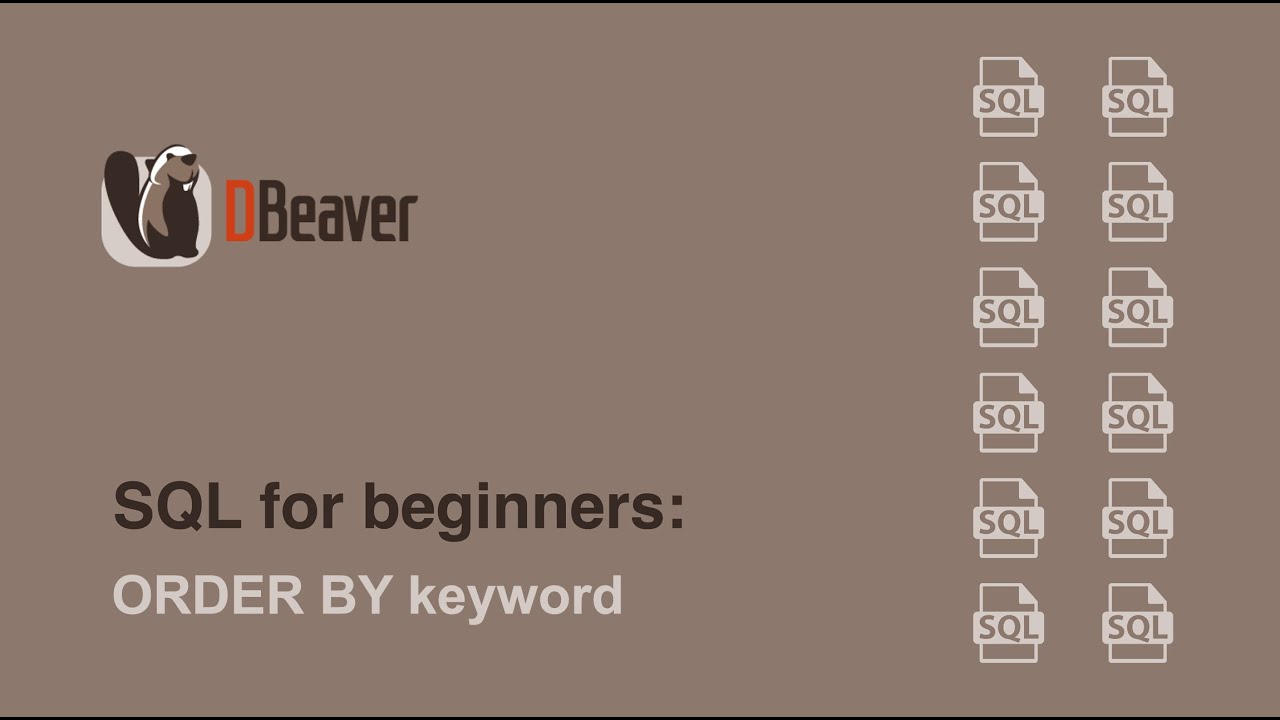
SQL for beginners: ORDER BY keyword

Introduction To Lists In Python (Python Tutorial #4)
5.0 / 5 (0 votes)