Circular Queue Implementation - Linked List
Summary
TLDRIn this video, we explore the implementation of a circular queue using a circular linked list. The circular queue operates on the FIFO principle, where elements are added and removed sequentially. We delve into the enqueue and dequeue operations, demonstrating how nodes are added and removed in a circular structure. Key methods such as peek and isEmpty are also covered, along with their time and space complexity. This tutorial is ideal for those seeking a deeper understanding of circular queues and their efficient implementation using linked lists.
Takeaways
- 😀 A queue is a linear data structure that follows FIFO (First In, First Out) order for adding and removing elements.
- 😀 Circular queues use a circular linked list, where the last node points back to the first node, forming a circle.
- 😀 Enqueue operation involves adding a node to the rear of the queue and adjusting pointers to maintain the circular structure.
- 😀 Dequeue operation removes the front node of the queue by updating the 'rear.next' pointer, or setting 'rear' to null if there's only one node.
- 😀 A circular linked list eliminates the need to track the front node explicitly, as it can be accessed via 'rear.next'.
- 😀 If the queue is empty (rear == null), both enqueue and dequeue operations handle this by special checks and exceptions.
- 😀 The 'peek' operation allows accessing the front element without removing it, while checking for an empty queue.
- 😀 The 'isEmpty' method simply checks if the queue has no elements by verifying if 'rear' is null.
- 😀 The class structure for the circular queue consists of a 'rear' pointer to the last node and a node class with data and a next reference.
- 😀 Time complexity for enqueue, dequeue, peek, and isEmpty methods is constant (O(1)), as they involve a fixed number of operations.
- 😀 Space complexity for circular queue operations is also constant (O(1)), as each node only holds a reference to the next node.
Q & A
What is the basic concept of a queue in data structures?
-A queue is a linear data structure that follows the FIFO (First In, First Out) principle, meaning the first element added to the queue is the first one to be removed.
What is the primary difference between a regular queue and a circular queue?
-In a circular queue, the last element points back to the first element, forming a circle. This allows the queue to efficiently reuse space, unlike a regular queue where elements may leave unused space at the front.
Why is a circular linked list used to implement a circular queue?
-A circular linked list is used because the last node in the list points to the first node, making it easier to manage the queue without needing a separate front reference. This allows for efficient circular operations such as enqueueing and dequeuing.
How does the 'enqueue' operation work in a circular queue?
-In the 'enqueue' operation, a new node is added to the queue. If the queue is empty, the new node points to itself. If not, the new node's next points to the front of the queue, and the rear node's next points to the new node.
What is the role of the 'rear' pointer in a circular queue implemented with a linked list?
-The 'rear' pointer tracks the last node in the queue. It is crucial for adding new elements and managing the circular structure since the rear node’s 'next' always points to the front of the queue.
How is the 'dequeue' operation different when there’s only one node in the queue?
-When there’s only one node in the queue, the 'dequeue' operation sets the 'rear' pointer to null, indicating that the queue is empty. For more than one node, it updates the 'rear.next' pointer to remove the front node.
What happens if you try to dequeue from an empty circular queue?
-If you try to dequeue from an empty circular queue, the method will throw a 'NoSuchElementException' to indicate that the queue is empty and no elements can be removed.
What is the purpose of the 'peek' method in a circular queue?
-The 'peek' method allows you to view the front element of the queue without removing it. It retrieves the data from the node pointed to by 'rear.next' if the queue is not empty.
How do you determine if a circular queue is empty?
-A circular queue is considered empty if the 'rear' pointer is null. In that case, there are no elements in the queue.
What is the time complexity of the enqueue, dequeue, peek, and isEmpty methods in a circular queue?
-All of these methods have a time complexity of O(1), meaning they execute in constant time regardless of the size of the queue.
Outlines
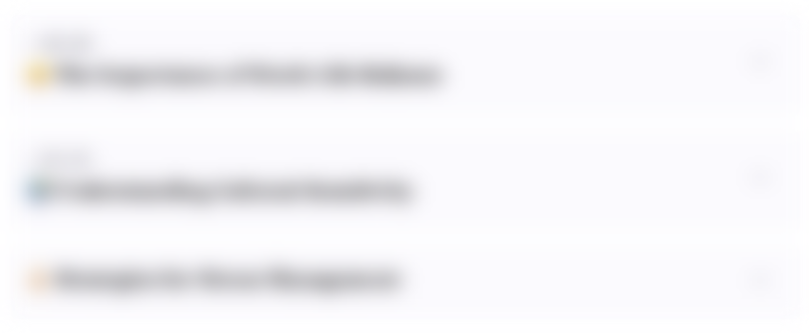
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
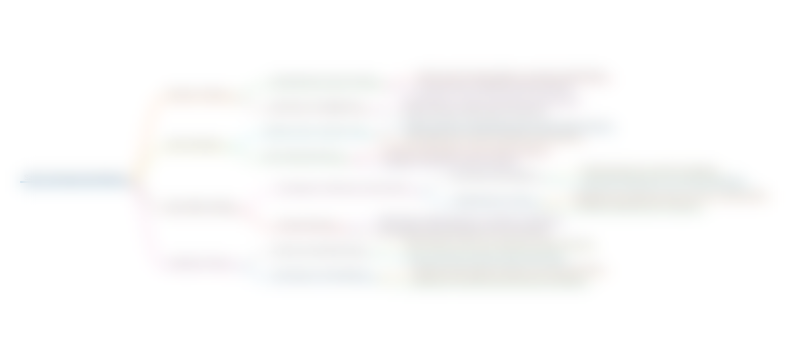
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
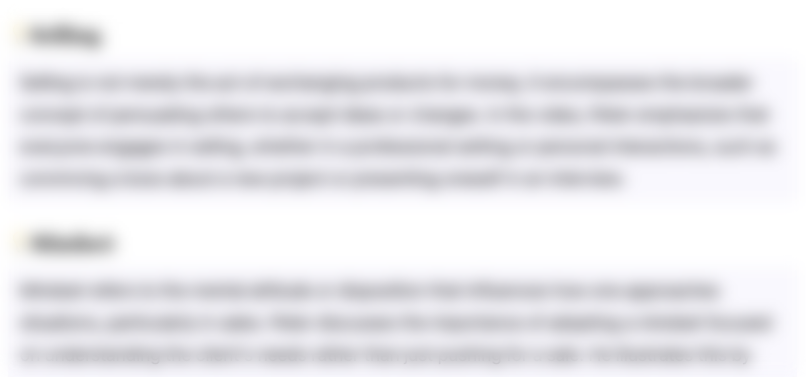
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
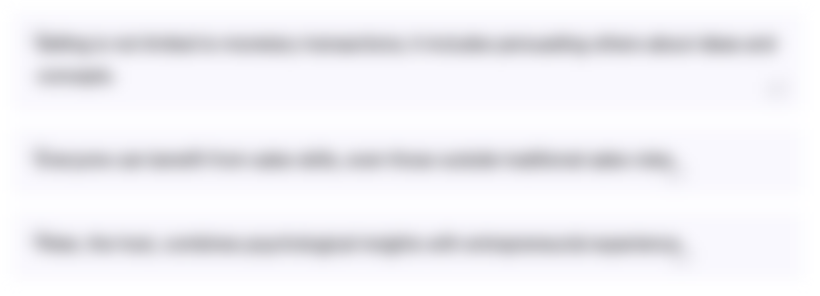
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
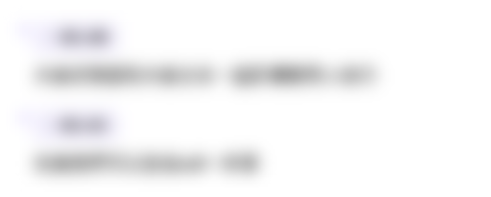
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тариф5.0 / 5 (0 votes)