2D arrays - A Level Computer Science
Summary
TLDRIn this informative video, Mr. Palmer explains the fundamentals of two-dimensional arrays in programming. He begins by defining arrays as collections of the same data type, highlighting their memory allocation and indexing. The discussion covers one-dimensional arrays, leading into two-dimensional arrays, which are structured as arrays of arrays, suitable for storing related data, such as student names. Mr. Palmer emphasizes memory representation, access methods, and practical looping techniques for both 1D and 2D arrays, equipping viewers with essential skills for effective array manipulation.
Takeaways
- 😀 An array is a collection of data elements of the same type stored at contiguous memory locations.
- 😀 One-dimensional arrays can be declared in Java using the syntax: String[] arrayName = new String[size];
- 😀 Array indexing starts at 0, meaning the last index is always one less than the total number of elements.
- 😀 Two-dimensional arrays can be visualized as an array of arrays, where each element points to another array.
- 😀 In Java, a two-dimensional array can be declared as: String[][] arrayName = new String[rows][columns];
- 😀 The memory for a two-dimensional array is allocated in a linear fashion, with each row being a separate one-dimensional array.
- 😀 To access elements in a two-dimensional array, use the syntax: arrayName[rowIndex][columnIndex].
- 😀 Looping through an array efficiently is done using nested loops, where the outer loop iterates through rows and the inner loop through columns.
- 😀 The length of a one-dimensional array can be obtained using: arrayName.length.
- 😀 To find the number of columns in a two-dimensional array, use: arrayName[0].length.
Q & A
What are arrays, and how are they defined?
-Arrays are collections of data that are all of the same type, stored in contiguous memory locations. Each element in the array can be accessed using an index.
How is a one-dimensional array declared in Java?
-A one-dimensional array in Java is declared using the syntax: 'String[] arrayName = new String[length];' where 'length' specifies the number of elements in the array.
What is the significance of a zero-based index in arrays?
-In zero-based indexing, the first element of an array is accessed with index 0. This means that for an array of length 'n', the last element is accessed with index 'n-1'.
How can two-dimensional arrays be visualized?
-Two-dimensional arrays can be visualized as a grid or table with rows and columns, where each row can store multiple items of related data.
What is the difference between a one-dimensional and a two-dimensional array?
-A one-dimensional array stores a linear sequence of elements, while a two-dimensional array stores elements in a matrix format, allowing for more complex data organization.
How is memory organized for multi-dimensional arrays?
-In memory, multi-dimensional arrays are represented as an array of arrays. Each element of the main array points to another array in memory, which stores the actual data.
How can you access an element in a two-dimensional array?
-To access an element in a two-dimensional array, you use two indices: array[rowIndex][columnIndex], where 'rowIndex' specifies the row and 'columnIndex' specifies the column.
What is the method to determine the length of an array in Java?
-In Java, you can determine the length of an array using 'arrayName.length' for one-dimensional arrays, and 'arrayName[0].length' for the number of columns in a two-dimensional array.
How do you loop through a one-dimensional array?
-To loop through a one-dimensional array, you can use a for loop that iterates from 0 to 'array.length - 1' and accesses each element with 'array[i]'.
What is the looping mechanism for iterating over a two-dimensional array?
-To iterate over a two-dimensional array, you can use nested loops: an outer loop for the rows and an inner loop for the columns, accessing elements using array[row][column].
Outlines
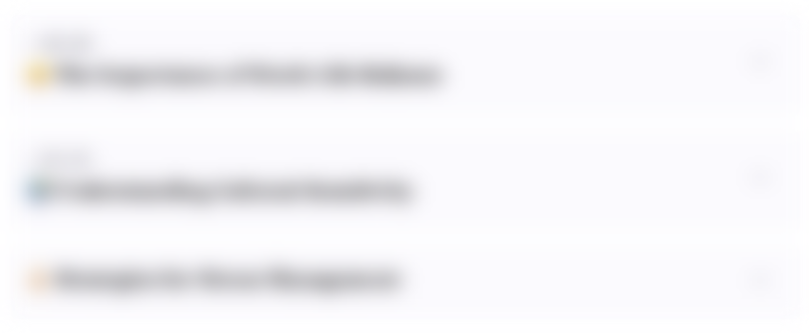
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
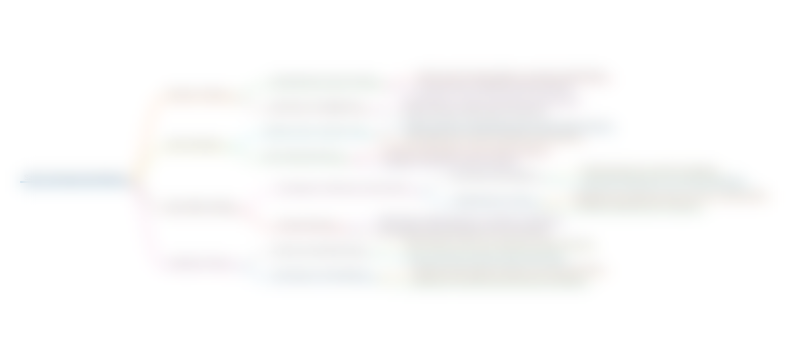
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
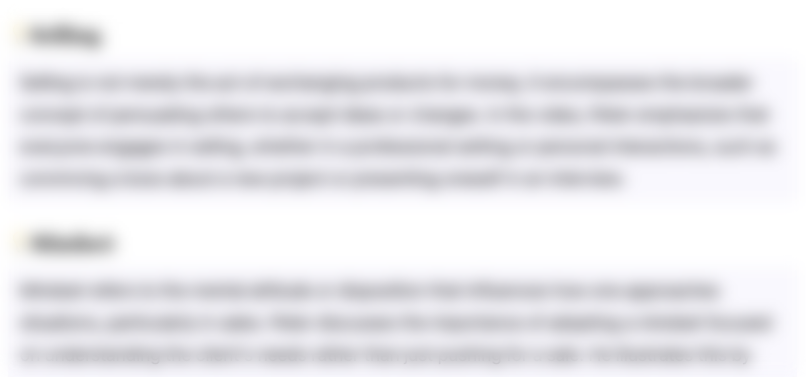
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
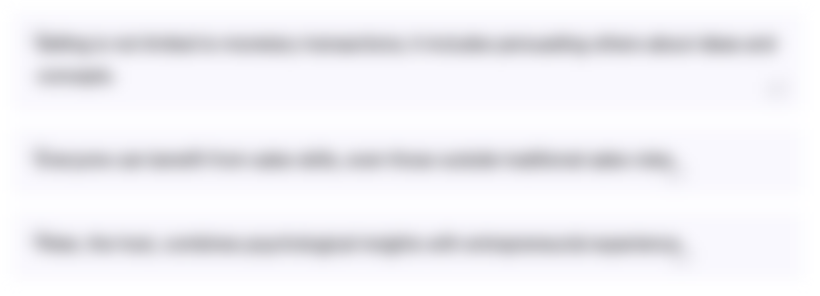
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
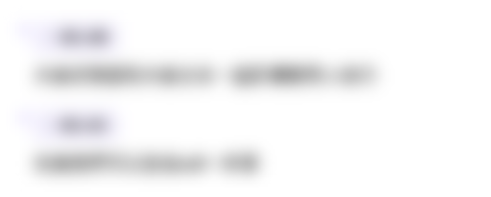
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
5.0 / 5 (0 votes)