Longest Substring Without Repeating Characters - Leetcode 3 - Sliding Window (Python)
Summary
TLDRIn this video, Greg demonstrates how to tackle the coding problem of finding the length of the longest substring without repeating characters using the sliding window technique. He explains the concept of substrings, the importance of maintaining a valid window with unique characters, and provides a step-by-step approach to implementing the algorithm. By using a set to track characters and adjusting pointers as needed, the solution achieves linear time complexity, making it efficient for coding interviews. This foundational technique is essential for mastering data structures and algorithms.
Takeaways
- 😀 The problem focuses on finding the length of the longest substring without repeating characters, a common coding interview question.
- 🔍 A substring is defined as a contiguous, non-empty sequence of characters within a string.
- 🧩 The sliding window algorithm is an effective approach for solving this problem, involving two pointers (L and R) to track the current substring.
- 📈 The time complexity of this algorithm is O(n), making it efficient for large strings.
- 📦 A set data structure is utilized to keep track of characters in the current substring, allowing for constant-time lookups.
- ❗ The window is considered valid if it contains no duplicate characters; otherwise, adjustments must be made by moving the left pointer (L).
- 🔄 When a duplicate character is encountered, the algorithm removes characters from the left until the window becomes valid again.
- ✏️ The length of the current substring can be calculated using the formula: R - L + 1.
- 📊 The space complexity of this algorithm is also O(n) due to the use of a set to store characters.
- 👍 The video emphasizes that mastering the sliding window technique is fundamental for tackling various data structure and algorithm problems.
Q & A
What is the primary focus of the video script?
-The video script focuses on discussing the significance of effective communication in both personal and professional settings, highlighting its impact on relationships and teamwork.
How does the script define effective communication?
-Effective communication is defined as the ability to convey information clearly and understandably, ensuring that the message is received as intended by the listener.
What are some key elements of effective communication mentioned in the script?
-Key elements include active listening, clarity in message delivery, nonverbal cues, empathy, and feedback.
Why is active listening important according to the script?
-Active listening is important because it helps build trust and understanding, allowing the speaker to feel valued and fostering a more open dialogue.
What role does nonverbal communication play in the message delivery?
-Nonverbal communication plays a crucial role by reinforcing or contradicting spoken words, conveying emotions, and providing context to the verbal message.
How can clarity in communication improve teamwork?
-Clarity in communication can improve teamwork by minimizing misunderstandings, ensuring that all team members are on the same page, and enhancing overall productivity.
What strategies does the script suggest for improving communication skills?
-Strategies include practicing active listening, seeking feedback, being mindful of body language, and engaging in regular communication training or workshops.
What is the impact of empathy on communication as discussed in the script?
-Empathy enhances communication by allowing individuals to connect on a personal level, understand diverse perspectives, and respond more thoughtfully to others.
How does feedback contribute to effective communication?
-Feedback contributes by providing opportunities for improvement, clarifying misunderstandings, and fostering an environment of open communication where individuals feel comfortable expressing their thoughts.
What overall message does the script convey about the importance of communication?
-The overall message emphasizes that effective communication is vital for successful relationships, collaboration, and achieving common goals, both in personal and professional contexts.
Outlines
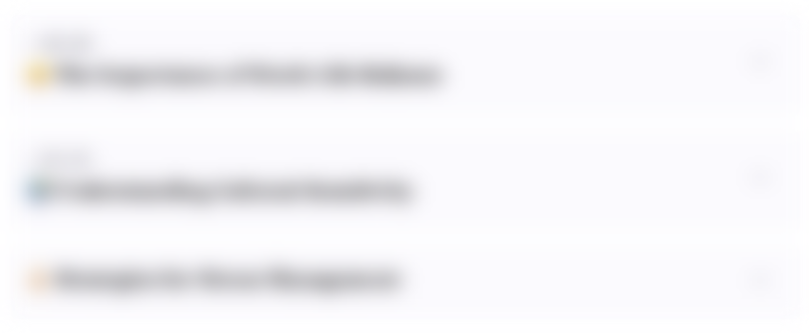
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
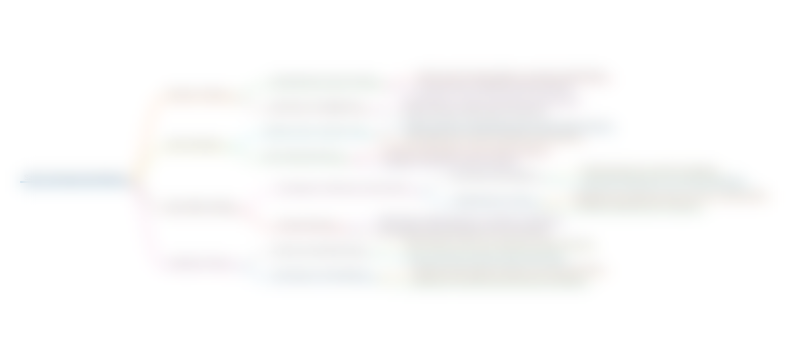
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
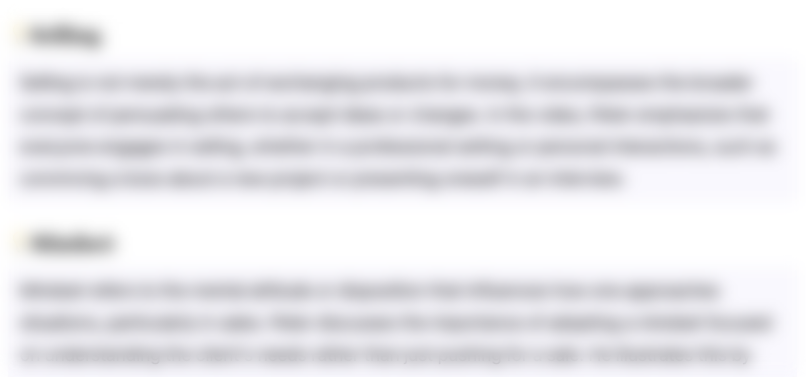
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
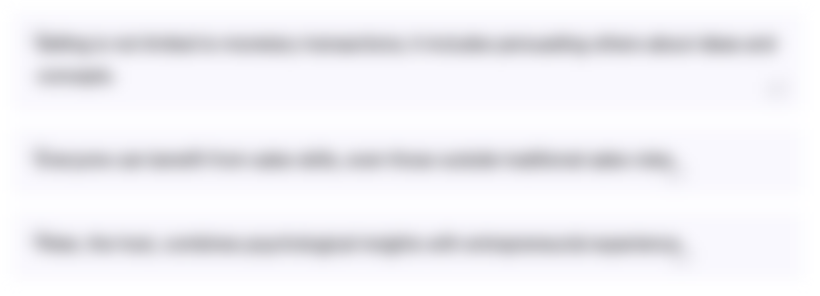
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
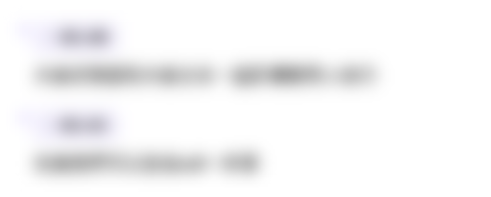
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

Sliding Window in 7 minutes | LeetCode Pattern
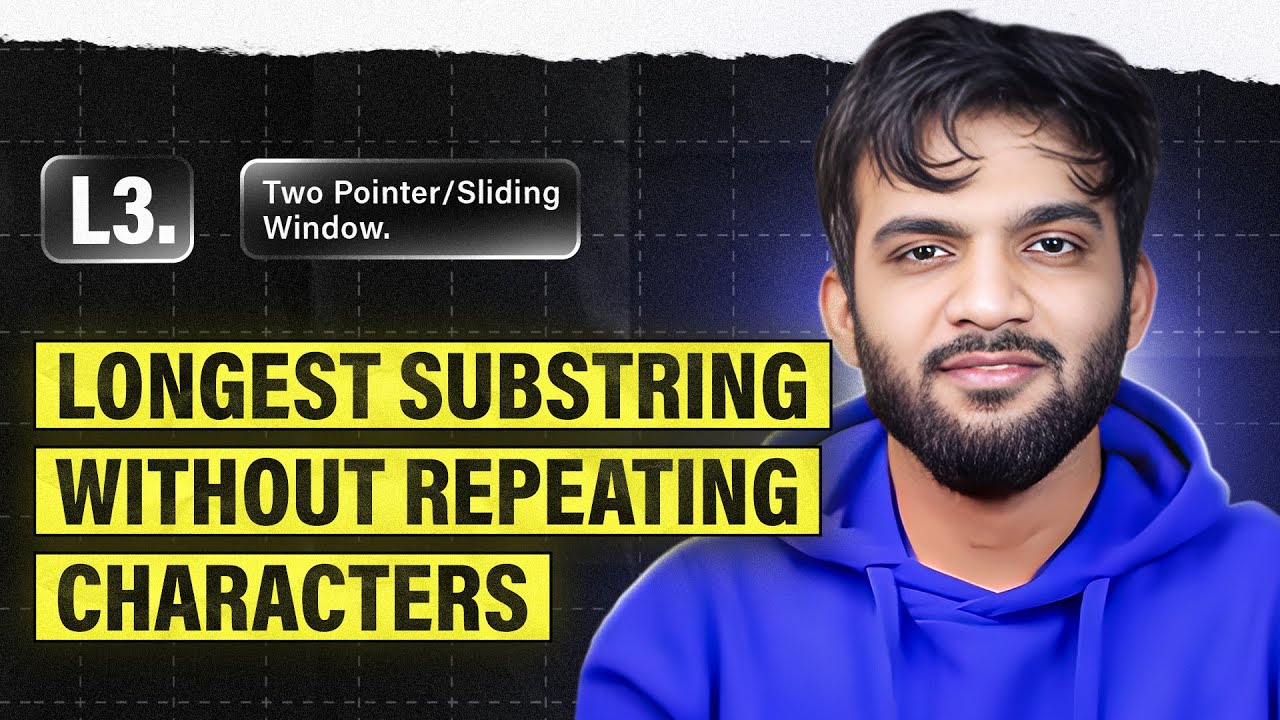
L3. Longest Substring Without Repeating Characters | 2 Pointers and Sliding Window Playlist
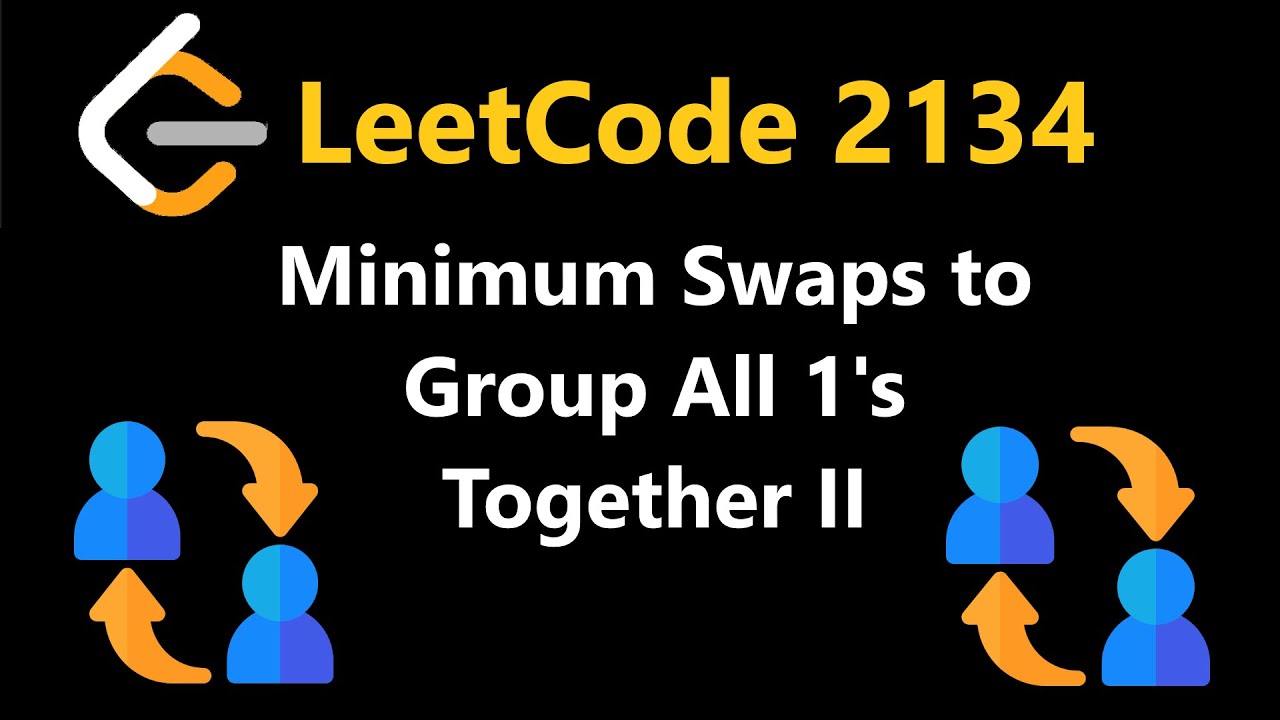
Minimum Swaps to Group All 1's Together II - Leetcode 2134 - Python

5 steps to solve any Dynamic Programming problem
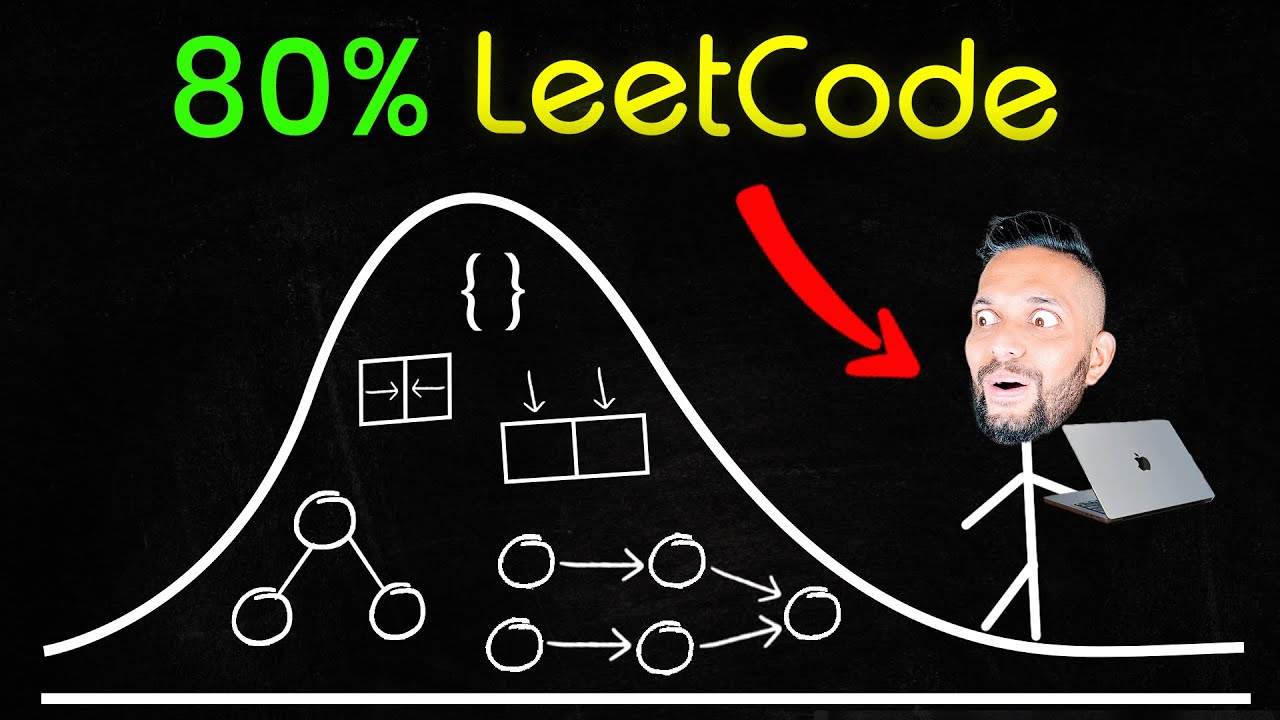
8 patterns to solve 80% Leetcode problems

4 Steps to Math Problem Solving
5.0 / 5 (0 votes)