8 Data Structures Every Programmer Should Know
Summary
TLDRThe video introduces eight essential data structures that every programmer should know, explaining their definitions, use cases, and performance characteristics. The video covers arrays, linked lists, stacks, queues, hash tables, trees, heaps, and graphs, discussing their importance for optimizing algorithms and code performance. Real-world applications, time complexity, and programming examples are provided for each structure. The creator emphasizes understanding trade-offs and properties of these data structures for better coding, while also suggesting interactive learning tools to reinforce the concepts. The video aims to help viewers become more efficient programmers.
Takeaways
- 📚 Data structures are essential for efficient algorithm design and optimizing code performance.
- 🧱 Arrays are the simplest data structure, storing fixed numbers of values of a single type in contiguous memory for quick access.
- 🔗 Linked lists store elements in non-contiguous memory with nodes that point to the next node, making insertions and deletions fast.
- 🍽 Stacks follow the Last-In-First-Out principle, used for tasks like tracking function calls in recursion and undo/redo operations.
- 🚶♂️ Queues operate on the First-In-First-Out principle, ideal for processes like handling task queues and multi-threading.
- 🔑 Hash tables store key-value pairs, making data retrieval efficient through hash functions, but they can face collisions.
- 🌲 Binary trees and binary search trees organize nodes hierarchically, optimizing searching, inserting, and deleting operations.
- 📉 Heaps are special types of binary trees used in algorithms like Heap Sort and priority queues, ensuring the largest or smallest values are at the top.
- 🛤 Graphs represent relationships between objects, widely used in areas like networking, GPS, and social connections.
- 🛠 Understanding trade-offs between different data structures is crucial for optimizing code based on specific use cases.
Q & A
What is a data structure?
-A data structure is a specific format for storing, accessing, and organizing data. It forms the foundation of efficient algorithm design, problem-solving, and optimizing code performance.
Why should programmers learn data structures?
-Data structures are the fundamental building blocks of programming. Understanding them improves problem-solving, enhances code performance, and helps optimize algorithms. They are essential for developing efficient software, especially when dealing with large datasets.
What is the difference between an array in most programming languages and an array in JavaScript?
-In most programming languages, arrays are fixed in length and contain elements of a single data type. In JavaScript, arrays are dynamically resizable and can contain elements of different data types, making them technically array-like objects.
What is a linked list and how does it differ from an array?
-A linked list is a linear data structure where elements, called nodes, are stored in a sequence with each node containing a value and a pointer to the next node. Unlike arrays, linked lists do not store elements in contiguous memory locations, and they allow efficient insertion and deletion at the beginning.
What is a stack and how does it operate?
-A stack is a data structure that operates on the Last In, First Out (LIFO) principle. It has two main operations: push (inserting an element at the top) and pop (removing and returning the top element). Additional operations include peek (viewing the top element without removing it), and checking if the stack is empty or full.
What is a queue, and where is it commonly used?
-A queue operates on the First In, First Out (FIFO) principle, meaning the first element added is the first one removed. It is used in scenarios where items are processed in order, like task scheduling, printing jobs, and managing processes in multi-threading.
How does a hash table work?
-A hash table stores key-value pairs and uses a hash function to efficiently map keys to values. The hash function determines the index for each key-value pair, allowing for fast lookups. However, collisions can occur when two keys map to the same index, which are resolved using techniques like chaining or open addressing.
What is the difference between a binary tree and a binary search tree?
-A binary tree is a hierarchical structure where each node has at most two children. A binary search tree (BST) is a specialized form of a binary tree where the left child contains values less than the parent, and the right child contains values greater than the parent. This structure enables efficient searching, insertion, and deletion.
What is the significance of heap data structures, and what are its types?
-A heap is a special kind of binary tree where parent nodes are compared to their children based on a heap property. There are two types: Max Heap, where the parent has a greater value than its children, and Min Heap, where the parent has a smaller value than its children. Heaps are used in algorithms like heap sort and priority queues.
What is a graph, and how is it used in computer science?
-A graph is a nonlinear data structure made up of vertices (nodes) and edges connecting them. It represents relationships between elements and is used in applications like GPS routing, social networks, and computer networking. Graphs can be directed (with specified directions between vertices) or undirected.
Outlines
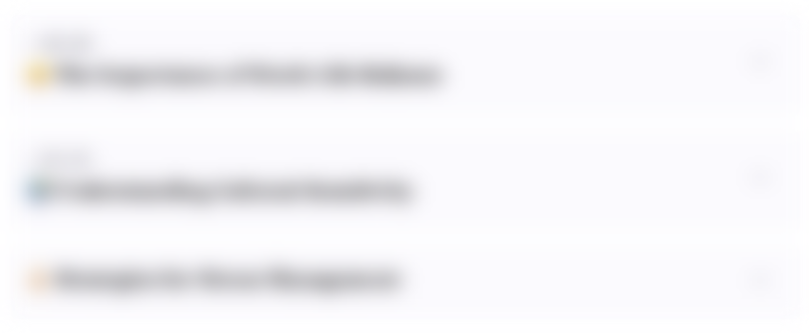
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
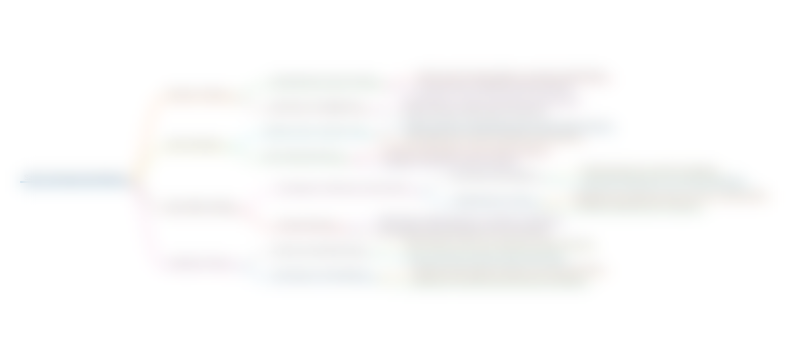
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
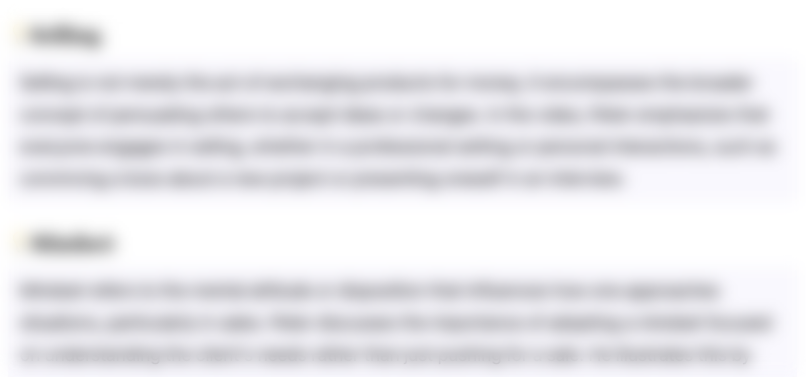
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
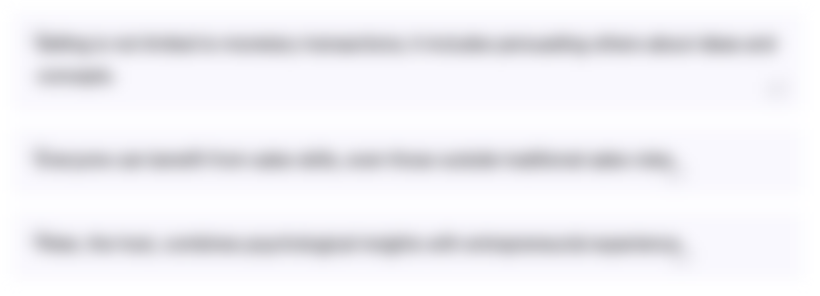
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
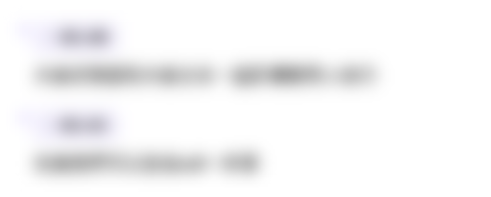
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тариф5.0 / 5 (0 votes)