#18 Two way Data Binding | Angular Components & Directives | A Complete Angular Course
Summary
TLDRThis lecture explains the concept of two-way data binding in Angular. It builds on previous discussions of one-way data binding (property and event binding) by demonstrating how to bind data in both directions—between the component class and the view template. The example provided includes creating a search box component, showcasing how input values update both the view and component class in real time. The video also highlights using Angular's built-in 'ngModel' directive to simplify two-way data binding. This foundational knowledge is essential for implementing dynamic and interactive forms in Angular applications.
Takeaways
- 🔗 One-way data binding involves binding data either from the component class to the view template or vice versa, but not both.
- 🔧 String interpolation and property binding are used to bind data from the component class to the view template.
- 🎯 Event binding is used to bind data from the view template back to the component class.
- 🛠 Two-way data binding allows for data to flow both ways between the component class and the view template, reflecting changes in both directions.
- 📝 An example of two-way data binding is implemented with a search text box in an e-commerce application, where user input updates a property in the component class.
- 📑 The 'search' component is created to handle the search functionality, including a text box and a button.
- 🖌 CSS is used to style the search component for better user interface.
- 🔑 The 'search-text' property in the component class is used to prepopulate the input element in the view template.
- 🔄 Two-way data binding is achieved by combining property binding for setting the initial value and event binding for updating the property when the user types in the input box.
- 📚 The 'FormsModule' from 'angular/forms' must be imported and added to the 'imports' array in the app module to use 'ngModel' for two-way data binding.
- 🔄 Using 'ngModel' simplifies the syntax for two-way data binding, eliminating the need to explicitly combine property and event bindings.
Q & A
What is one-way data binding?
-One-way data binding refers to binding data in a single direction, either from the component class to the view template or from the view template to the component class. It is achieved through string interpolation and property binding for component-to-view data flow and event binding for view-to-component data flow.
What are the two main types of one-way data binding?
-The two main types of one-way data binding are property binding, where data is passed from the component class to the view template, and event binding, where data is passed from the view template to the component class.
What is two-way data binding?
-Two-way data binding allows data to flow in both directions: from the component class to the view template and from the view template back to the component class. It combines both property binding and event binding.
How can two-way data binding be implemented?
-Two-way data binding can be implemented by combining property binding and event binding. It can also be achieved using the built-in Angular directive 'ngModel', which simplifies the process.
What is the role of the 'ngModel' directive in two-way data binding?
-The 'ngModel' directive is used to achieve two-way data binding in a simplified manner. It binds an input element to a component property, allowing changes in the view to update the component property and vice versa.
What error might you encounter when using 'ngModel' and how can it be fixed?
-When using 'ngModel', an error stating 'Can't bind to ngModel since it isn't a known property of input' may occur. This can be fixed by importing the 'FormsModule' from '@angular/forms' and registering it in the 'Imports' array of the module.
What is the initial step when starting to implement the search functionality in the application?
-The initial step is to create a new search component inside the 'product-list' component using Angular's 'ng generate' command.
How can you pre-populate an input element with a property value in Angular?
-To pre-populate an input element with a property value, you can use one-way property binding by binding the input element's 'value' property to the desired component property using square brackets, e.g., '[value] = searchText'.
What does event binding achieve in this context?
-Event binding allows the input event in the text box to update the corresponding component property (e.g., 'searchText') when the user types something in the text box. It ensures that changes in the view are reflected in the component class.
What is the purpose of combining property and event binding?
-Combining property and event binding allows two-way data binding, enabling the data to be synchronized between the component class and the view template in both directions. This ensures that any change made in the view is reflected in the component and vice versa.
Outlines
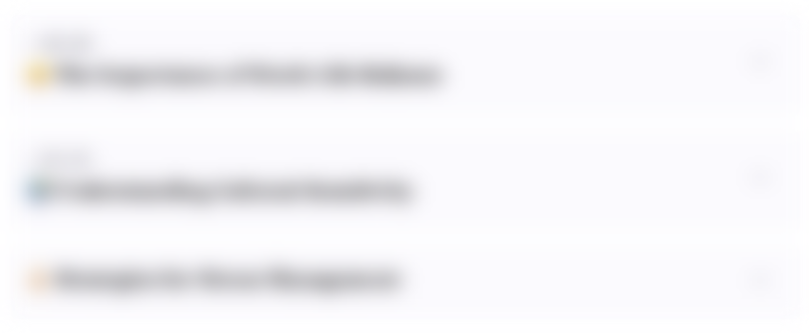
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
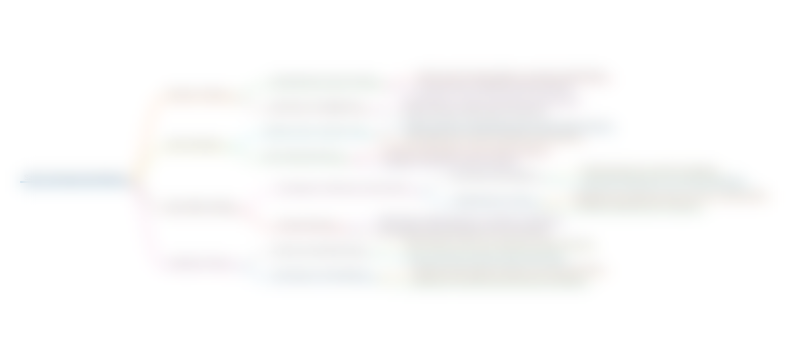
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
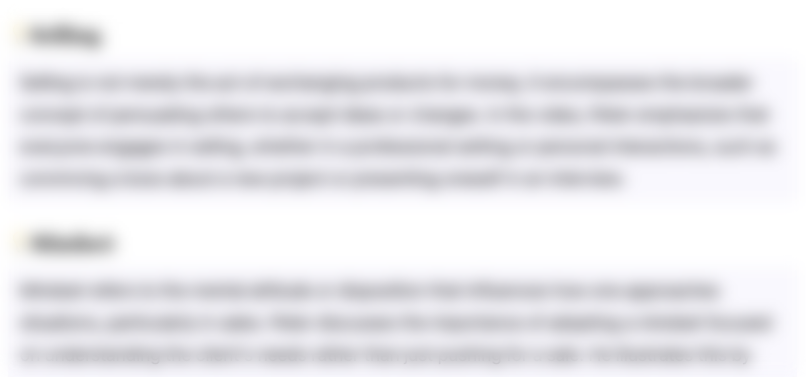
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
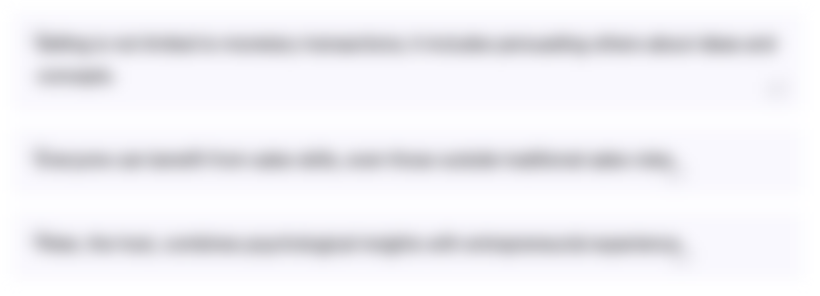
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
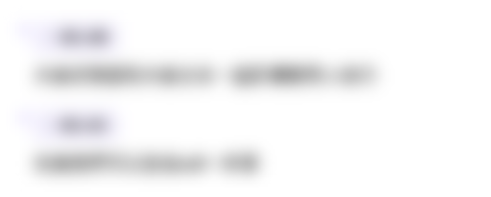
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
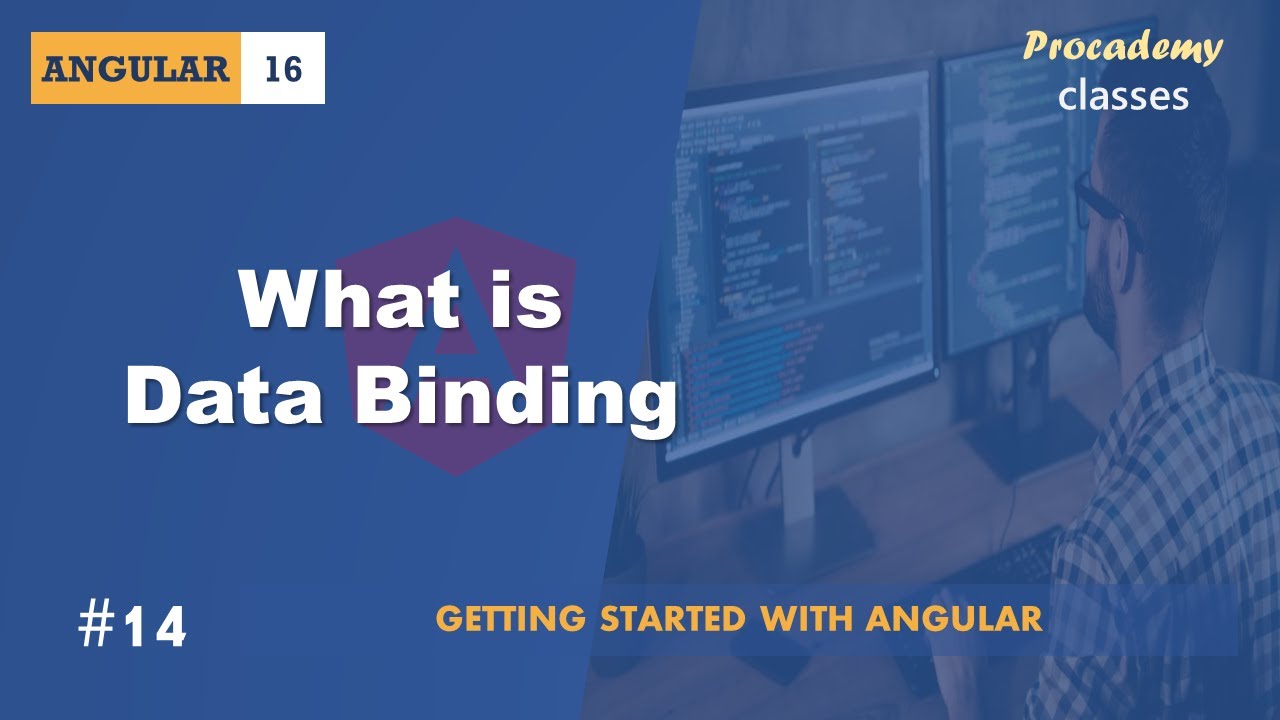
#14 What is Data Binding | Angular Components & Directives | A Complete Angular Course

#17 Event Binding | Angular Components & Directives | A Complete Angular Course
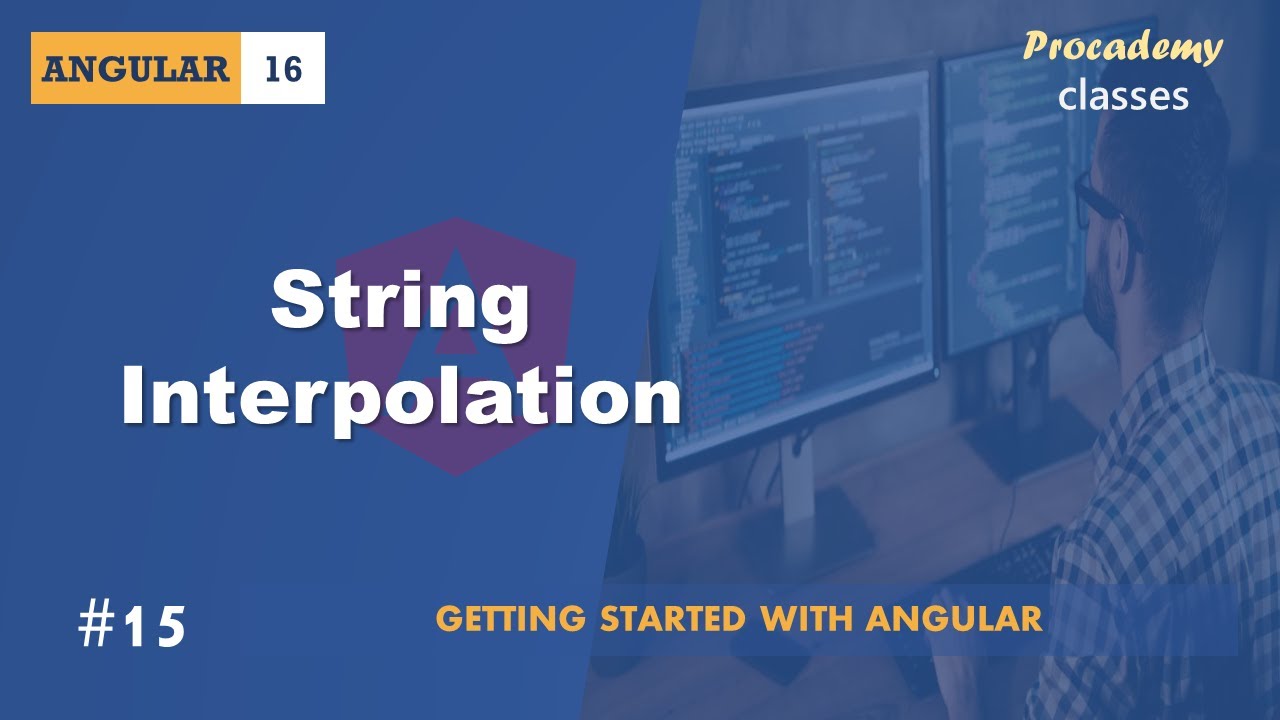
#15 String Interpolation | Angular Components & Directives | A Complete Angular Course
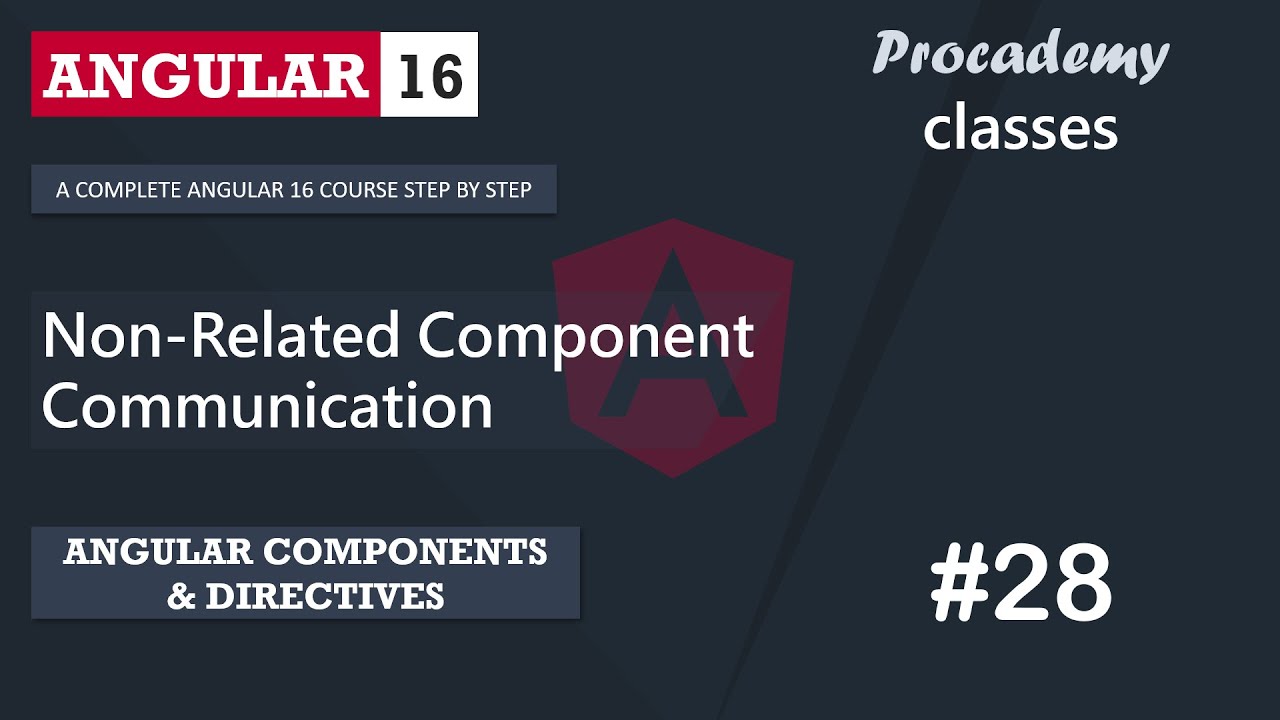
#28 Non-Related Component Communication | Angular Component & Directives | A Complete Angular Course
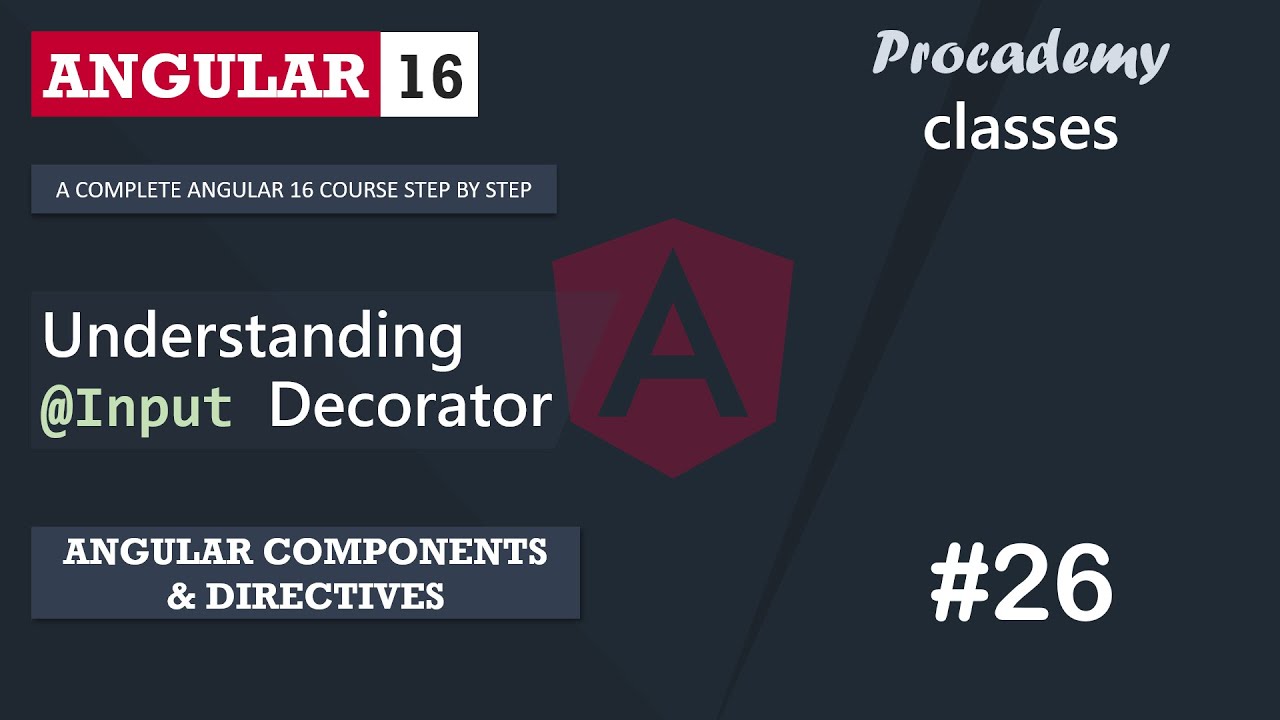
#26 Understanding Input Decorator | Angular Components & Directives | A Complete Angular Course
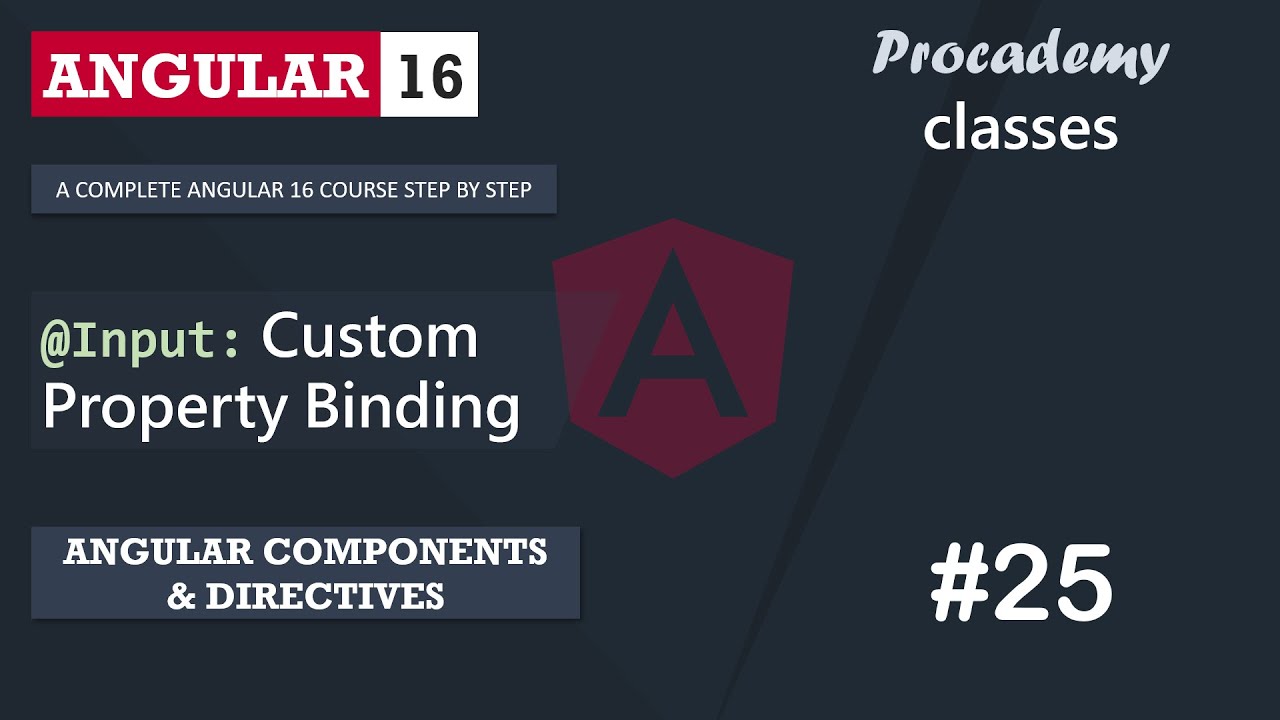
#25 @Input: Custom Property Binding | Angular Components & Directives | A Complete Angular Course
5.0 / 5 (0 votes)