Object Oriented JavaScript Tutorial #1 - Introduction
Summary
TLDRThis introductory tutorial on object-oriented JavaScript explains the core concepts of objects in JavaScript, drawing comparisons to real-life objects like cars with properties and actions. The tutorial demonstrates built-in objects such as arrays and the global window object, discussing their properties and methods. It also explains primitive types like strings and how JavaScript wraps them in objects to provide additional functionality. The video outlines the course structure, covering topics like creating custom objects, using classes and constructors, inheritance, method chaining, and prototype inheritance. Viewers are encouraged to have a basic understanding of JavaScript before proceeding.
Takeaways
- 😀 JavaScript objects are similar to real-life objects, with properties (e.g., color, model) and actions (methods) they can perform.
- 🔧 Methods in JavaScript are just functions associated with objects, and objects have properties and methods.
- 📋 Arrays are objects in JavaScript, and they have properties (e.g., length) and methods (e.g., sort).
- 🖥️ The window object is a global object in JavaScript, providing many methods and properties like `innerWidth`.
- 💡 Not everything in JavaScript is an object; primitive types like numbers, booleans, and strings are not objects.
- ⚙️ JavaScript can wrap primitive types (e.g., strings) in an object temporarily, allowing them to behave like objects.
- 🧱 You can create string objects explicitly using the `new String()` method, which provides access to properties and methods.
- 🚗 JavaScript allows users to create custom objects, such as a car object, with properties and methods like `drive` or `reverse`.
- 📚 Object-oriented programming (OOP) in JavaScript focuses on creating and managing objects to simplify code structure.
- 🎓 The course will cover object literal notation, JavaScript classes, constructors, inheritance, method chaining, and prototypes.
Q & A
What is an object in JavaScript?
-An object in JavaScript is similar to a real-life object. It can have properties (like color, model, registration number in a car) and methods (things it can do, like drive or reverse). In JavaScript, methods are functions associated with the object.
Can you give an example of a built-in object in JavaScript?
-An example of a built-in object in JavaScript is an array. Arrays have properties like 'length' and methods like 'sort'. Another example is the global 'window' object in the browser, which contains properties like 'innerWidth' and various methods.
What is the 'length' property in a JavaScript array?
-The 'length' property in a JavaScript array returns the number of elements in the array. For example, if you have an array called 'names' with three elements, 'names.length' would return 3.
What are methods in a JavaScript object?
-Methods in a JavaScript object are functions that are associated with the object. For instance, an array object can have methods like 'sort' that sorts the elements of the array.
What is the window object in JavaScript?
-The window object is a global object in JavaScript available in the browser environment. It represents the browser's window and provides properties and methods related to the window, such as 'innerWidth', which returns the width of the window in pixels.
Are all types in JavaScript objects?
-No, not everything in JavaScript is an object. Primitive types like null, numbers, booleans, and strings are not objects. However, they can temporarily behave like objects when JavaScript wraps them in an object for certain operations.
How does JavaScript handle primitive types like strings when you use object-like properties or methods?
-JavaScript temporarily wraps primitive types, like strings, in an object so that object-like properties (e.g., 'length') or methods can be called on them. For example, calling 'name.length' on a string would work because JavaScript wraps the string in an object behind the scenes.
What is the difference between creating a primitive string and a string object in JavaScript?
-A primitive string can be created by assigning a value directly, like 'var name = "Mario"', while a string object is created using the 'new String()' constructor, like 'var name2 = new String("Ryu")'. The string object can be expanded to see properties and methods, unlike a primitive string.
Can you create your own objects in JavaScript?
-Yes, you can create your own objects in JavaScript. For example, you can create an object that represents a car, with properties like 'color' and 'make', and methods like 'drive' or 'reverse'.
What topics will be covered in this object-oriented JavaScript tutorial series?
-The tutorial series will cover creating objects using object literal notation, JavaScript classes and constructors, inheritance, method chaining, and exploring prototypes and prototype inheritance.
Outlines
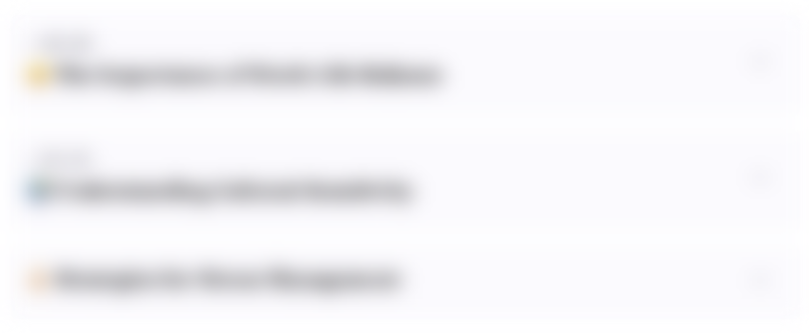
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
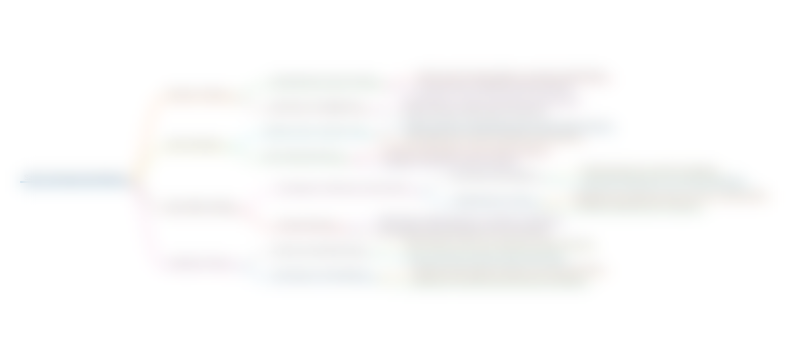
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
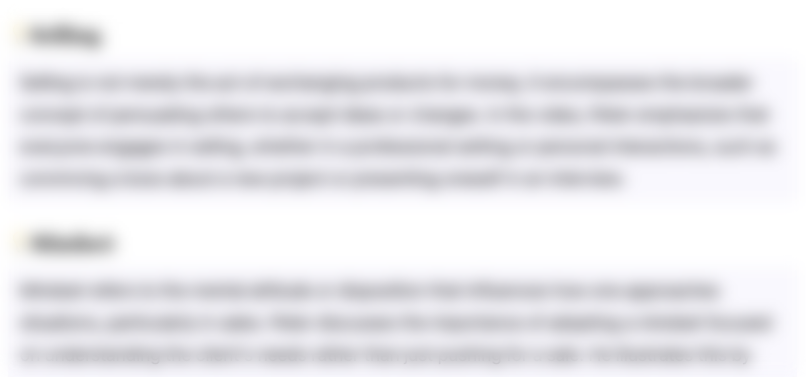
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
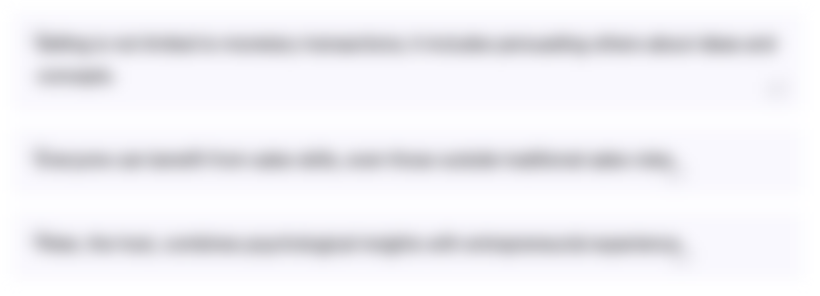
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
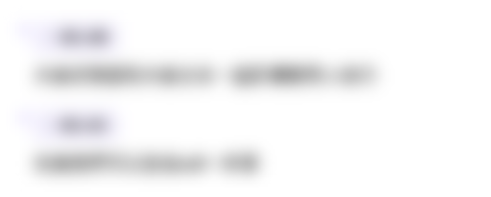
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
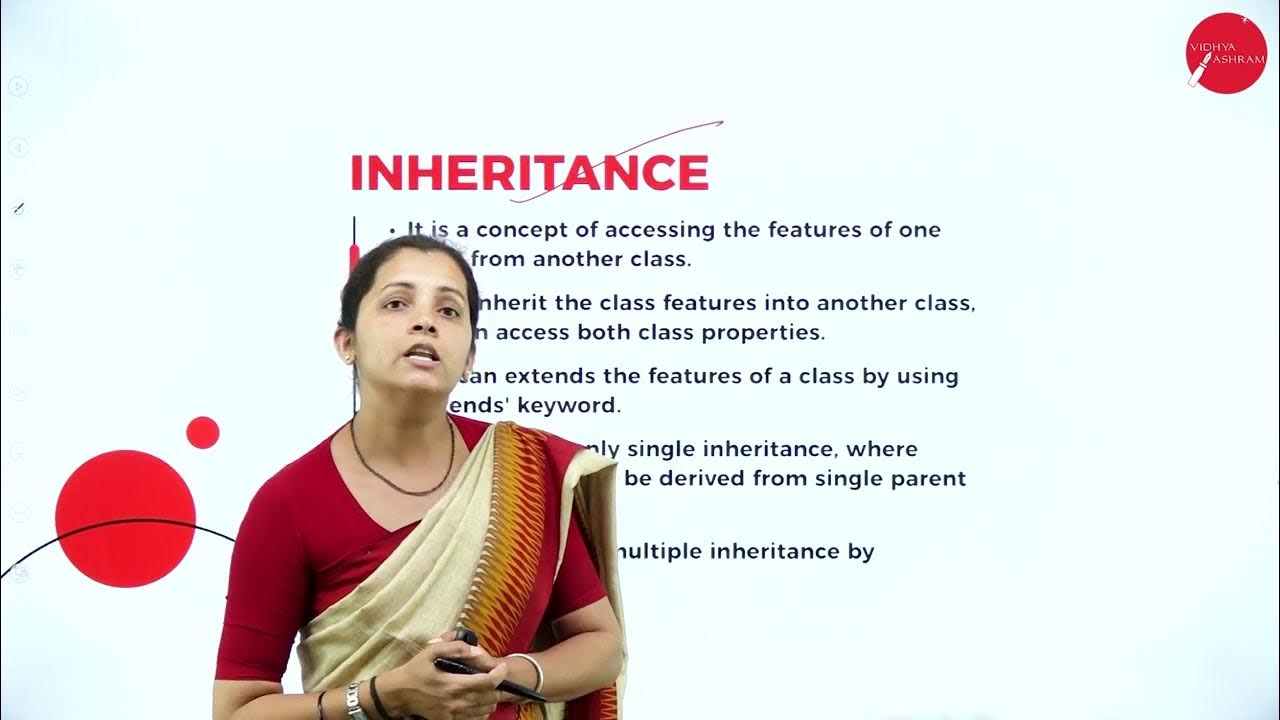
DAY 07 | PHP AND MYSQL | VI SEM | B.CA | CLASS AND OBJECTS IN PHP | L1
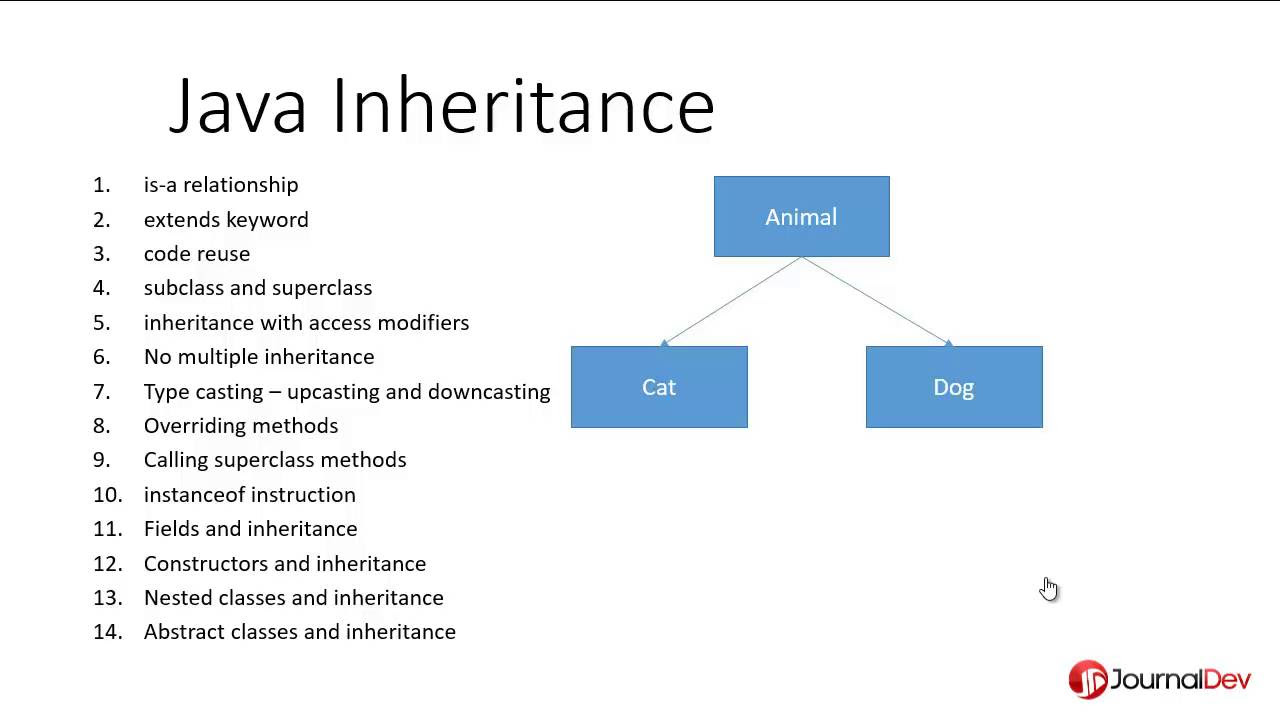
Inheritance in Java - Java Inheritance Tutorial - Part 2
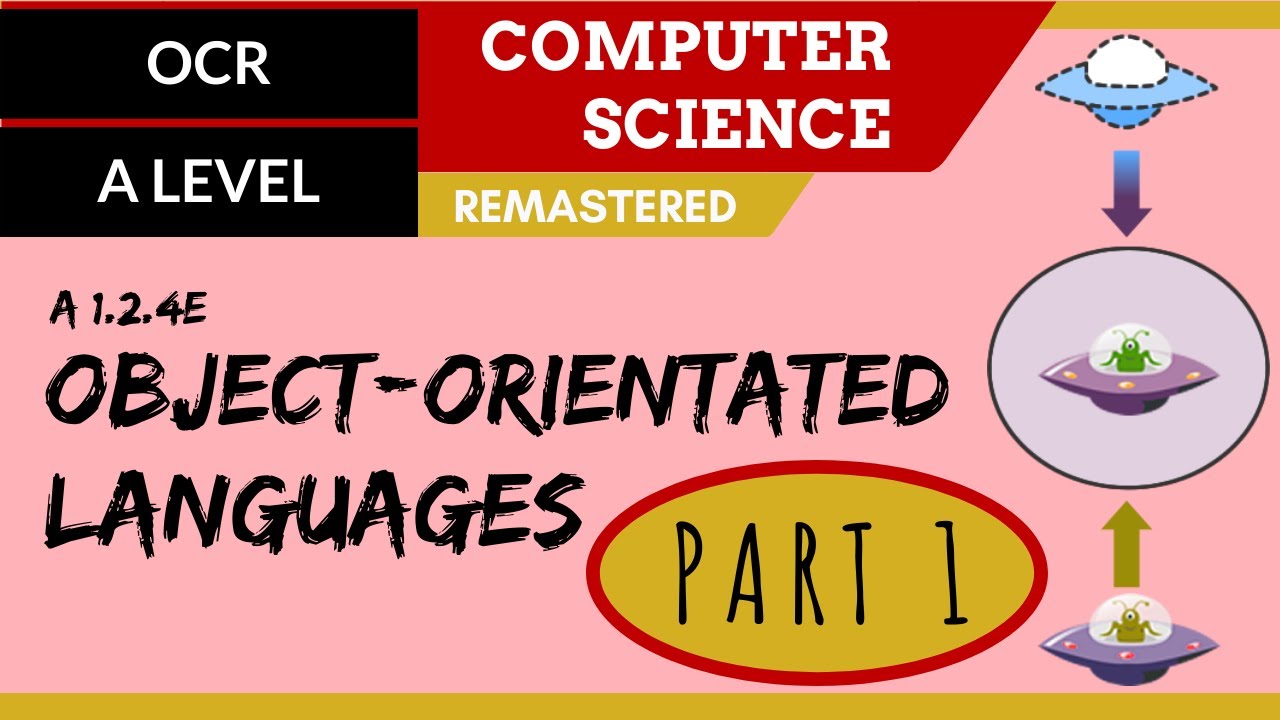
36. OCR A Level (H446) SLR7 - 1.2 Object-oriented languages part 1
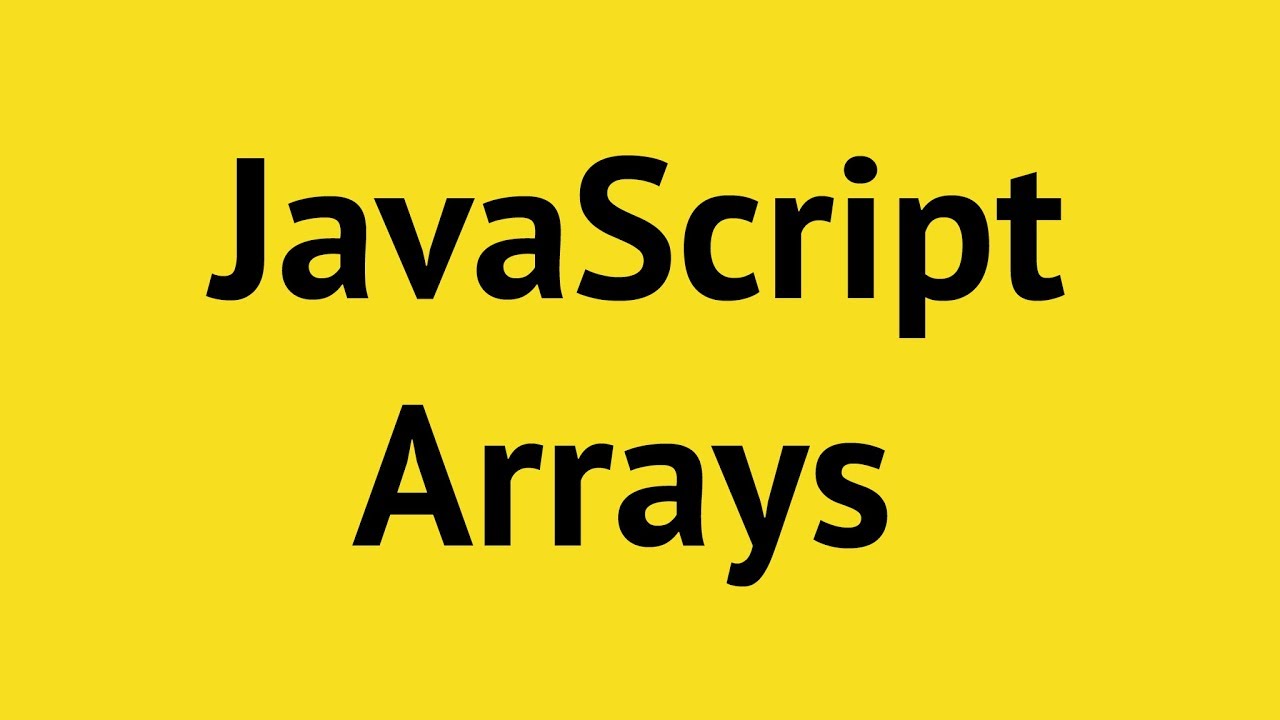
JavaScript Arrays

Object Methods | JavaScript 🔥 | Lecture 041

Dot vs Bracket Notation | JavaScript 🔥 | Lecture 040
5.0 / 5 (0 votes)