Prim's Algorithm - Implementation in Python
Summary
TLDRIn this video, Alessia explains Prim's Algorithm, a method for finding the minimum spanning tree in a connected, weighted graph. She covers the algorithm's steps, data structures like vertices, edges, and priority queues, and its implementation. The video also includes tests to validate the algorithm and discusses its time and space complexity. Alessia concludes with real-world applications, like designing road networks and electrical grids.
Takeaways
- 🌳 Prim's Algorithm calculates the minimum spanning tree (MST) in a connected and weighted graph starting from a selected vertex.
- 🔍 A spanning tree connects all vertices in a graph, and a minimum spanning tree does so with the smallest possible total edge weight.
- 🏃♂️ The algorithm uses a greedy approach, making local decisions without considering the global structure.
- 🔄 Prim's Algorithm repeatedly selects the smallest weight edge that connects the tree to a new vertex.
- 📚 Data structures needed include a graph with vertices and edges, a distance dictionary, and a priority queue for remaining vertices.
- 💻 The Vertex class stores its value, edges, parent vertex, and a boolean for tree inclusion.
- 🔗 The Edge class represents connections between vertices.
- 📈 The Graph class manages vertices and their addition to the MST.
- 🔄 The algorithm initializes distances to infinity, then iteratively updates them based on edge weights.
- 🔍 The final weight of the MST is calculated by summing the weights of edges connecting vertices to the tree.
- 📊 The space complexity of Prim's Algorithm is O(V), considering the dictionary and priority queue.
- ⏱️ The time complexity is O((v + e) log v), which approximates to O(e log v) for connected graphs due to a higher number of edges.
- 🚀 Prim's Algorithm has applications in designing infrastructure projects like road networks, pipelines, electrical grids, and more.
Q & A
What is Prim's Algorithm?
-Prim's Algorithm is a greedy algorithm that calculates the minimum spanning tree in a connected and weighted graph starting from a selected vertex.
What is a spanning tree?
-A spanning tree of a connected graph is a subset of edges that forms a tree connecting all vertices.
What is a minimum spanning tree?
-A minimum spanning tree is the spanning tree whose sum of edge weights is as small as possible.
How does Prim's Algorithm make decisions?
-Prim's Algorithm uses a greedy approach, making decisions by selecting the best local option without regard to the global structure.
What is the role of the 'distance' dictionary in Prim's Algorithm?
-The 'distance' dictionary keeps track of the cost of adding a vertex to the tree and is initialized to infinity.
What is the purpose of the 'remaining' priority queue?
-The 'remaining' priority queue contains the remaining vertices to add to the tree, with each vertex's priority defined by its value in the distance dictionary.
How does Prim's Algorithm handle disconnected graphs?
-Prim's Algorithm should return a weight equal to infinity when trying to obtain the minimum spanning tree of a disconnected graph.
What data structures are used in the implementation of Prim's Algorithm?
-The implementation uses a Vertex class, an Edge class, a Graph class, a dictionary, a priority queue, and a variable to calculate the weight of the minimum spanning tree.
What is the space complexity of Prim's Algorithm?
-The space complexity of Prim's Algorithm is O(V), considering the use of a dictionary and a priority queue.
What is the time complexity of Prim's Algorithm?
-The time complexity of Prim's Algorithm is O((v + e) log v), which can be approximated to O(e log v) for connected graphs.
What are some applications of Prim's Algorithm?
-Prim's Algorithm can be used to design projects such as road networks, natural gas pipelines, electrical grids, irrigation channels, and fiber-optic grids.
Outlines
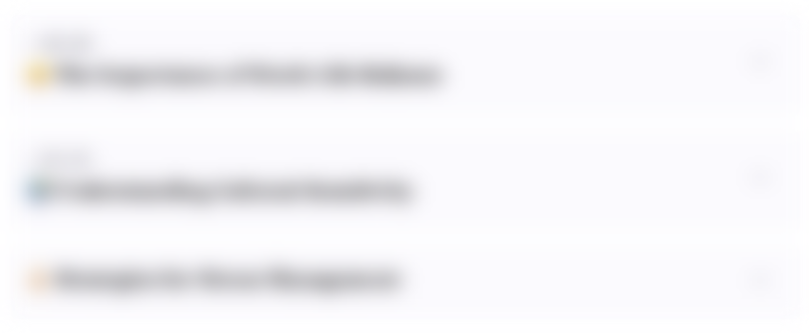
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
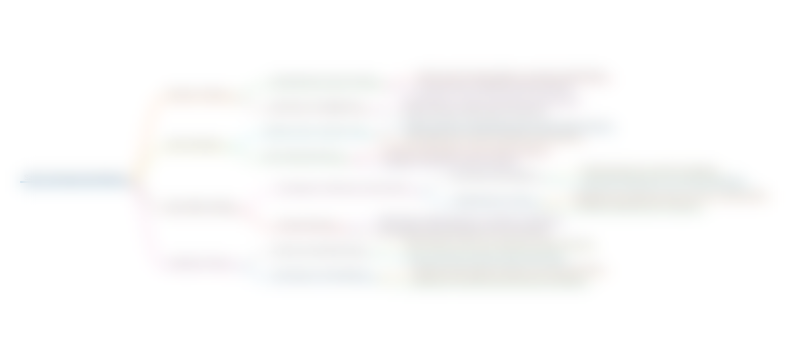
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
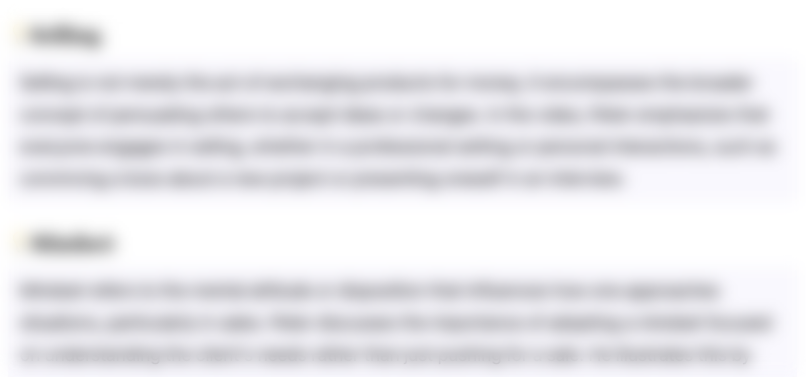
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
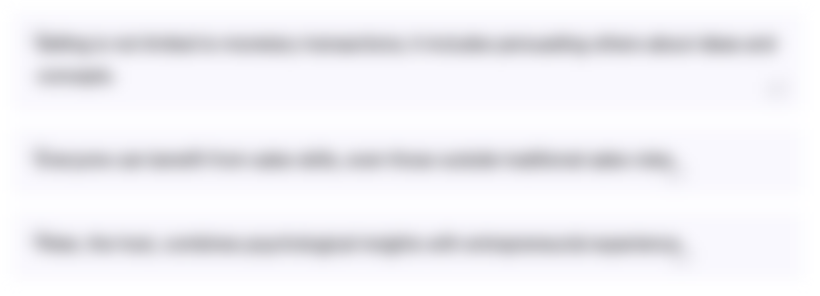
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
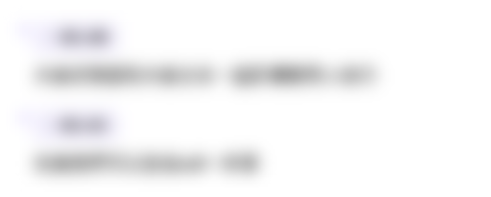
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
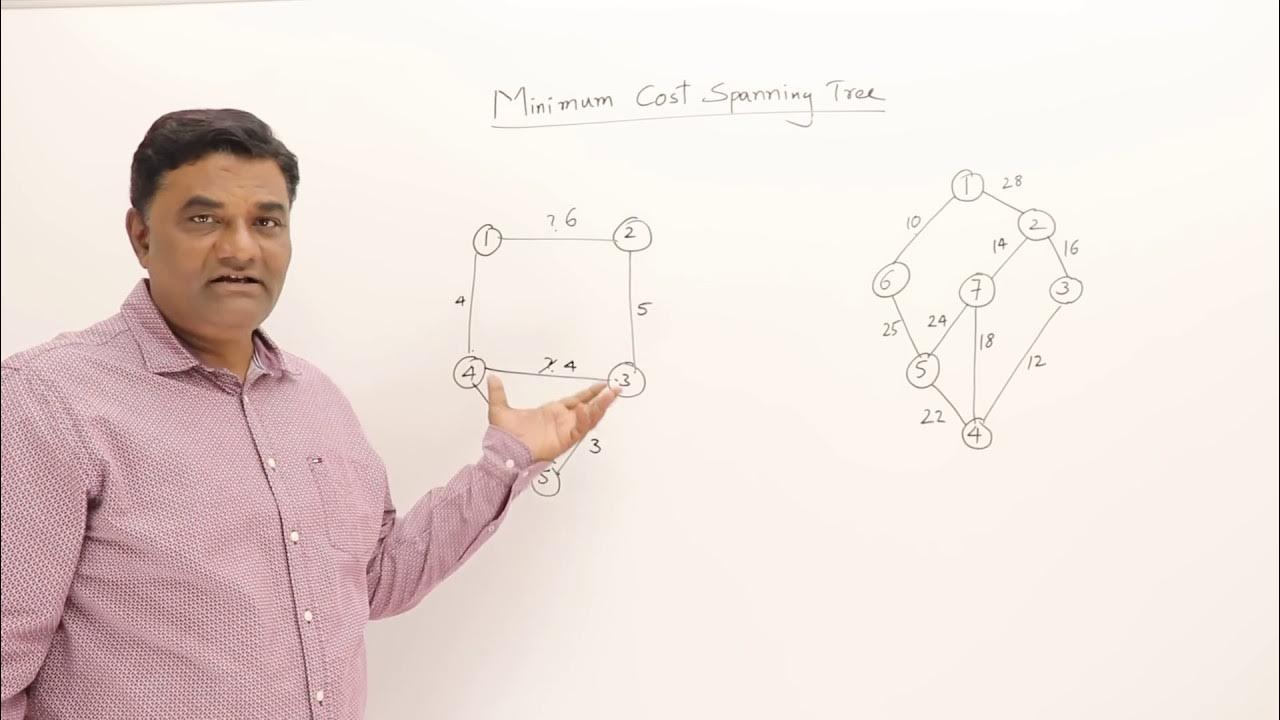
3.5 Prims and Kruskals Algorithms - Greedy Method
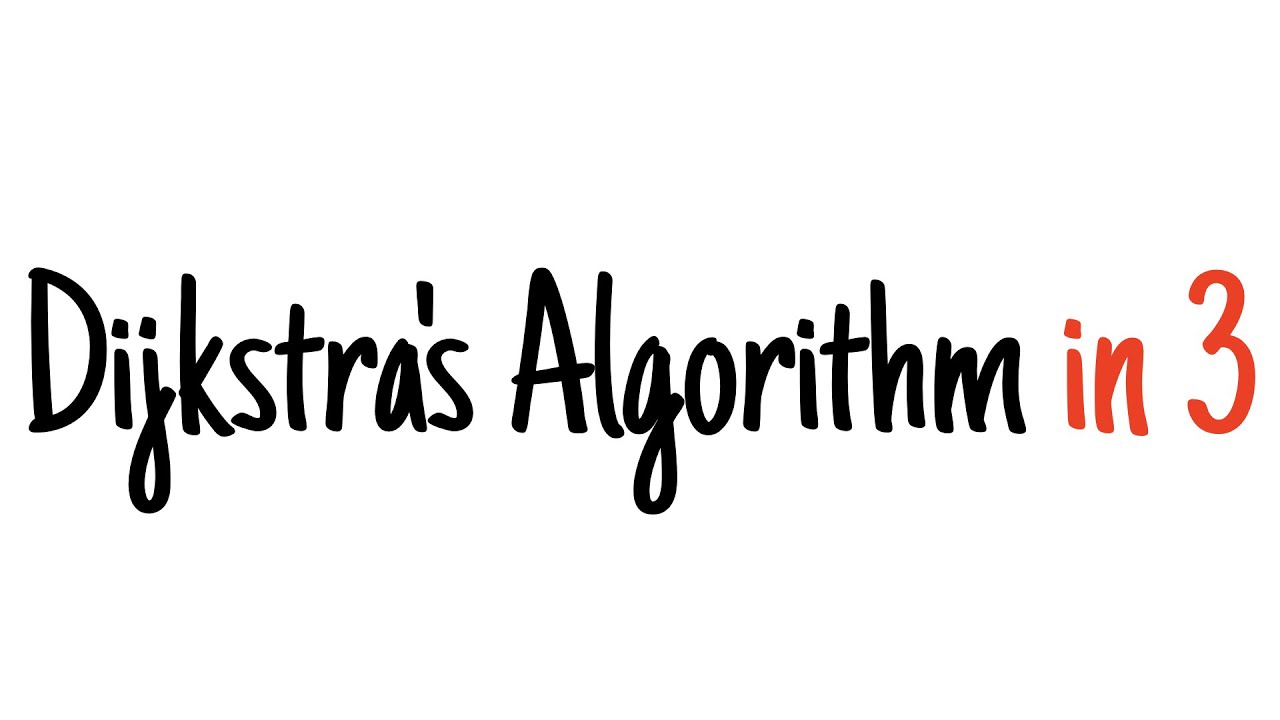
Dijkstra's algorithm in 3 minutes

ALGORITMA DIJKSTRA
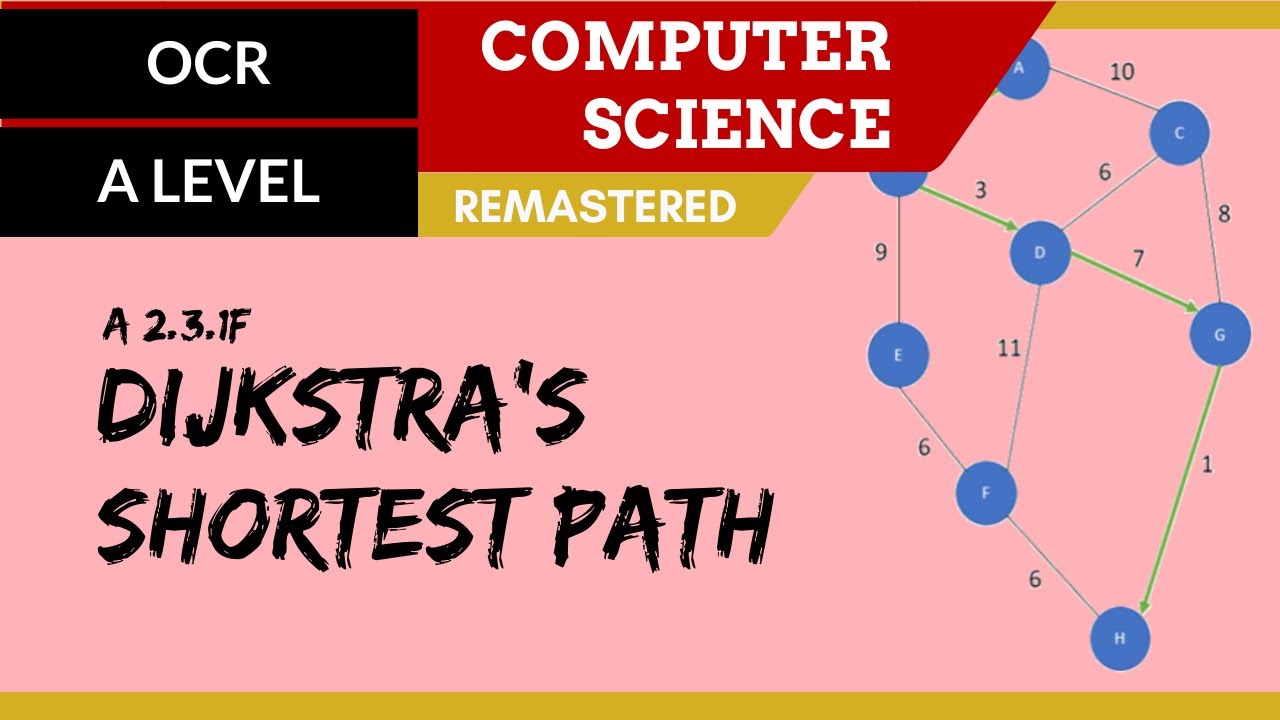
157. OCR A Level (H446) SLR26 - 2.3 Dijkstra's shortest path
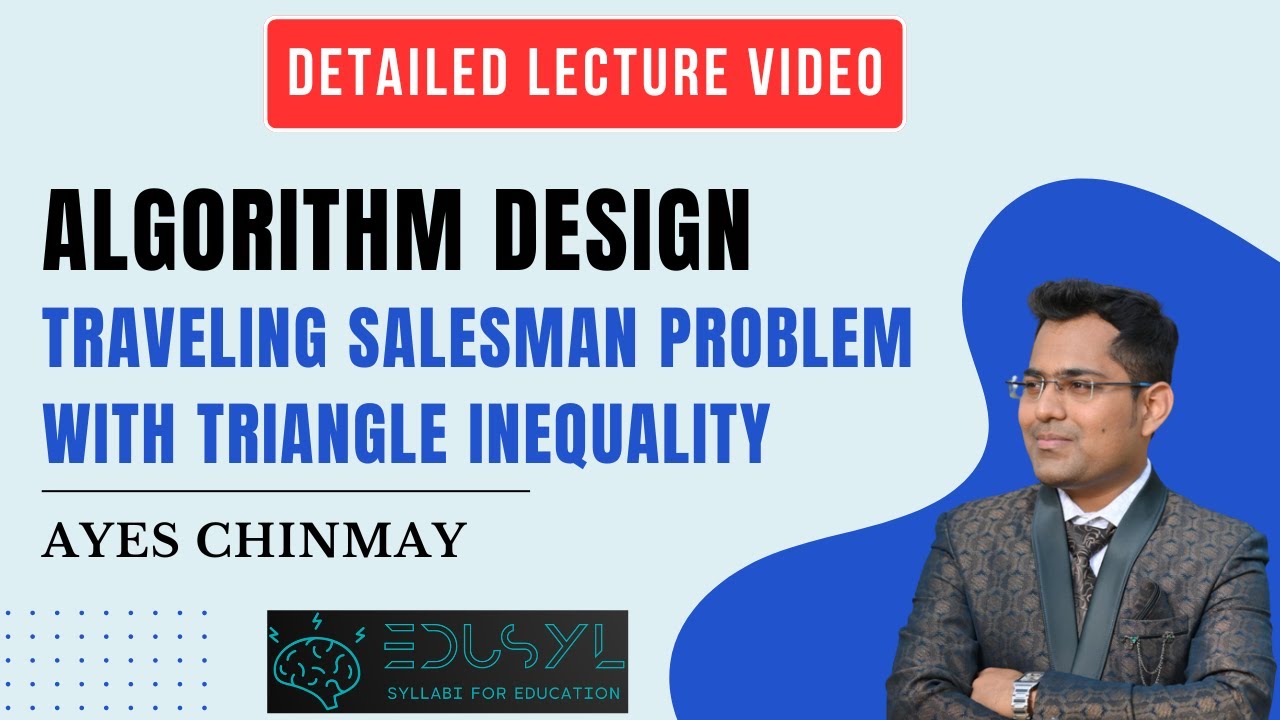
Algorithm Design | Approximation Algorithm | Traveling Salesman Problem with Triangle Inequality
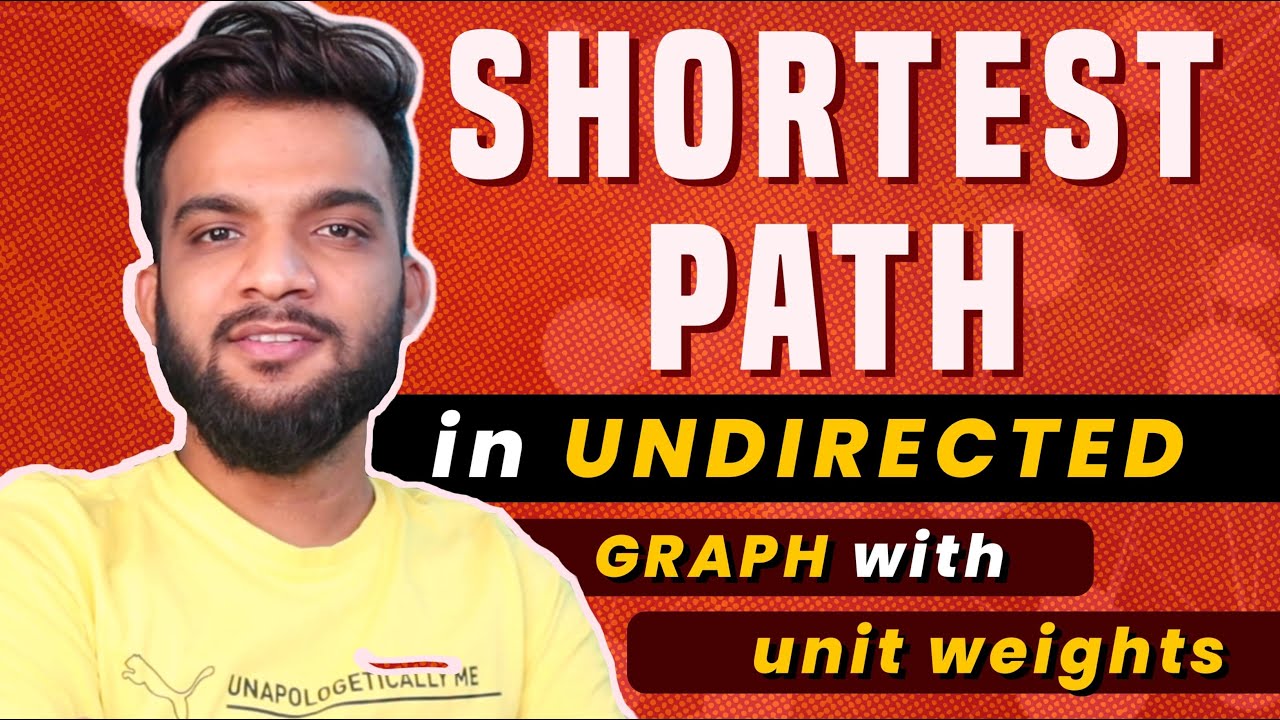
G-28. Shortest Path in Undirected Graph with Unit Weights
5.0 / 5 (0 votes)