Intro To Numpy - Numpy For Machine Learning 1
Summary
TLDRIn this video, John Elder from CodingMe.com introduces the basics of NumPy, a fundamental library for machine learning and data science. He explains the limitations of Python lists for handling large datasets and how NumPy arrays solve these issues by offering a more efficient, homogeneous data structure. John demonstrates how to create and manipulate NumPy arrays, covering basic functions such as `shape`, `arange`, `zeros`, and `full`. He also outlines the importance of learning these foundational skills for progressing into more advanced topics like pandas and machine learning algorithms.
Takeaways
- 😀 Numpy is fundamental to machine learning and data science, helping manage large data sets efficiently.
- 💡 Python lists are versatile but become inefficient with large data sets due to varying data types and memory usage.
- 🚀 Numpy arrays are like Python lists but optimized for performance, especially with large, numerical datasets.
- 📦 Numpy arrays require all elements to be of the same data type, making them more efficient for machine learning tasks.
- 🔢 Numpy stands for 'Numeric Python,' and its arrays are called 'ndarrays,' representing n-dimensional arrays.
- 💻 To use Numpy, you must install it via pip (`pip install numpy`) and import it as `import numpy as np`.
- 🔧 You can create arrays with functions like `np.array`, `np.arange`, `np.zeros`, and `np.full` for different types of data initialization.
- 🧮 Numpy arrays support multi-dimensional data structures, useful for more complex data analysis in machine learning.
- ⚡ Numpy arrays offer various mathematical operations and functions for data manipulation, like `shape` to get array size.
- 📘 Converting Python lists to Numpy arrays is straightforward using `np.array()`.
Q & A
What is NumPy and why is it important for machine learning?
-NumPy is a powerful library in Python used for working with arrays, which is fundamental for handling large datasets in machine learning. It's designed for numerical operations and is optimized for efficiency, making it essential when dealing with large amounts of data.
Why are Python lists not ideal for machine learning tasks?
-Python lists can store different data types, which can lead to inefficiency when working with large datasets. Lists are computationally expensive in terms of memory and processing speed, especially when handling millions or billions of data points, which is common in machine learning.
How does a NumPy array differ from a Python list?
-Unlike Python lists, NumPy arrays require all elements to be of the same data type, which allows for more efficient processing. NumPy arrays are optimized for numerical operations and can handle multi-dimensional data.
How can you create a NumPy array from a Python list?
-You can convert a Python list to a NumPy array by using the `numpy.array()` function and passing the list as an argument. For example: `np.array([1, 2, 3])` converts a Python list to a NumPy array.
What is the function of the `np.shape` method in NumPy?
-The `np.shape` method in NumPy is used to return the dimensions of the array. It provides information on how many elements exist in each dimension of the array.
How can you generate an array of zeros in NumPy?
-To generate an array filled with zeros in NumPy, you can use the `np.zeros()` function, passing the number of elements or dimensions as an argument. For example, `np.zeros(10)` creates a one-dimensional array with 10 zeros.
What does the `np.arange()` function do?
-The `np.arange()` function creates a NumPy array with a sequence of numbers within a specified range. You can also define the step size for the range. For example, `np.arange(0, 10, 2)` creates an array with values `[0, 2, 4, 6, 8]`.
What is an N-dimensional array in NumPy?
-An N-dimensional array (ndarray) in NumPy refers to an array that can have any number of dimensions, not limited to one or two. This is particularly useful for machine learning and data science, where multi-dimensional data is common.
How can you create multi-dimensional arrays in NumPy?
-You can create multi-dimensional arrays in NumPy by passing a tuple representing the dimensions to functions like `np.zeros()` or `np.full()`. For example, `np.zeros((2, 10))` creates a 2D array with two rows and 10 columns filled with zeros.
What are some basic functions provided by NumPy for array manipulation?
-NumPy provides many functions for array manipulation, such as `np.zeros()` to create arrays of zeros, `np.full()` to create arrays filled with a specific value, `np.shape` to get the dimensions, and `np.arange()` to create sequences of numbers.
Outlines
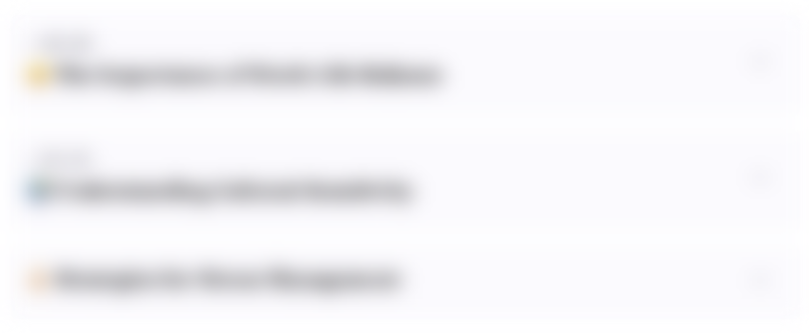
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
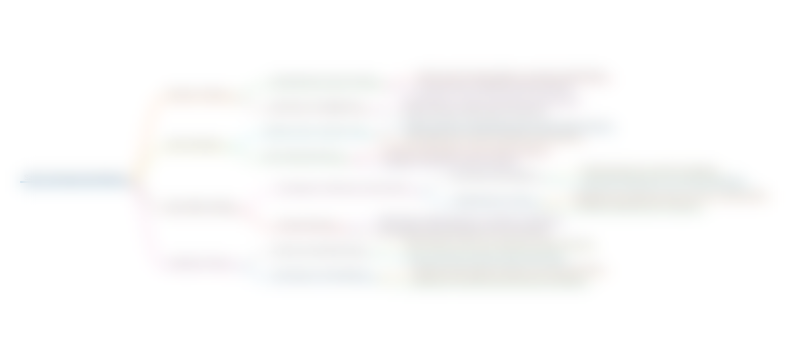
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
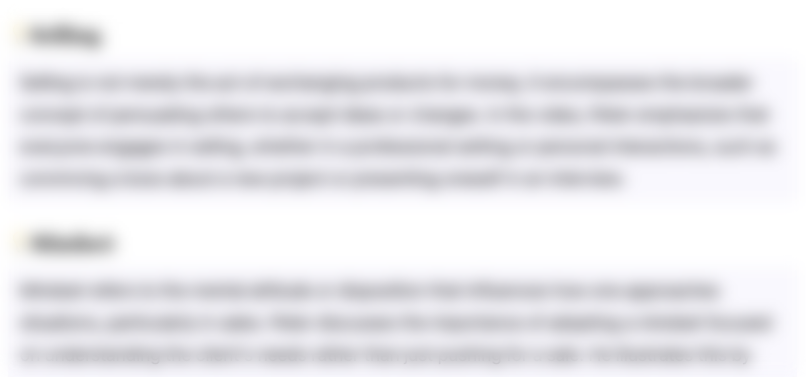
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
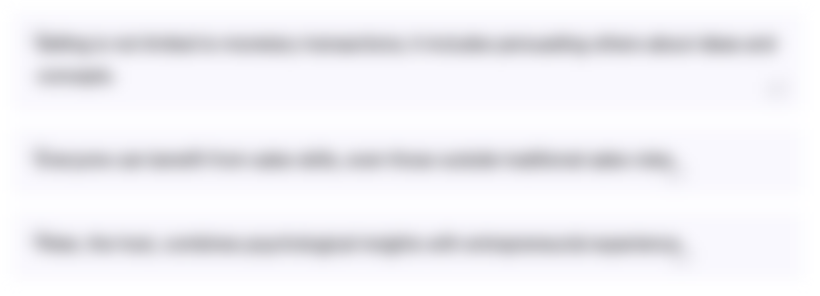
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
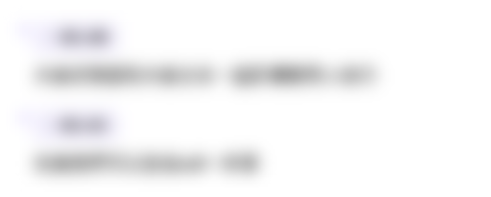
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
5.0 / 5 (0 votes)