Role-Based Authentication in React (Complete Tutorial)
Summary
TLDRIn this instructional video, Darius Cosden teaches role-based authentication in React, a crucial concept for protecting certain components or data based on a user's role. He introduces 'Project React' for comprehensive React learning and 'Import React' newsletter. The tutorial demonstrates creating an authentication setup using a mock backend and React's context API. It then implements role checks using a 'ProtectedRoute' component, ensuring users with the correct role can access specific content. The video emphasizes best practices like the single responsibility principle and encourages hands-on practice for deeper understanding.
Takeaways
- 📘 The video provides an in-depth tutorial on implementing role-based authentication in React applications, emphasizing its importance for securing specific parts of an application.
- 🎓 The instructor mentions 'Project React', a comprehensive course for learning React in a structured manner, and 'Import React', a newsletter for staying updated on React topics.
- 🔐 The script outlines creating an 'auth.ts' file to simulate a backend for authentication, including user data with roles that determine access to certain resources.
- 👤 The 'auth context' in React is introduced as a way to handle user authentication state and provide it throughout the application via the 'AuthProvider' component.
- 🔄 The use of React's custom hooks, specifically 'useAuth', is highlighted for accessing authentication state and functions within components.
- 🛡️ The tutorial demonstrates how to create a 'ProtectedRoute' component to guard access to certain routes based on user roles, showcasing the use of TypeScript for type safety.
- 🔑 The 'ProtectedRoute' component checks for user authentication and role permissions, rendering content, showing a loading state, or displaying a 'Permission Denied' message accordingly.
- 🔄 The script describes using 'useEffect' to automatically fetch user authentication status when the component mounts, improving user experience by not requiring manual login checks.
- 🔧 The importance of handling different states of user authentication (undefined, authenticated, unauthenticated) is stressed for building a robust authentication system.
- 🛠️ The video encourages viewers to clone and experiment with the provided code to deepen their understanding and improve their React development skills.
- 👋 The instructor, Darius Cosden, concludes by inviting feedback and engagement, promoting further learning and interaction within the React community.
Q & A
What is the main topic of the video?
-The main topic of the video is role-based authentication in React applications.
Why is role-based authentication important in applications?
-Role-based authentication is important because it ensures that certain parts of an application are only accessible to users with the correct role, adding an extra layer of security and access control.
What is the purpose of the auth.ts file mentioned in the video?
-The auth.ts file serves as a mock backend to implement custom authentication without needing a real backend, providing functions for user login and retrieval.
What does the 'get user' and 'login' function in the auth.ts file do?
-The 'get user' and 'login' functions simulate waiting for a response from a backend, generate an authentication token, and return a mock response with the token and test user object.
How is React Router DOM used in the context of role-based authentication?
-React Router DOM is used to implement protected routes that require a signed-in user with the correct role to access.
What is the role of the Auth Provider component in the React application?
-The Auth Provider component is responsible for handling authentication, calling the backend with the correct user credentials, and providing the authenticated user to the rest of the application.
Why is it necessary to create a custom hook for the Auth Provider?
-A custom hook is created to prevent the use of the Auth Provider's context outside of the actual provider, ensuring that the authentication status is correctly managed within the component tree.
What are the three different states represented by the 'current user' state in the Auth Provider?
-The three states are: 'no', indicating the user is not authenticated; a user object, indicating the user is authenticated and known; and 'undefined', indicating the authentication status is pending.
How does the video script handle errors in the authentication process?
-Errors in the authentication process are handled by setting the auth token and current user to null, which communicates to the rest of the application that the authentication attempt failed.
What is the purpose of the 'useEffect' hook in the Auth Provider component?
-The 'useEffect' hook is used to automatically fetch the user's authentication status when the component mounts, ensuring that the application has the most up-to-date information on the user's authentication state.
How can the viewer extend the role-based authentication implementation provided in the video?
-The viewer can extend the implementation by cloning the provided code repository, experimenting with the code, understanding the reasoning behind each implementation choice, and attempting to make modifications or fixes to improve or customize the functionality.
Outlines
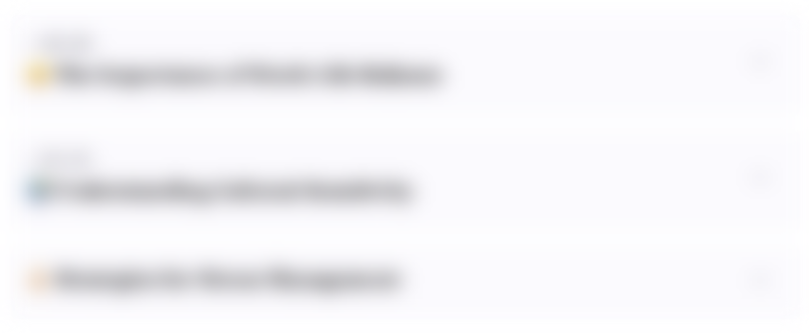
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
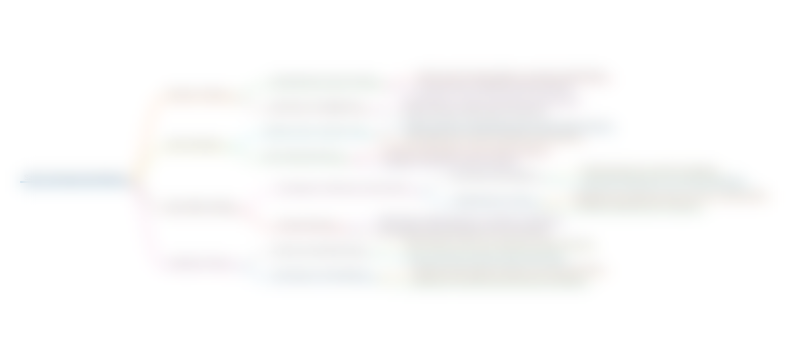
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
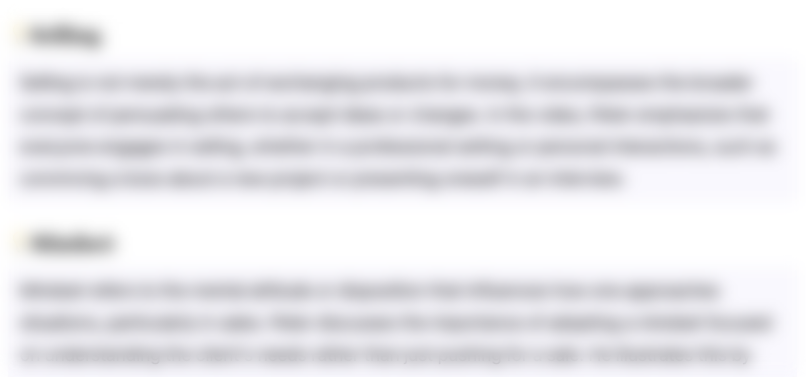
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
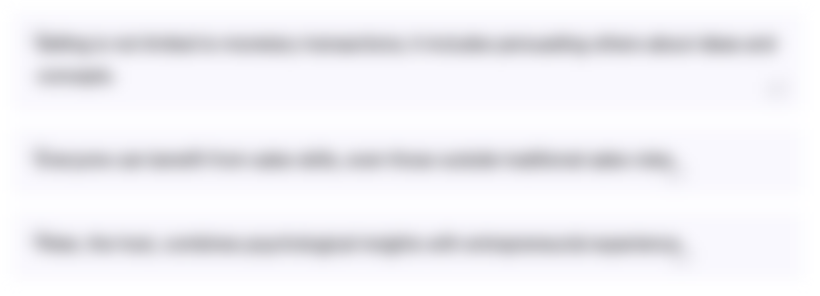
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
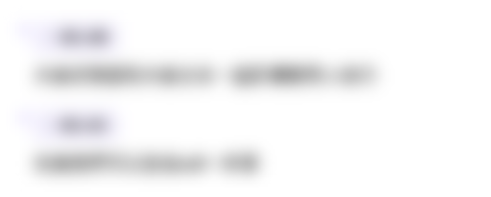
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

Authentication, Authorization, and Accounting - CompTIA Security+ SY0-701 - 1.2
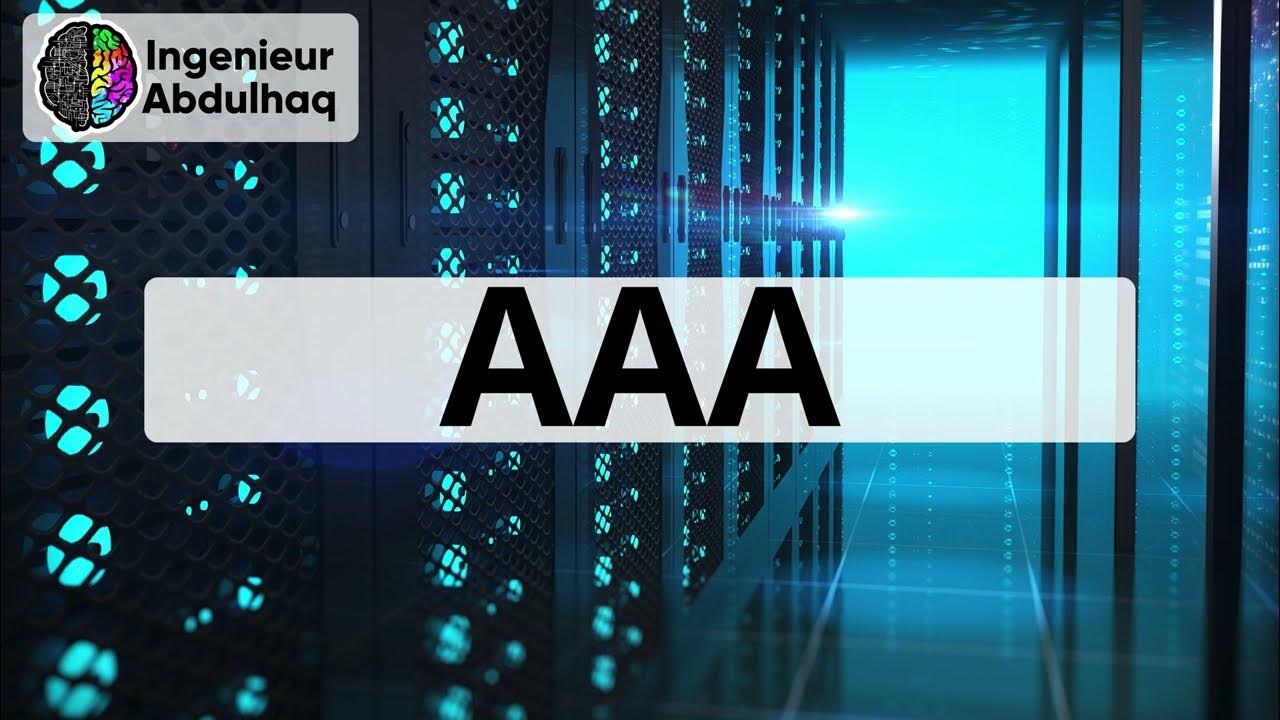
4 - AAA (Authentication - Authorization - Accounting) Security+ SY0-701 - عربي
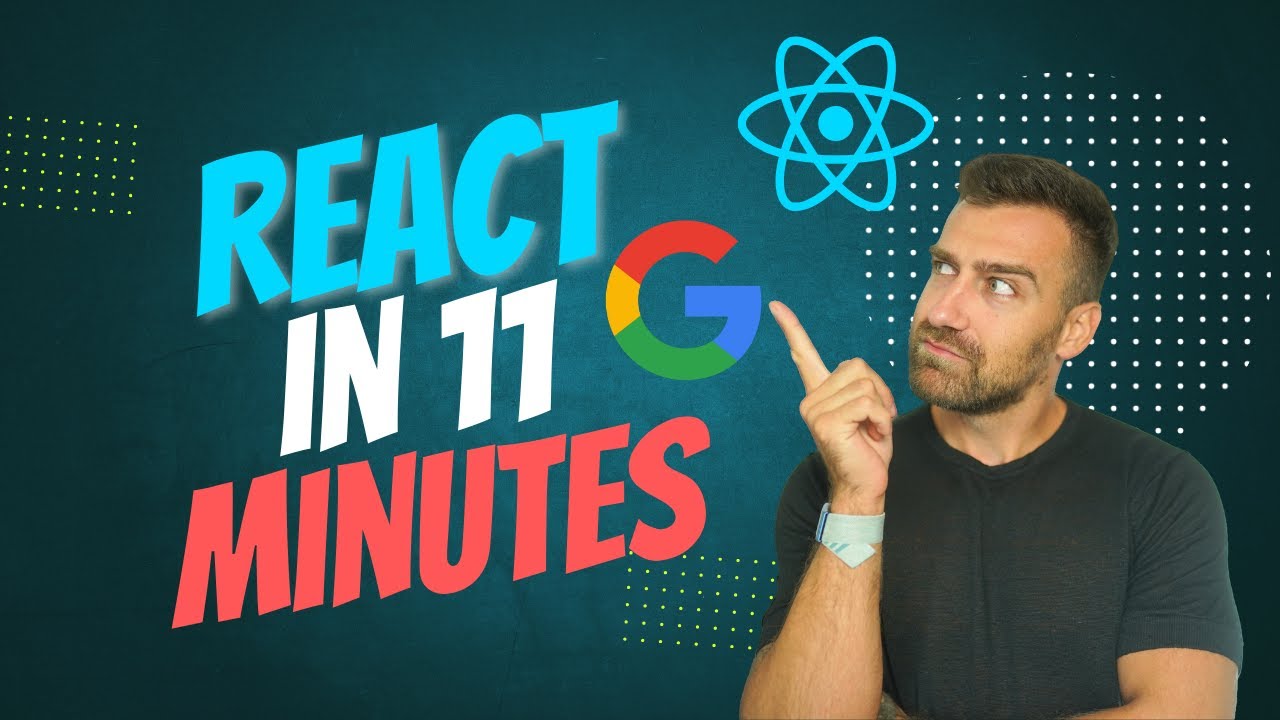
How to Learn React Fast in 2024: A Beginner's Guide

Apa itu firewall? Cara kerja firewall dan Jenis- jenis firewall
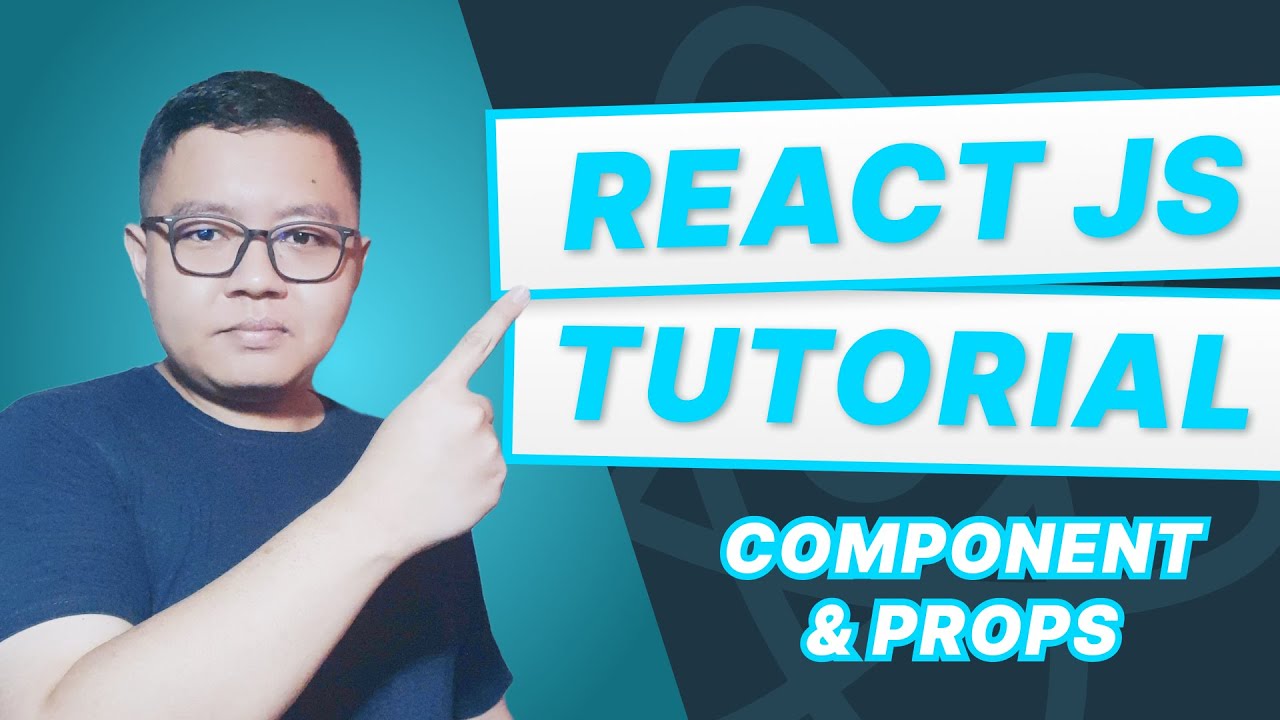
Tutorial React JS Bahasa Indonesia : 3.Component & Props

What is Firebase Authentication?
5.0 / 5 (0 votes)