#16 Property Binding | Angular Components & Directives | A Complete Angular Course
Summary
TLDRThis lecture delves into the concept of property binding in web development, contrasting it with string interpolation for one-way data binding. It illustrates how property binding is used to assign dynamic values to HTML attributes, as opposed to static data display with string interpolation. Examples provided include binding an image source, enabling/disabling a button based on stock status, and dynamically setting input values. The tutorial also clarifies the difference between HTML attributes and properties, and introduces attribute binding for accessibility and data attributes.
Takeaways
- 📝 String interpolation and property binding are both used for one-way data binding in Angular, passing data from the component class to the view template.
- 🖼 String interpolation is used for displaying data in HTML, such as product titles or names.
- 🔗 Property binding is used to bind a DOM object's property to a value in the component class, allowing dynamic manipulation of the DOM.
- 📸 An example of property binding is setting the 'src' attribute of an image tag to a product's image path stored in the component class.
- 🔄 Property binding can dynamically assign values to HTML attributes, such as enabling or disabling a button based on product stock.
- 🚫 String interpolation cannot be used for certain HTML attributes like 'disabled', 'hidden', and 'checked', where property binding is necessary.
- 🔑 The difference between HTML attributes and properties is that attributes represent initial values and do not change, while properties represent current values and can be dynamic.
- 🛑 For HTML attributes that do not have corresponding properties, such as 'aria-hidden', attribute binding must be used instead of property binding.
- 🔄 An alternative syntax for property binding is using 'bind' followed by the attribute name, like 'bind-value' for input elements.
- 🔧 Property binding is essential for creating interactive and dynamic web applications, allowing for real-time updates to the UI based on data changes.
- 🤔 Understanding the distinction between string interpolation and property binding is crucial for developers to make the right choice in different scenarios in Angular applications.
Q & A
What is the purpose of string interpolation and property binding in Angular?
-String interpolation and property binding in Angular are used for one-way data binding, allowing data to be passed from the component class to the view template. String interpolation is typically used for displaying data in HTML, while property binding is used to bind a property of a DOM object to a value in the component class, enabling dynamic manipulation of the DOM.
How does string interpolation differ from property binding in Angular?
-String interpolation is used for displaying static or dynamic text within an HTML template, whereas property binding is used to bind a dynamic value to an HTML attribute, allowing for more complex interactions with the DOM elements.
What is an example of when to use string interpolation in Angular?
-String interpolation can be used to display the title or name of a product within an HTML template, as it is suitable for showing a piece of data without the need for any further DOM manipulation.
Can property binding be used to manipulate the DOM elements in Angular?
-Yes, property binding allows for the manipulation of DOM elements by binding their properties to dynamic values from the component class, enabling actions such as showing or hiding elements, enabling or disabling buttons, and more.
How can you bind an image source to a product object in Angular?
-You can create a property within the product object, such as 'pImage', and assign the image path to this property. Then, in the view template, use property binding syntax to bind the 'src' attribute of the 'img' tag to the 'pImage' property of the product object.
What is the syntax for property binding in Angular?
-The syntax for property binding in Angular involves wrapping the HTML attribute you want to bind within square brackets and assigning a TypeScript expression within double quotes.
Why can't string interpolation be used for all HTML attributes?
-String interpolation cannot be used for certain HTML attributes like 'disabled', 'hidden', and 'checked' because they require a boolean value to enable or disable their functionality, which is not suitable for the text-based output of string interpolation.
What is the alternative syntax to using square brackets for property binding in Angular?
-The alternative syntax for property binding in Angular is to use the 'bind' prefix followed by the attribute name, such as 'bindValue' for the 'value' attribute of an input element.
How can you make a button disabled based on the stock status of a product in Angular?
-You can use property binding to bind the 'disabled' attribute of the button to a TypeScript expression that checks the 'inStock' property of the product. If 'inStock' is less than or equal to 0, the button will be disabled; otherwise, it will be enabled.
What is the difference between HTML attributes and HTML properties?
-HTML attributes represent the initial values set in the markup and are static, while HTML properties represent the current values that can change dynamically as the page loads and scripts execute.
Can you provide an example of using attribute binding in Angular?
-Attribute binding can be used for accessibility attributes like 'aria-hidden'. Instead of using square brackets, you would use 'attr.' followed by the attribute name, and assign a TypeScript expression to it within double quotes.
Outlines
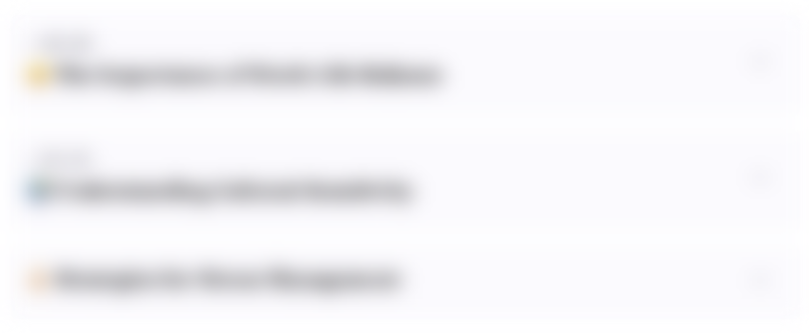
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
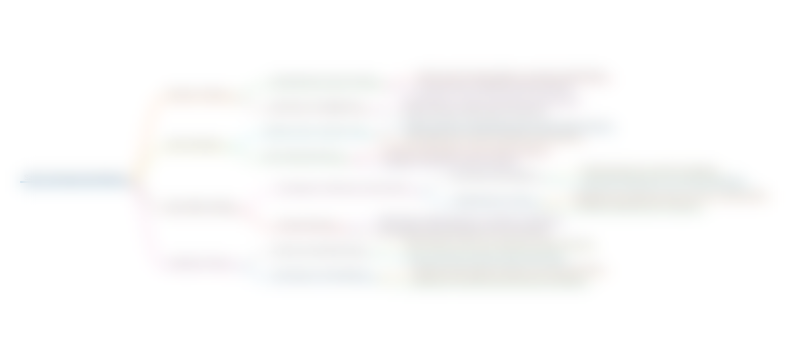
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
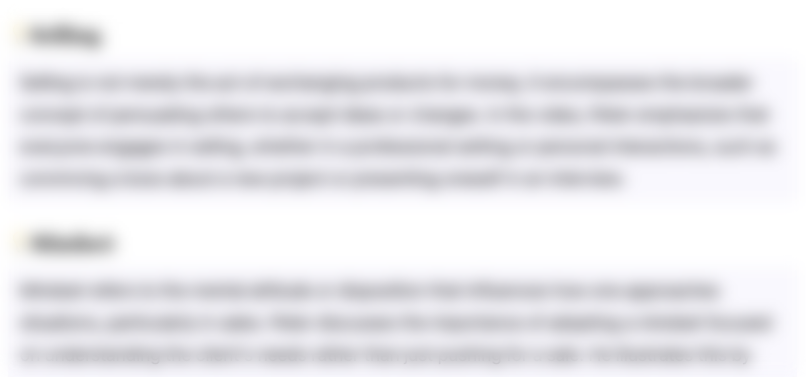
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
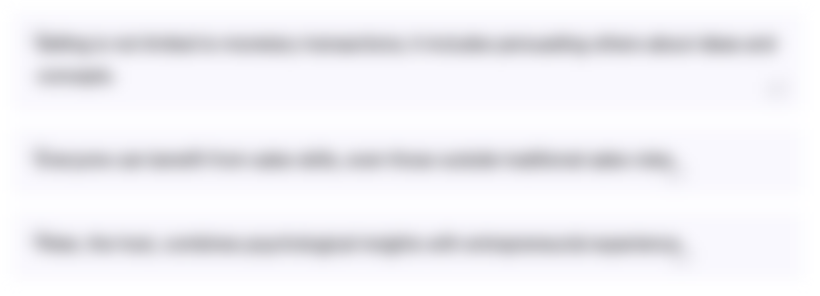
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
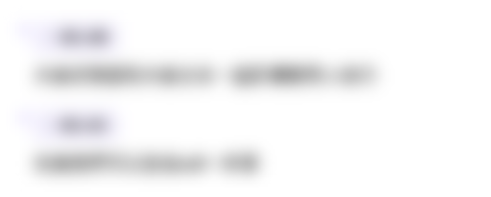
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
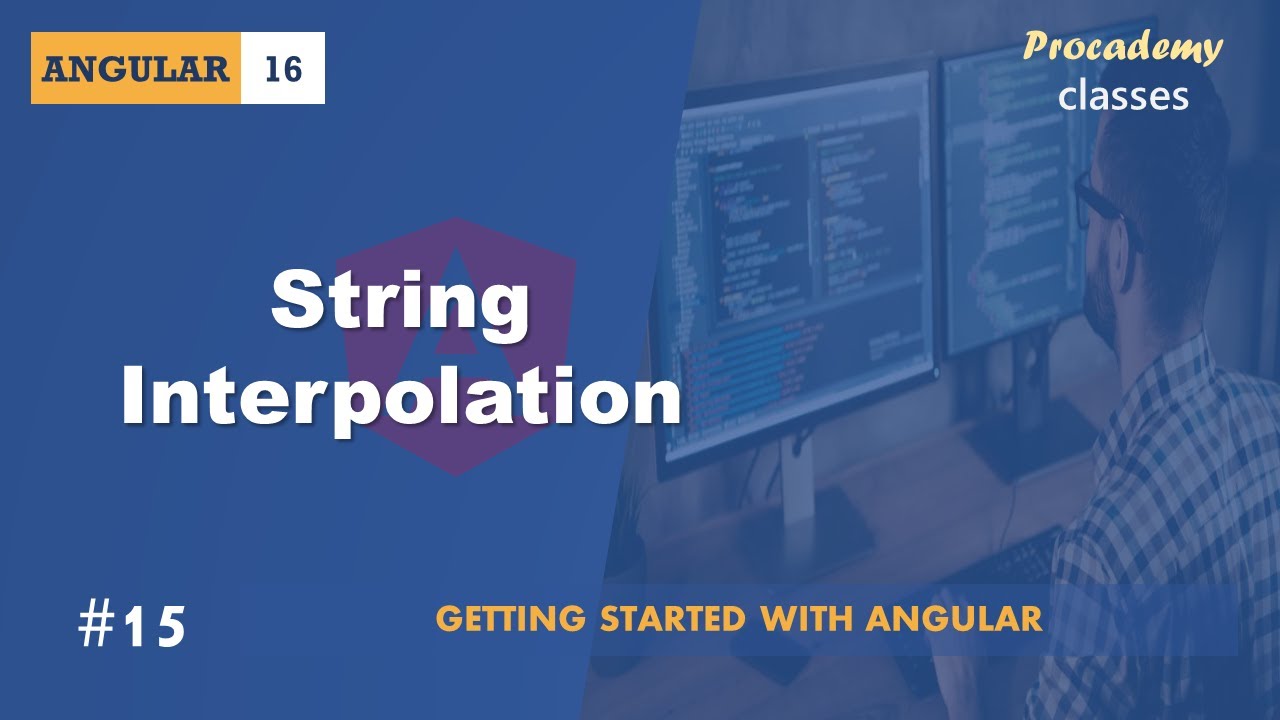
#15 String Interpolation | Angular Components & Directives | A Complete Angular Course

#17 Event Binding | Angular Components & Directives | A Complete Angular Course
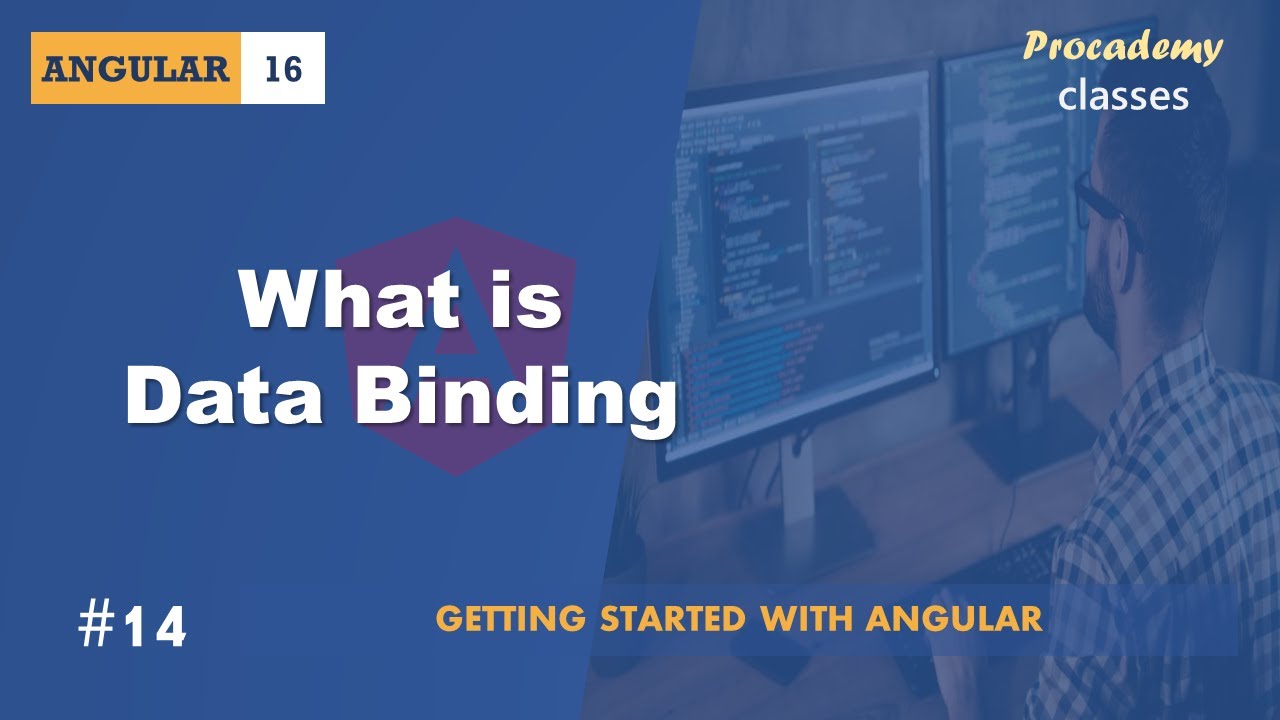
#14 What is Data Binding | Angular Components & Directives | A Complete Angular Course

#18 Two way Data Binding | Angular Components & Directives | A Complete Angular Course
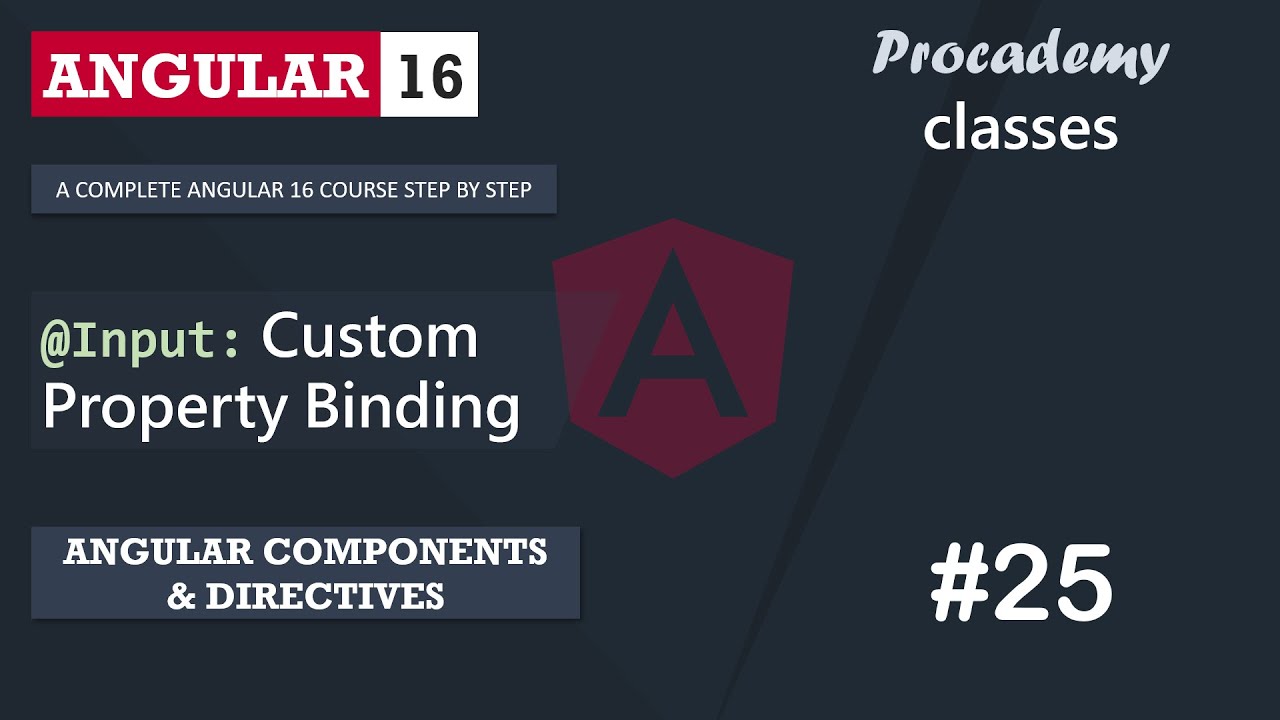
#25 @Input: Custom Property Binding | Angular Components & Directives | A Complete Angular Course
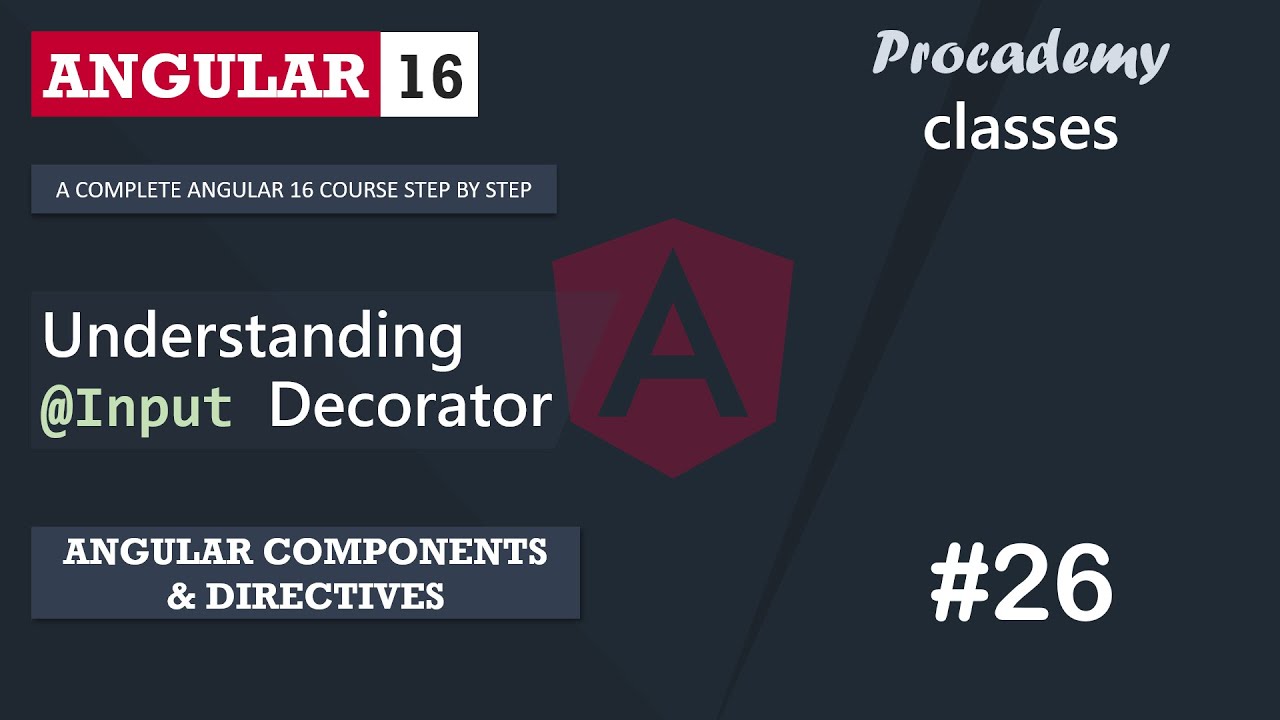
#26 Understanding Input Decorator | Angular Components & Directives | A Complete Angular Course
5.0 / 5 (0 votes)