Delegatecall | Solidity 0.8
Summary
TLDRThe video explains delegate call in Solidity, where a contract can execute code in another contract while retaining the context of the calling contract. It demonstrates an example where Contract A calls Contract B which delegate calls Contract C. Despite Contract C's code executing, message.sender remains Contract A and message.value remains what A sent since the context is preserved from B. The video then shows how delegate call allows upgrading a contract's logic by deploying a new version of the called contract. However, it warns that the called contract must keep identical state variable declarations to avoid issues.
Takeaways
- 😀 With delegatecall, the called contract executes with the context of the calling contract. Message sender and msg.value are preserved.
- 👍🏻 When contract B delegatecalls contract C, msg.sender in C is the original caller to B. This differs from a regular call.
- 🔑 State variables are stored in the calling contract, not the one being delegatecalled. So contract C uses B's storage.
- ✨ You can upgrade a contract's logic via delegatecall since it uses the calling contract's state.
- 🚧 If you change state variable order when upgrading, it will break compatibility and cause issues.
- 👀 delegatecall allows "logic separation" - state stored separately from logic.
- ⚠️ Security risk: delegatecalled code can withdraw funds from the calling contract.
- 📝 delegatecall returns a bool for success and bytes for any return data.
- 💡 Can specify the function sig instead of a string when encoding for delegatecall.
- 😊 Overall delegatecall allows you to efficiently reuse and upgrade code & logic in contracts.
Q & A
What is delegate call in Solidity?
-Delegate call is a function in Solidity that allows a contract to execute code from another contract, while retaining the context of the calling contract. The called contract's code executes in the environment of the calling contract.
How does message.sender work with delegate call?
-With delegate call, message.sender inside the called contract will be the original caller to the calling contract. This preserves the original message sender context.
How does message.value work with delegate call?
-Message.value inside the called contract via delegate call will be the original amount sent to the calling contract that initiated the delegate call. The context including the amount sent is preserved.
Which contract's state variables are updated with delegate call?
-The calling contract's state variables are updated when using delegate call. The called contract code executes using the calling contract's storage and state.
Can you upgrade contract logic using delegate call?
-Yes, delegate call allows upgrading the logic executed by a contract even though the contract code itself is immutable. The calling contract delegates execution to updated logic contracts.
What are the risks of changing state variable order with delegate call upgrades?
-If new state variables are added or order is changed in an upgrade, it can break the delegate call mapping to storage. Existing state must be preserved in original order.
Does ether sent from the called contract go to the calling contract?
-Yes, any ether sent from the called contract is deducted from the calling contract's balance. The context of the calling contract is fully preserved.
Can new state variables be safely added to upgrade contracts?
-New state variables can be safely added in upgrade contracts as long as they are appended after existing variables, preserving original order.
What data is returned from a delegate call function?
-Like a regular call, delegate call returns a boolean indicating success and bytes data returned from the function call.
When would you use delegate call over a regular function call?
-Delegate call is useful to leverage an existing contract's storage and context for execution. It saves deploying new contracts in some upgrade cases.
Outlines
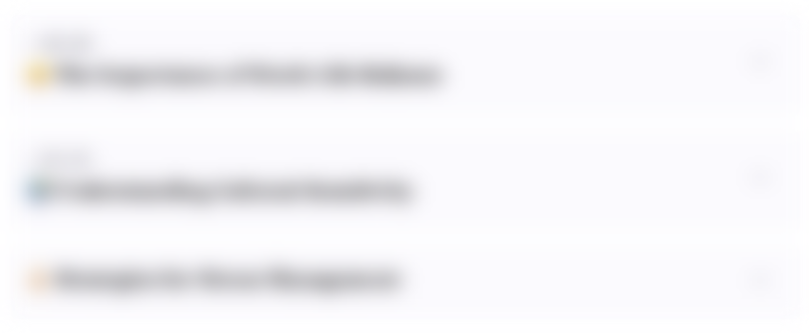
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
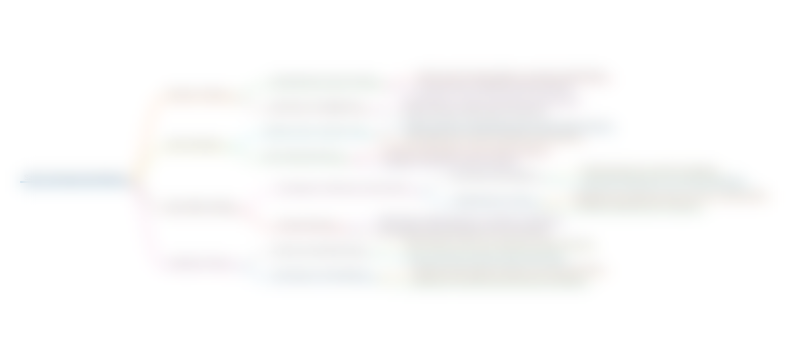
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
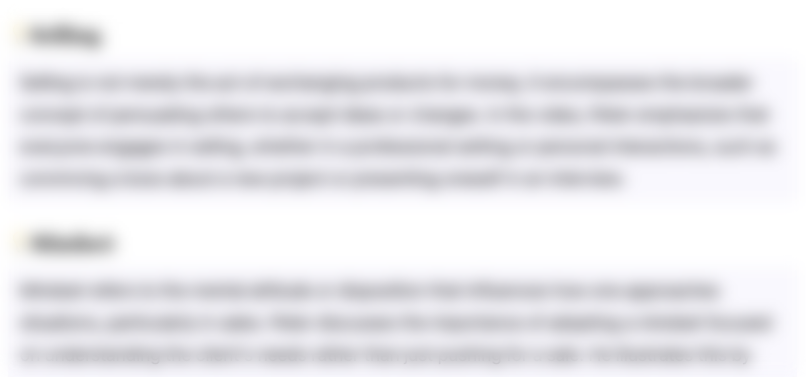
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
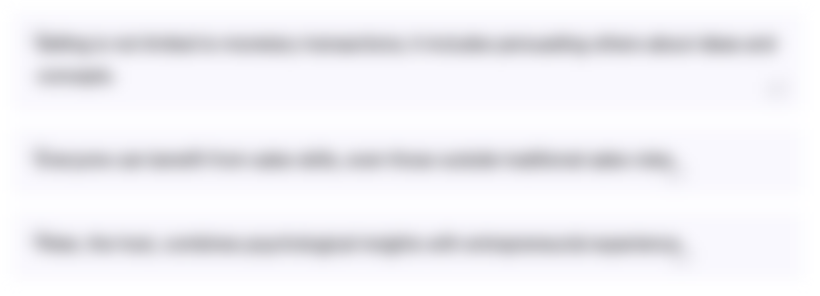
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
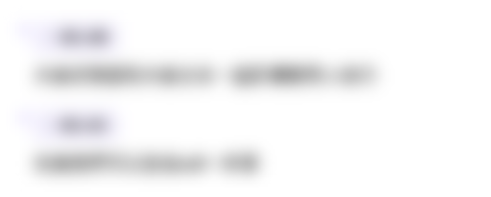
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
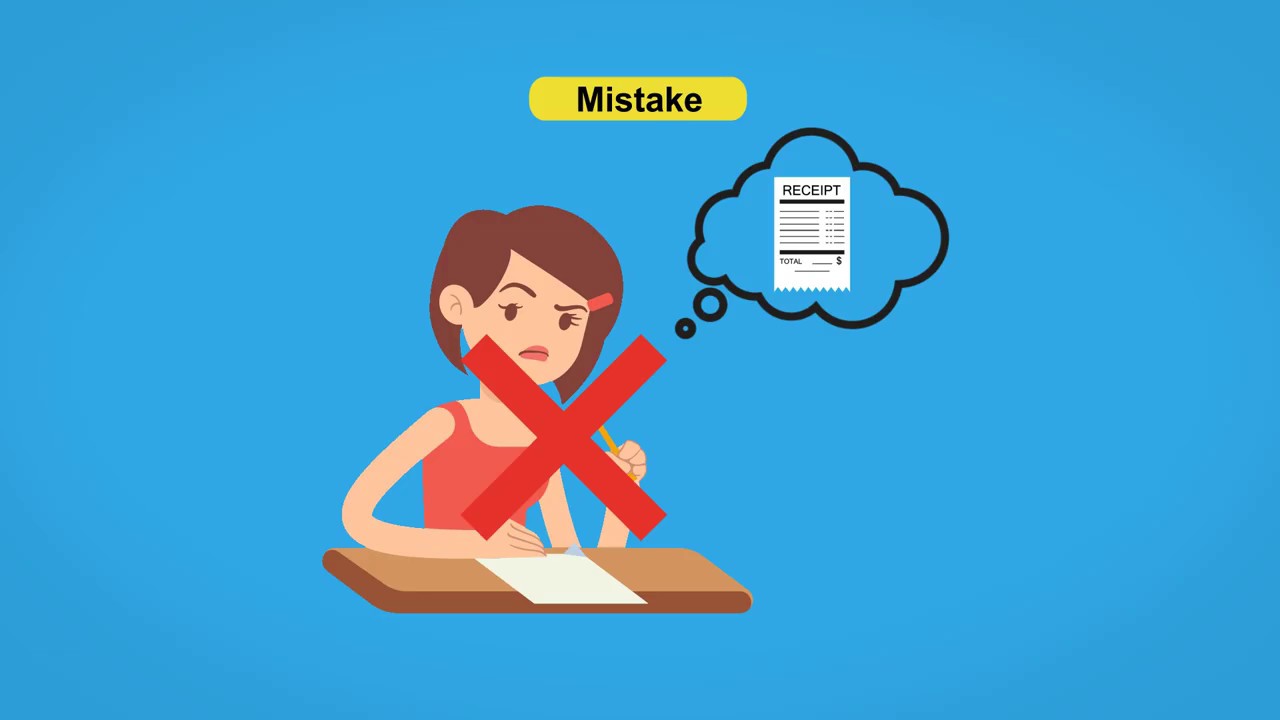
Contract Defenses for Lack of Mutual Assent: Mistake, Misunderstanding and Misrepresentation
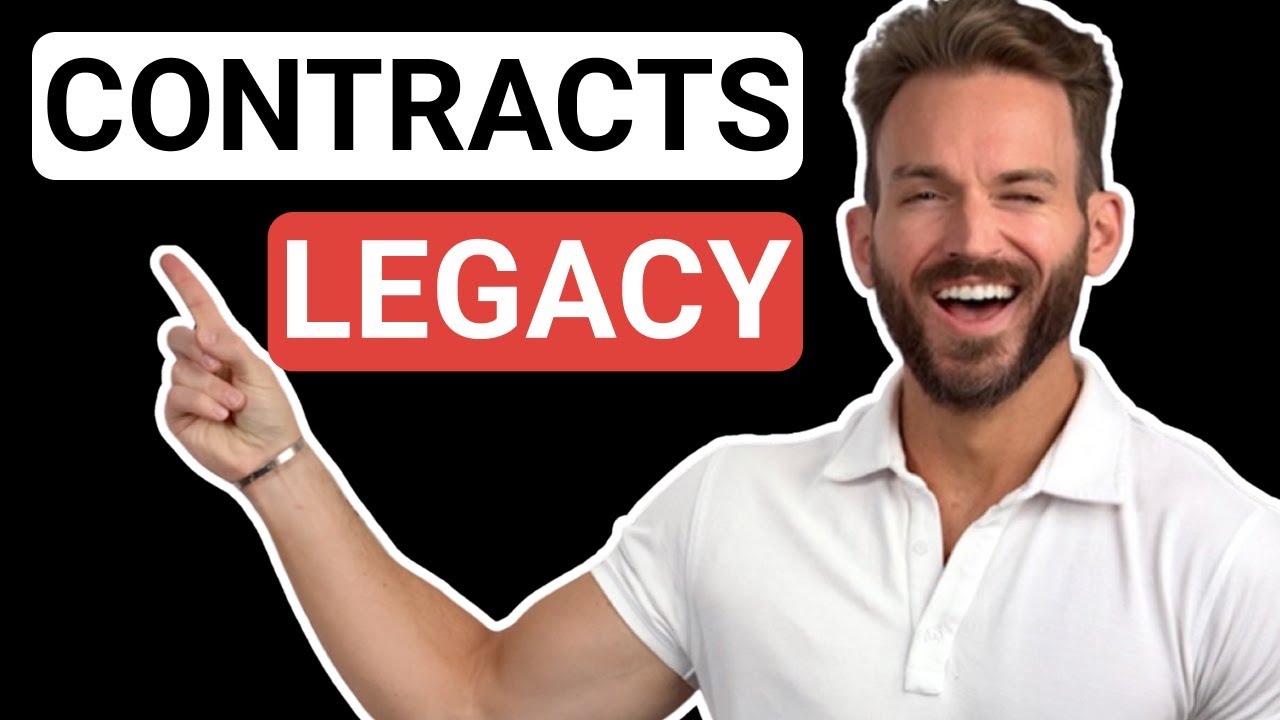
Contract Law Overview: What is the Gateway Issue on ALL Contracts Essays?

Mau Pengadaan Jasa Konstruksi? Ini Dia Jenis Kontrak yang Sesuai Untuk Proyek Tersebut!
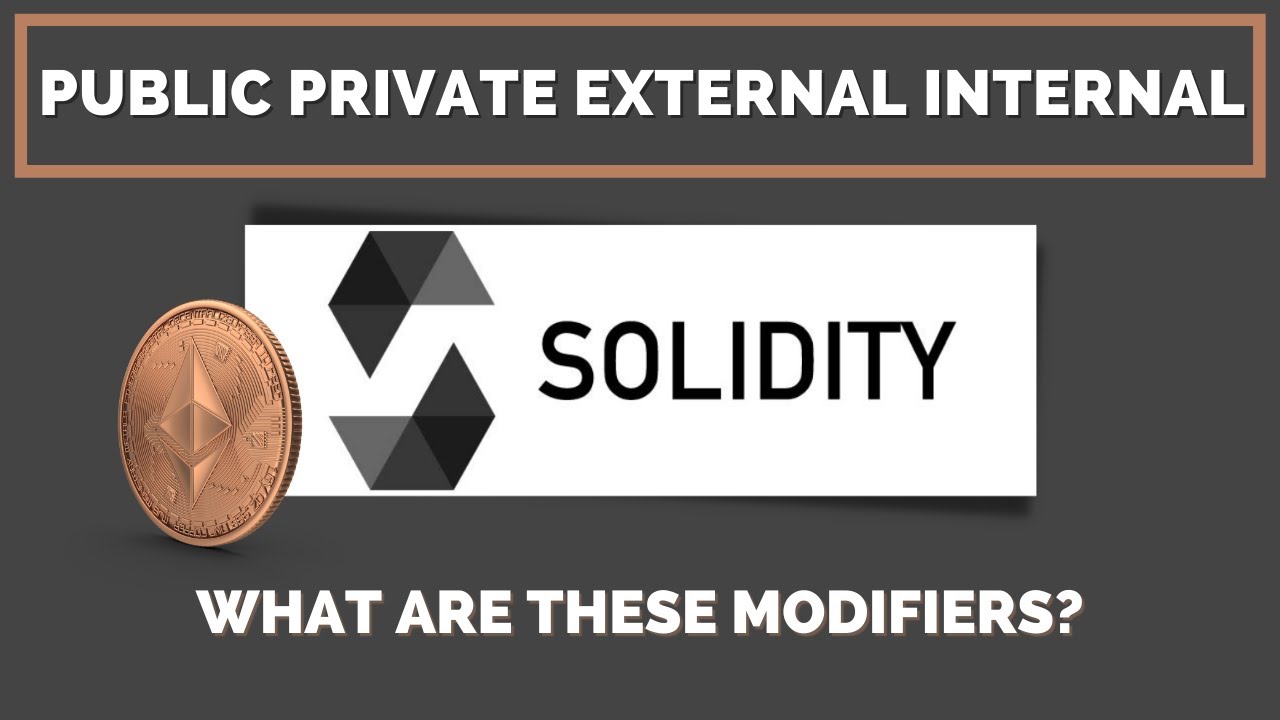
Solidity Tutorial | Visibility Modifiers - Public, External, Internal Private
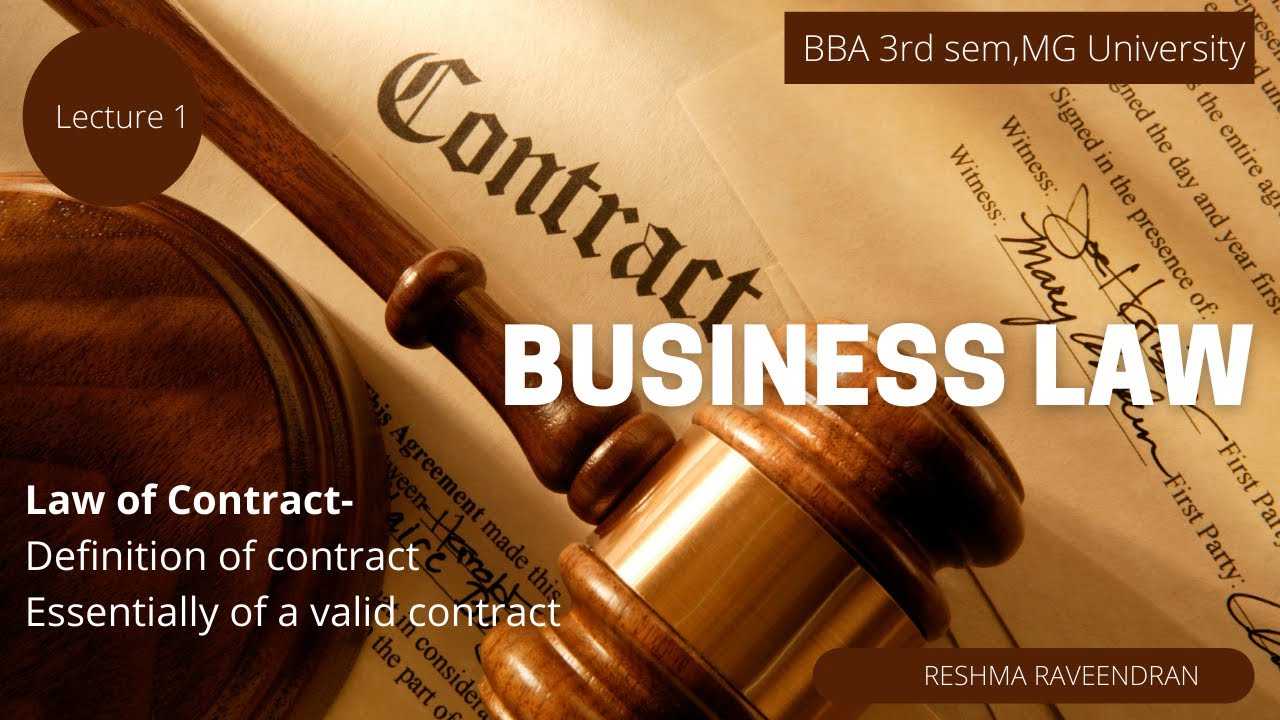
Business law, lecture 1 ,BBA 3rd sem,MG University
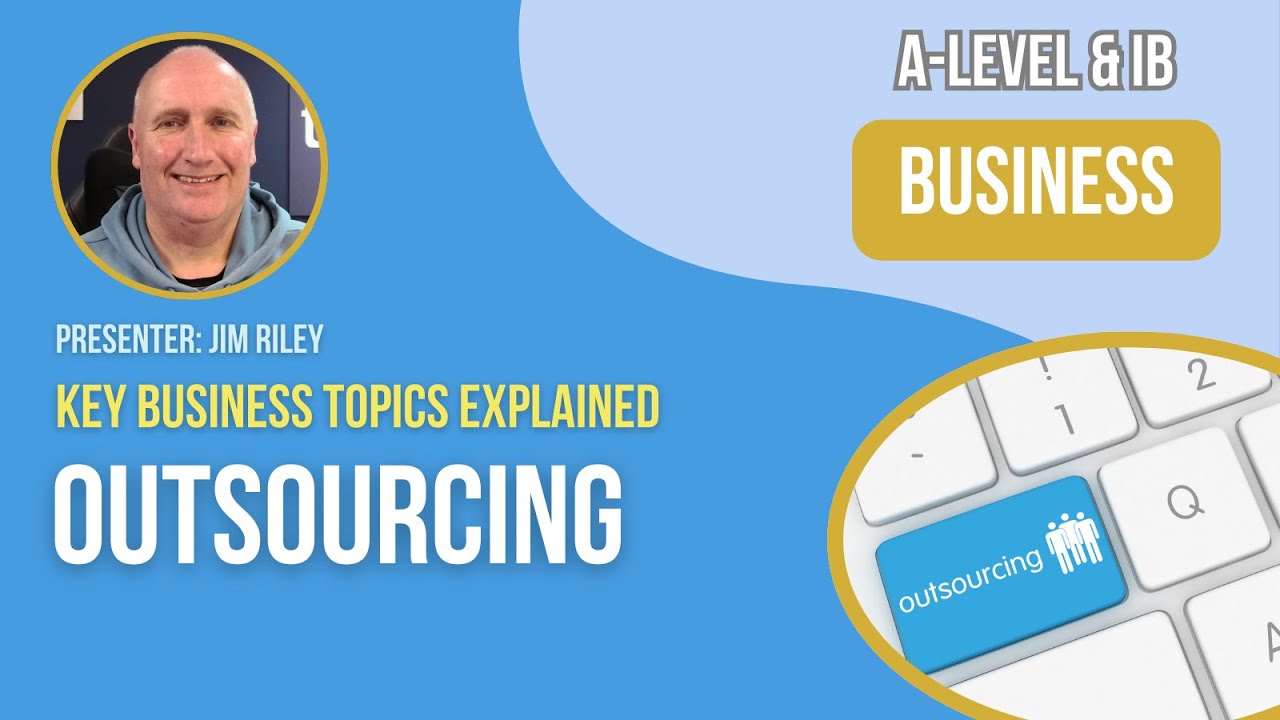
Outsourcing | A-Level & IB Business
5.0 / 5 (0 votes)