Build a Matrix With Conditions - Leetcode 2392 - Python
Summary
TLDRIn this coding tutorial, the presenter tackles the complex problem of building a matrix with specific row and column conditions. They start by explaining the problem and its constraints, then dive into the intuition behind the solution, drawing parallels to previous problems like the course schedule and alien dictionary. The core algorithm discussed is topological sorting, a concept integral to solving this problem, which is compared to depth-first search (DFS). The presenter outlines the steps for topological sort, including cycle detection, before demonstrating the solution in Python code. The video concludes with a walkthrough of the code, ensuring viewers understand the logic and implementation.
Takeaways
- 😀 The video aims to solve a problem involving building a matrix with specific conditions.
- 🔍 The problem description is lengthy and complex, involving a K by K square matrix filled with numbers 1 through K, with the remaining elements being zero.
- 🔢 Two input arrays provide conditions for the placement of numbers in rows and columns, indicating which numbers must be above or to the left of others.
- 🔄 The solution involves a topological sort, similar to the algorithm used in the 'course schedule' problem, which is a prerequisite for understanding the solution.
- 📈 The core idea is to transform the two-dimensional problem into a one-dimensional one by first focusing on the rows and then applying the same technique to the columns.
- 🔎 A graph is constructed based on the row and column conditions, which helps in visualizing the problem and applying the topological sort algorithm.
- 🌐 The topological sort algorithm is used to determine a valid order for the elements in the matrix, ensuring that all prerequisites are met for each element.
- 🔁 The process involves running a depth-first search (DFS) to detect cycles in the graph, which would make the matrix invalid.
- 📚 The solution includes converting lists into hashmaps (dictionaries in Python) to efficiently look up the row and column positions for each number.
- 💻 The final implementation involves coding the topological sort and DFS in Python, with careful attention to cycle detection and the correct ordering of elements.
Q & A
What is the main problem being discussed in the script?
-The main problem discussed in the script is building a matrix with specific conditions based on two input arrays that dictate the placement of numbers within a K by K matrix.
What is the significance of the numbers 1 through K in the matrix?
-The numbers 1 through K are the only values that will be populated in the matrix, with the remaining elements being zero. Each number must be placed according to the conditions given in the input arrays.
What does the term 'above' in the row conditions signify?
-In the row conditions, 'above' means that one number must be placed in a row that is strictly above another number, and they cannot share the same row.
What is the core algorithm that needs to be understood to solve this problem?
-The core algorithm needed to solve this problem is Depth-First Search (DFS), which is used in conjunction with topological sorting to order the elements in the matrix according to the given conditions.
Why is it important to know the topological sort algorithm for this problem?
-Topological sort is important because it helps in ordering the elements in a way that respects the directed edges (or prerequisites) of a graph, which in this case is analogous to the conditions for the rows and columns of the matrix.
What is the role of the adjacency list in the script?
-The adjacency list is used to represent the graph of conditions for both rows and columns, making it easier to perform DFS and topological sort to determine the order of elements.
How does the script simplify the two-dimensional problem into a one-dimensional one?
-The script suggests focusing on the rows first, treating the problem as a one-dimensional ordering issue, and then applying the same technique to the columns after determining the row order.
What is the condition that would make the matrix invalid according to the script?
-The matrix would be invalid if there is a contradiction in the conditions, such as a cycle in the graph, where a number is above another and that second number is above the first, creating a loop with no valid ordering.
How does the script handle the possibility of a disconnected graph?
-The script handles disconnected graphs by running topological sort from every node and marking them visited as it goes, ensuring that all nodes are considered in the final matrix.
What is the time complexity of the solution provided in the script?
-The time complexity of the solution is determined by the traversal of the graph, which in the worst case could be O(K^2), where K is the dimension of the matrix.
How does the script ensure that the final matrix satisfies the row and column conditions?
-The script ensures this by using the results of the topological sort to create mappings for the row and column positions of each number, and then populating the matrix according to these mappings.
Outlines
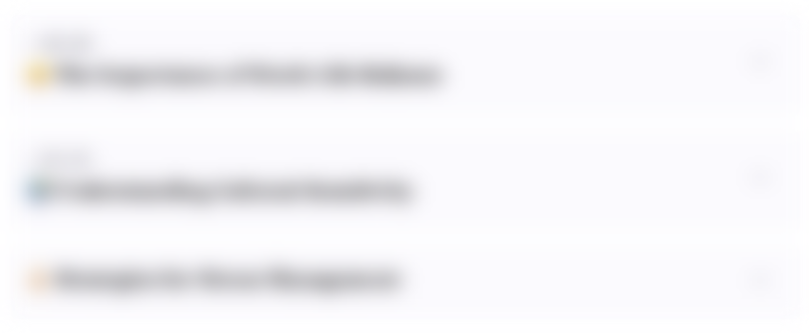
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
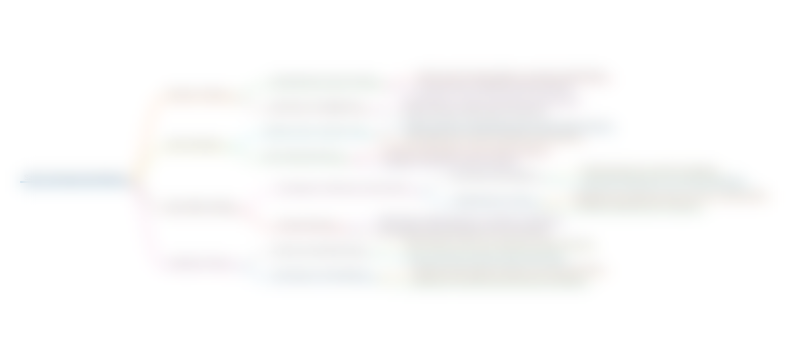
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
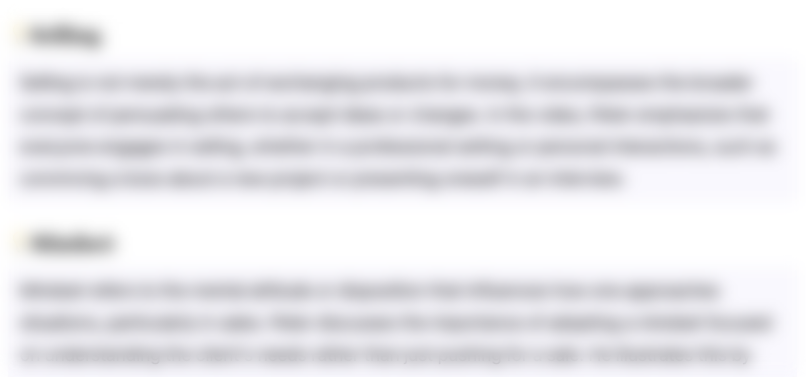
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
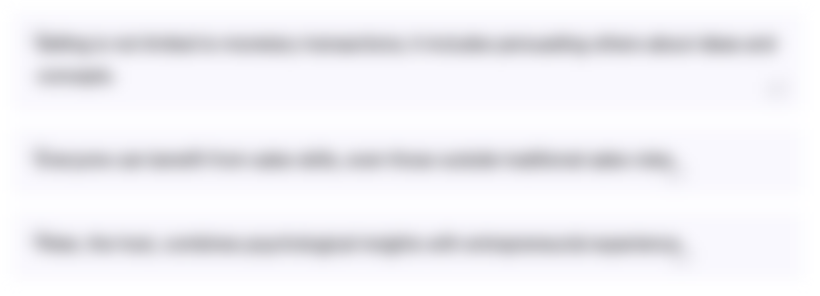
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
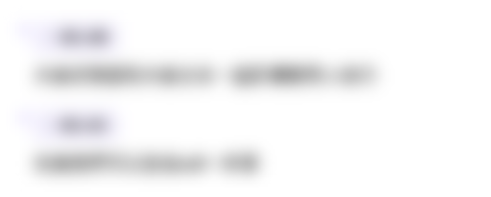
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示

Ruang Baris, Ruang Kolom dan Rank dari Sebuah Matriks (Bagian Pertama)

Matrices and Matrix Operations

Capítulo 6 - Solucionando o tabuleiro

MATRIKS RUANG VEKTOR | MENCARI DETERMINAN DENGAN METODE OBE
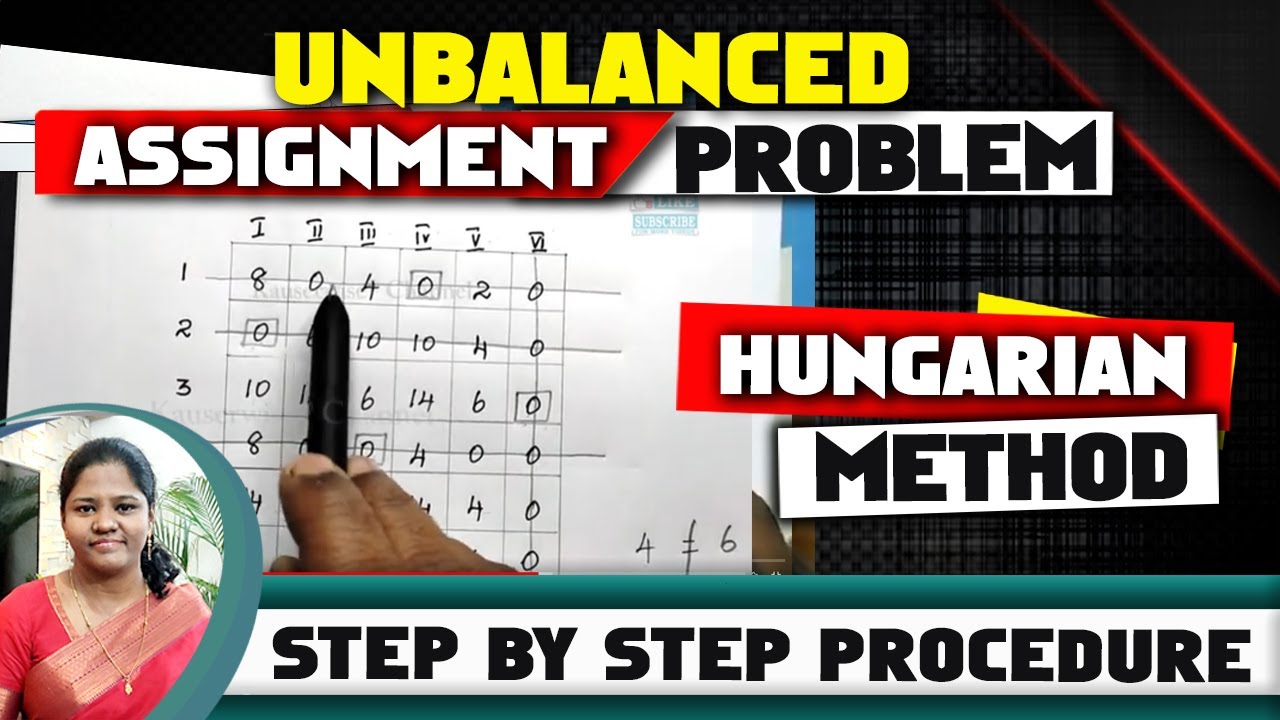
Unbalanced Assignment Problem | Non-Square Matrix Assignment | Hungarian Method | :-By Kauserwise
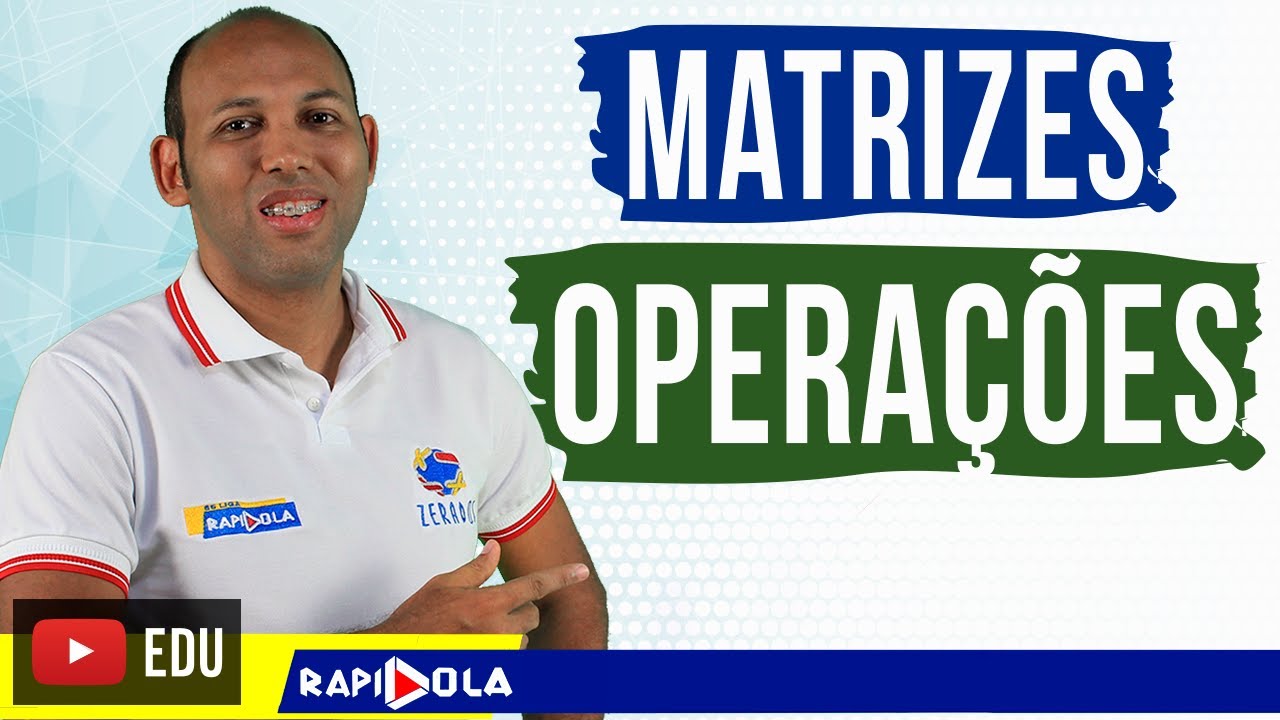
MATRIZES - OPERAÇÕES COM MATRIZES EP 2
5.0 / 5 (0 votes)