Importing Development Data | Lecture 92 | Node.JS 🔥
Summary
TLDRIn this instructional video, the presenter takes a break from API development to demonstrate a script that imports tour data from a JSON file into a MongoDB database. The script operates independently from the express application and includes steps to connect to the database, read the JSON file, and utilize the tour model for data import. Additionally, the video covers command-line interaction, allowing users to run the script with options to import or delete data. The presenter addresses potential validation errors and concludes with a successful data import, setting the stage for future API enhancements.
Takeaways
- 🛠️ The script's purpose is to import tour data from a JSON file into a MongoDB database, separate from the main Express application.
- 📝 The script is created in a 'data' folder and named 'importDevData.js', emphasizing its standalone nature.
- 📚 Mongoose is required for database interactions, and environment variables are needed for database connection details.
- 🔌 The script requires the file system module 'fs' to read the JSON file and the 'tour' model to interact with the database.
- 📖 The JSON file is read synchronously with a specified encoding of 'utf eight'.
- 🔍 An async function is used to import data into the database, utilizing 'tour.create' to handle an array of objects.
- 🗑️ There's a function to delete all data from the MongoDB collection using 'deleteMany', a method provided by Mongoose.
- 📋 The script can be run with command-line options to either import or delete data, demonstrated by checking 'process.argv'.
- 🔄 The script includes error handling with try-catch blocks to log issues during data import or deletion.
- 🚫 Validation errors are encountered due to incomplete data in the JSON file, highlighting the importance of data integrity.
- 🔚 The script exits the process after completing its task using 'process.exit', ensuring the script doesn't continue running unnecessarily.
Q & A
What is the purpose of the script mentioned in the video?
-The script's purpose is to import tour data from a JSON file into a MongoDB database, which is a standalone task separate from the main Express application.
Why was the decision made to include the script-building exercise in the course?
-The script-building exercise was included because it was considered a nice little exercise that adds value to the course, despite the possibility of just providing the script in the starter files.
What does the script need to perform its task?
-The script requires mongoose for database interaction, the .env package for environment variables, the file system module to read the JSON file, and the tour model to write the data into the database.
How does the script handle reading the JSON file?
-The script uses the synchronous version of `fs.readFile` to read the JSON file named 'toursSimple.json' and specifies the encoding as 'utf eight'.
What is the significance of the 'importData' function in the script?
-The 'importData' function is an asynchronous function that imports data into the database by using the 'tour.create' method with an array of JavaScript objects obtained from parsing the JSON file.
How can the script handle the deletion of existing data in the database?
-The script includes a function to delete all data from the database using the 'delete many' function of the tour model, which removes all documents in the tours collection.
What is the role of 'process.argv' in the script?
-'process.argv' is used to interact with the command line, allowing the script to accept arguments that determine whether to import or delete data from the database.
Why is the 'process.exit' function used in the script?
-The 'process.exit' function is used to stop the script execution after completing the import or delete operation, as it is a small standalone script and not part of a larger application.
What issue occurred when the script was first run to import data?
-The script encountered validation errors because the JSON file contained some tours with incomplete data, specifically missing 'group size' and other required fields.
How can the script be improved to avoid importing incomplete data?
-The script can be improved by adding checks to ensure that all required fields are present in the JSON data before attempting to import it into the database.
What is the final step in the script after successfully importing the data?
-The final step is to log a success message to the console indicating that the data has been successfully loaded into the database and then exit the process using 'process.exit'.
Outlines
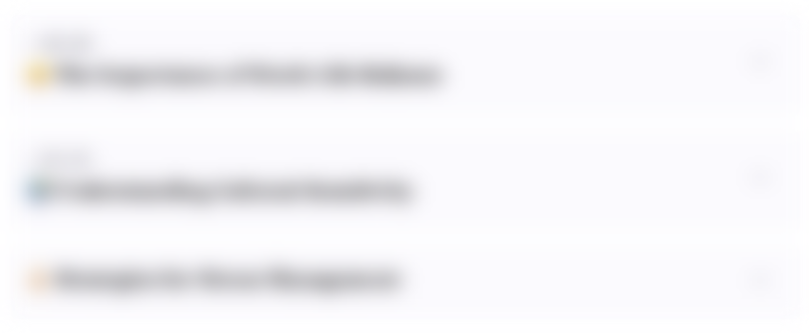
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
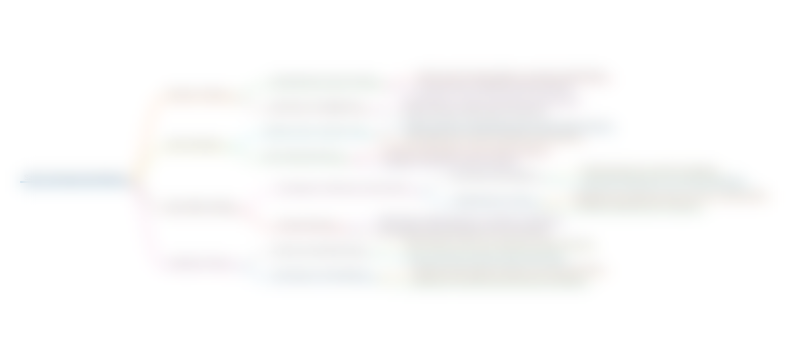
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
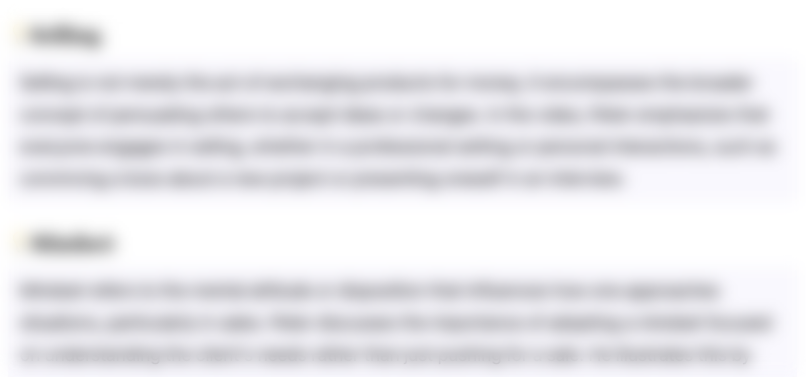
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
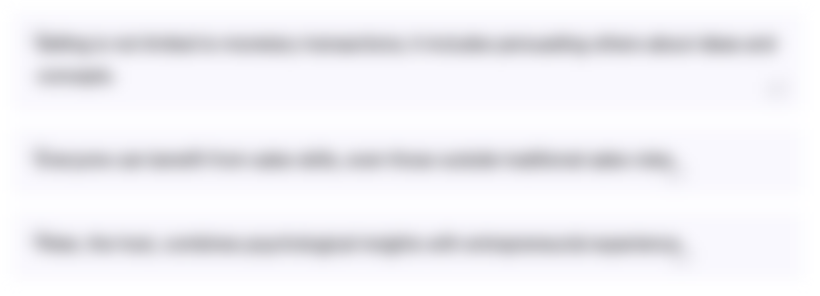
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
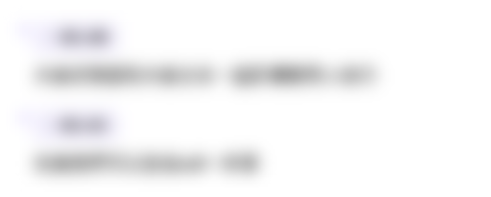
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
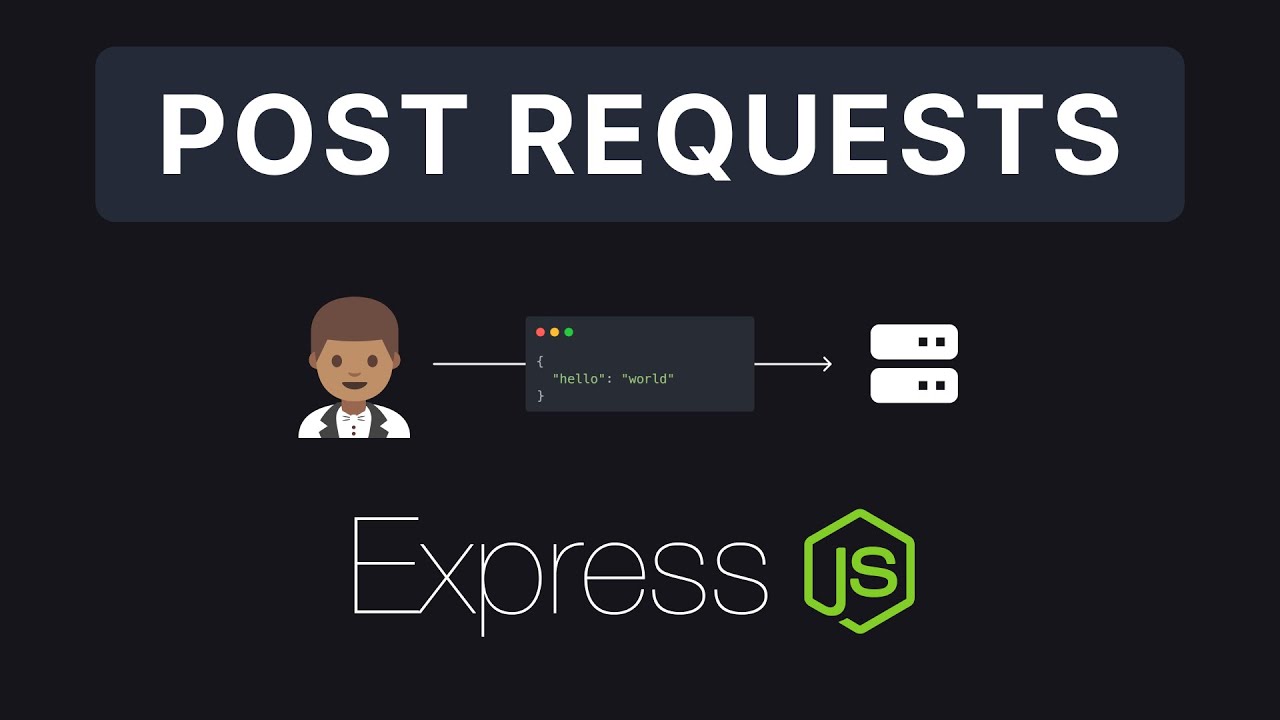
Express JS #5 - Post Requests
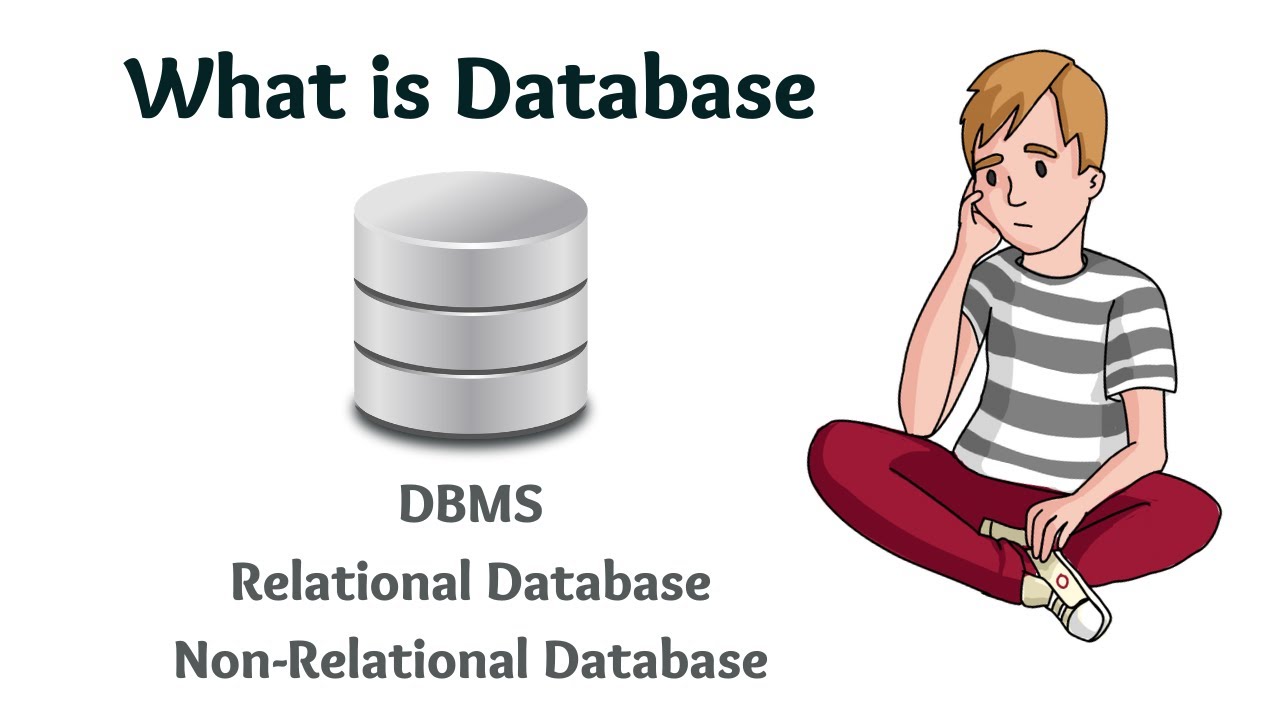
Learn What is Database | Types of Database | DBMS
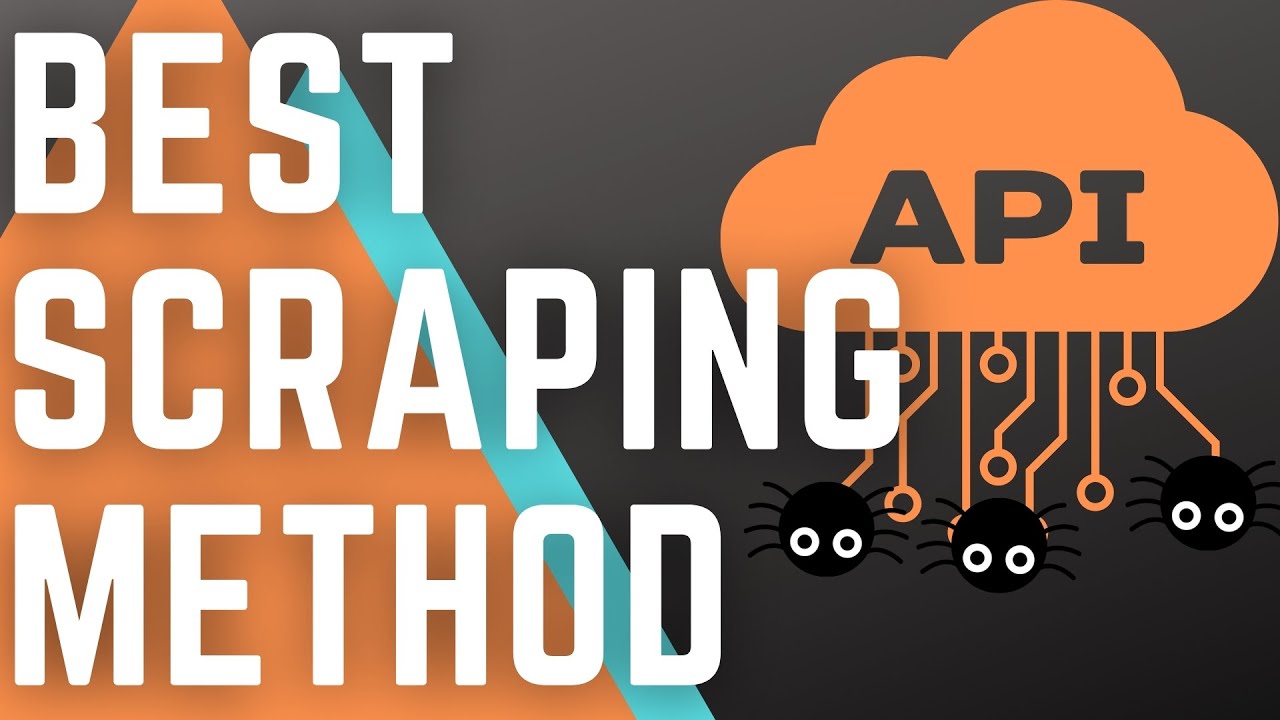
Always Check for the Hidden API when Web Scraping
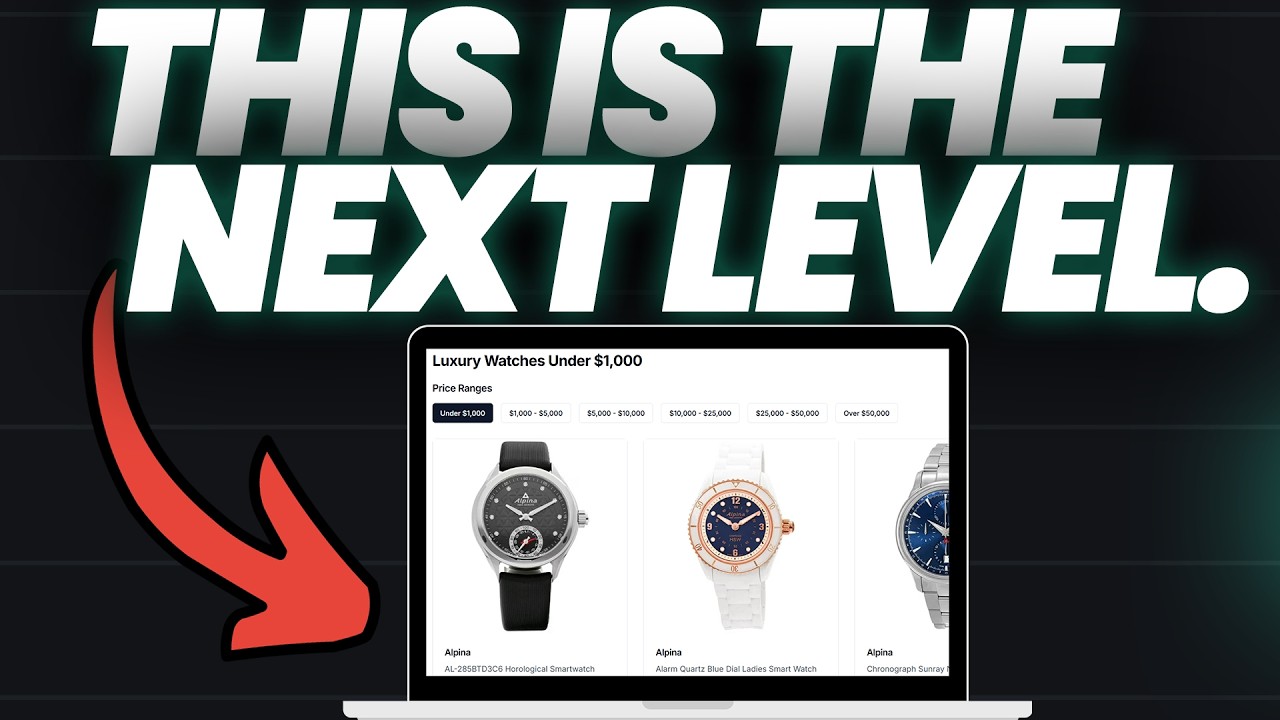
🛑 Stop Making WordPress Affiliate Websites (DO THIS INSTEAD)
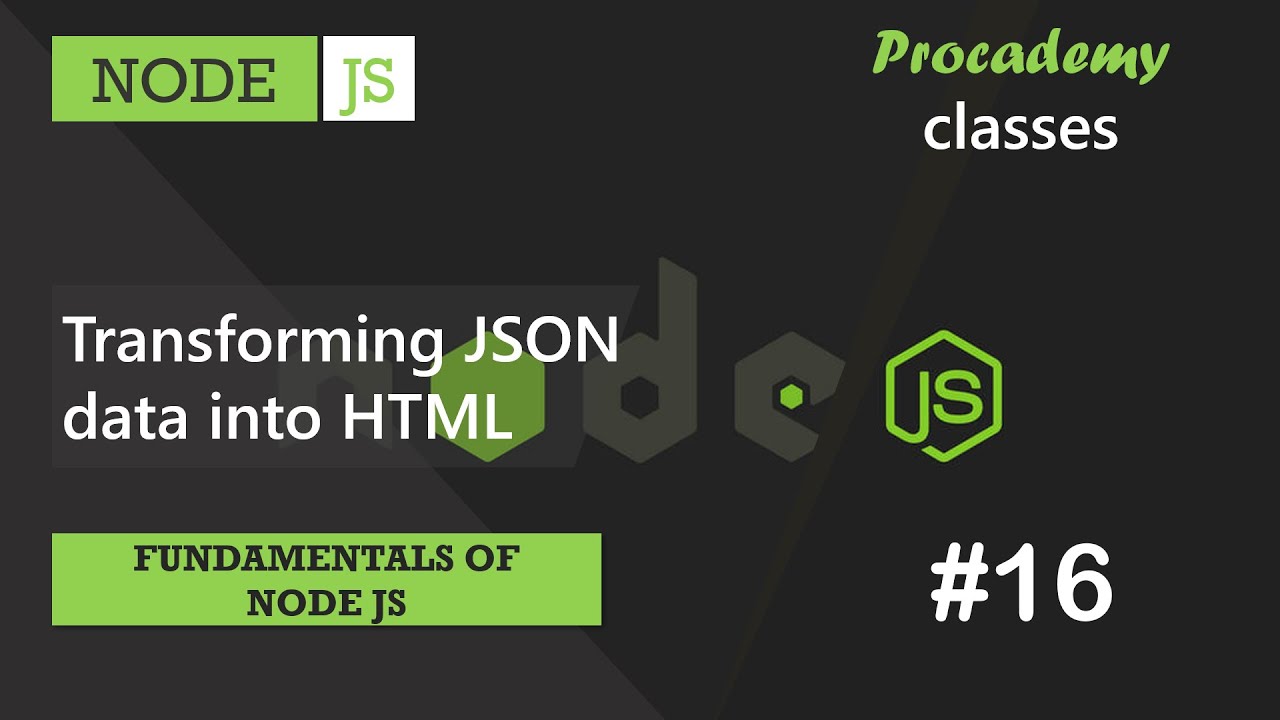
#16 Transforming JSON data into HTML | Fundamentals of NODE JS | A Complete NODE JS Course

MongoDB Insert Document in Collection in Urdu / Hindi Tutorial || Dr Rabia kanwal
5.0 / 5 (0 votes)