C++ Tutorial for Beginners 10 - While Loops
Summary
TLDRIn this tutorial, the speaker introduces the concept of the while loop in C++ programming, explaining its syntax and usage. The video demonstrates how a while loop runs as long as a condition is true, using examples to print values, increment variables, and sum user inputs. The tutorial also explores the creation of an infinite loop using 'while(1)' and shows how to take user inputs to calculate a sum over multiple iterations. Ideal for beginners, this video provides a clear, hands-on approach to mastering while loops in C++.
Takeaways
- 😀 The `while` loop in C++ executes a block of code repeatedly as long as the given condition is true.
- 😀 The syntax of the `while` loop is: `while (condition) { // code }`.
- 😀 A `while` loop continues to execute until the condition inside the parentheses becomes false.
- 😀 In a basic `while` loop, the loop’s condition is evaluated before each iteration.
- 😀 You can increment or modify a variable inside the loop to eventually make the condition false and break the loop.
- 😀 In the example provided, `x` is initialized to 1 and incremented with `x++` until it reaches 6, at which point the loop exits.
- 😀 The `while` loop can be used for various purposes, including calculating sums or repeatedly executing tasks based on user input.
- 😀 In the user input example, the program asks for five numbers, adds them up, and prints the total sum.
- 😀 The `while` loop can also be used to create an infinite loop by using `while (true)`, which keeps executing until manually interrupted.
- 😀 An infinite loop might be useful in scenarios like continuously asking for user input, but it should be controlled carefully to avoid unintended program freezes.
- 😀 The `while` loop is an essential construct in C++ for scenarios where you need to repeatedly check a condition and execute code accordingly.
Q & A
What is a `while` loop in C++?
-A `while` loop in C++ repeatedly executes a block of code as long as a given condition is true. It is one of the loop constructs in C++ used for iteration.
What is the basic syntax of a `while` loop?
-The basic syntax of a `while` loop is: `while (condition) { // Code to execute }`. The loop executes as long as the condition evaluates to true.
What happens if the condition in a `while` loop is never met?
-If the condition is never met, the `while` loop will not execute at all. The code inside the loop will be skipped.
What is the purpose of `x++` inside the `while` loop in the example?
-`x++` is an increment operator that increases the value of `x` by 1 after each iteration. It ensures that the condition (`x <= 5`) eventually becomes false, ending the loop.
What happens when the `while` loop condition becomes false?
-When the `while` loop condition becomes false, the loop exits, and the program continues executing the code after the loop.
How does the second example in the video calculate the sum of entered numbers?
-In the second example, the program prompts the user for input five times, storing each value in the `number` variable. The value of `number` is added to the `sum` variable in each iteration to calculate the total sum.
Why do we initialize the `number` and `sum` variables to 0 in the second example?
-The variables `number` and `sum` are initialized to 0 to avoid any garbage values and to ensure that the sum calculation starts from zero and can accumulate the user inputs correctly.
What would happen if we remove the `x++` statement inside the first loop example?
-If the `x++` statement is removed, the value of `x` will remain constant (in this case, 1), and the loop will run infinitely because the condition `x <= 5` will always be true.
How can we create an infinite `while` loop?
-An infinite `while` loop can be created by setting the condition to a value that is always true, such as `while (true)`. This condition will never become false, causing the loop to run indefinitely.
Why is the `while (true)` loop used by some programmers?
-The `while (true)` loop is often used by programmers when they want to create an infinite loop, such as in cases where the loop must continually process user input or monitor system status until manually stopped.
Outlines
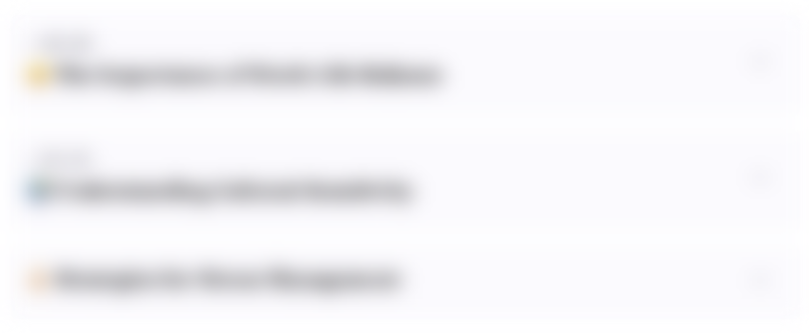
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
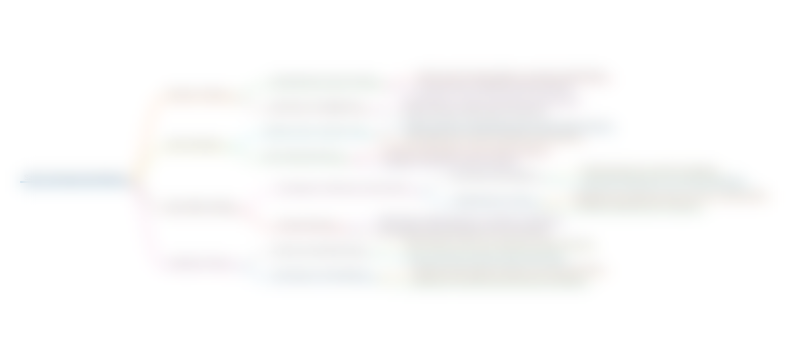
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
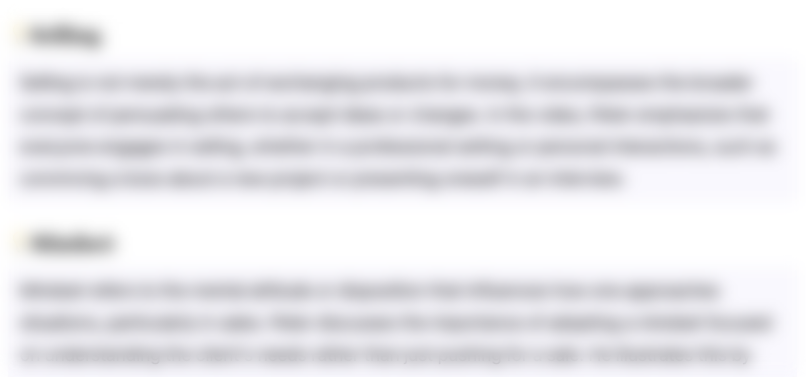
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
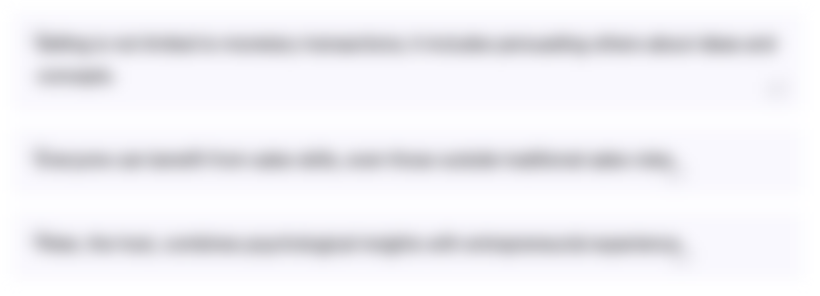
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
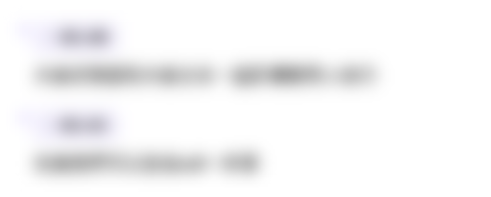
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
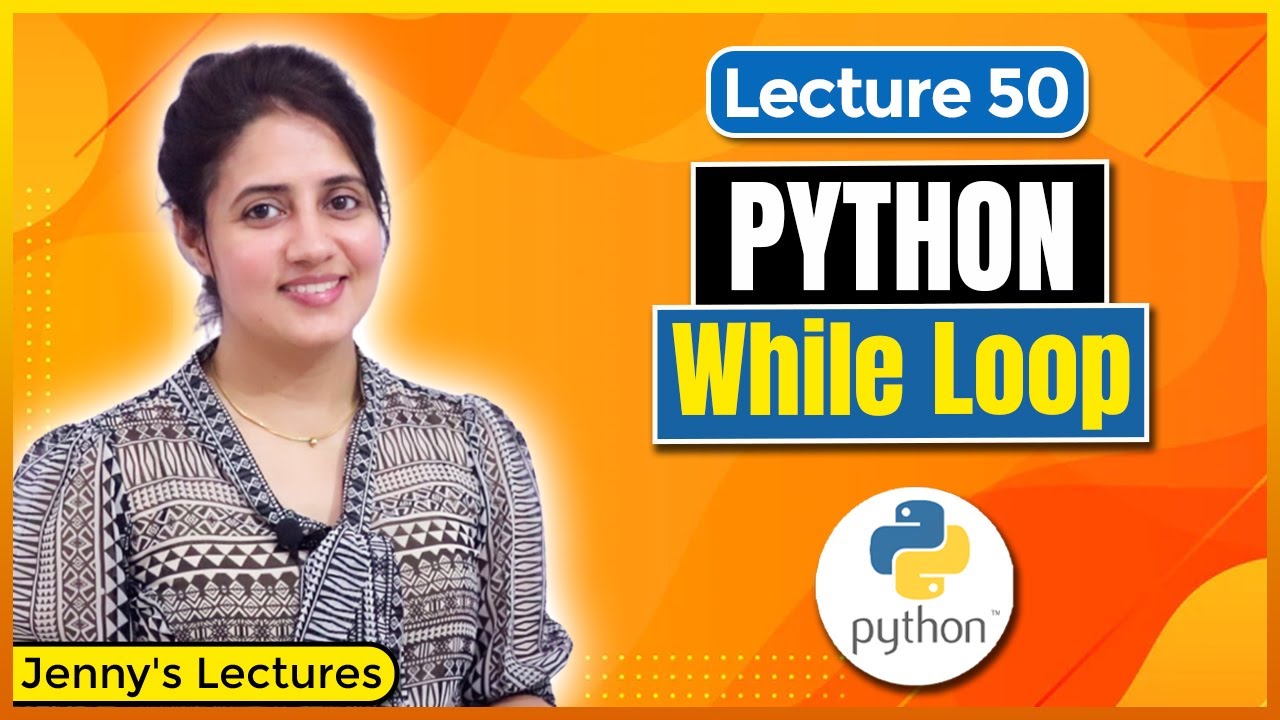
While Loop in Python | Python Tutorials for Beginners #lec50

The 'while' Statement in C++
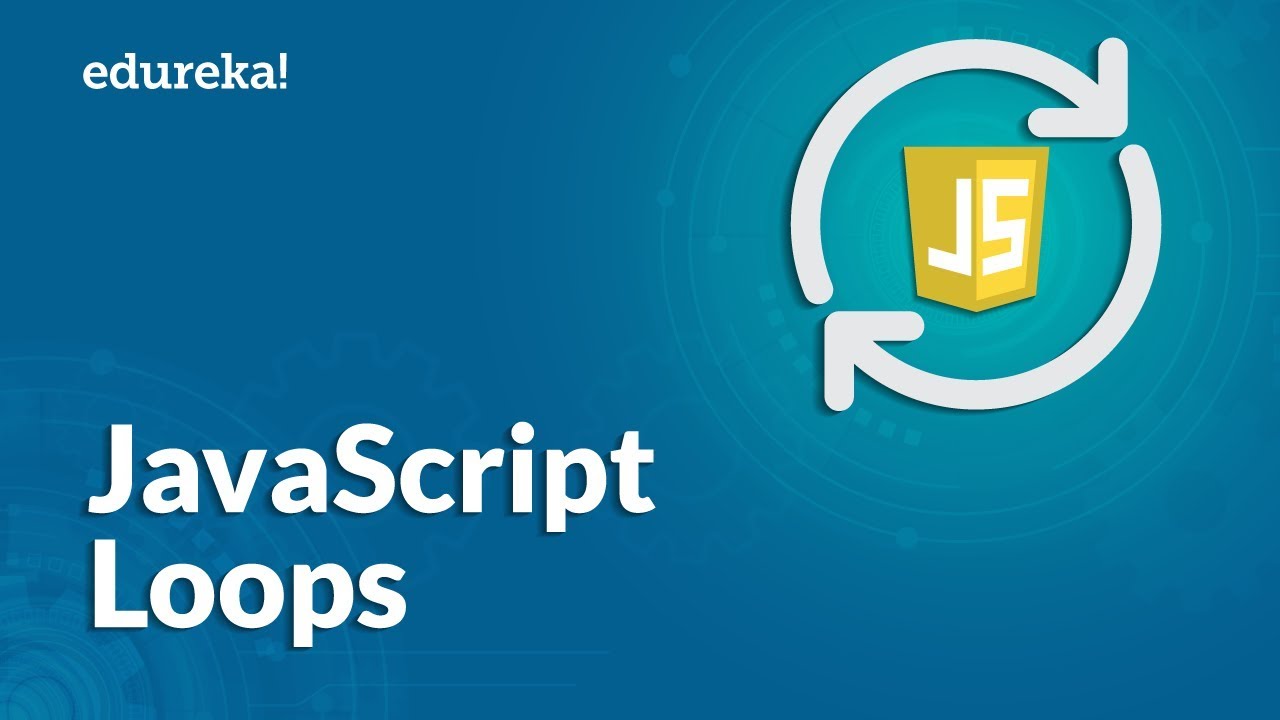
JavaScript Loops Explained | For Loop, While and Do-While Loop | JavaScript Tutorial | Edureka

C++ 03 | Tipe Data pada Pemrograman C++ | Tutorial Dev C++ Indonesia
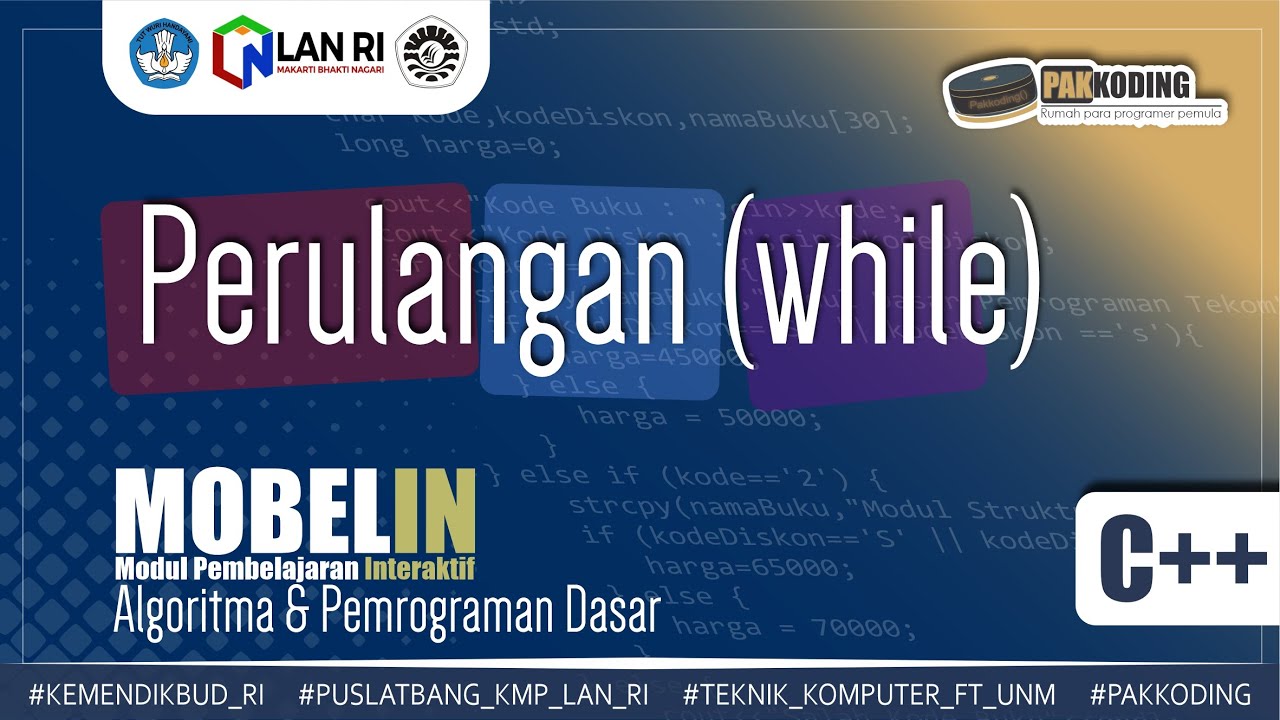
#43 Belajar Perulangan While C++
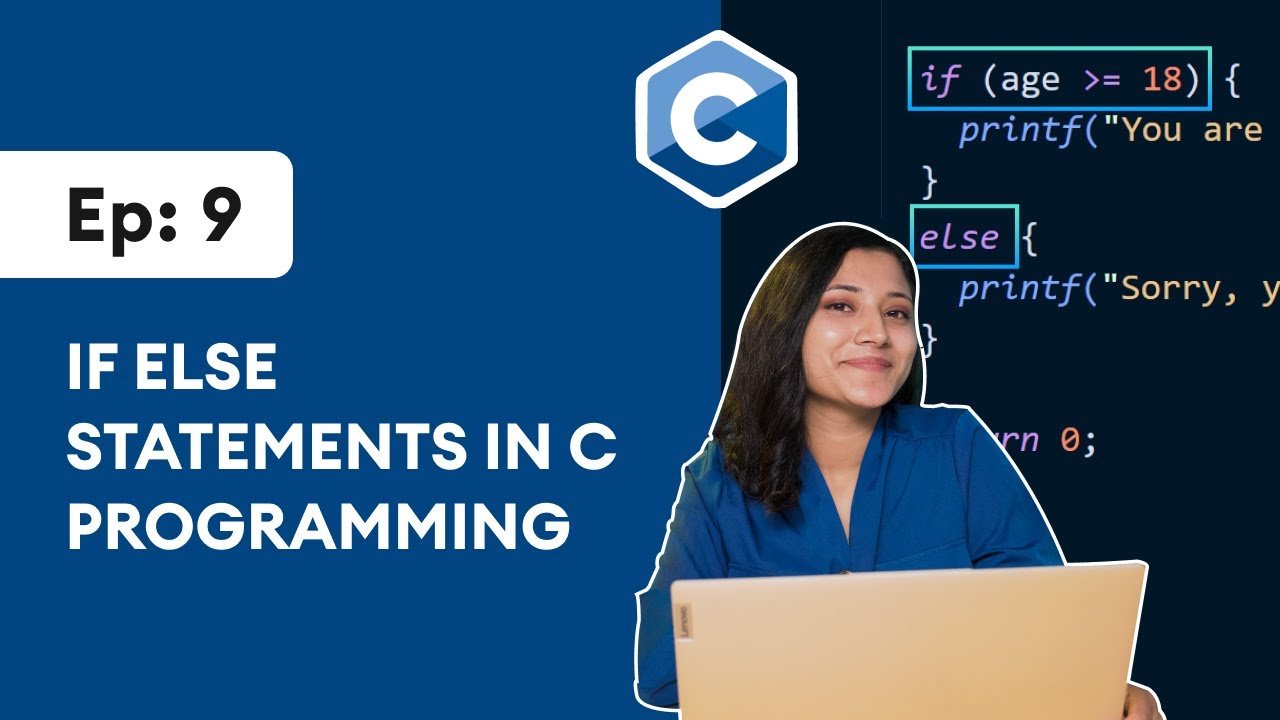
#9: If Else Statements in C | C Programming for Beginners
5.0 / 5 (0 votes)