Exception Handling In C++ | What Is Exception Handling In C++ | C++ Programming | Simplilearn
Summary
TLDRThis video tutorial from Simply Learn delves into exception handling in C++. It begins by defining exceptions as unexpected events that disrupt program execution and explains the necessity of managing these errors. The video covers the key keywords: try, catch, and throw, detailing how they work together to improve code readability and maintainability. Practical examples demonstrate handling scenarios such as age validation for drinking and division by zero, showcasing how exceptions can be effectively managed. Viewers will gain a solid understanding of how to implement exception handling in their C++ programs.
Takeaways
- 😀 Exceptions are unexpected events that occur during the execution of a program, potentially disrupting its flow.
- 😀 Exception handling is essential in C++ to manage errors and maintain program stability.
- 😀 The main keywords for exception handling in C++ are `try`, `catch`, and `throw`.
- 😀 The `try` block contains code that may cause an exception, allowing for error detection.
- 😀 The `catch` block handles exceptions thrown by the `try` block and provides relevant messages to users.
- 😀 The `throw` keyword is used to signal that an exception has occurred, passing control to the appropriate `catch` block.
- 😀 Exception handling separates error management from the rest of the code, improving readability and maintainability.
- 😀 An example of exception handling is enforcing age restrictions for drinking, where an exception is thrown if the age is below 24.
- 😀 Another example demonstrates how to handle division by zero, using exceptions to inform users of invalid input.
- 😀 Effective exception handling ensures that users receive clear feedback when errors occur, aiding in debugging and error correction.
Q & A
What are exceptions in C++?
-Exceptions are unexpected events or situations that arise during the execution of a program, causing disruptions in the normal flow of control.
Why is exception handling important in C++?
-Exception handling is crucial because it allows developers to manage errors gracefully, separate error-handling code from regular code, and provide users with informative feedback about problems.
What are the main keywords used for exception handling in C++?
-The main keywords for exception handling in C++ are try, catch, and throw.
What is the purpose of the 'try' block?
-'Try' blocks contain code that might throw an exception. If an exception occurs, control is passed to the corresponding catch block.
How does the 'catch' block function?
-The 'catch' block handles exceptions thrown by the try block. It executes code designed to respond to the specific type of exception.
What does the 'throw' keyword do?
-The 'throw' keyword is used to signal that an exception has occurred, passing the control to the corresponding catch block for handling.
Can a try block have multiple catch blocks?
-Yes, a try block can have multiple catch blocks, each designed to handle different types of exceptions.
What happens if an exception is not caught?
-If an exception is not caught, it can lead to program termination or undefined behavior, as the program does not know how to handle the error.
In the first example, what condition leads to throwing an exception?
-In the first example, an exception is thrown when the person's age is less than 24, indicating they do not have access to drink alcohol.
How is division by zero handled in the second example?
-In the second example, if the denominator is zero, an exception is thrown, which is caught in the catch block, prompting an error message to the user.
Outlines
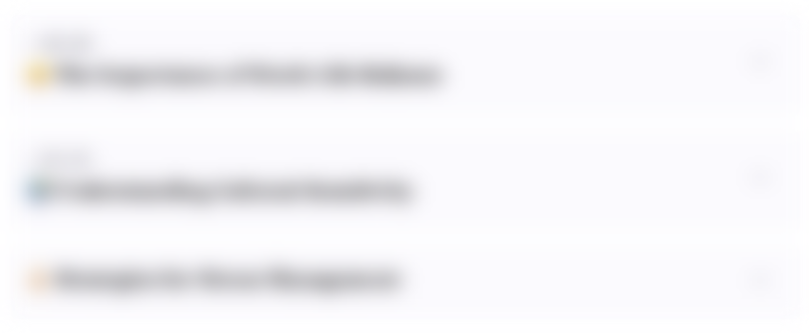
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
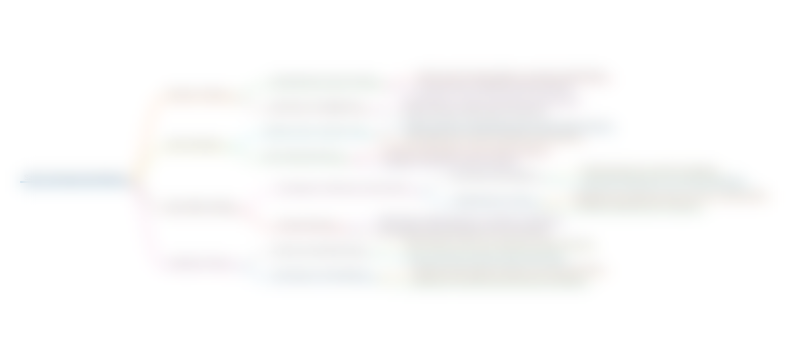
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
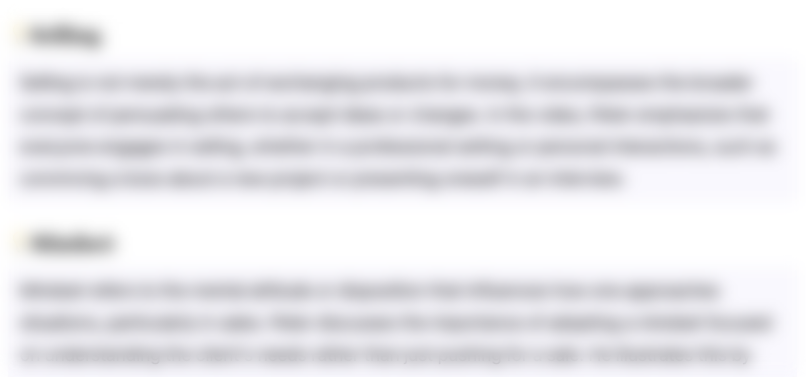
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
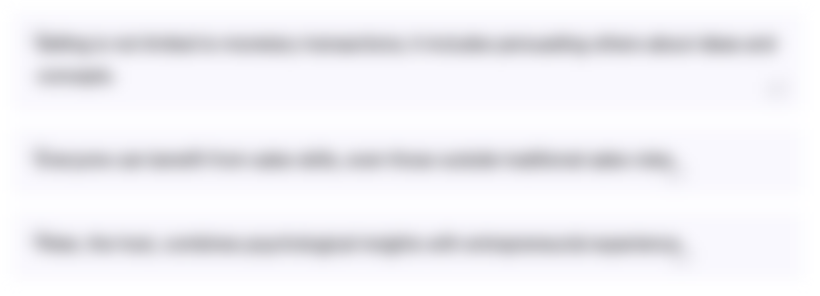
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
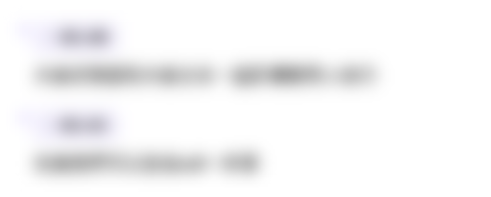
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
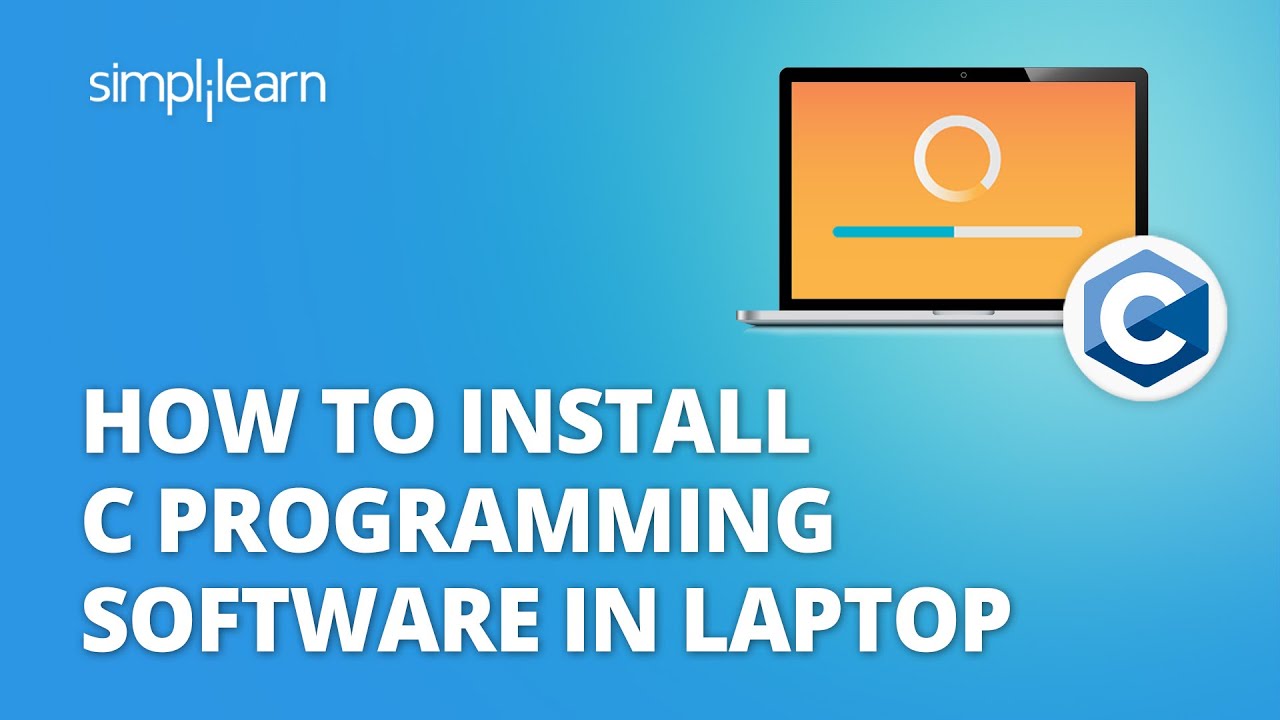
How To Install C Programming Software In Laptop | C Installation Tutorial For Beginners |Simplilearn

Part 1 Introduction || C#.Net Tutorials For Beginners & Experienced || @NehanthWorld

Top 50 Accenture Interview Questions and Answers | Accenture Interview for Freshers | Simplilearn
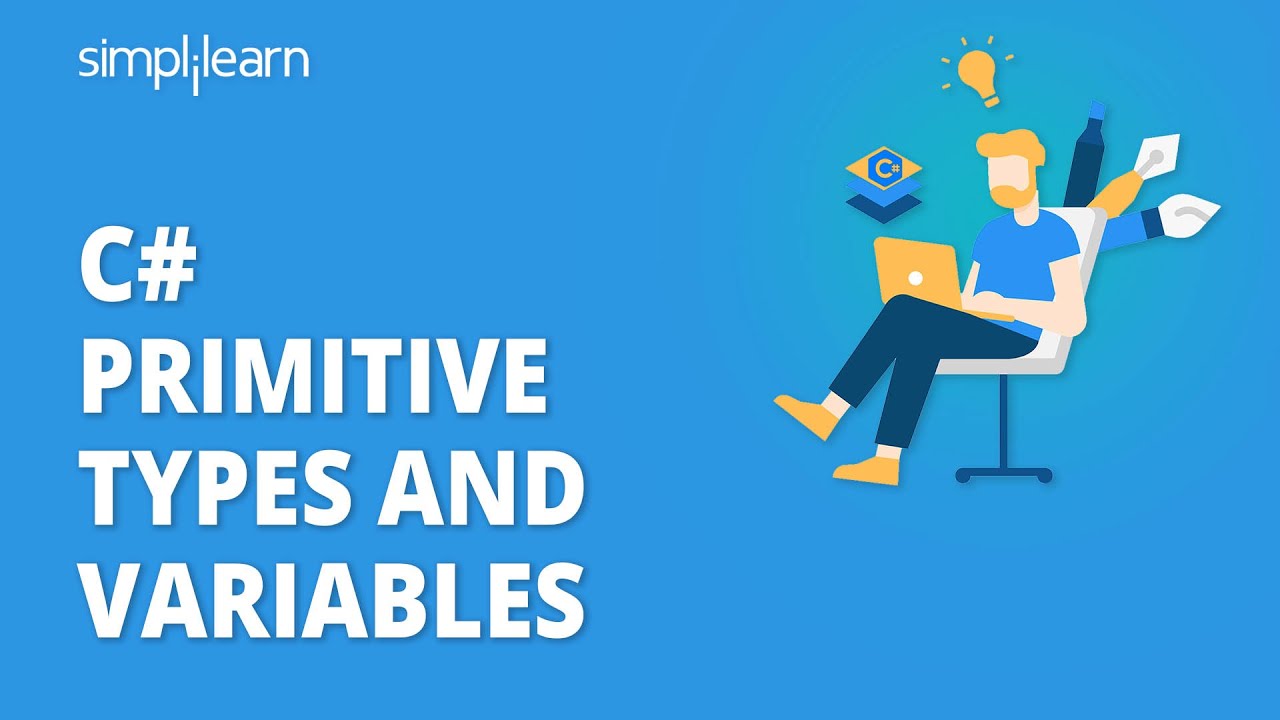
C# Primitive Types and Variables | Datatype Literals and Variables | C# Tutorial | Simplilearn

12 التنفيئذ اللحظي للإستعلامات LINQ Immediate execution methods
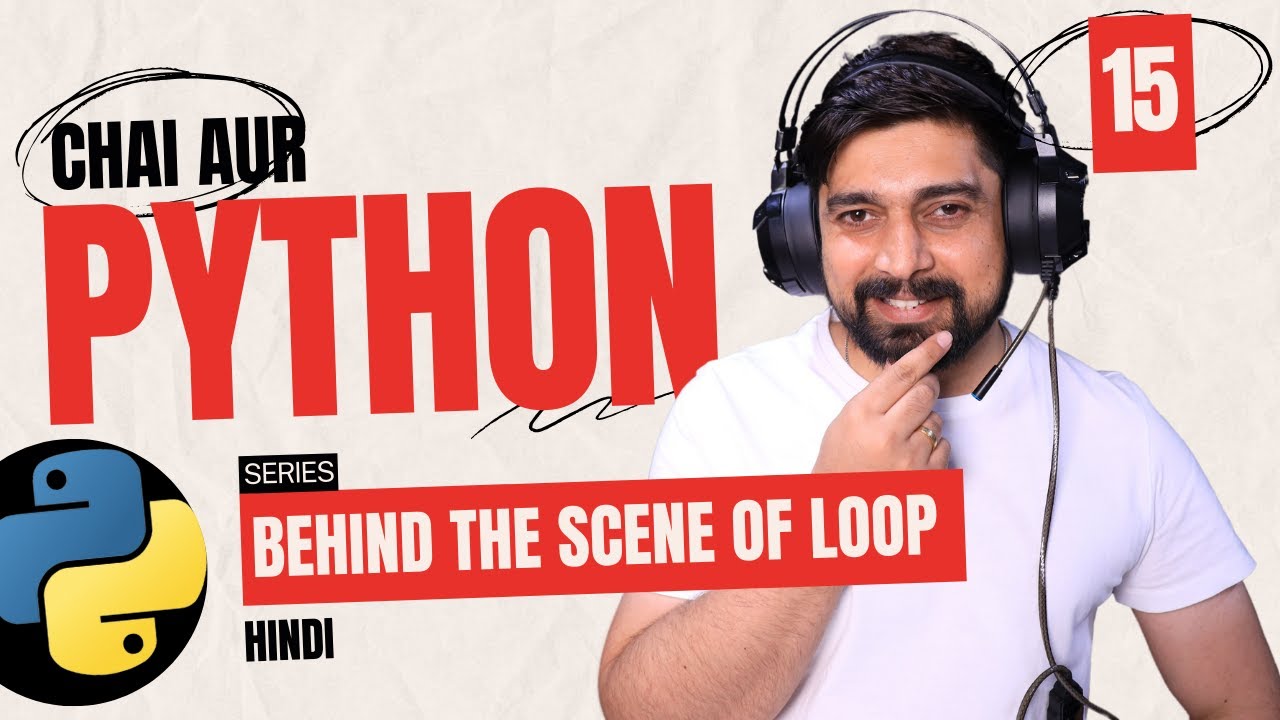
Behind the scene of loops in python
5.0 / 5 (0 votes)