#12 Create Component using Angular CLI | Angular Components & Directives | A Complete Angular Course
Summary
TLDRThis lecture explains how to create Angular components manually and with Angular CLI. It starts by detailing the process of defining a component class, adding the view template and styles, and registering the component manually in the app module. The instructor then introduces the Angular CLI command 'ng generate component' to automate these tasks, showing how it speeds up development. The lecture also covers fixing a compilation error due to a missing Bootstrap reference and demonstrates the refactoring of HTML into child components, styling them, and verifying results in the browser.
Takeaways
- 🔧 Manually creating components in Angular involves defining a class, decorating it with @Component, and registering it in the app.module.ts.
- ⚙️ Using the Angular CLI simplifies component creation by automating the generation of the component class, view template, and registration in the main module.
- 🛠️ The command 'ng generate component [component name]' automatically creates a new component with the required files and registers it in the app module.
- 🗂️ Removing unnecessary dependencies (like Bootstrap) requires cleaning up references in the angular.json file to prevent compilation errors.
- 🖥️ The CSS and HTML for a component are scoped to that component, meaning styles in one component won't affect another unless applied globally.
- 🚀 Using Angular CLI for component generation speeds up the development process by automating tasks such as registering components.
- 📁 Child components can be created inside parent component folders and referenced within the parent’s HTML for a cleaner structure.
- 🔄 Refactoring large HTML sections into separate components makes code more modular and easier to manage, as shown with the top menu and main menu components.
- 🎨 Styles for components can be moved from parent components to their respective child component CSS files for better encapsulation.
- 📜 The Angular CLI ensures that any new components are automatically declared in the main module, reducing manual intervention.
Q & A
What is the purpose of the @Component decorator in Angular?
-The @Component decorator is used to define an Angular component. It decorates a class by providing metadata about the component, such as the selector, template, and styles.
How do you manually create and register a component in Angular?
-To manually create a component, you define a class decorated with the @Component decorator. Then, register the component by adding it to the 'declarations' array in the appmodule.ts file.
What is the Angular CLI command to generate a component?
-The Angular CLI command to generate a component is 'ng generate component <component-name>'. This can also be shortened to 'ng g c <component-name>'.
What tasks are automated by using the Angular CLI to generate a component?
-When using Angular CLI, it automates the creation of the component class, view template, stylesheet, and also registers the component in the app module. This eliminates the need for manual registration.
How do you fix the error caused by uninstalling Bootstrap in an Angular project?
-The error occurs because the Angular.json file is still referring to the Bootstrap CSS file. To fix this, you need to remove the reference to 'bootstrap.min.css' from the 'styles' array in the Angular.json file.
What happens if you try to use a component selector without importing its CSS styles?
-If you use a component selector without importing its specific CSS styles, the HTML will be rendered but without any styles being applied. This is because Angular's CSS is scoped to each component, and styles are not applied globally unless specified.
How can global styles be applied to all anchor elements in an Angular project?
-To apply global styles to all anchor elements, you should add the necessary styles for the 'a' tag inside the 'styles.css' file. This will apply the styles globally across all components.
Why would you refactor the HTML inside a component?
-Refactoring the HTML in a component helps to organize the code better. For example, separating different sections like 'top menu' and 'main menu' into their own components improves maintainability and clarity.
How do you handle the CSS styles for child components in Angular?
-In Angular, CSS styles are scoped to individual components. If a child component requires specific styles, you need to move those styles from the parent component's CSS to the child component's CSS file.
Why don't you need to manually declare a component when using Angular CLI to create it?
-When using Angular CLI, it automatically declares the component in the 'declarations' array of the app module. This is one of the conveniences of using CLI, as it speeds up development by handling this task for you.
Outlines
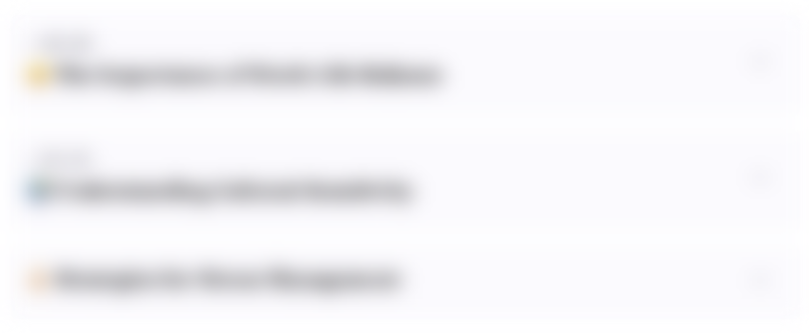
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
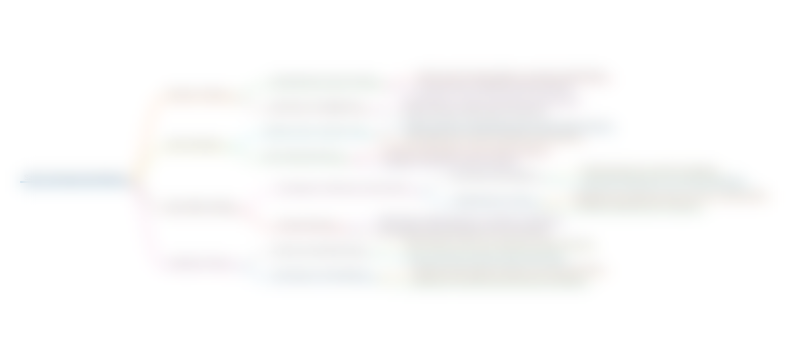
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
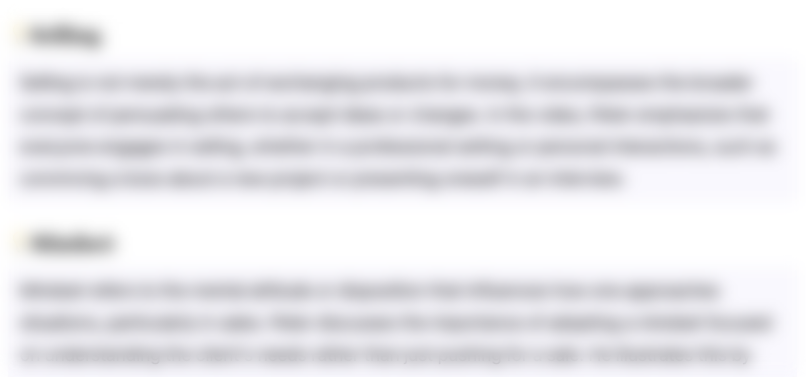
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
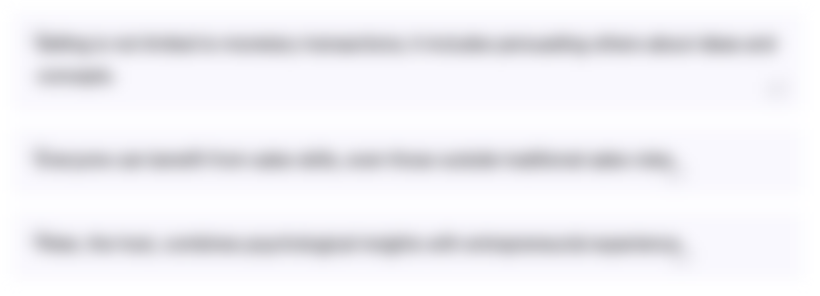
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
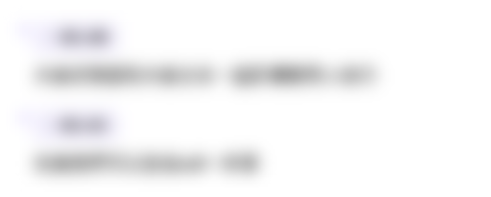
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
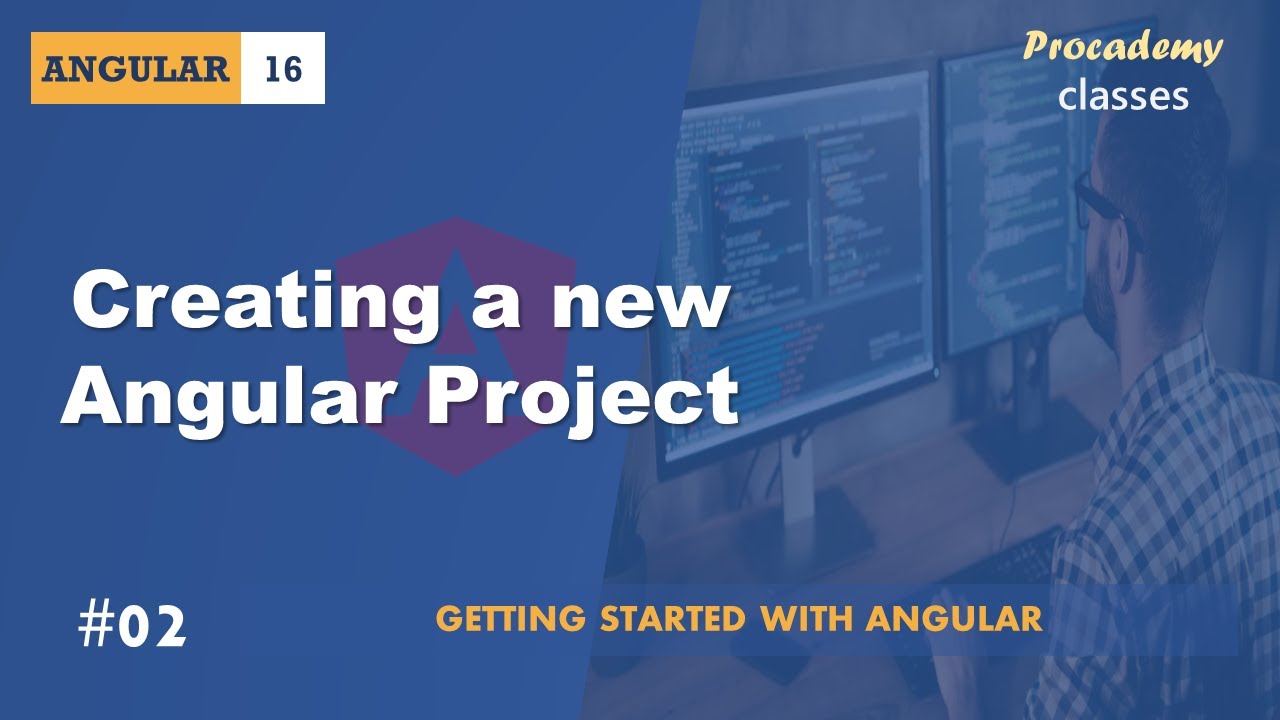
#02 Creating a new Angular Project | Getting Started with Angular | A Complete Angular Course
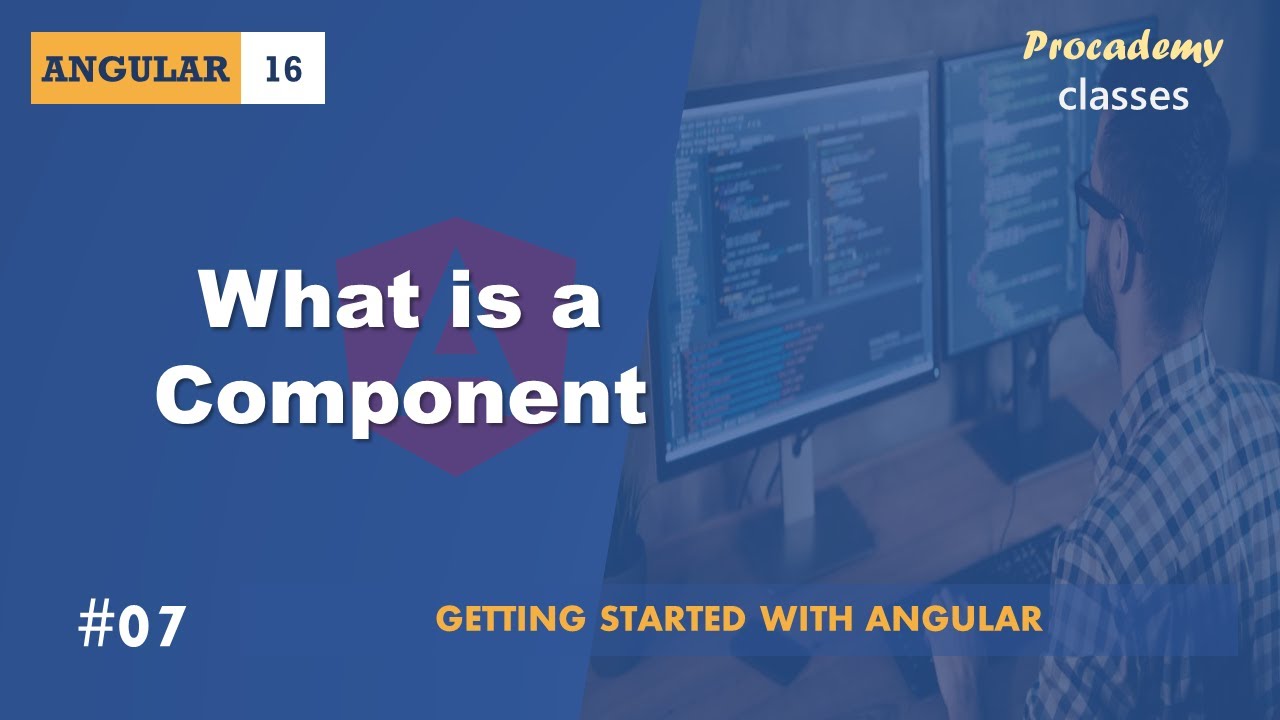
#07 What is a Component | Angular Components & Directives| A Complete Angular Course
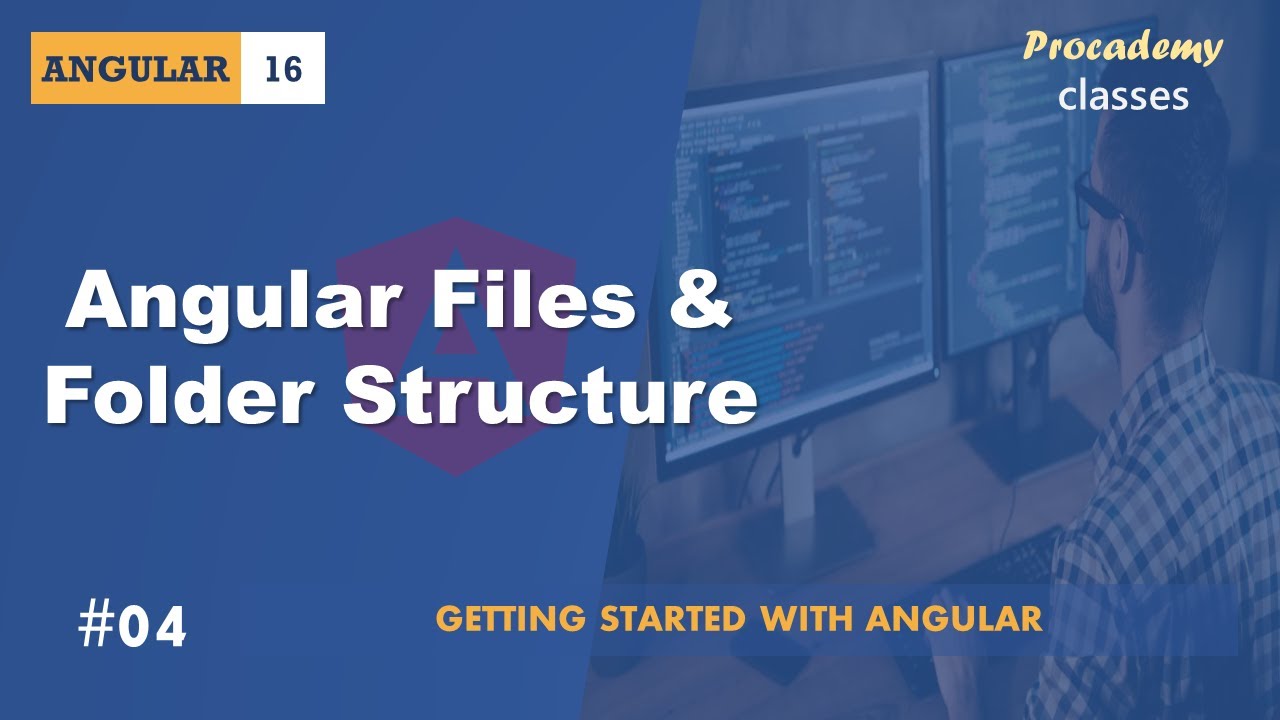
#04 Angular files and folder structure| Getting Started with Angular | A Complete Angular Course
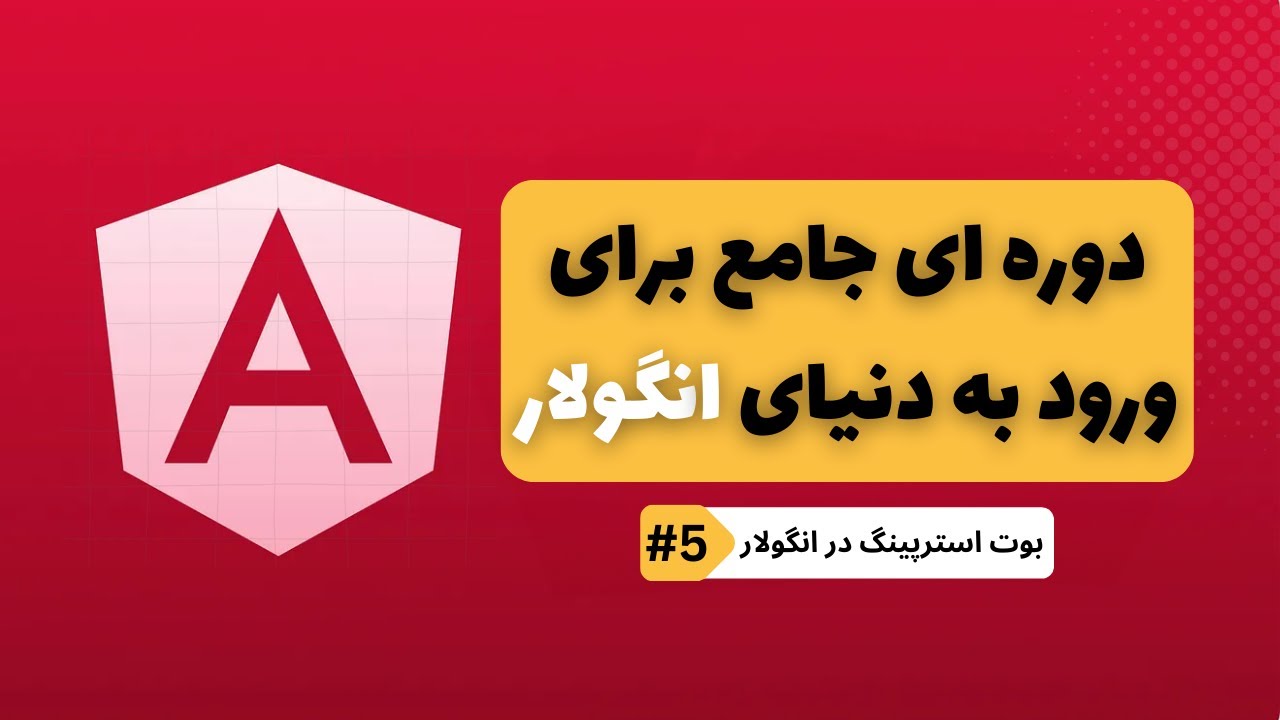
#5 بوت استرپینگ در انگولار| دوره ای جامع برای ورود به دنیای انگولار

#03 Editing the First Angular Project | Getting Started with Angular | A Complete Angular Course
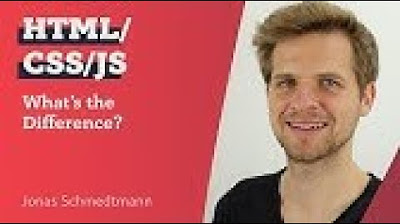
FAQs Angular Maximilian Schwarzmuller
5.0 / 5 (0 votes)