Finalize vs Dispose | C# Interview Questions
Summary
TLDRIn this video, Nathan from Code First Channel explains the difference between 'Dispose' and 'Finalize' in .NET. Managed resources are handled by the garbage collector, while unmanaged resources require manual disposal. Nathan clarifies when to use 'Dispose' and 'Finalize', emphasizing the importance of implementing the Dispose pattern for unmanaged resources. He provides a step-by-step guide on implementing the Dispose pattern and the optional use of Finalize, concluding with advice to avoid Finalize unless necessary.
Takeaways
- 🧠 **Understanding Dispose and Finalize**: .NET objects can use managed and unmanaged resources. Managed resources are handled by the garbage collector, while unmanaged resources require manual disposal.
- 🔄 **Dispose Pattern**: The Dispose pattern is used to release unmanaged resources as soon as they are no longer needed, like database connections or file handles.
- 📜 **Syntax Differences**: `Finalize` is implemented as a method within a class, while `Dispose` is implemented by deriving from `IDisposable` interface and overriding the `Dispose` method.
- ⏱️ **Execution Timing**: `Finalize` is called by the garbage collector in a non-deterministic way, whereas `Dispose` can be explicitly called to release resources immediately.
- 🚫 **Finalize Limitations**: It's not recommended to rely on `Finalize` to dispose of unmanaged resources because its execution timing is unpredictable.
- 📅 **Dispose Method**: Can be called explicitly to clean up unmanaged resources at a desired time, providing more control over resource management.
- 🔄 **Implementing Dispose and Finalize**: Microsoft suggests a pattern where `Dispose` is implemented to clean up both managed and unmanaged resources, and `Finalize` is only used if necessary.
- 🔗 **GC.SuppressFinalize**: When `Dispose` is called, `GC.SuppressFinalize` is used to prevent the garbage collector from calling `Finalize`, as resources are already cleaned up.
- 🛠️ **Virtual Dispose Method**: The virtual `Dispose(bool disposing)` method is used to clean up both managed and unmanaged resources, with `disposing` indicating whether managed resources should be cleaned up.
- 🔒 **Safe Handle**: An alternative to `Finalize` for disposing of unmanaged resources is using a `SafeHandle`, which abstracts the unmanaged resources and ensures they are released when `Dispose` is called.
Q & A
What are the two types of resources an object can use in .NET?
-In .NET, an object can use managed resources, which are created and handled by the common language runtime and the garbage collector, and unmanaged resources, which lie outside the domain of the common language runtime and require manual cleanup by the programmer.
Why is it necessary to manually dispose of unmanaged resources?
-Unmanaged resources, such as open database connections or file handles, are not automatically cleaned up by the garbage collector. Therefore, it is necessary for programmers to manually dispose of these resources to prevent memory leaks or other resource-related issues when they are no longer needed.
What is the primary difference between the Dispose method and the Finalize method?
-The Dispose method can be explicitly called to release resources immediately, while the Finalize method is called by the garbage collector in a non-deterministic way, meaning there is no guarantee when it will be executed.
How does the garbage collector handle managed resources by default?
-By default, the garbage collector disposes of an object's managed resources when the object is no longer referenced from anywhere in the application.
What is the purpose of implementing the IDispose interface?
-Implementing the IDispose interface allows a class to define a pattern for releasing both managed and unmanaged resources, ensuring that resources are cleaned up deterministically when the object is no longer needed.
What is the role of the 'disposing' parameter in the Dispose method?
-The 'disposing' parameter in the Dispose method indicates whether the method has been called from the public Dispose method (disposing == true) or from the object's finalizer (disposing == false). This allows the method to differentiate between cleaning up managed resources and cleaning up both managed and unmanaged resources.
Why is it important to suppress finalization after calling Dispose?
-Suppressing finalization after calling Dispose is important because it prevents the garbage collector from calling the Finalize method on the object again, which is unnecessary if the resources have already been cleaned up by Dispose.
Can you provide an example of a common pattern for disposing of unmanaged resources?
-A common pattern for disposing of unmanaged resources is to implement the Dispose method and use a 'using' block in C#, which ensures that the Dispose method is called automatically when the object goes out of scope.
What is a safe handle and how does it relate to resource disposal?
-A safe handle is a type of object that encapsulates an unmanaged resource and provides a mechanism for releasing that resource deterministically. When the Dispose method is called on an object that uses a safe handle, the safe handle's Dispose method can be invoked to release the unmanaged resource.
Why might a developer choose to implement a Finalize method even after implementing Dispose?
-A developer might choose to implement a Finalize method as a fallback mechanism to clean up unmanaged resources in case the Dispose method is not called. However, this is generally discouraged because it introduces non-determinism and can lead to performance issues.
What is the recommended approach to resource management in .NET classes?
-The recommended approach is to implement the Dispose method following the dispose pattern suggested by Microsoft, which includes implementing the IDispose interface, creating a public Dispose method, and a protected virtual Dispose(bool disposing) method. This pattern ensures that both managed and unmanaged resources are cleaned up properly.
Outlines
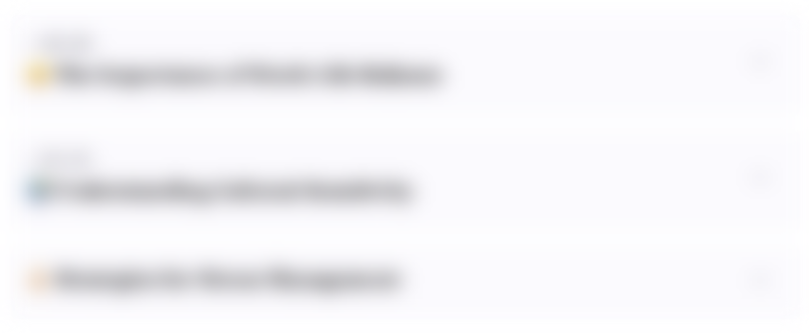
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
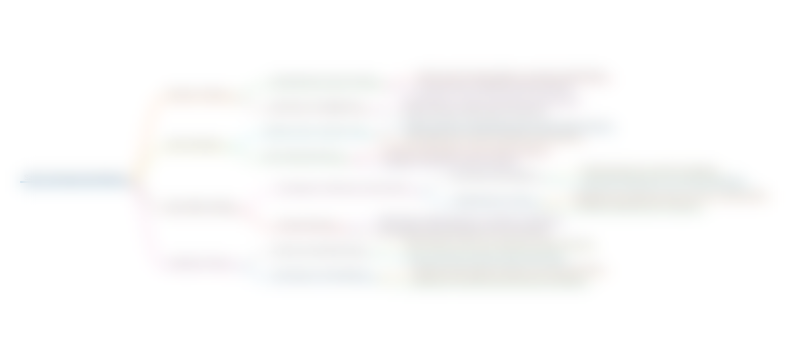
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
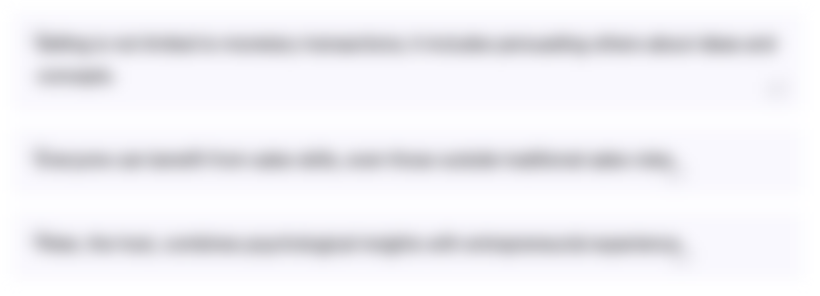
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
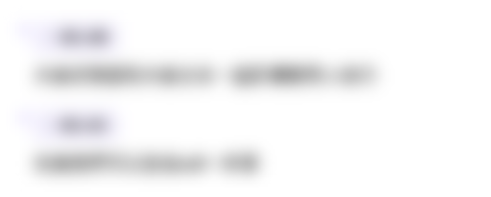
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
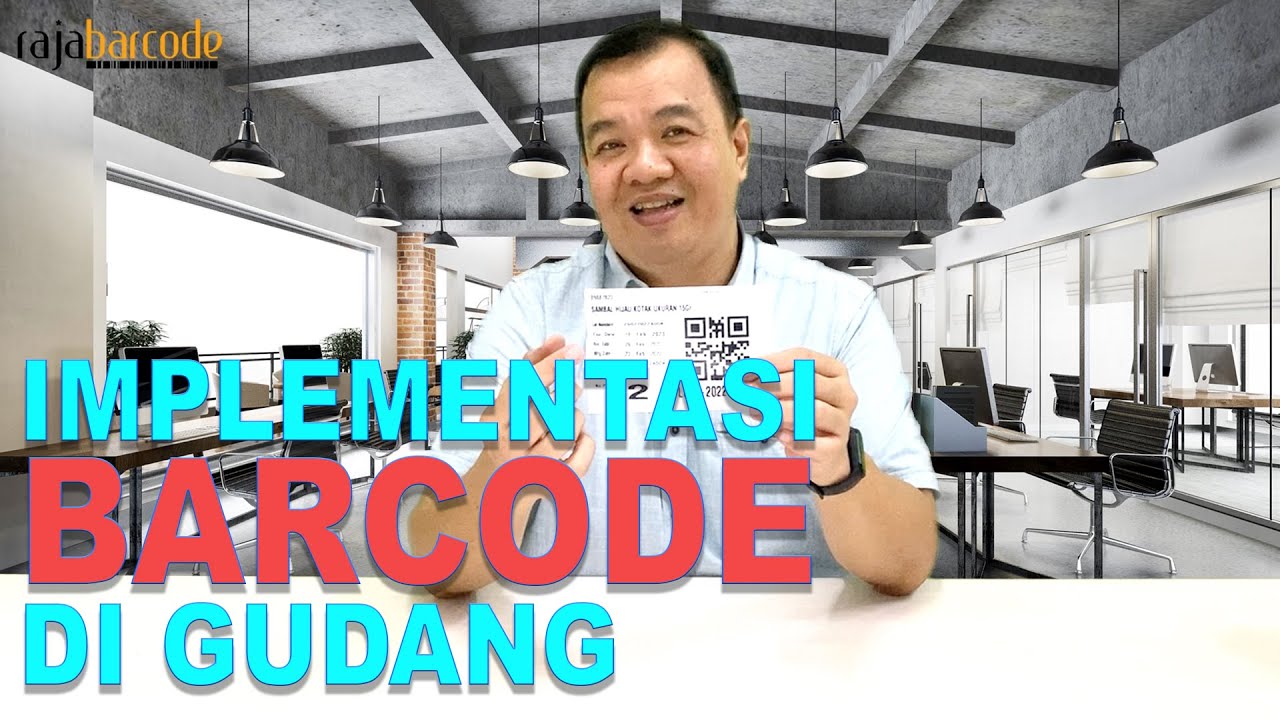
Penggunaan BARCODE di Gudang

Qu’est-ce qu’un cookie ? (web) - 1 Minute pour comprendre

Passive transport | membrane transport lecture
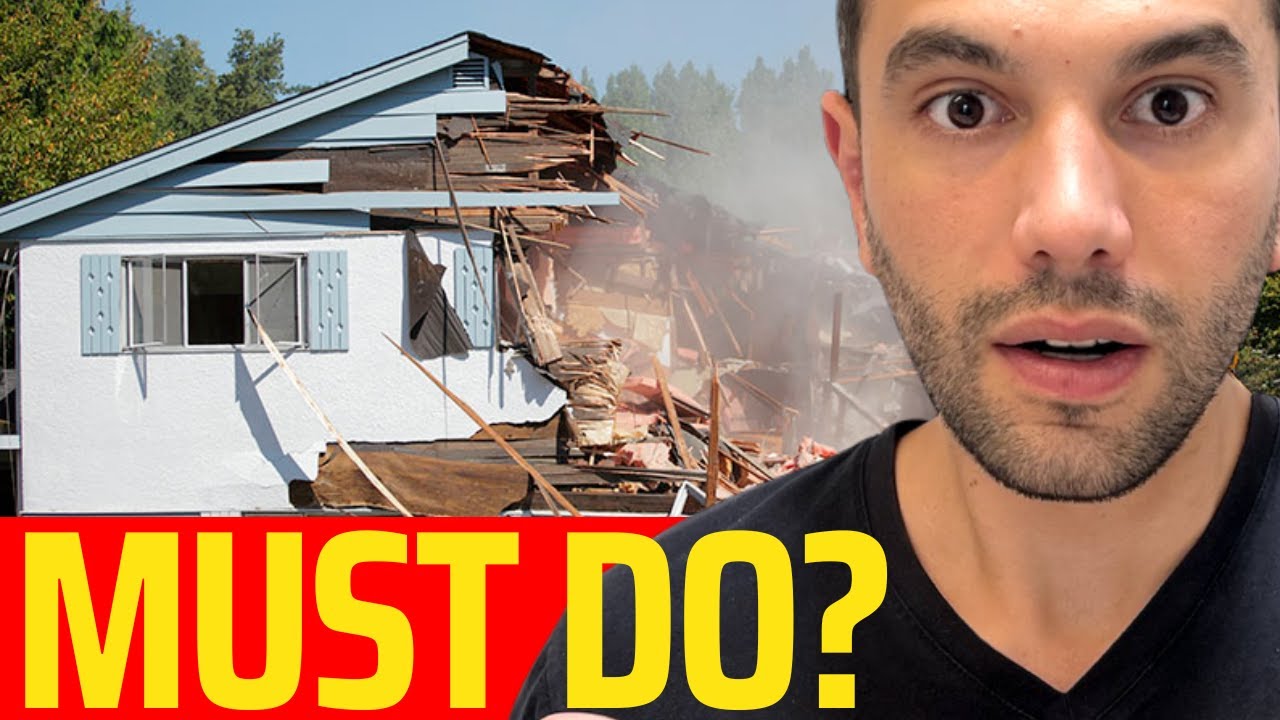
Building and Pest Inspection Australia - The Ultimate Guide [Everything You Need To Know]

PERBANDINGAN BAND 20MHZ DAN 40MHZ DAN CARA SETTING NYA DI DALAM ROUTER WIFI INDOOR DAN OUTDOOR
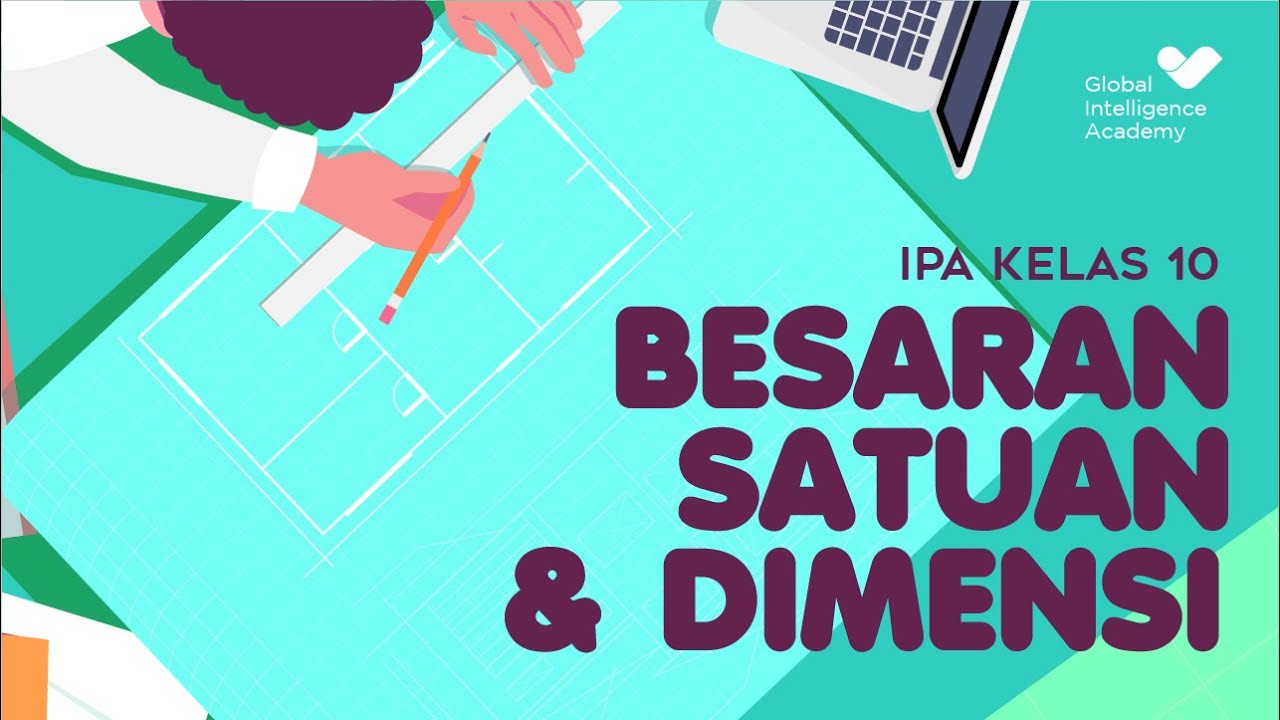
IPA Kelas 10 - Besaran, Satuan, dan Dimensi | GIA Academy
5.0 / 5 (0 votes)