Comparison, Logical, and Membership Operators in Python | Python for Beginners
Summary
TLDRThis video tutorial offers a comprehensive overview of Python's comparison, logical, and membership operators. It explains how to use operators like '==', '!=', '>', '<', '>=', and '<=' to compare values. The tutorial also covers logical operators 'and', 'or', and 'not', illustrating their use with examples. Finally, it introduces membership operators 'in' and 'not in' to check for the presence of values within sequences, enhancing understanding of fundamental Python operations.
Takeaways
- 🔢 **Comparison Operators**: Python uses comparison operators like `==` (equal), `!=` (not equal), `>` (greater than), `<` (less than), `>=` (greater than or equal to), and `<=` (less than or equal to) to compare values.
- ❌ **Literal Assignment Error**: Trying to compare a literal like `10 == 10` without assignment will result in an error because it's interpreted as an assignment attempt.
- 📌 **Boolean Results**: Comparison operations return boolean values (`True` or `False`).
- 🔄 **Comparing Different Types**: You can compare different data types like numbers and strings using comparison operators.
- 🔄 **Variable Comparison**: Variables can be compared to check if they hold the same value.
- 📈 **Logical Operators**: Logical operators `and`, `or`, and `not` are used to combine comparison operations.
- 🔒 **AND Operator**: The `and` operator returns `True` only if both conditions are `True`.
- 🔄 **OR Operator**: The `or` operator returns `True` if at least one of the conditions is `True`.
- ❌ **NOT Operator**: The `not` operator inverts the result of a condition, turning `True` to `False` and vice versa.
- 📚 **Membership Operators**: `in` and `not in` are used to check if a value or string is present within another sequence.
- 🍦 **String Membership**: You can use `in` to check if a substring exists within a larger string.
- 📝 **List Membership**: Similarly, `in` can be used to check if an element is in a list, and `not in` for its absence.
Q & A
What are comparison operators in Python?
-Comparison operators in Python are used to compare two values to determine their relationship. They include operators like '==' for equality, '!=' for inequality, '>' for greater than, '<' for less than, '>=' for greater than or equal to, and '<=' for less than or equal to.
Why can't you assign a value to a literal in Python?
-In Python, you cannot assign a value to a literal because literals are not variables. They represent fixed values and cannot be reassigned. For example, trying to assign a value to '10' directly would result in an error because '10' is not a variable but a literal number.
How do you check for equality in Python?
-To check for equality in Python, you use the '==' operator. For example, '10 == 10' would return 'True' because both sides of the operator are equal.
What is the output of '10 != 50' in Python?
-The expression '10 != 50' checks if 10 is not equal to 50, which is true, so the output would be 'True'.
Can comparison operators be used with strings in Python?
-Yes, comparison operators can be used with strings in Python. They compare the strings based on alphabetical order. For example, 'vanilla != chocolate' would return 'True' because 'vanilla' comes after 'chocolate' in the alphabet.
What is the purpose of logical operators in Python?
-Logical operators in Python are used to combine multiple conditions. They include 'and', 'or', and 'not'. 'and' returns True if both conditions are True, 'or' returns True if at least one condition is True, and 'not' inverts the result of a condition.
How does the 'and' operator work in Python?
-The 'and' operator in Python returns True only if both of the conditions it combines are True. If both conditions are True, the overall result is True; otherwise, it's False.
What is the result of '10 > 50 or 50 > 10'?
-The expression '10 > 50 or 50 > 10' uses the 'or' operator. Since one of the conditions ('50 > 10') is True, the overall result is True, even though the other condition ('10 > 50') is False.
How can you check if a value is in a list using membership operators?
-To check if a value is in a list, you can use the 'in' membership operator. For example, if you have a list called 'scoops' containing [1, 2, 3, 4, 5], then '2 in scoops' would return True because 2 is an element of the list.
What does the 'not in' operator do in Python?
-The 'not in' operator in Python checks if a specified value is not present in a sequence. If the value is not found, it returns True. For example, '6 not in scoops' would return True if 'scoops' is a list that does not contain the number 6.
Outlines
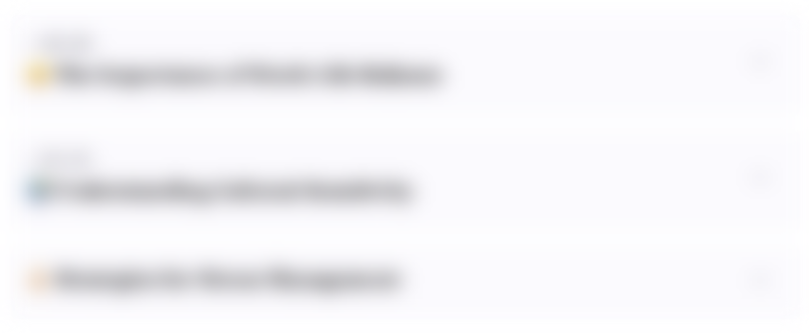
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
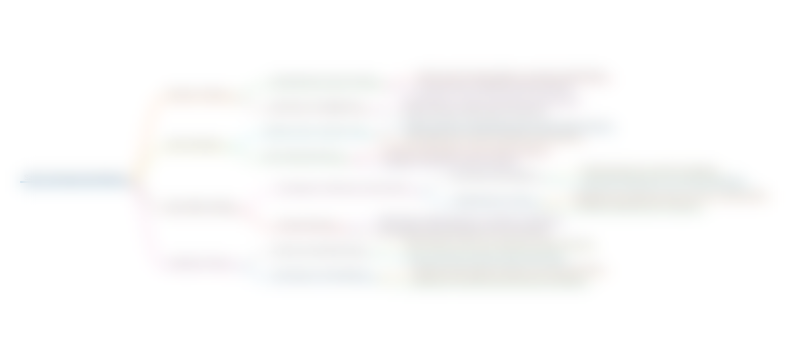
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
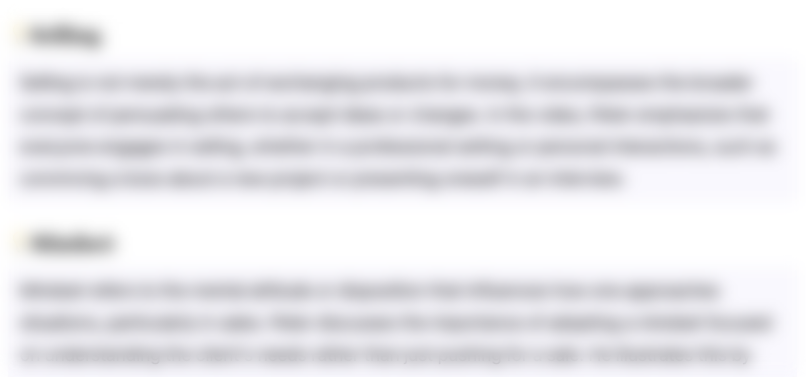
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
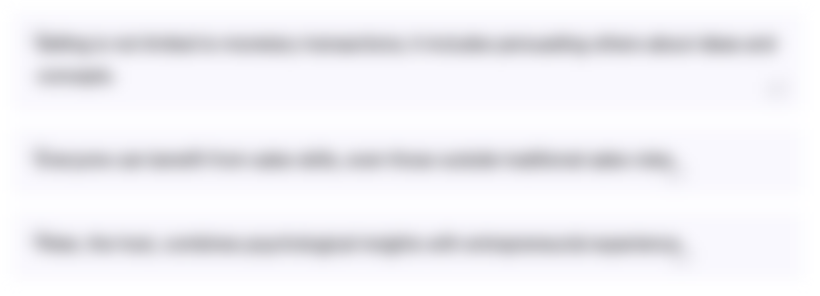
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
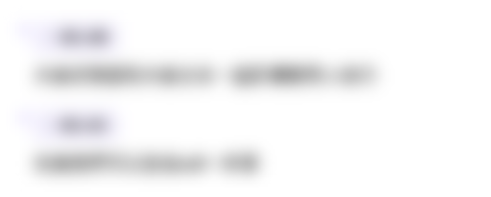
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
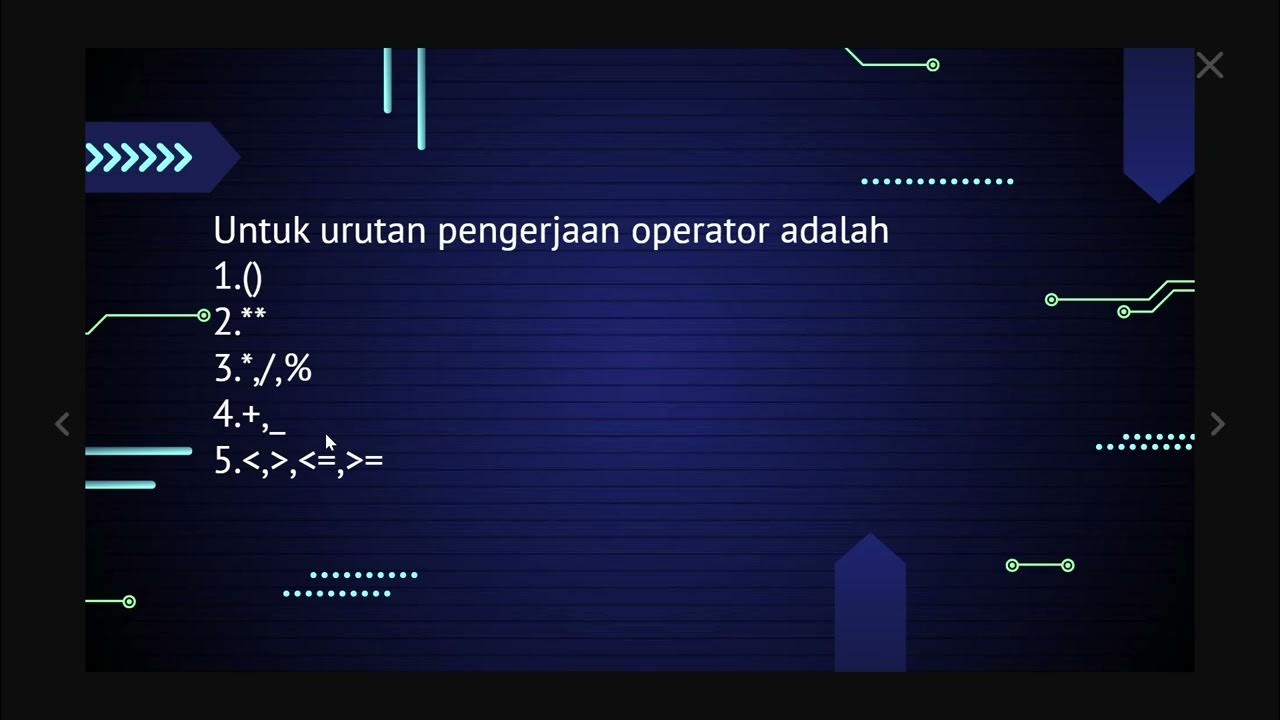
ALGORITMA dan PEMROGRAMAN || OPERATOR
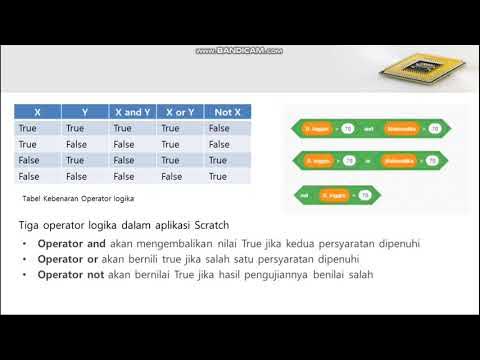
Informatika Kelas 8 Menggunakan Kondisi Percabangan Hal. 93-100
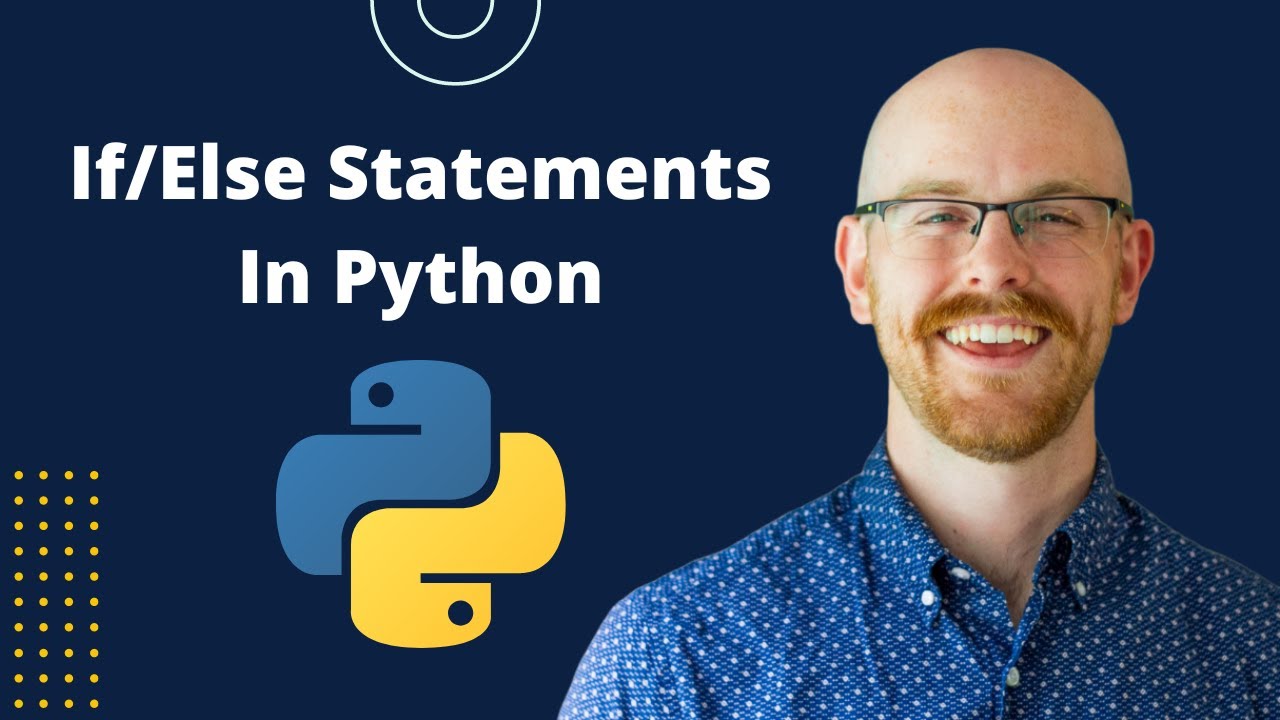
If Else Statements in Python | Python for Beginners

Penerapan Konsep Variabel Tipe Data dan Operator Pada Pemrograman Visual

81 Math Symbols Explained

Operators & Operands | Godot GDScript Tutorial | Ep 02
5.0 / 5 (0 votes)