42. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 3 procedures & functions
Summary
TLDRIn this segment of a programming video series, the focus is on subroutines, procedures, and functions. Subroutines are blocks of code with unique names that simplify complex problems into manageable parts, aiding in code writing, debugging, and reuse. Procedures are subroutines that perform tasks without returning values, while functions perform tasks and return values. In object-oriented programming, these are known as methods. The video uses a Python program to illustrate how subroutines are called and executed, highlighting their role in making code modular, reusable, and easier to test. It also touches on pseudocode formatting for exams and the benefits of using subroutines.
Takeaways
- 💡 A subroutine is a block of code with a unique name that simplifies programming by breaking down complex problems into smaller, manageable tasks.
- 🔍 The term 'subroutine' encompasses two concepts: 'procedures', which are blocks of code that perform a task, and 'functions', which perform a task and return a value.
- 📚 In object-oriented programming, subroutines are referred to as 'methods', which can either return a value or not, similar to functions and procedures.
- 👀 Subroutines in a program are typically defined at the top, with the main program starting at the bottom, making the structure clear and organized.
- 🔑 The main program calls subroutines, which are executed in sequence until completion, after which control returns to the main program.
- 🎯 Procedures and functions are fundamental to programming, with functions being a type of subroutine that returns a value, useful for calculations and data manipulation.
- 🧩 Subroutines promote code reusability, making it easier to maintain and test programs, as they can be grouped into libraries for use across different programs.
- 📝 The script introduces a Python program example to illustrate how subroutines are defined and called within a program, emphasizing practical application.
- 🔄 Flowcharts use specific symbols to represent the calling of subroutines, showing the flow of control between different parts of a program.
- 📖 For exams, students are not expected to memorize pseudocode syntax but should be familiar with it, as exam questions might use unfamiliar syntax.
Q & A
What is a subroutine?
-A subroutine is a block of code given a unique, identifiable name within a program. It is used to break down a larger problem into smaller, more manageable problems, making the code easier to write, debug, and reuse.
What is the difference between a procedure and a function?
-Procedures are blocks of code that carry out a set task, while functions are blocks of code that carry out a set task and also return a value.
In the context of object-oriented programming, what are procedures and functions called?
-In object-oriented programming, procedures and functions are referred to as methods. Methods can either return a value or not.
How are subroutines identified in a program?
-Subroutines can be identified as they start with either the word 'procedure' or 'function' and end with 'end procedure' or 'end function'.
What is a procedure call?
-A procedure call is when a program jumps to a subroutine with the same name and starts executing the code inside it until it reaches the end, at which point it returns to the place where it left off originally.
Why are subroutines useful in programming?
-Subroutines are useful because they make programs easier to write and debug, create reusable components, can be grouped in libraries for easy reuse across different programs, and make programs easier to test.
How does a function differ from a procedure in terms of its execution?
-A function differs from a procedure in that it returns a value, which is typically the contents of a variable at the point of return, whereas a procedure does not return a value.
What is the purpose of the 'import random' line in the provided Python program?
-The 'import random' line imports the random library of functions into the program, allowing the use of its functionalities within the program.
What is pseudocode and why is it used in exams?
-Pseudocode is a high-level description of the operating logic of a program or other algorithm. It is used in exams to provide a familiar format for learners, regardless of the programming language they have been learning, to demonstrate their understanding of programming concepts.
Why is it important for students to be familiar with the language guide used in external assessments?
-It is important for students to be familiar with the language guide used in external assessments to understand the format and syntax that procedures and functions will be displayed in during the exams, even if they are not expected to memorize or replicate the exact syntax.
How does the use of subroutines affect the main program's code?
-The use of subroutines typically results in the main program having very little code, with most of the functionality contained in separate blocks or subroutines, which are called from within the main program.
Outlines
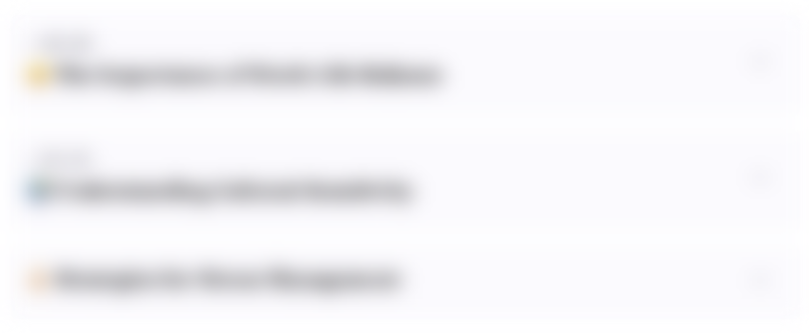
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
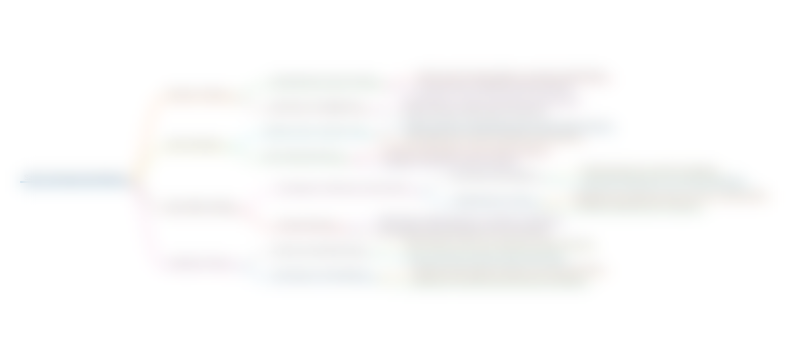
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
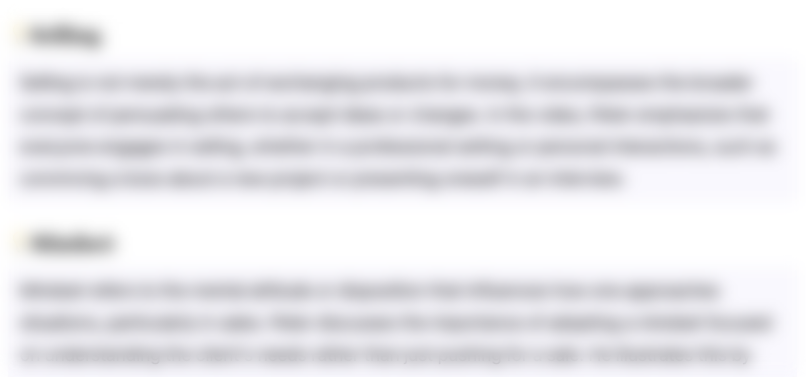
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
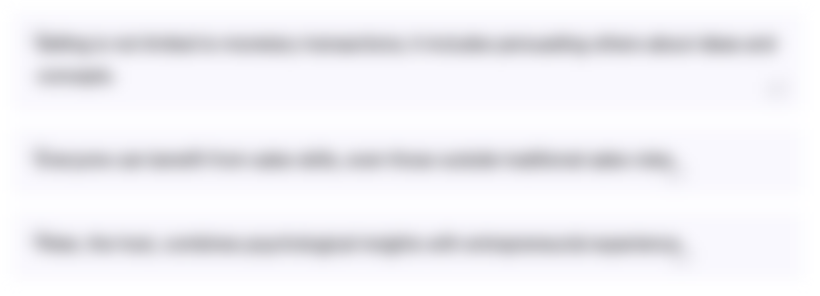
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
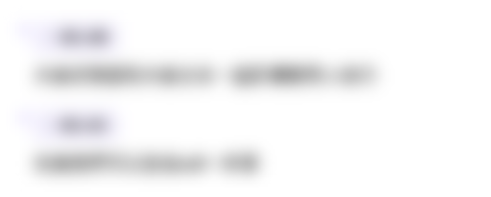
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
5.0 / 5 (0 votes)