4.2 All Pairs Shortest Path (Floyd-Warshall) - Dynamic Programming
Summary
TLDRThis video tutorial addresses the All Pairs Shortest Path problem, proposing a dynamic programming solution. It contrasts Dijkstra's algorithm, which finds single-source shortest paths, with the presented approach, which computes the shortest paths between every pair of vertices. The video demonstrates how to construct a distance matrix and iteratively improve it by considering intermediate vertices. The formula for updating the matrix is explained, and sample code is provided to implement the solution, culminating in a time complexity of O(n^3).
Takeaways
- 😀 The problem discussed is the All Pairs Shortest Paths, which involves finding the shortest path between every pair of vertices in a graph.
- 🔍 The video explains that while Dijkstra's algorithm can solve the single-source shortest path problem, applying it to all vertices would result in an O(n^3) time complexity.
- 🛠️ Dynamic programming is introduced as a method to solve the All Pairs Shortest Paths problem more efficiently by breaking it down into smaller subproblems.
- 📊 The concept of a distance matrix is used to represent the shortest paths, where each cell represents the shortest distance between two vertices, either directly or via an intermediate vertex.
- 🔄 The video demonstrates how to iteratively improve the distance matrix by considering intermediate vertices, starting from the first vertex and continuing to the last.
- 💡 The formula for updating the distance matrix is presented, which involves taking the minimum of the existing distance or the sum of the distances through the intermediate vertex.
- 💻 The script includes a step-by-step explanation of how to implement the dynamic programming solution in code, emphasizing the nested loops required to calculate the shortest paths.
- 📝 The code snippet provided in the video script is a practical example of how to apply the dynamic programming approach to solve the All Pairs Shortest Paths problem programmatically.
- ⏱️ The time complexity of the dynamic programming solution for the All Pairs Shortest Paths problem is O(n^3), which is more efficient than applying Dijkstra's algorithm to each vertex individually.
- 🔑 The key takeaway is that dynamic programming offers a powerful approach to solving optimization problems by building up solutions to complex problems from solutions to simpler subproblems.
Q & A
What is the problem discussed in the video?
-The problem discussed in the video is the All Pairs Shortest Paths problem, which involves finding the shortest path between every pair of vertices in a graph.
How does the All Pairs Shortest Paths problem differ from the Single Source Shortest Path problem?
-The All Pairs Shortest Paths problem differs from the Single Source Shortest Path problem in that it aims to find the shortest paths between every pair of vertices, whereas the Single Source Shortest Path problem focuses on finding the shortest path from one source vertex to all other vertices.
What is the time complexity of solving the All Pairs Shortest Paths problem using Dijkstra's algorithm on all vertices?
-If Dijkstra's algorithm is run on all vertices one by one, the time complexity for solving the All Pairs Shortest Paths problem would be O(n^3), where n is the number of vertices.
How does the video suggest solving the All Pairs Shortest Paths problem using dynamic programming?
-The video suggests solving the problem by taking a sequence of decisions at each stage, starting with selecting a middle vertex and checking for shorter paths through that vertex, iteratively updating the distance matrix for each intermediate vertex.
What is the role of the distance matrix in solving the All Pairs Shortest Paths problem?
-The distance matrix plays a crucial role in solving the problem by storing the shortest path distances between vertices. It is iteratively updated with the shortest paths found using dynamic programming.
How does the video explain the process of updating the distance matrix?
-The video explains the process by taking the minimum of the direct path and the path that includes an intermediate vertex, updating the matrix values accordingly to reflect the shortest paths found.
What is the formula used in the video to update the distance matrix for an intermediate vertex?
-The formula used to update the distance matrix for an intermediate vertex is min(a[i][j], a[i][k] + a[k][j]), where 'a' represents the distance matrix, 'i' and 'j' are the vertices, and 'k' is the intermediate vertex.
How many matrices are generated in total for the dynamic programming approach shown in the video?
-In the video, a total of n-1 matrices are generated, where n is the number of vertices in the graph, with each matrix representing the shortest paths considering an additional intermediate vertex.
What is the time complexity of the dynamic programming approach for the All Pairs Shortest Paths problem as described in the video?
-The time complexity of the dynamic programming approach described in the video is O(n^3), due to the three nested loops required to generate all the matrices.
Can you provide an example of how the video demonstrates the application of the dynamic programming approach?
-The video demonstrates the approach by first showing the working with a small example, then deriving the formula used for updating the distance matrix, and finally presenting the code that implements the algorithm with a time complexity of O(n^3).
Outlines
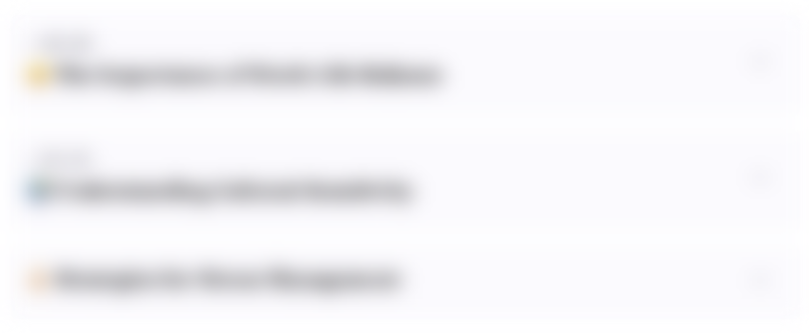
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
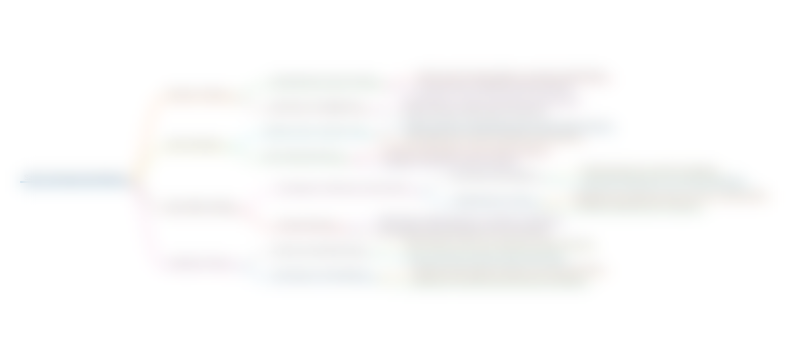
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
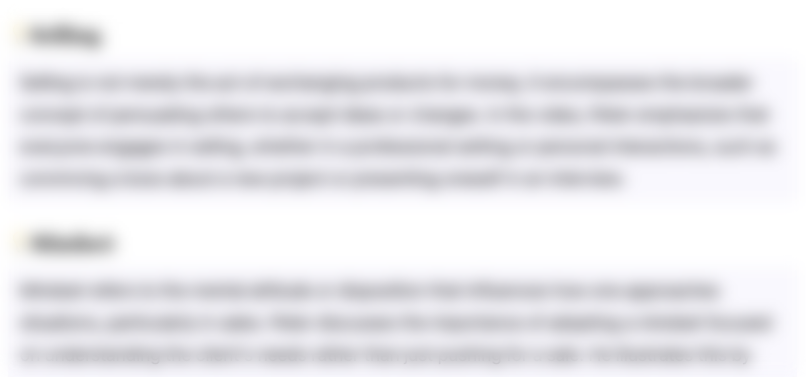
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
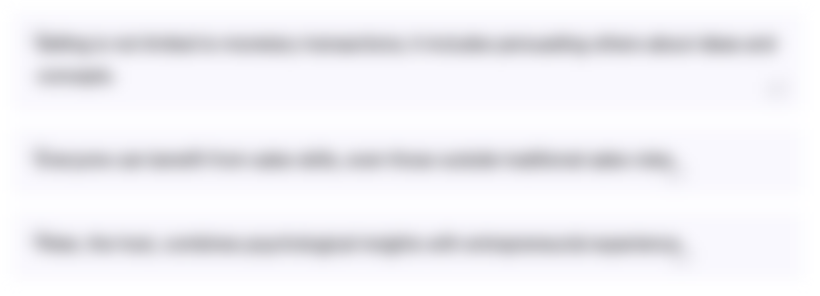
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
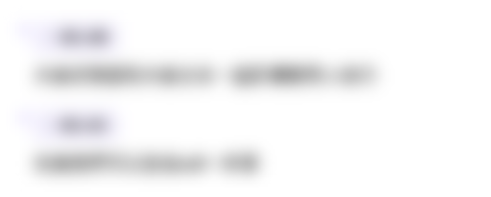
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示

Pemrograman Dinamis: Masalah Stage Coach

Projeto e Análise de Algoritmos - Aula 13 - Problema do caminho mais curto
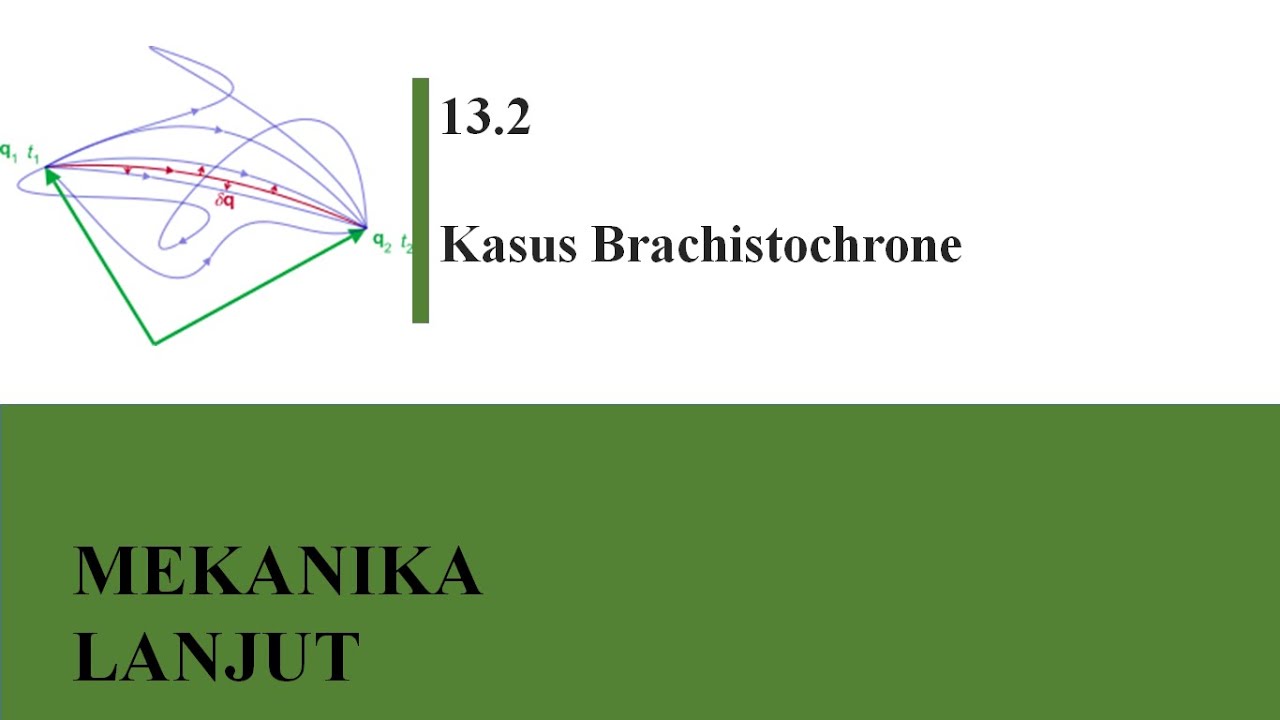
Mekanika Lanjut 13.2 Kasus Brachistochrone Aplikasi Identitas Beltrami
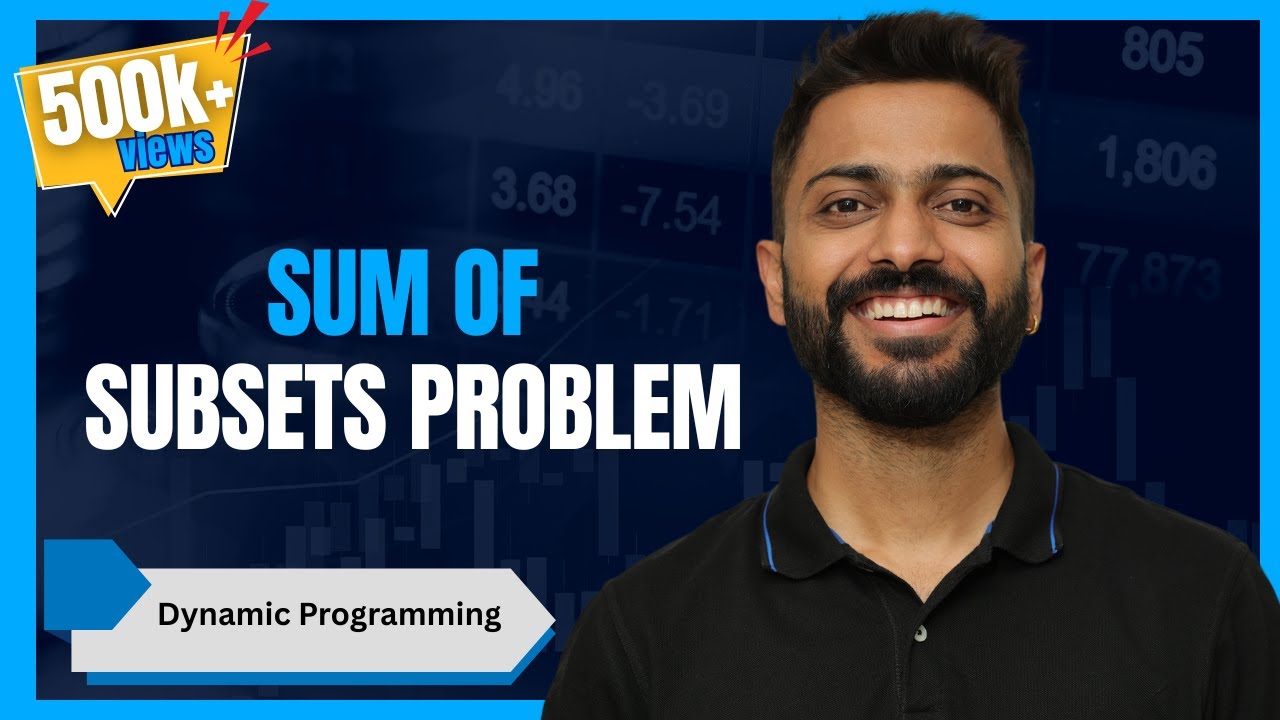
L-5.5: Sum of Subsets Problem | Dynamic Programming
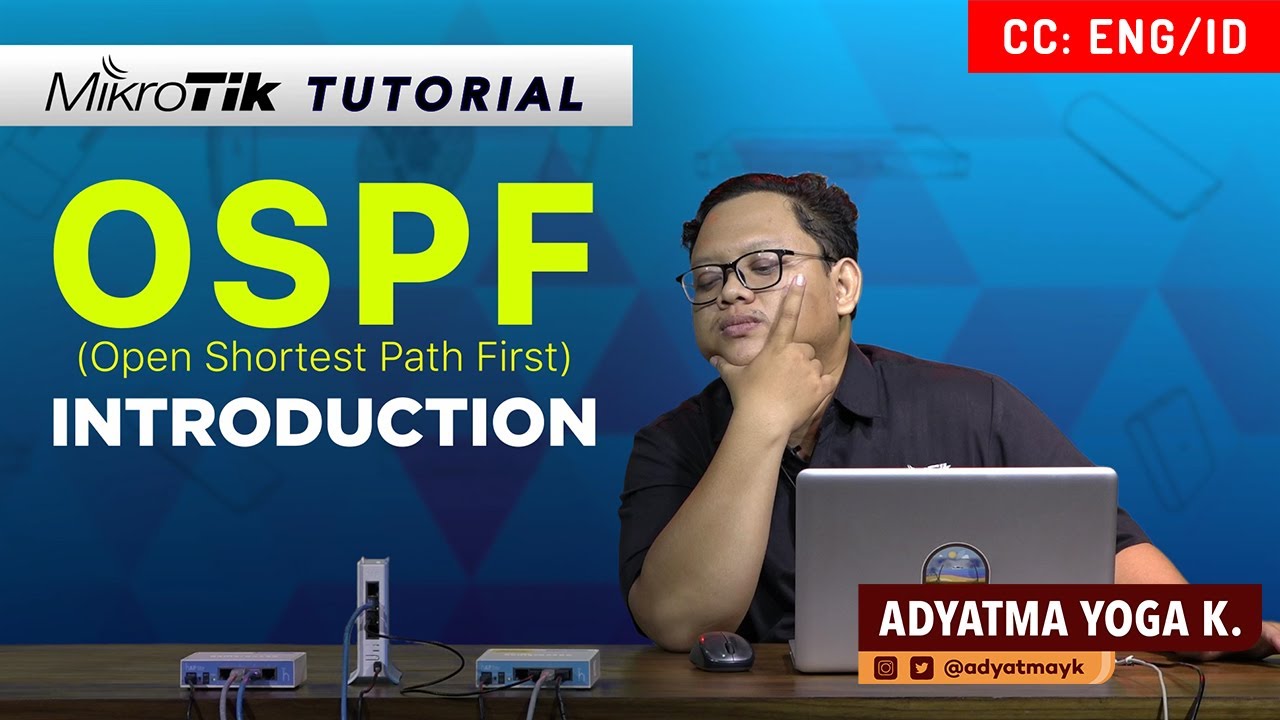
OSPF Introduction - MIKROTIK TUTORIAL [ENG SUB]
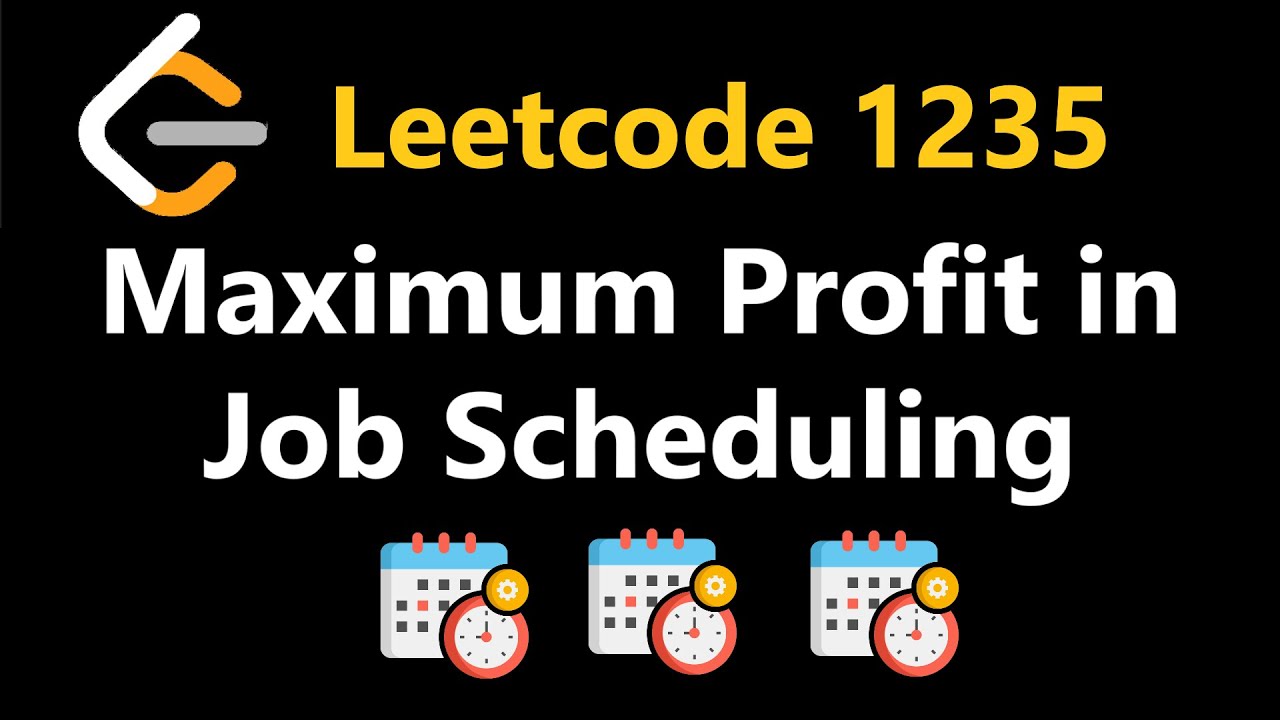
Maximum Profit in Job Scheduling - Leetcode 1235 - Python
5.0 / 5 (0 votes)