【Python入門 #7】関数 | 処理を使いまわそう
Summary
TLDRThe video script is a comprehensive tutorial on the concept and application of functions in Python programming. It begins with an introduction to functions as a fundamental and essential part of programming, emphasizing their necessity for writing efficient and practical code. The script uses a quiz format to engage viewers, asking them to calculate the BMI (Body Mass Index) of different individuals using their weight and height. The presenter shares personal anecdotes about his own weight fluctuations and the impact on his physical fitness. The core of the tutorial focuses on defining functions in Python, explaining how to name a function, pass arguments, and return a value. It demonstrates the use of functions with a step-by-step guide on calculating BMI, highlighting the benefits of using functions, such as code reusability and readability. The script also touches on the concept of local and global variables, advising the use of local variables for safety and global variables sparingly. The tutorial concludes with an exercise for viewers to define and use a function to calculate the area of rectangles with given dimensions, reinforcing the practical use of functions in Python.
Takeaways
- 📚 **Understanding Functions**: Functions in Python are fundamental for practical programming, allowing you to reuse code and avoid repetition.
- 🏋️ **Functions Simplified**: Despite initial perceptions, functions are not as difficult as they seem. They are essentially simple structures that perform a set of tasks.
- 🤔 **Quiz Interaction**: The video uses a quiz about calculating BMI to illustrate the necessity and practical use of functions, making the concept more relatable.
- 🍲 **Real-life Analogy**: The presenter uses the analogy of cooking to explain the concept of functions, likening them to a recipe that takes ingredients (arguments) and produces a dish (return value).
- 📊 **BMI Calculation Example**: The script provides a detailed example of calculating BMI using functions, demonstrating how to define, use, and call functions with arguments.
- 💡 **Function Definition**: Functions are defined using the `def` keyword, followed by the function name and arguments, with the body of the function indented afterward.
- 🔁 **Reusability**: One of the key advantages of functions is their reusability, allowing you to call the same function multiple times without rewriting the code.
- 🧩 **Local vs. Global Variables**: The script distinguishes between local variables, which are only accessible within the function, and global variables, which can be accessed from anywhere in the program.
- 🚧 **Best Practices**: It is recommended to use local variables as much as possible for safety and to minimize the risk of bugs, reserving global variables for when necessary.
- 📈 **Progression to Advanced Topics**: After covering the basics, the script moves on to more advanced topics, such as using functions to calculate the total price of groceries in a shopping cart scenario.
- 🔍 **Debugging and Maintenance**: Using local variables makes code easier to debug and maintain because their scope is limited, reducing the chances of unintended side effects.
Q & A
What is the main theme of the video script?
-The main theme of the video script is the introduction and explanation of functions in Python programming.
Why are functions considered essential in programming?
-Functions are essential in programming because they allow for the reuse of code, making it more efficient and easier to manage, especially in longer programs.
What is the BMI calculation based on?
-The BMI (Body Mass Index) calculation is based on a person's weight in kilograms divided by the square of their height in meters.
What is the normal range for BMI according to the script?
-According to the script, a BMI between 18.5 and 25 is considered to be within the normal range.
How does the speaker describe the structure of a function in Python?
-The speaker describes the structure of a function in Python as simple, where you define a function with a name, specify the parameters it accepts, write the processing code inside the function, and return a value using the 'return' statement.
What is the purpose of using a black box analogy for functions?
-The black box analogy is used to simplify the concept of functions, illustrating that you input values (arguments) into the function and it processes them to produce an output (return value), without needing to know the internal workings.
Why is it beneficial to use functions to perform repetitive tasks in code?
-Using functions to perform repetitive tasks is beneficial because it reduces the amount of code you need to write, minimizes the chance of errors, and makes the code more readable and maintainable.
What is the concept of local and global variables as mentioned in the script?
-Local variables are those that are defined within a function and can only be used inside that function. Global variables, on the other hand, can be accessed and modified from anywhere in the program.
Why should global variables be used with caution?
-Global variables should be used with caution because they can be accessed and modified from anywhere in the program, which can lead to unexpected behavior and make the code harder to debug.
How does defining functions help in making the code more readable?
-Defining functions with meaningful names helps in making the code more readable because it provides a clear indication of what the code block is intended to do, without having to read through the entire code.
What is the speaker's personal experience with weight and body mass index (BMI) mentioned in the script?
-The speaker shares their personal experience of having a weight that fluctuated between 54 to 56 kilograms, with a peak of 65 kilograms during their university days when they were part of the rowing club. They also discuss their strategy for losing weight, which involved running and reducing dinner portions.
How does the script demonstrate the practical use of functions with a quiz question?
-The script demonstrates the practical use of functions by creating a function to calculate the BMI for different individuals, thus avoiding the repetition of the same calculation code and making the script more efficient and easier to understand.
Outlines
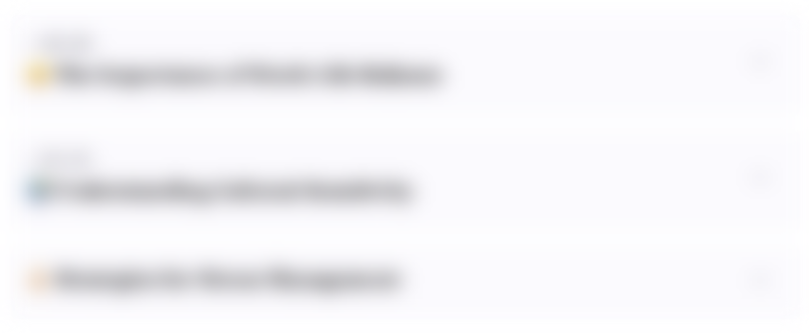
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
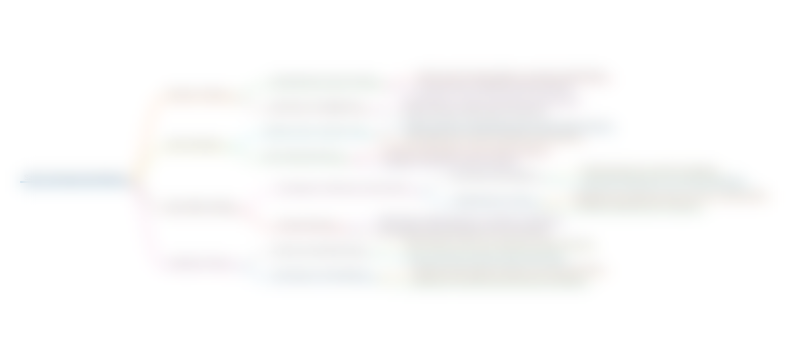
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
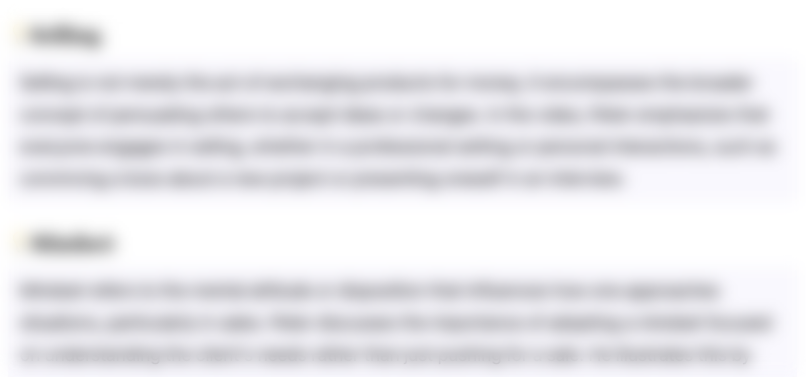
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
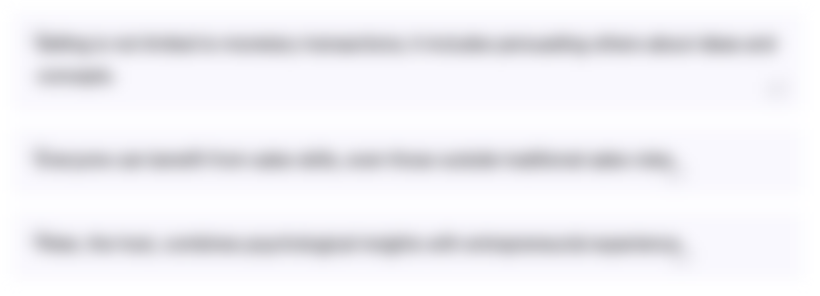
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
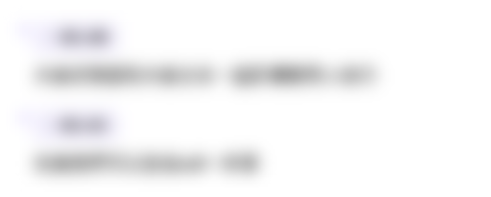
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenant5.0 / 5 (0 votes)