Lab Exp07:: Prevention of Race condition using mutex in C programming in multithreaded process
Summary
TLDRThe video script discusses the issue of race conditions in multi-threaded programming, demonstrating how a shared variable can be incorrectly incremented due to improper context switching. It then shows how to resolve this using a mutex, a synchronization mechanism that ensures mutual exclusion, thus preventing race conditions by allowing only one thread to access a shared resource at a time. The script concludes with a demonstration that, with mutex in place, the final value of the shared variable consistently reaches the expected two million, regardless of thread execution order.
Takeaways
- đ The script discusses the problem of race conditions in multi-threaded programming.
- đ The issue arises when two threads try to increment a shared variable, expecting a final value of two million but getting inconsistent results.
- đ·ââïž The script demonstrates how to resolve race conditions using mutexes, which provide mutual exclusion for shared resources.
- đš Mutexes are declared and initialized using specific pthread functions to ensure safe access to shared data.
- đâđ Before accessing the shared variable, threads must lock the mutex with `pthread_mutex_lock`.
- đ After updating the shared variable, threads must unlock the mutex with `pthread_mutex_unlock`.
- đ ïž The script shows the implementation of mutex locking within the threads' increment loop to prevent race conditions.
- đ The use of mutex ensures that only one thread can update the shared variable at a time, blocking others until the lock is released.
- đĄ The script illustrates that with mutex locking, the final value of the shared variable consistently reaches the expected two million.
- đ The script mentions that the order of thread execution may vary, but the use of mutex ensures a consistent outcome.
- đ The script concludes by stating that mutexes and semaphores can effectively address race conditions, with a follow-up session planned to discuss semaphores.
Q & A
What is the primary issue discussed in the video script?
-The primary issue discussed in the video script is the race condition in multi-threaded programming, where two or more threads access a shared variable simultaneously and lead to unpredictable results.
What was the expected outcome if the shared variable was incremented correctly by two threads one million times each?
-The expected outcome would be that the final value of the shared variable should be two million, as each thread increments the value one million times.
What is the actual result observed when the race condition occurs?
-When the race condition occurs, the final value of the shared variable is not consistent and varies with each execution, deviating from the expected two million.
How is a mutex used to solve the race condition problem?
-A mutex (mutual exclusion) is used to ensure that only one thread can access a shared resource at a time. It is used to lock and unlock access to the shared variable, preventing simultaneous access and thus avoiding the race condition.
What function is used to declare a mutex variable in the script?
-The function used to declare a mutex variable in the script is pthread_mutex_t.
What function is used to initialize a mutex variable?
-The function used to initialize a mutex variable is pthread_mutex_init, which takes the address of the mutex variable and an optional attributes argument.
What are the two functions used to control access to the shared variable using a mutex?
-The two functions used to control access to the shared variable using a mutex are pthread_mutex_lock and pthread_mutex_unlock.
What happens when a thread calls pthread_mutex_lock?
-When a thread calls pthread_mutex_lock, it attempts to acquire the lock on the mutex. If the lock is already held by another thread, the calling thread will be blocked until the lock becomes available.
How does using a mutex ensure the correct final value of the shared variable?
-Using a mutex ensures that only one thread can update the shared variable at a time, preventing simultaneous updates that could lead to incorrect values. This sequential execution guarantees that the final value will be the expected two million.
What is the next topic the speaker mentions will be discussed in the next session?
-The next topic the speaker mentions will be discussed in the next session is semaphores, which are another synchronization mechanism to handle similar issues as mutexes.
Outlines
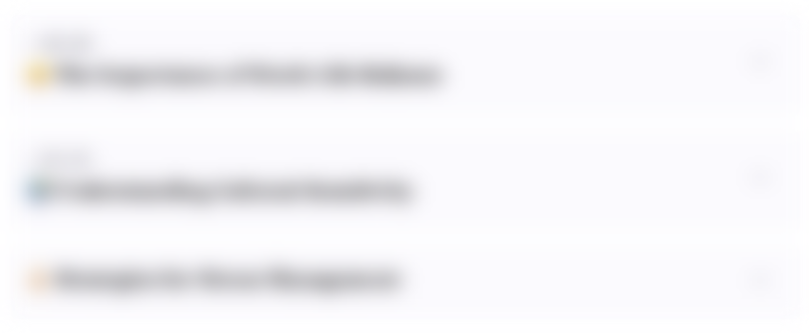
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
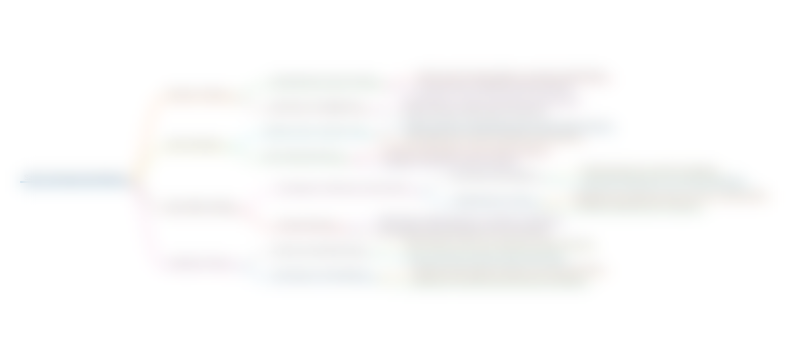
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
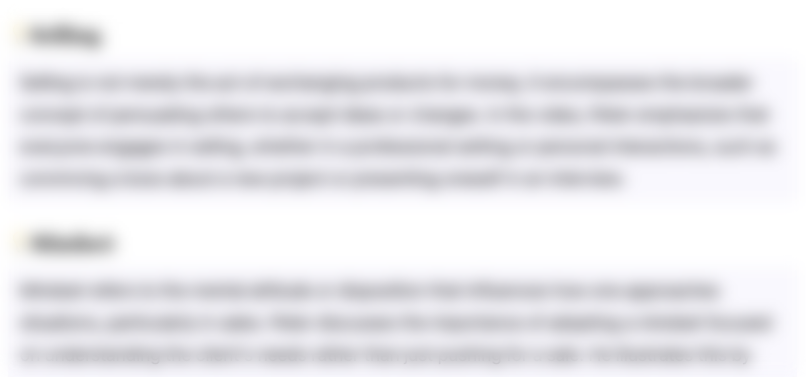
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
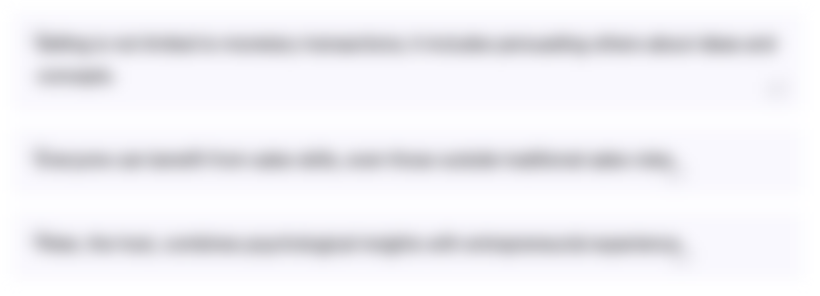
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
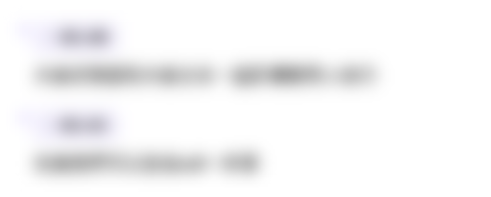
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
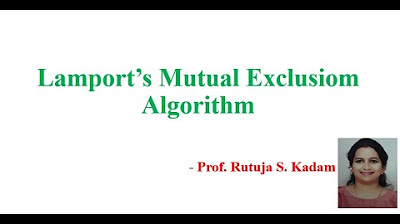
Lamport's Mutual Exclusion Distributed Systems Synchronization

How JavaScript Works đ„& Execution Context | Namaste JavaScript Ep.1

L-3.2: Producer Consumer Problem | Process Synchronization Problem in Operating System

L-3.4: Critical Section Problem | Mutual Exclusion, Progress and Bounded Waiting | Operating System

The Readers Writers Problem
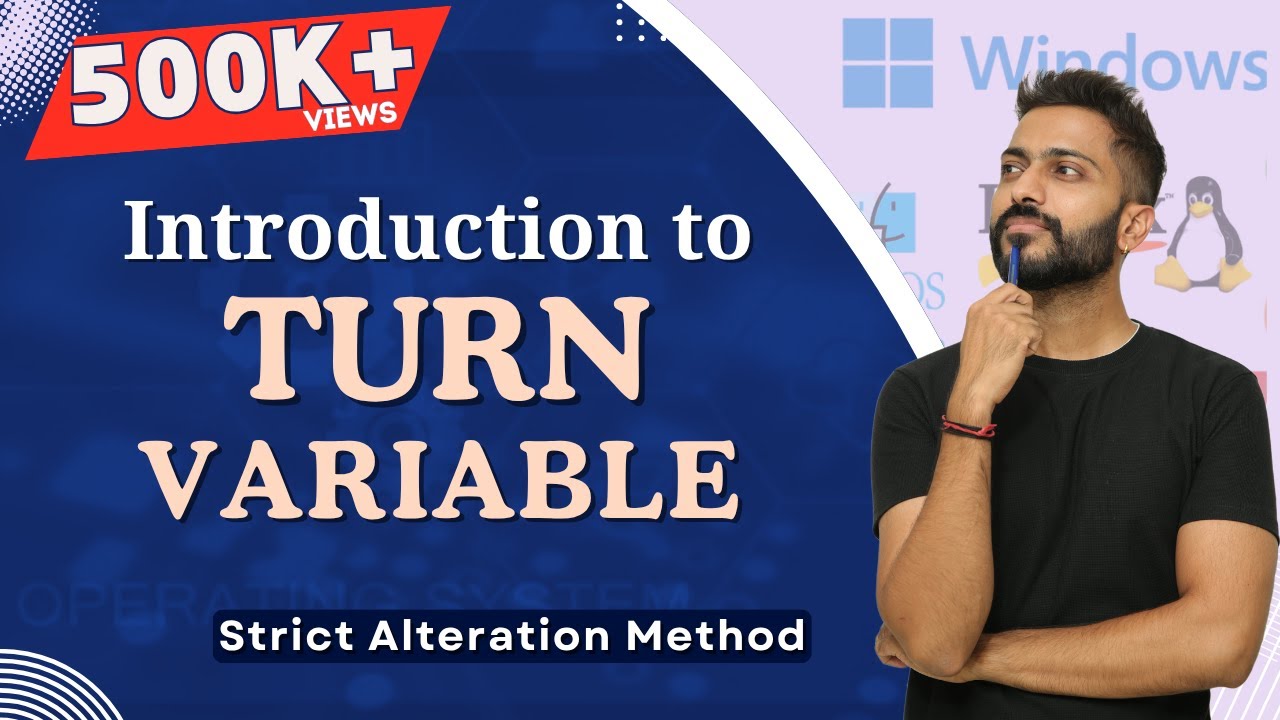
L-3.7: Turn Variable | Strict Alteration Method | Process Synchronization
5.0 / 5 (0 votes)