String Builder | Java Placement Course Lecture 13
Summary
TLDRThis video script discusses the intricacies of Java's StringBuilder class, emphasizing its efficiency over the traditional StringBuffer. The tutorial covers the need for StringBuilder due to the inefficiency of memory operations within StringBuffer, particularly when dealing with string modifications. It illustrates how StringBuilder reduces memory usage and processing time through direct memory manipulation, bypassing the need to create new strings. The script also provides practical examples, demonstrating how to use StringBuilder for tasks like string concatenation, character insertion, and deletion, all while optimizing performance in Java applications.
Takeaways
- 😀 The video discusses the concept of 'String Builder' in Java, which is used to optimize operations involving string manipulation.
- 👨🏫 The traditional approach of using 'String' in Java for operations like addition and deletion can be memory-intensive and time-consuming.
- ⏰ The video explains that 'StringBuilder' is introduced to overcome the inefficiencies of the 'String' class, especially in scenarios where frequent modifications are needed.
- 🛠️ 'StringBuilder' allows for mutable sequences of characters, which is more efficient in terms of performance and memory usage compared to immutable 'String' objects.
- 🔍 The script highlights the technical details of how 'StringBuilder' handles operations internally, emphasizing its role in reducing the time complexity of string manipulations.
- 💡 The video provides a practical demonstration of using 'StringBuilder' to create, modify, and delete strings, showcasing its utility in real-world programming scenarios.
- 📝 The script includes a discussion on the limitations of Java's 'String' class, such as the inability to change the content of a string once it's created.
- 🔧 The video script walks through the process of declaring and using 'StringBuilder', including how to append, insert, and delete characters within a string.
- 📈 It emphasizes the importance of understanding the performance implications of using 'String' versus 'StringBuilder', particularly in large-scale or performance-critical applications.
- 🎓 The educational content is aimed at enhancing the viewer's understanding of Java's handling of strings and the benefits of using 'StringBuilder' for optimized string operations.
Q & A
What is the main topic discussed in the video script?
-The main topic discussed in the video script is about the 'String Builder' in Java, its usage, and how it can optimize operations involving strings within the Java framework.
Why is the String Builder class necessary according to the script?
-The String Builder class is necessary because it helps in optimizing the time taken by operations that involve modifications such as add, delete, and modify within strings, which are computationally expensive in Java's standard string handling.
What problem does the String Builder class aim to solve?
-The String Builder class aims to solve the problem of time-consuming operations on strings in Java, which can lead to poor user experience in applications due to increased processing time.
How does the String Builder class differ from normal string operations in Java?
-The String Builder class differs from normal string operations in Java by allowing modifications without creating new string objects each time, thus reducing memory usage and improving performance.
What is the process of creating a new String in Java as described in the script?
-The process of creating a new String in Java involves initializing a string with certain characters and then adding or modifying characters using operations like 'plus', 'append', and others, which may lead to increased memory usage and time consumption.
Why might using the standard string operations in Java lead to performance issues?
-Using standard string operations in Java can lead to performance issues because each modification operation creates a new string object, which is stored in memory, leading to increased memory usage and slower execution times, especially in large-scale applications.
What is the significance of the 'modify' operation in the context of the String Builder class?
-The 'modify' operation in the context of the String Builder class is significant because it allows for efficient changes to be made to the string without the overhead of creating new string objects, thus improving the overall performance of the application.
How does the script describe the memory management when using String Builder?
-The script describes that using the String Builder helps in managing memory more efficiently by reducing the need to create new string objects for each modification, thus optimizing both memory usage and processing time.
What is the role of the 'append' method in the String Builder class as per the script?
-The 'append' method in the String Builder class is used to add new characters or strings to the existing string without creating a new string object, which is more efficient than standard string concatenation in Java.
How does the script suggest optimizing string operations in Java applications?
-The script suggests optimizing string operations in Java applications by using the String Builder class, which provides methods like 'append', 'insert', 'delete', and others that allow for modifications without the high time and memory costs associated with standard string operations.
What is the time complexity implication of using the String Builder class compared to standard strings?
-The time complexity implication of using the String Builder class is that it reduces the time taken for string modifications, as opposed to standard strings in Java where each operation can lead to the creation of a new string object, thus increasing time complexity.
Outlines
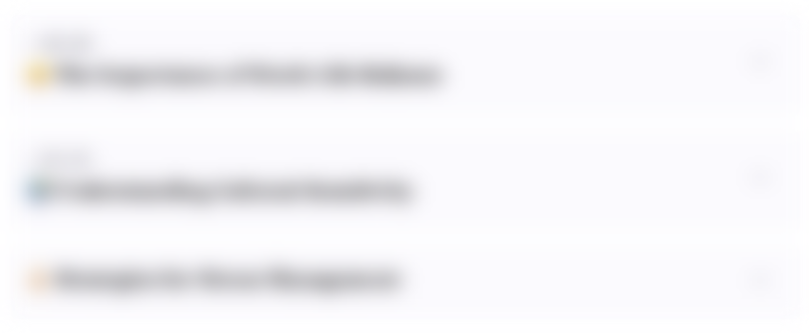
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
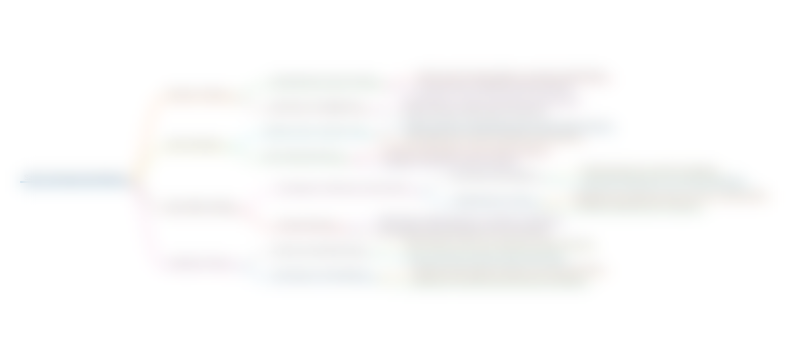
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
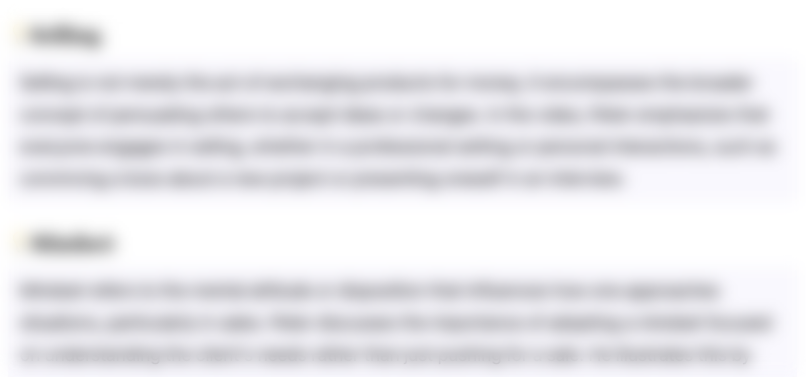
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
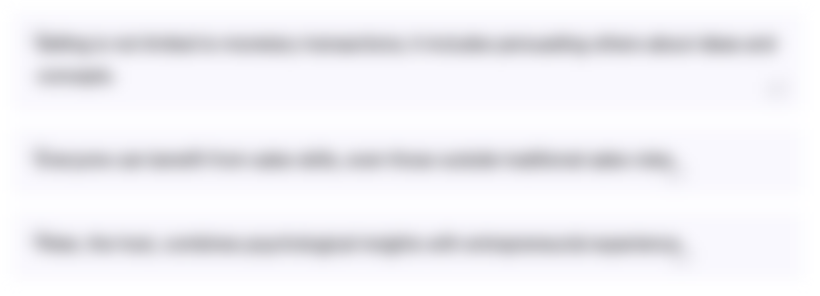
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
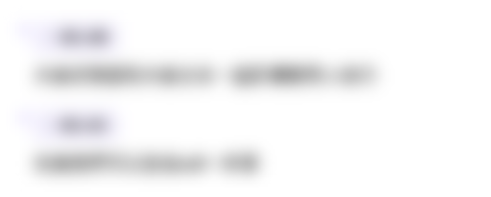
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenant5.0 / 5 (0 votes)