Flutter Crash Course #6 - Widgets
Summary
TLDRThis Flutter tutorial introduces the fundamental concept of widgets, which are the building blocks of any Flutter application. It explains that everything in Flutter is a widget, with the root widget being the starting point passed to the runApp function. The script delves into the distinction between stateless and stateful widgets, the importance of the build method in defining widget behavior, and the structure of a widget tree. It also touches on the use of pre-built widgets for UI elements and the creation of custom widgets for specific functionalities, like the example of a 'Footer' widget. The lesson aims to clarify the basics of widget-based UI development in Flutter.
Takeaways
- 📚 Flutter apps are primarily built using widgets, which form a widget tree.
- 🌟 The root widget is the main entry point of the application, passed into the runApp function.
- 🔄 Widgets can be either stateless or stateful, with stateless widgets not containing any changing state or data.
- 🏗️ The 'build' method in a widget class is responsible for constructing and returning the widget or widget tree.
- 🎨 Flutter provides a wide range of pre-built UI widgets for common elements like text, images, and layout features.
- 🛠️ Developers can create custom widgets by defining a class with a build method that returns a widget tree.
- 📦 Custom widgets allow for reusability, similar to components in React, and can be inserted into any widget tree.
- 📝 The 'Material App' widget is a built-in widget used for building the app's structure, which will be explored in more detail in subsequent lessons.
- 🔑 Understanding the concept of widgets is fundamental to developing in Flutter, as most UI elements are represented as widgets.
- 🔍 The script provides an example of a 'My App' widget and a 'Footer' widget to illustrate the creation and use of custom widgets.
- 🚀 The lesson emphasizes the importance of recognizing that widgets in Flutter are essentially classes that can be instantiated as needed.
Q & A
What is the fundamental concept discussed in the Flutter lesson?
-The fundamental concept discussed in the Flutter lesson is the idea of widgets, which are the building blocks for most things in Flutter.
What is the root widget in a Flutter application?
-The root widget is the top-level widget in a Flutter application that is passed into the runApp function. It is the starting point of the widget tree.
What does the term 'stateless' in 'StatelessWidget' signify in Flutter?
-The term 'stateless' in 'StatelessWidget' signifies that the widget does not contain any state or data that changes over time or in response to user interactions.
What is the purpose of the 'build' method in a Flutter widget class?
-The 'build' method in a Flutter widget class is responsible for constructing the widget and defining what is displayed on the screen. It must return a widget or a widget tree.
What is a widget tree in the context of Flutter?
-A widget tree in Flutter is a hierarchical structure where widgets are nested within each other, forming the user interface of the application.
How are built-in widgets different from custom widgets in Flutter?
-Built-in widgets are pre-made widgets provided by the Flutter framework for common UI elements, while custom widgets are created by developers using a class with a build method that returns a widget tree for specific needs.
Why might a developer create a custom widget in Flutter?
-A developer might create a custom widget in Flutter to encapsulate a specific UI pattern or component that can be reused across the application, providing consistency and reducing code duplication.
Can you provide an example of a built-in Flutter widget mentioned in the script?
-Examples of built-in Flutter widgets mentioned in the script include the Text widget for displaying text, the Image widget for displaying images, and the Container widget for layout purposes.
What is the relationship between the 'MyApp' widget and the 'MaterialApp' widget in the script?
-In the script, the 'MyApp' widget is a custom widget that extends 'StatelessWidget' and its build method returns a 'MaterialApp' widget, which is a built-in Flutter widget used for the application's material design scaffolding.
How does the script relate the concept of widgets to a previous lesson?
-The script relates the concept of widgets to a previous lesson by mentioning that the argument passed into the 'runApp' function, which is the 'MyApp' widget, was discussed as the root widget in the previous lesson.
What is the significance of the 'Column' and 'Row' widgets in the script's explanation of widget trees?
-The 'Column' and 'Row' widgets are used in the script to demonstrate how widgets can be nested within each other to create a vertical or horizontal layout, contributing to the overall structure of the widget tree.
Outlines
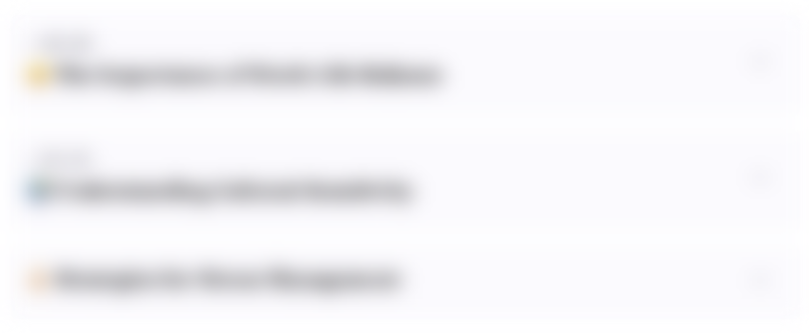
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
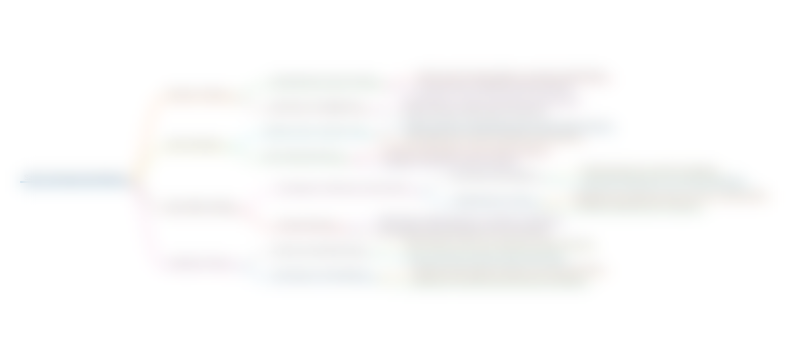
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
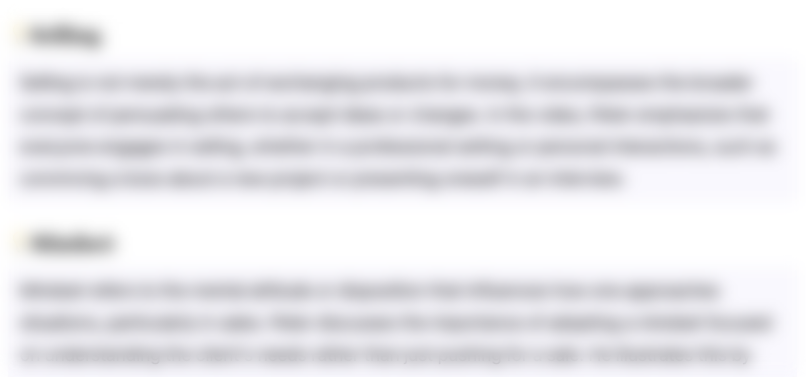
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
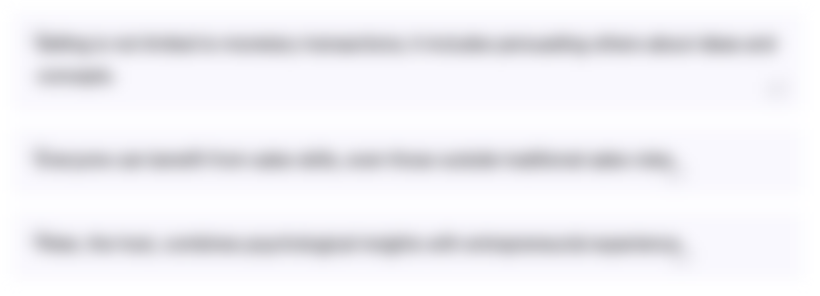
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
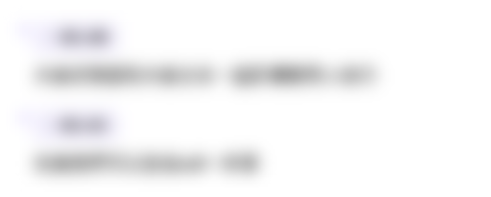
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
5.0 / 5 (0 votes)