25 Nooby Pandas Coding Mistakes You Should NEVER make.
Summary
TLDRThis video educates new pandas users on 25 common mistakes to avoid for efficient data manipulation. It covers issues like unnecessary CSV indexing, using spaces in column names, underutilizing the query method, and misusing in-place operations. The script emphasizes the importance of leveraging vectorization, proper data type settings, and pandas' built-in functions for tasks like plotting and string manipulation. It also advises against practices like creating duplicate columns and encourages the use of categorical data types for efficiency.
Takeaways
- 📝 Avoid writing to CSV with an unnecessary index; set `index=False` when saving or set an index column when reading in.
- 🔑 Use underscores instead of spaces in column names for easier access and querying.
- 🔍 Leverage the `query` method for powerful data frame filtering instead of basic syntax.
- 📝 Use the `@` symbol to incorporate external variables in pandas queries without manually formulating strings.
- ❌ Avoid using `inplace=True` as it may be deprecated; explicitly overwrite data frames with modifications instead.
- 🔁 Prefer vectorized functions over iterating over rows for performance and cleaner code.
- 📉 Use vectorized operations instead of `apply` when possible for efficiency.
- 📚 Treat slices as views, not copies; use the `copy` method to ensure independence from the original data frame.
- 🔄 Encourage chaining commands for transformations to avoid creating multiple intermediate data frames.
- 📅 Set column data types properly, especially for dates, to ensure correct parsing and usage.
- 👍 Use boolean values instead of strings for conditions and comparisons; map strings to booleans if necessary.
- 📈 Utilize pandas' built-in plotting methods for quick and easy data visualization.
- 🔠 Apply string methods directly to entire columns using `.str` method for consistency and simplicity.
- 🔄 Avoid repeating data transformations; write a function for the pipeline and apply it to each data frame.
- 🔄 Use the `rename` method with a dictionary for a cleaner way to rename columns.
- 👥 Use `groupby` for aggregations based on groupings in the data frame instead of manual filtering and calculation.
- 🔢 Calculate changes like percent difference using built-in pandas methods like `pct_change` and `diff`.
- 📊 Consider saving large data sets in formats like parquet, feather, or pickle for efficiency and space.
- 🖥️ Explore the `style` attribute of pandas data frames for extensive HTML formatting capabilities.
- 🔗 Explicitly set suffixes when merging data frames to avoid confusion with default suffixes.
- 🔄 Use the `validate` parameter in pandas merge to automatically check for correct merge types.
- 📏 Break down long chained commands into readable lines for better code maintenance.
- 📊 Convert columns with few unique values to categorical data types for memory efficiency and speed.
- 🔍 Check for and remove duplicate columns when concatenating data frames to avoid confusion.
Q & A
Why is writing to a CSV with an unnecessary index considered a mistake in pandas?
-Writing to a CSV with an unnecessary index is a mistake because by default, pandas includes the index without a column header name when writing to CSV, which can be redundant if the index contains no valuable information. This can lead to confusion when the CSV is read back in with an unnamed zero column included.
What is the recommended way to handle column names with spaces in pandas?
-It is recommended to use underscores in place of spaces for column names in pandas. This allows for easier access using dot syntax and simplifies querying of the columns.
Why is the query method in pandas preferred over manually constructing query strings?
-The query method is preferred because it allows for writing powerful queries in a more concise and readable manner. It is especially helpful for complex query criteria and avoids the need for manual string concatenation or f-strings.
How can external variables be used in pandas queries without manually constructing the query strings?
-External variables can be used in pandas queries by simply prefixing the variable name with the '@' symbol. This allows pandas to access the variable directly within the query.
What is the general consensus on using the 'in place' parameter in pandas?
-Using the 'in place' parameter is generally frowned upon in the pandas community, and the core developers even plan to remove this functionality altogether. It is better to explicitly overwrite the data frame with the modifications.
Why should iterating over rows in a data frame be avoided when vectorization is an option?
-Iterating over rows should be avoided in favor of vectorization because vectorized operations are not only cleaner and more readable but also typically faster, especially for large data sets.
What is the main advantage of using the apply method over iterating over rows in a data frame?
-The apply method allows you to run any function across an axis of your data frame, which is usually more efficient than iterating over each row. However, when vectorization is possible, it is still the preferred approach for performance reasons.
Why should a slice of a data frame not be treated as a new data frame?
-A slice of a data frame should not be treated as a new data frame because modifications to the slice will result in a 'SettingWithCopyWarning', indicating that changes are being made to the original data frame. To avoid this, it's best to use the copy method to create a true copy of the data frame slice.
What is the recommended approach when making multiple transformations to a data frame?
-It is recommended to use chaining commands where all transformations are applied in a single sequence rather than creating multiple intermediate data frames. This approach is more efficient and results in cleaner code.
Why is it important to properly set column data types in pandas?
-Properly setting column data types is important because it ensures that the data is stored and processed in the most efficient way. Incorrect data types can lead to performance issues and may cause errors in data processing and analysis.
How can boolean values be represented in pandas instead of using string values?
-Boolean values should be represented using actual boolean types (True or False) rather than string values like 'yes' or 'no'. This can be achieved by casting the string values to booleans when creating a new column or by mapping string values to booleans if they already exist in the data set.
What are some benefits of using pandas built-in plotting methods over manual plotting?
-Pandas built-in plotting methods provide a quick and easy way to visualize data directly from a data frame. They are more convenient and often result in better-formatted plots compared to manually setting up a plot using matplotlib or other plotting libraries.
Why is it recommended to use the string method 'upper' on the entire array instead of applying it to each string individually?
-Using the string method 'upper' on the entire array is recommended because it is more efficient and results in cleaner code. It applies the method to all elements in the column without the need for explicit iteration or looping.
What is the best practice for avoiding repeated code when creating data pipelines in pandas?
-The best practice is to write a function for the data pipeline that can be applied to each data frame. This ensures consistent processing and makes the code easier to read and maintain.
Why is it more efficient to use the group by method for aggregations rather than looping over rows?
-The group by method is more efficient because it allows for aggregations to be performed on groups independently in a single operation, eliminating the need for explicit looping and reducing the potential for errors.
What are some advantages of using the percent change and diff methods in pandas over manual calculations?
-The percent change and diff methods in pandas provide built-in functionality for calculating changes in a series, which is more efficient and less error-prone than manual calculations. They also integrate seamlessly with pandas data structures.
Why might saving large data sets as CSVs be inefficient, and what are some alternative file formats?
-Saving large data sets as CSVs can be inefficient due to slow write speeds and large disk space usage. Alternative file formats such as parquet, feather, and pickle files are more efficient, retain data types, and can be faster for both reading and writing.
How can conditional formatting be achieved in pandas data frames without reverting to Excel?
-Conditional formatting can be achieved in pandas data frames using the style attribute, which allows for extensive formatting options when the data frame is displayed as HTML. This provides a powerful alternative to Excel for data presentation.
What is the purpose of setting suffixes when merging two data frames in pandas?
-Setting suffixes when merging two data frames helps to differentiate columns that appear in both data frames but are not used for merging. This avoids confusion and makes it easier to track the origins of the columns in subsequent data processing.
How can the validate parameter in pandas merge help ensure the integrity of a one-to-one match?
-The validate parameter in pandas merge automatically checks for different merge types and throws a merge error if the validation fails, ensuring that the merge is a one-to-one match and maintaining data integrity.
Why is it important to avoid stacking chained commands into one line of code in pandas?
-Avoiding stacking chained commands into one line of code improves readability. By wrapping expressions in parentheses and splitting the code so that each line has one component of the expression, the code becomes easier to understand and maintain.
What are the benefits of using categorical data types in pandas for columns with a limited number of unique values?
-Using categorical data types for columns with a limited number of unique values reduces memory usage and can significantly speed up operations on large data sets, as categorical data types are more memory-efficient and optimized for such cases.
How can duplicate columns be identified and removed when concatenating data frames in pandas?
-Duplicate columns can be identified and removed by using the 'duplicated' function in pandas, which checks for duplicated columns and can be used to filter them out, ensuring a clean and error-free data frame.
Outlines
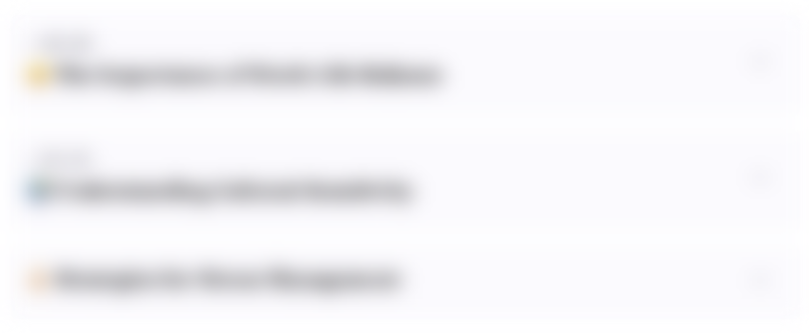
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
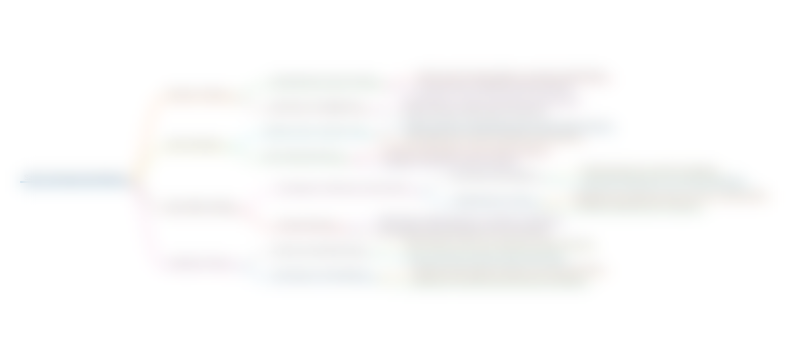
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
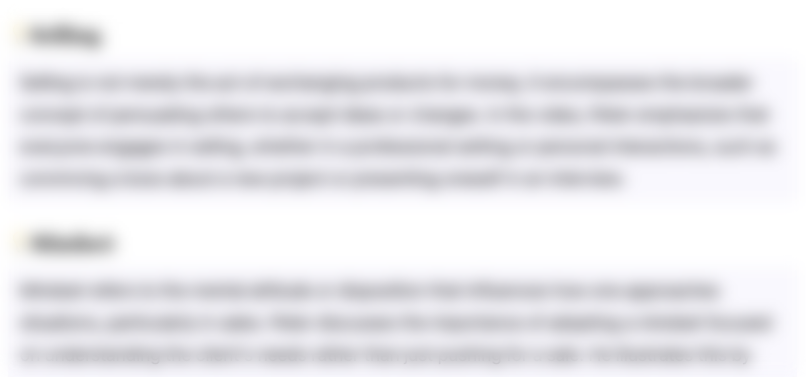
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
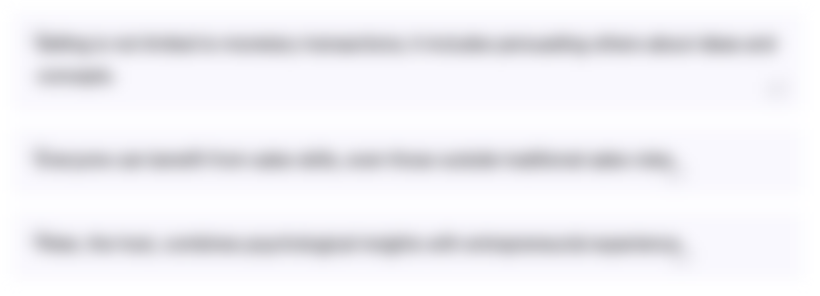
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
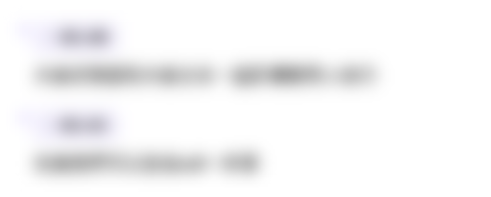
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
5.0 / 5 (0 votes)