Karel Can't Turn Right - Python
Summary
TLDRThis video tutorial introduces the concept of functions in programming, specifically for a character named Carol in a 'make a tower' exercise. It explains that Carol can't turn right with her basic commands, but the issue can be resolved by using functions to teach her new commands. The video demonstrates how to define a function in Python to make Carol turn right by turning left three times, emphasizing the importance of defining functions before calling them. It also shows how to implement the new 'turn right' function within the program to control Carol's movements effectively.
Takeaways
- 🤖 Carol, the robot, cannot turn right using the original four commands.
- 🔄 As a workaround, Carol can turn left three times to effectively face the same direction as if it had turned right.
- 📚 Introduction of functions as a way to teach Carol new commands beyond the initial set.
- 🔑 The term 'function' may also be known as 'procedure', 'task', or 'process', but in this context, it is primarily referred to as a function.
- 👨🏫 A function in Python is defined using 'def' (short for define), followed by the function name, parentheses, and a colon.
- 🔧 Indentation in Python is used to delineate the body of a function from the rest of the code.
- 🛑 Functions must be defined before they are called in a Python program; the program reads from top to bottom.
- 🔄 Demonstration of teaching Carol to turn right by defining a function that contains the sequence of 'turn left' commands.
- 📝 The function body includes the specific commands that make up the new 'turn right' command for Carol.
- 🔑 Calling the function 'turn right' in the code will execute the defined sequence of commands, enabling Carol to turn right.
- 🎓 Encouragement for the viewer to practice and experiment with functions in the programming editor.
Q & A
Why can't Carol turn right in the script?
-Carol can't turn right because she only knows the original four commands and 'turn right' is not one of them.
What is the alternative method to make Carol face East if she can't turn right?
-The alternative method is to make Carol turn left three times, which results in her facing East.
What is a function in the context of the script?
-A function is a way to teach Carol new commands or words, breaking away from the original four commands she knew.
What is the purpose of teaching Carol new commands with functions?
-The purpose is to expand Carol's capabilities beyond the original commands, allowing her to perform more complex tasks.
What does 'def' stand for in the script?
-'def' stands for 'define', which is used to start the definition of a new function in Python.
How does Python use indentation to delineate code?
-Python uses indentation to indicate the start and end of a block of code, such as the body of a function.
Why is it necessary to define a function before calling it in Python?
-In Python, functions must be defined before they are called because Python reads from the top down, and it needs to know what the function does before it is executed.
What is the error that occurs if you try to call a function that hasn't been defined yet in Python?
-An error will occur stating that the function is not defined, with a reference to the line number where the call was made.
How does the script demonstrate teaching Carol a new command?
-The script demonstrates it by defining a function called 'turn right' and then calling that function to make Carol execute the new command.
What is the significance of the 'make a tower' exercise in the script?
-The 'make a tower' exercise is used as a practical example to illustrate how to teach Carol new commands using functions.
How does the script suggest to fix the issue of Carol not being able to turn right?
-The script suggests creating a function named 'turn right' that contains the sequence of commands to turn left three times, effectively turning right.
Outlines
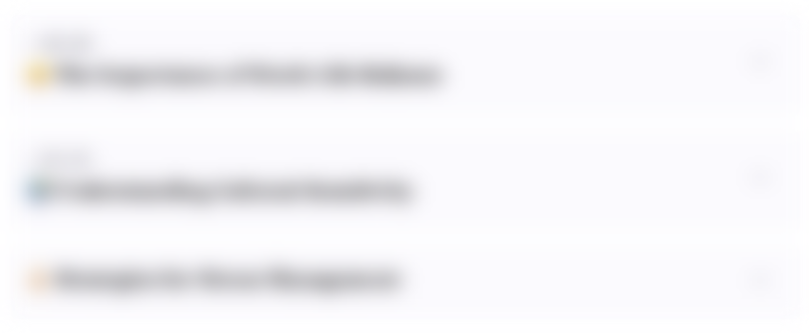
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
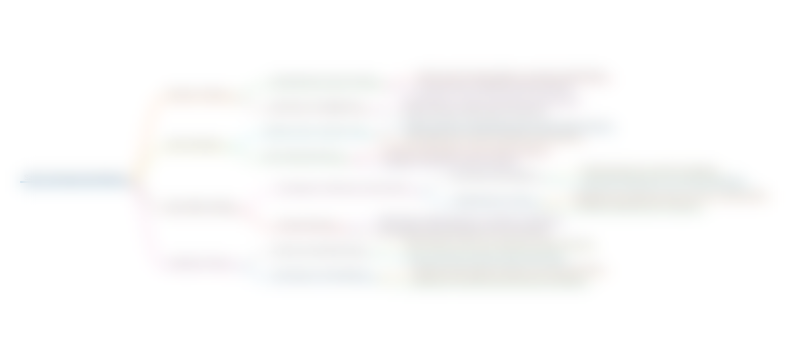
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
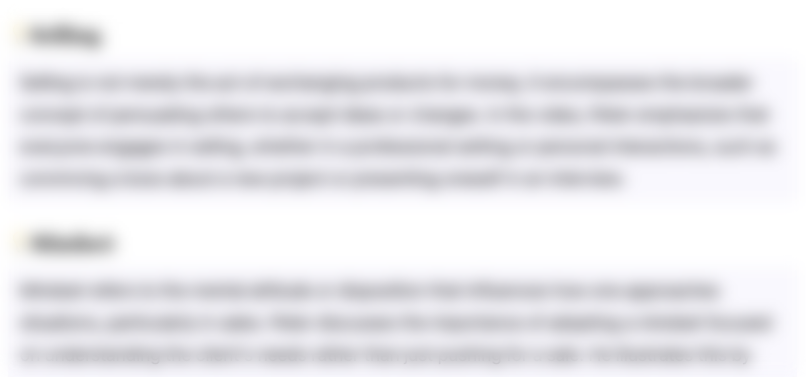
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
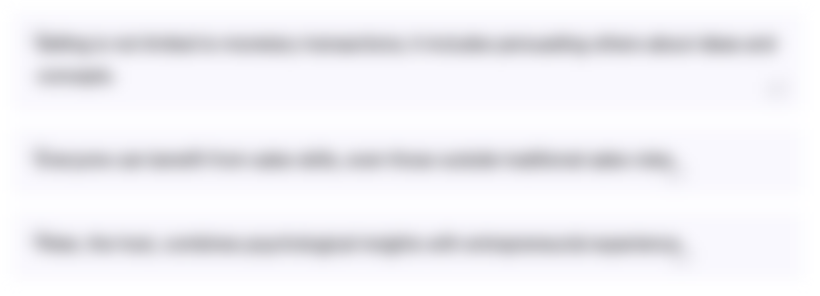
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
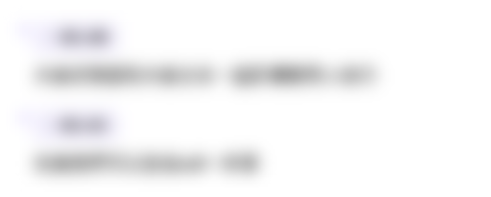
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahora5.0 / 5 (0 votes)