Pandas Creating Columns - Data Analysis with Python Course
Summary
TLDRThis script offers an insightful tutorial on manipulating data with pandas, a powerful Python library. It covers creating new columns by combining existing ones, like calculating GDP per capita, and emphasizes the importance of understanding series in pandas. The video also demonstrates reading external data, such as CSV files, with detailed steps for customization using the read_csv function. Additionally, it touches on data visualization using pandas' plotting capabilities, showcasing a comparison between Bitcoin and Ether over time.
Takeaways
- 📊 Modifying Data: The script discusses how to create new columns in a dataset by combining or modifying existing columns using operations like division.
- 🔢 GDP per Capita Example: It illustrates the process of calculating GDP per capita by dividing the GDP column by the population column in a dataset.
- 💡 Broadcasting Operations: The importance of understanding broadcasting operations between columns in pandas is highlighted, which are fast and result in a series.
- 📈 Statistical Methods: The script mentions various methods for summary statistics in pandas, including minimum, maximum, mean, and median.
- 📋 DataFrame and Series: It explains the difference between a DataFrame, which has multiple rows and columns, and a Series, which is a single column of data.
- 📚 Reading External Data: The script introduces methods for reading data from external sources like CSV, SQL, Excel, and HTML files using pandas.
- 📝 Customizing Read CSV: It provides insights into customizing the read CSV function in pandas, including handling headers, column names, and data types.
- 🗓️ Timestamps and Indexing: The process of converting a timestamp column into a datetime object and setting it as the DataFrame index for easy data access is explained.
- 🛠️ Data Cleaning: The script touches on the topic of data cleaning, including parsing dates and handling data types, which is crucial for accurate analysis.
- 📈 Plotting with Pandas: It demonstrates the simplicity of creating plots with pandas using the built-in plot method, which integrates with the matplotlib library.
- 📉 Analyzing Cryptocurrency Data: The script gives a real-life example of analyzing cryptocurrency data, showing how to read, clean, and visualize data for Bitcoin and Ether.
Q & A
What is the purpose of creating new columns that are combinations of other columns in a dataset?
-The purpose is to derive new insights or metrics from existing data. For example, calculating GDP per capita by dividing GDP by population.
How can you create a new column for GDP per capita in a pandas DataFrame?
-You can create a new column by assigning the result of a calculation between two existing columns to a new column name. For instance, `df['GDP per capita'] = df['GDP'] / df['Population']`.
What is the concept of broadcasting in pandas and how is it used?
-Broadcasting in pandas refers to the ability to perform operations between columns, which automatically aligns data on index and propagates the operation across all elements. It's used for efficient calculations across entire columns.
What is a pandas Series and how does it differ from a DataFrame?
-A pandas Series is a one-dimensional labeled array that can hold any data type, while a DataFrame is a two-dimensional labeled data structure with columns of potentially different types. A DataFrame can contain multiple Series.
How can you perform summary statistics on a pandas Series?
-You can perform summary statistics using methods like `df.describe()`, `df.min()`, `df.max()`, `df.mean()`, and `df.median()`.
What is the purpose of the `read_csv` function in pandas and what are some of its customization options?
-The `read_csv` function is used to read CSV files into a pandas DataFrame. Customization options include specifying column names, handling missing values, selecting specific columns, and parsing dates.
How can you handle a CSV file without column headers using pandas?
-You can handle a CSV file without headers by setting the `header` parameter to `None` in the `read_csv` function and manually specifying the column names.
What is the significance of setting the index of a DataFrame to a timestamp?
-Setting the index to a timestamp allows for easy access and manipulation of time series data. It enables operations like querying data for specific dates or time ranges.
How can you convert a column of strings to datetime objects in pandas?
-You can use the `pd.to_datetime()` function to convert a column of strings to datetime objects, which can then be used to set the DataFrame index or perform time series analysis.
What are some of the plotting capabilities provided by pandas?
-Pandas provides a simple interface to create plots using the `plot()` method of a DataFrame or Series, which internally uses the matplotlib library for visualization.
How can you automate the process of reading, processing, and analyzing data on a regular basis?
-You can automate the process by writing a script that includes all the steps from reading the data with `read_csv`, processing it, and then scheduling the script to run at specific times using a task scheduler.
Outlines
📊 Data Manipulation and Calculation with Pandas
This paragraph introduces the concept of data manipulation in Pandas, focusing on creating new columns based on existing ones. The speaker demonstrates how to calculate GDP per capita by dividing GDP by population, and assigns the result to a new column. The importance of understanding the difference between a DataFrame and a Series in Pandas is emphasized, as operations often return a Series which can then be used to set the value of a column in a DataFrame. The paragraph also touches on the speed of these operations, which are backed by NumPy arrays, and mentions the availability of summary statistics methods.
📚 Customizing the Read CSV Function in Pandas
The second paragraph delves into the customization options available in the read_csv function of Pandas. It explains how to handle CSV files without headers by setting the 'header' parameter to 'none' and manually specifying column names. The speaker also discusses the use of the 'parse_dates' function to convert timestamp strings into actual date objects and setting the DataFrame index to the timestamp for easier data access. The paragraph concludes with an example of automating the CSV reading process, including renaming columns, converting data types, and setting the index in a single call to read_csv.
📈 Introduction to Plotting with Pandas
In the final paragraph, the focus shifts to the plotting capabilities of Pandas. The speaker provides a brief overview of how to create plots using the plot method, which is powered by the matplotlib library. The paragraph demonstrates the ease of plotting with Pandas by showing a simple example of plotting Bitcoin and Ether prices on the same chart. It also touches on the process of data cleaning and the handling of missing values, as well as the potential for customizing plots further. The speaker hints at more detailed exploration of data sources and cleaning in future tutorials.
Mindmap
Keywords
💡Data Manipulation
💡Broadcasting
💡Pandas
💡DataFrame
💡Series
💡CSV
💡Timestamp
💡Indexing
💡Matplotlib
💡Data Cleaning
💡Plotting
Highlights
Creating new columns by combining data from existing ones, such as calculating GDP per capita.
Demonstrating the use of broadcasting operations for efficient data manipulation within a DataFrame.
The importance of understanding the difference between DataFrames and Series in pandas for data analysis.
Quick statistical analysis using summary statistics methods available in pandas.
Customizing the read CSV function to handle different data formats and requirements.
Reading external data from various sources like CSV, SQL, and Excel using pandas.
Automatic parsing of CSV files into DataFrames with pandas' read CSV function.
Handling large data files with methods like df.head() and df.tail() for data exploration.
Converting data types and parsing dates with the pd.to_datetime function for accurate data analysis.
Setting the DataFrame index to a timestamp for quick data retrieval and time series analysis.
Automating data processing scripts to run at specific times using pandas for regular updates.
Using the read_csv method's parameters to streamline data import and preprocessing.
Introduction to data visualization with pandas by invoking the plot method.
Creating plots with matplotlib library integration in pandas for data visualization.
Comparing different cryptocurrencies like Bitcoin and Ether on the same chart using pandas.
Handling missing data and gaps in time series data for accurate plotting and analysis.
Overview of pandas' capabilities for data cleaning, reading, and processing from various sources.
Transcripts
A few more examples of modifying data just for you to look at. And something that is
very common for us is creating columns that are combinations of other columns. So again,
this is read only, but you can you can imagine, that I could do is hear something
like, for example, GDP per capita, right? If I go here, and I do GDP per capita GDP, p per
capita, per capita, and here I say is equals to the GDP, this column divided by this
column, right, so, I do something like B, B three, actually c three, C three, divided by
b three, right. And then we would extend the values all the way along here. In pen this,
we could do something very similar, we can do just any column, we can just perform
operations, broadcasting operations between them, in this case is GDP by population. And
we can assign that series, which is the result right there. So it's a series, we are
going to assign that series to a new column. So, GDP per capita, there you go is now a
column of our data for. Again, all these broadcasting operations are extremely fast,
they are backed by their NumPy array, and they result in a series. So, very quick
statistical information, a few methods right to do summary statistics. We saw them with
this crime method. But minimum maximums mean, median, all that works as expected. Something
that I want you to note here, if possible, is that with pandas, we have, I'm going to
change colors here, we're going to use red. With Panis, you have this concept of a data
frame, right data frame that has multiple columns, multiple rows, and these operations
are resulting operations are resulting in just one series. So in pandas, you have your
data frame, and you have your series. And we could say we have individual numbers. And
it's like always, the data frame is always resorting back to this, it's like some
operations will just return a series. And the series can be used in a data frame, right. So
in this case, these resulted in a series. But then we merely use the series to set the
value of a column. Right. So that's why understanding series is so important. So
there are a few more assignment exercises for you here. So you can check them out and
complete them if it's going to make a little bit more sense once you're working with it.
Finally, I want to give you a very quick introduction to reading the external data
imploding. And to do that, we're going to use a few methods that are very popular in the
maybe we can look them up very quickly here, we can say read CSV, use that read CSV
function from pandas. So this function, read CSV. And as we have read CSV, we actually
have a few others read sequel, read Excel, read XML, there are multiple adjacent or
multiple ones, read HTML will be able to automatically parse an HTML page and read it.
So a few functions like these like, what we're gonna do with these read CSV, right
here is the structure of it.
A few of these functions will let us import data from an external source into our pain,
this workflow. So in this case, what we're going to read is these BTC market prize
volumes, so it's right here. If I open the CSV, this is what it looks like. It's the
date of the price. They again read and devalue the bridge, the timestamp, and the
value the timestamp of the value no decide the price of bitcoin 2017 and now it's close
to $9,000, I think, but just note inside, but again, this is a CSV and this is a CSV that
we're going to be writing. To do that. Again, we're going to use these methods read CSV,
the method will automatically parse the CSV as expected. And there you go. And the
process now will be for us to start tuning it to get to the right point. So I'm going to
show you a few customization, ISP customizations, we can do with the read
speed, read CSV function. So the first one, and sorry, let me tell you first, we have a
ton of attributes here. So we have a ton of customization to do with read CSV, you will
not remember all this, you will not remember everything out of the top of your head. So
don't worry, you can always go back again to the documentation and just practice, it's
going to come naturally. So the first thing, the first row of the CSV was considered to be
the column names. So in this case, this one lesson have a column name, let's say I add
it, I'm going to do timestamp timestamp price, we're going to save it, I'm going to
reiterate the file and re re read it. There you go. So by default, pandas is assuming
that the first line of the CSV is the rd columns, I'm going to go back into what it
was. Right, and I'm gonna show you again, that's the assumption that pandas is doing.
We're gonna, of course, of course, change that assumption, because in this case, our
CSV file does not have column names. So we're gonna just say, Heather equals none. And this
is when we start seeing the attributes that we're going to use from the read CSV
function, read CSV. And when I do Heather equals none, for us gonna be known. That
means don't infer, don't read a header, don't try to infer a header, a header from the CSV
file, and the columns are zero, and one. So now I'm going to change the columns, and I
say, actually, to be time sum in price. And now what I'm going to do is show you the
first rows. So you're saying here that I have these df dot head method that I'm doing.
That's because this is a significantly large file. So we're going to say not not that
long, but at least it doesn't fit in my screen, what's the shape of the data, the CSV
or the data frame, it has 365 rows, and we have two columns. So we can do df to info for
example, to have a little bit more reference about we have 365 values, there are no no
values, and price is actually float. The timesten is an object and we're going to fix
that in a second. I'm sorry, that the F that head on the F dot tail, are the methods we
use to get either the first and files or the end row sorry, are the last n rows, which are
five rows, by default, you can change that and say, Show me the last three rows, for
example. That's something you can do. And again, the types so the types is the
timestamp in this case, the timestamp column was not properly parses the date, he was
parsed as an object as a string, which we don't want. So we're going to use the
function PD dot
today time,
something we're gonna explore in more detail in the reading in the cleaning data cleaning
course. Part sorry, if it weren't tutorial, we're gonna use it today time function to
turn these column D f, the timestamp into an actual date. And now we're going to say the F
that timestamp equals to this function resulting, and now everything looks as
expected, there is one more change that we want to do, we want to set the index of the
data frame to be the timestamp, because by doing so, we can quickly access price
information. Let me see what was the price of bitcoin in 2000 1709 29. And I make a mistake
here, I forgot to do the LLC. There you go. So we have the value of Bitcoin. On this
particular date, forgot, look, remember that to get value from a particular row, you have
to do dot lock. There we go. So we are getting Dodd's particular value. Because
we've made a timestamp at the index, we get x as a value directly from the index. So what
happens if you want to turn this thing into an automated script? For example, when I run
this process, every day at 5am, whatever we can, we want to read the CSV stripped the
columns, rename them turn into timestamps, etc. This is what we've done so far. Read the
CSV without a header, create the columns, turn it into a daytime timestamp into a
daytime and assign it to the index and that's the result again Well, actually, the read
CSV, oh, sorry, the read CSV method is so powerful that it will let us do all these
actions in just one call of the read CSV method, we there are parameters that will let
you customize the behavior to achieve the same results that we did with four lines of
code right here. So in this case, we're going to say, read this CSV, don't assign a header,
that's something we do already or don't don't infer our header from the first line. These
are the column names. So we don't need an extra line, we can just say these are the
columns names. Oh, and by the way, the first column is going to be the index of the data
frame, oh, and also part of the date, they've the index, it's a date, so part of the date.
And we have the same result, as before. So now I'm going to pro try and same thing.
There you go. So you can see it's work. So very quickly, Pan this plotting. Alright, so
we're going to be doing here is I'm going to show you very quickly, I don't know what's
this thing is, as a vertical scrolling, I want to show you very quickly that you can
create plots with hand this interest a breeze, it's so simple to create a plot. So
in this case, what we're going to be doing is, given a data frame, you can always invoke
the plot method. And the plot method, what it's doing, it's using the map load live
library, something that you can check if you want in the docs. But for now, it's not
necessary with these, we're going to be more than enough. What it's doing is just using,
again, the regular plug library, as you can see the mapple in library, which is part of
the standard PI Data stack. And again, for us to access using pandas is extremely simple,
just df dot, you're done, you can set the plot as you want, we're gonna see more
details of matplotlib. So don't worry too much about that later. So there is a more
challenging example here that I can just run very quickly, you can inspect the process we
follow to fix the data. But this is what we have. There we go. And what you can see right
here is the difference between the
Bitcoin and ether in this period of time right here, and they are both bloated in the
same chart. And that's because this is the resulting data frame, we have Bitcoin on one
side, and we have ether on the other side on we are plotting it right here. We're creating
one plot with all of it. And we are noticing these empty value right here. So what we can
do is we can go from December the first up to January the first these period, so we can
select that period is in that lock. And we can just go ahead and plot it again. And this
is what you see right here the gap that we're seeing. So again, this was the introduction
to pindus. We have a real life example of pandas following up. Also we have a little
bit of more data cleaning on reading all the interesting files and sources of data for in
getting more data into the pipeline, right. So the idea is going to be showing you how
you can import data from Excel from SQL and then do the actual processing and analysis
Weitere ähnliche Videos ansehen
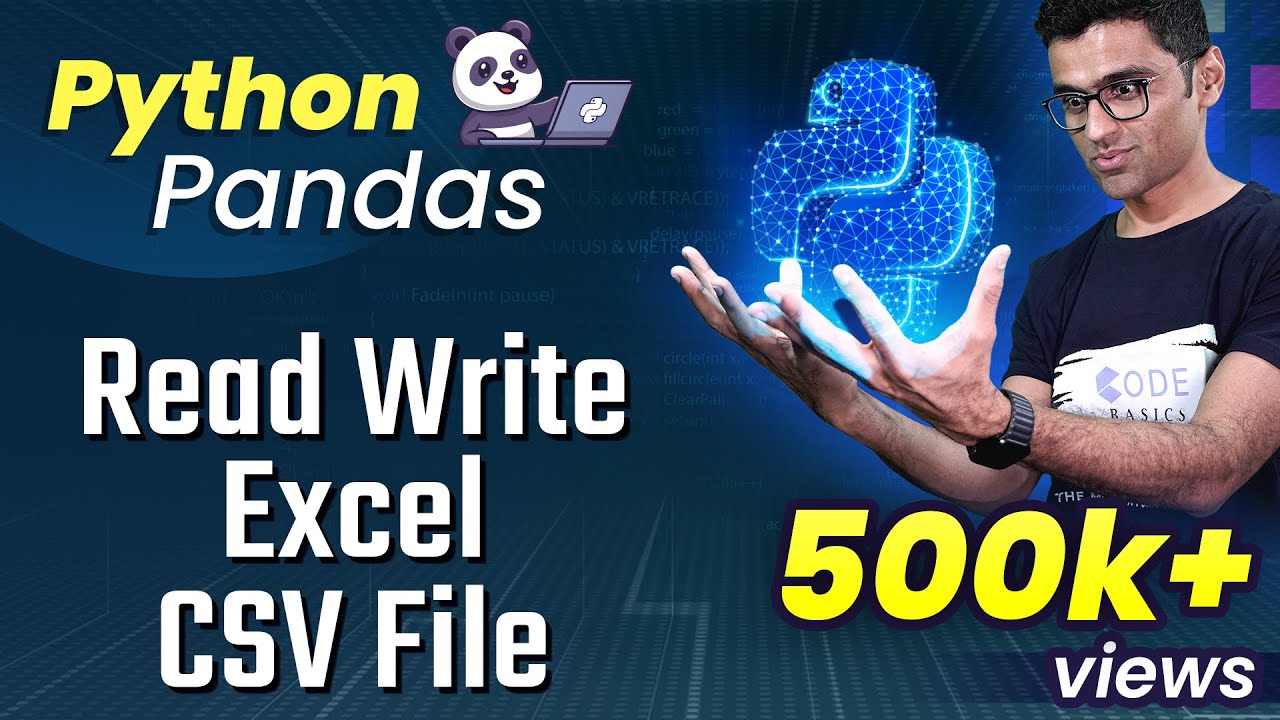
Python Pandas Tutorial 4: Read Write Excel CSV File
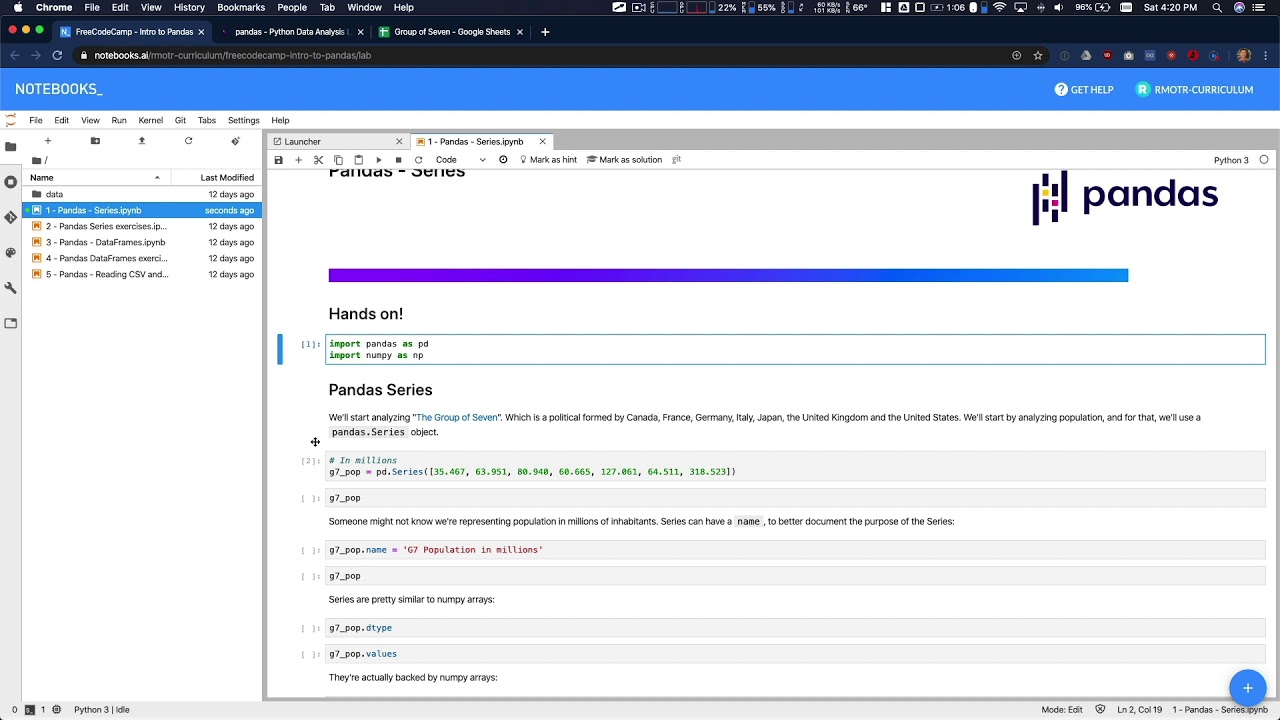
Pandas Introduction - Data Analysis with Python Course
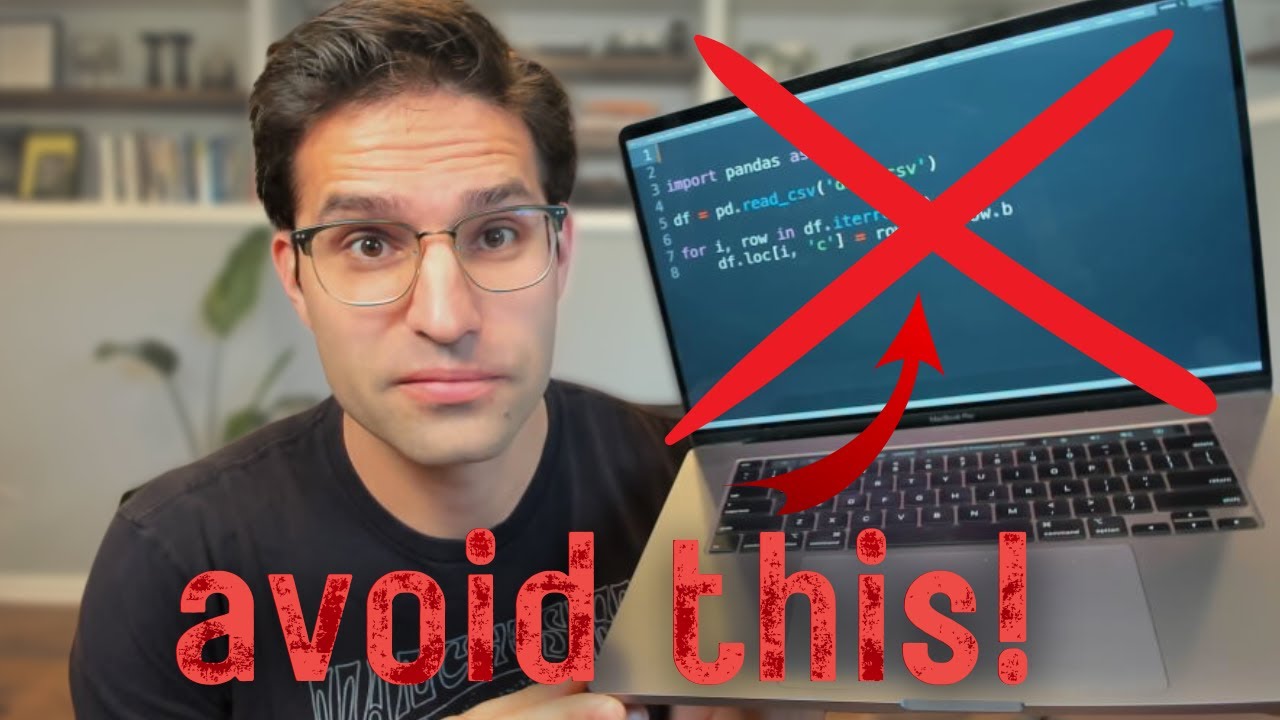
25 Nooby Pandas Coding Mistakes You Should NEVER make.
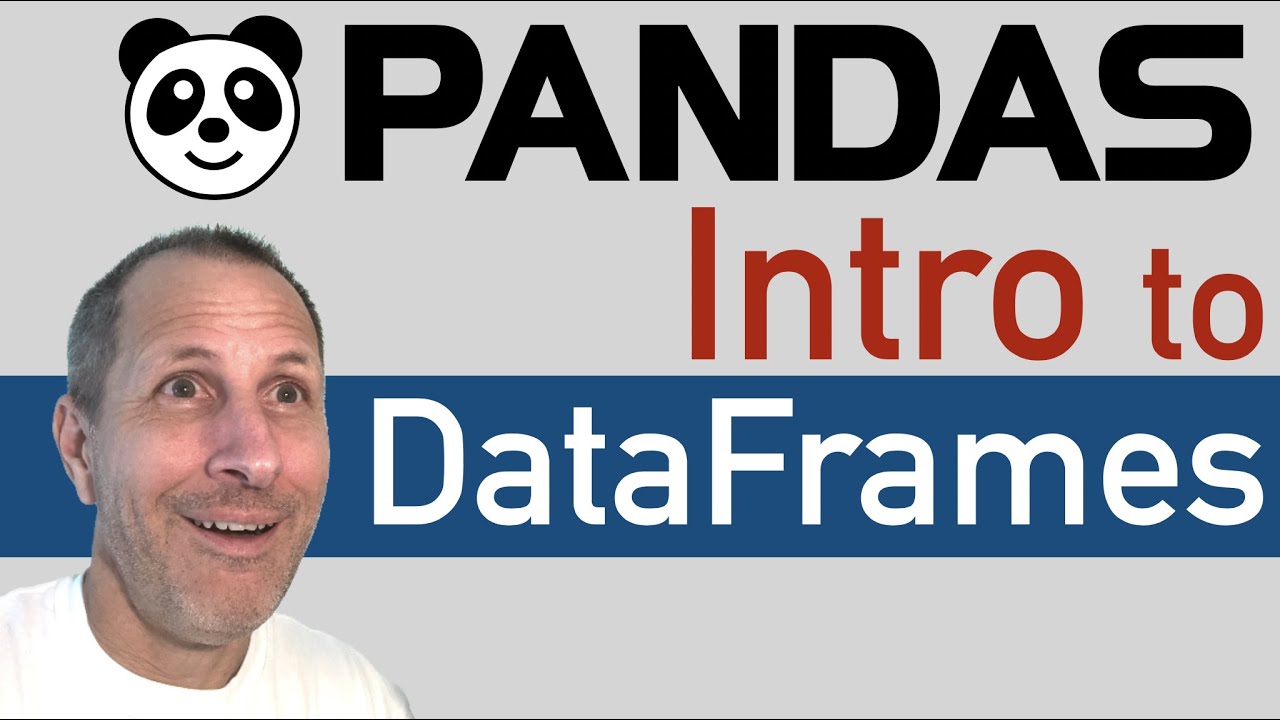
Python: Pandas Tutorial | Intro to DataFrames
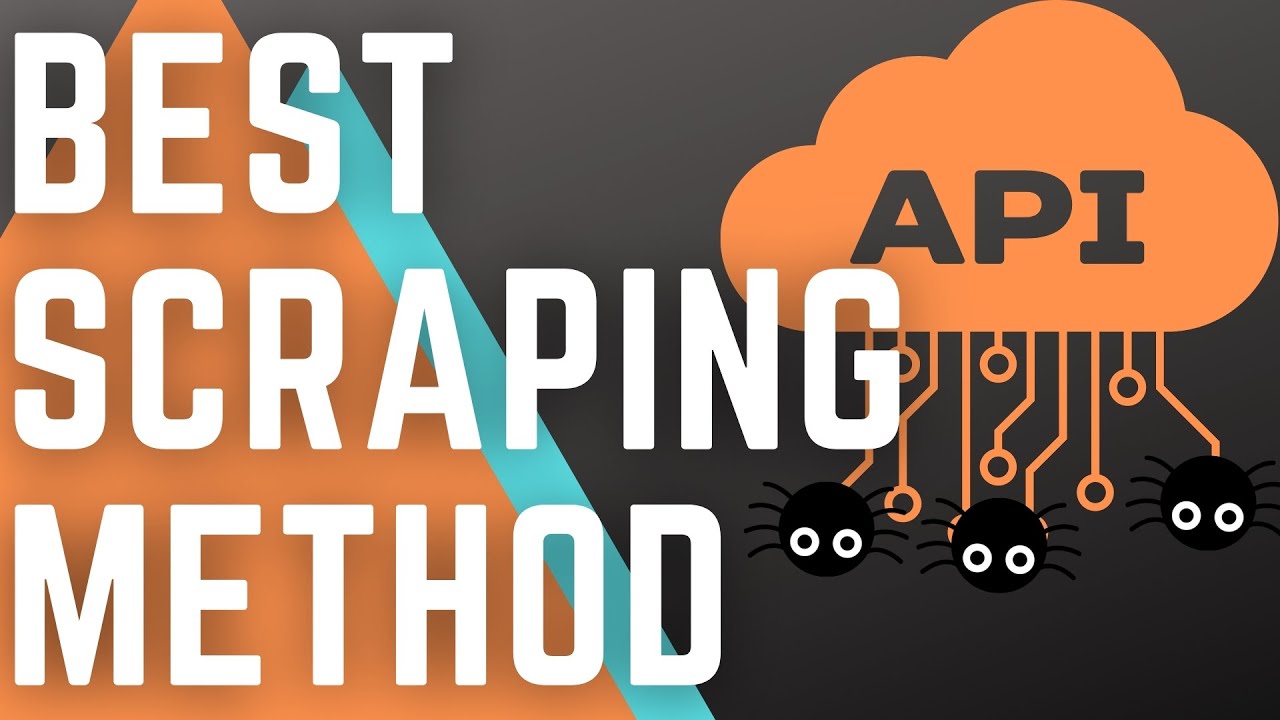
Always Check for the Hidden API when Web Scraping
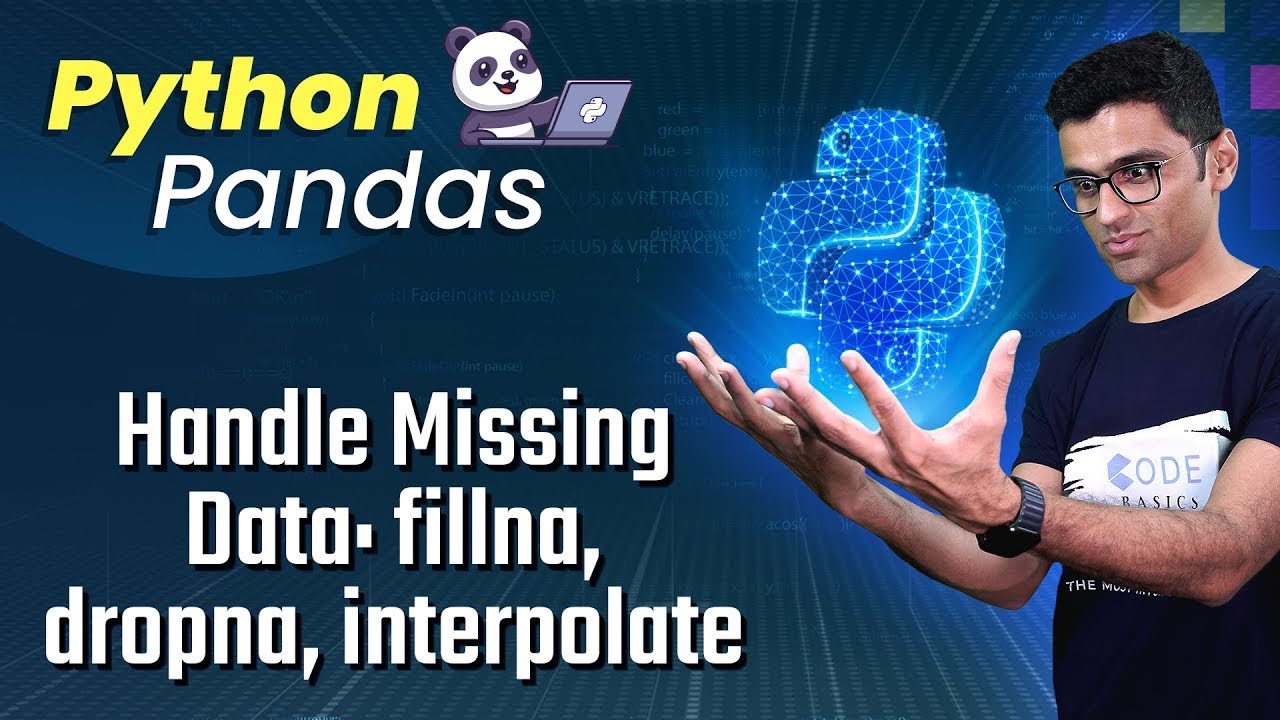
Python Pandas Tutorial 5: Handle Missing Data: fillna, dropna, interpolate
5.0 / 5 (0 votes)