8 patterns to solve 80% Leetcode problems
Summary
TLDRIn this insightful video, the presenter shares eight essential coding patterns to master for solving problems efficiently. Starting with the sliding window technique for processing data subsets, the video explores subset patterns for generating all possible arrangements, modified binary search for complex queries, and top-k elements using heaps. It also delves into binary tree traversals with DFS and BFS, topological sorting for dependency management, and the two-pointer technique for sorted arrays. The speaker emphasizes the importance of understanding data structures and algorithms, offering a free email crash course to enhance these skills.
Takeaways
- 🔍 The importance of recognizing patterns in coding problems to improve efficiency.
- 🪟 The sliding window pattern is useful for processing subsets of data in lists or strings to find elements that satisfy certain conditions.
- 🍽️ The subset pattern helps in finding all combinations of elements from a set, with or without repetitions, akin to a breath-first search approach.
- 🔍 Modified binary search involves adjusting the standard binary search algorithm to fit specific problem requirements, such as searching in a rotated sorted array.
- 📈 The top K elements pattern is for selecting the top K elements from a data set based on a given condition, often using a heap for efficient tracking.
- 🌳 Binary tree DFS (Depth-First Search) involves exploring each node in a tree by focusing on one branch at a time, typically using recursion.
- 🔄 Topological sort is essential for arranging elements with dependencies, especially in directed acyclic graphs, to determine a sequence of processing.
- 🌐 Binary tree BFS (Breadth-First Search) explores all nodes at the same level before moving to the next level, using a queue to maintain order.
- 👀 The two-pointer pattern is effective for iterating through sorted arrays to solve problems like finding pairs or triplets that meet specific sum conditions.
- 📚 A strong foundation in data structures and algorithms is crucial for effectively applying these patterns to solve coding problems.
- 📧 The speaker offers a free 5-day email crash course on 'interview.io' to master data structures and algorithms.
Q & A
What is the sliding window pattern used for in coding problems?
-The sliding window pattern is used for processing a series of data elements like a list or string by examining a smaller part of the list at a time, known as the window, which then slides one step at a time until the entire list is scanned. It's particularly useful when you need to find a subset of elements that satisfy a given condition.
Can you provide an example of a problem that would use the sliding window pattern?
-An example of a problem that uses the sliding window pattern is finding the longest substring with K unique characters in a given string. You need to find the longest substring that contains no more than K unique characters.
What is the subset pattern in coding and when should it be used?
-The subset pattern is used when you need to find all possible combinations of elements from a given set, with or without repetitions. It should be considered when a problem requires exploring all possible arrangements of elements from a set, such as in permutation problems.
How is the modified binary search pattern different from the standard binary search?
-The modified binary search pattern retains the core idea of dividing the search space in half repeatedly but requires adjustments to the logic to solve specific problems, such as searching in a rotated sorted array where the pivot point is unknown.
Why is it important to understand the core binary search algorithm well when dealing with modified binary search problems?
-Understanding the core binary search algorithm is crucial because it provides the foundation for adapting the logic to solve modified binary search problems. It helps in visualizing where the left and right pointers end up in various scenarios, such as when the array contains duplicates or does not contain the target.
What is the purpose of the top k elements pattern in coding problems?
-The top k elements pattern is used to select k elements from a larger data set based on a particular condition. It is useful when a problem asks to find the top ranking elements from a data set, such as finding the kth largest number in an array.
How does the depth-first search (DFS) pattern differ from the breadth-first search (BFS) pattern in a binary tree?
-The DFS pattern explores the tree by going deep into a branch and fully exploring it before moving on to other branches, typically using recursion. In contrast, the BFS pattern explores all nodes at the same level across different branches first, using a queue data structure to process nodes level by level.
What is the topological sort pattern used for in graph problems?
-The topological sort pattern is used to arrange elements in a specific order when they have dependencies on each other, particularly in directed acyclic graphs. It helps in determining the order of processing elements based on their prerequisites.
Can you give an example of a problem that would use the two-pointer pattern?
-An example of a problem that uses the two-pointer pattern is the two-sum problem, where you are given a sorted array and need to find the indices of two numbers that add up to a specific target sum. By moving two pointers smartly, one starting from the beginning and the other from the end of the array, the problem can be solved efficiently in a single pass.
What is the significance of understanding data structures and algorithms in solving coding problems discussed in the script?
-Understanding data structures and algorithms is fundamental to solving the coding problems discussed in the script. It provides the necessary knowledge to recognize patterns, apply appropriate algorithms efficiently, and develop solutions that can handle various problem scenarios.
How can one improve their understanding of binary search, as mentioned in the script?
-One can improve their understanding of binary search by implementing the 'bisect_left' and 'bisect_right' functions from Python's 'bisect' module in the language of their choice. This helps in visualizing how the binary search algorithm works and enhances the ability to adapt it to different scenarios.
Outlines
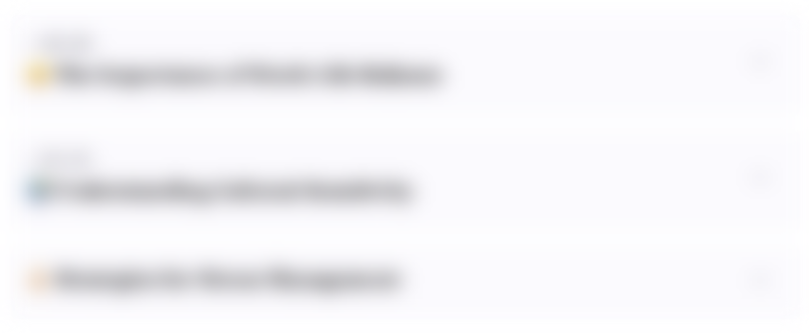
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
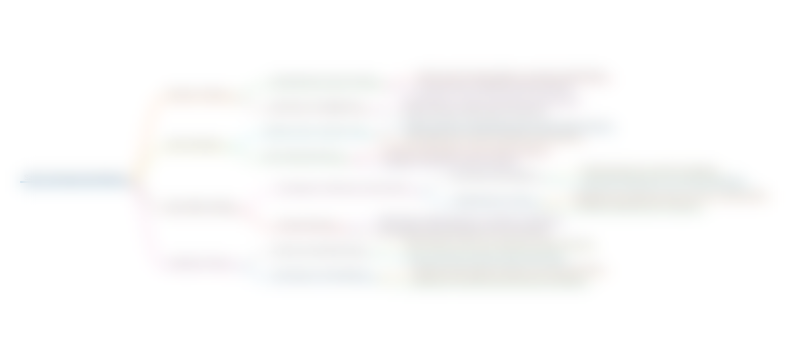
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
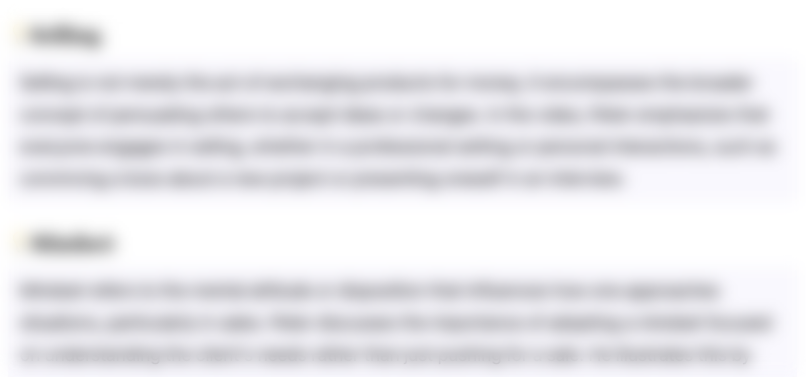
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
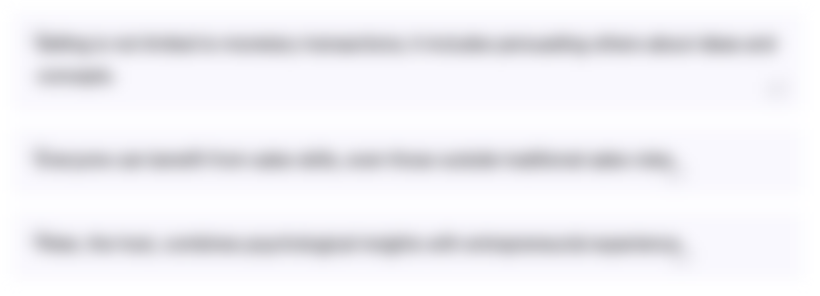
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
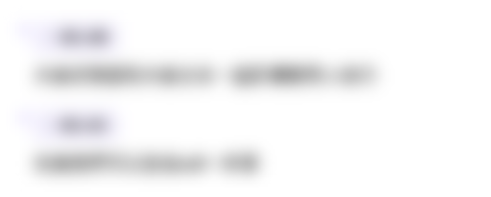
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
5.0 / 5 (0 votes)