Python Pandas Tutorial 4: Read Write Excel CSV File
Summary
TLDRThis tutorial covers the essentials of reading and writing CSV and Excel files using pandas in Python. It starts with setting up a Jupyter Notebook and proceeds to demonstrate how to import pandas, read CSV files with various headers, handle missing data with 'na_values', and write data back to CSVs with options to exclude index and select specific columns. The video also explains reading Excel files, converting cell content with 'converters', and writing to Excel with 'ExcelWriter', allowing multiple data frames in different sheets. The tutorial promises a follow-up on handling missing data in pandas.
Takeaways
- 📚 Start by launching a Jupyter Notebook for data visualization and analysis.
- 🔍 Import the pandas library as 'pd' for handling data in Python.
- 📈 Use `pd.read_csv()` to read a CSV file into a pandas DataFrame, specifying the file name within the function.
- 🚫 Handle extra headers in CSV files by using the `skiprows` argument to skip unnecessary rows.
- 📝 If a CSV file lacks headers, use `header=None` and provide column names manually with the `names` argument.
- 🔢 Utilize the `nrows` argument to limit the number of rows read from a large CSV file.
- 🧹 Clean messy data with `na_values` to replace specific text or patterns with NaN values for consistent analysis.
- 📉 Convert negative or invalid values to NaN where appropriate, such as negative revenue, using a dictionary in the `na_values` argument.
- 💾 Write DataFrames back to CSV files using `df.to_csv()`, with options to exclude the index and select specific columns.
- 📋 Read Excel files into pandas DataFrames with `pd.read_excel()`, specifying the file path and sheet name.
- 🛠 Use converters in `read_excel()` to transform cell content during file import, such as replacing 'NaN' with specific values.
- 📝 Write DataFrames to Excel files with `pd.to_excel()`, allowing for customization of sheet names, index inclusion, and starting cell positions.
- 📚 Use `ExcelWriter` to write multiple DataFrames to different sheets within the same Excel file.
Q & A
What is the primary focus of this tutorial?
-The tutorial focuses on reading and writing CSV and Excel files using the pandas library in Python.
Why does the author prefer Jupyter Notebook for this tutorial?
-The author prefers Jupyter Notebook because it integrates well with data visualization tools and is a versatile IDE for data analysis tasks.
What is a CSV file and how is it similar to an Excel file?
-A CSV file is a comma-separated values file, where values are separated by commas. It is similar to an Excel file in that both are used to store and organize data in a tabular format.
How does pandas handle an extra header in a CSV file by default?
-By default, pandas treats the first row of a CSV file as the header. If there are extra headers, the 'skiprows' argument can be used to skip them.
What is the purpose of the 'header' argument in pandas' read_csv function?
-The 'header' argument specifies the row number that contains the column names. If set to 'none', pandas generates column names automatically.
How can you read only a specific number of rows from a large CSV file?
-You can use the 'nrows' argument in pandas' read_csv function to specify the number of rows you want to read into the DataFrame.
What does the 'na_values' argument do when reading a CSV file?
-The 'na_values' argument allows you to specify which values should be considered as missing values (NaN) during the CSV file reading process.
How can you write a DataFrame back to a CSV file without including the index?
-You can use the 'index=False' argument in pandas' to_csv function to prevent the index from being written to the CSV file.
What is the role of the 'converters' argument in reading an Excel file with pandas?
-The 'converters' argument allows you to define custom conversion functions for specific columns in an Excel file, which can be used to transform or clean the data during the reading process.
How can you write multiple DataFrames to different sheets in a single Excel file?
-You can use the 'ExcelWriter' class in pandas to write multiple DataFrames to different sheets within the same Excel file.
What is the next topic the author plans to cover in the pandas tutorial series?
-The next tutorial will cover how to handle missing data in pandas.
Outlines
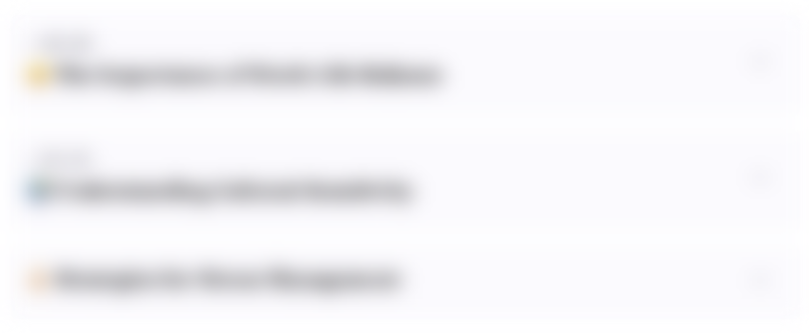
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
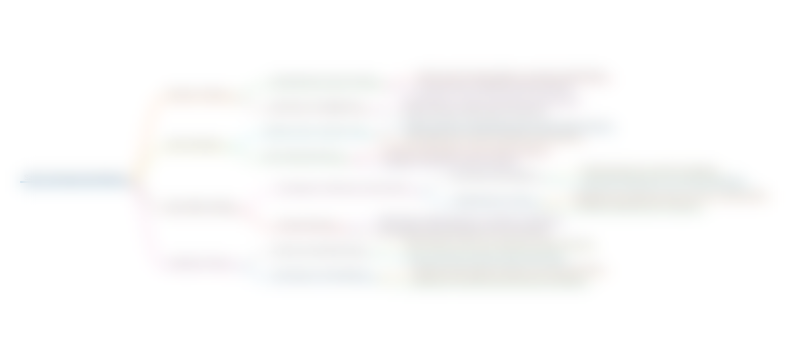
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
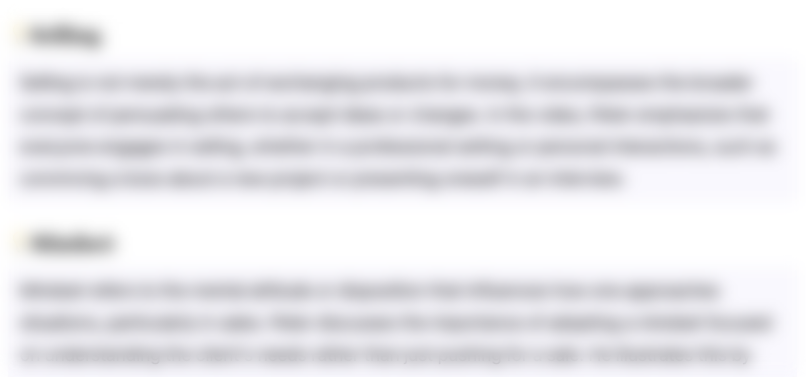
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
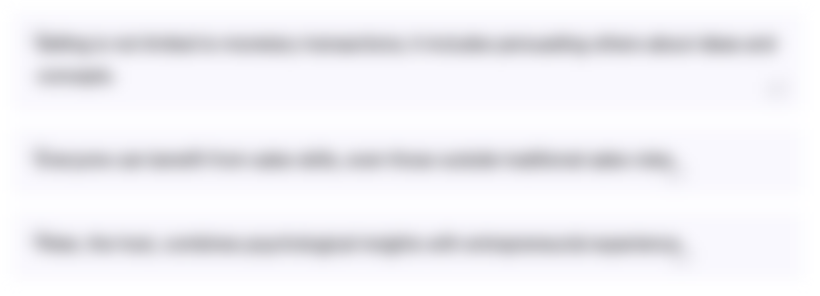
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
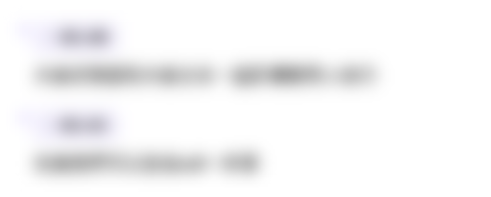
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
5.0 / 5 (0 votes)