Data Structures & Algorithms Roadmap - What You NEED To Learn
Summary
TLDRThis video script serves as a comprehensive guide for aspiring software engineers to master data structures and algorithms. It emphasizes the importance of understanding big O notation and time complexity, then delves into various data structures including arrays, linked lists, trees, and graphs. The script also covers essential algorithms like recursion, searching, sorting, and graph traversal, before touching on advanced topics and mathematical foundations. The speaker offers resources and tips for effective learning, aiming to equip viewers with the necessary skills for software engineering roles.
Takeaways
- 📚 Data structures and algorithms are essential for aspiring software engineers, and the video provides a roadmap for learning them effectively.
- 🔍 Understanding Big O notation and time complexity analysis is fundamental, as it helps evaluate the efficiency of code and is crucial for mastering data structures and algorithms.
- 🕒 Time and space complexity are key concepts that should be thoroughly understood, as they are applicable to all areas of data structures and algorithms.
- 📈 Arrays are the starting point for basic data structures, with a focus on understanding their operations, efficiency, and the differences between dynamic and fixed-sized arrays.
- 🔗 Linked lists, both singly and doubly, are fundamental data structures where understanding node references, traversal, and operation efficiencies is important.
- 🔁 Queues and stacks are linear data structures that require knowledge of their specific operations and the efficiency of those operations in different scenarios.
- 🌳 Trees, including binary trees, binary search trees, and heaps, introduce non-linear data structures with unique properties and traversal methods.
- 🔍 Graphs are complex data structures with various properties and require understanding of representation methods and traversal techniques.
- 🔑 Hashing is an important concept for understanding the efficiency of everyday data structures like hash maps.
- 🤔 Algorithms are categorized into several types, including recursion, searching, sorting, and more advanced ones like divide and conquer, dynamic programming, and backtracking.
- 🛠️ Recursion, searching algorithms (linear and binary search), sorting algorithms (like merge sort and quicksort), and graph algorithms (depth-first and breadth-first search) are fundamental and should be deeply understood.
- 📈 Advanced data structures and mathematical topics like tries, B-trees, AVL trees, red-black trees, skip lists, segment trees, Fenwick trees, disjoint sets, and discrete math are optional for those seeking deeper knowledge but are not required for most software engineering roles.
Q & A
What is the first and most crucial topic to master when studying data structures and algorithms for software engineering?
-The first and most crucial topic to master is Big O notation and time complexity analysis, which helps in understanding the efficiency of code and comparing different algorithms.
Why is it important to understand time complexity and efficiency when learning about data structures?
-Understanding time complexity and efficiency is important because it translates to every area of data structures and algorithms, allowing one to choose the most efficient data structure for different types of operations.
What are the four main operations typically associated with data structures?
-The four main operations typically associated with data structures are creating, deleting, inserting, and locating elements.
What is the difference between a dynamic array and a fixed size array?
-A dynamic array can grow in size as needed, whereas a fixed size array has a predetermined number of elements and does not change in size.
How does a singly linked list differ from a doubly linked list?
-A singly linked list allows traversal in one direction, from the head to the tail, while a doubly linked list allows traversal in both directions, with each node having a reference to both the previous and next nodes.
What are the advantages of using a queue over a stack in terms of data structure operations?
-A queue maintains the order of element insertion, which is useful for scenarios where items need to be processed in the order they arrive. A stack follows the Last In, First Out (LIFO) principle, which is beneficial for scenarios like function call stacks or undo mechanisms in applications.
What are the three main types of tree traversal methods that should be understood when studying binary trees?
-The three main types of tree traversal methods are pre-order, in-order, and post-order traversal.
What is a binary search tree and how does it differ from a regular binary tree?
-A binary search tree is a binary tree with the property that the value of each node is greater than all values in its right subtree and less than all values in its left subtree. This differs from a regular binary tree which does not enforce any order among the node values.
What are heaps and how do they relate to priority queues?
-Heaps are special tree-like data structures that satisfy the heap property, where the parent node is either greater than or equal to (in a max heap) or less than or equal to (in a min heap) the values of its children. They are used to implement priority queues efficiently.
What are some famous graph algorithms that one should be familiar with?
-Some famous graph algorithms include Depth-First Search (DFS), Breadth-First Search (BFS), Dijkstra's algorithm, and A* algorithm for pathfinding, as well as Kruskal's and Prim's algorithms for finding minimum spanning trees.
What are some sorting algorithms that are fundamental to computer science knowledge?
-Some fundamental sorting algorithms include Merge Sort, QuickSort, Insertion Sort, Selection Sort, Bubble Sort, and Heap Sort.
What are some advanced data structures that can be studied for a deeper understanding of computer science?
-Some advanced data structures include Tries, B-Trees, AVL Trees, Red-Black Trees, Skip Lists, Segment Trees, Fenwick Trees, and Disjoint Set.
What mathematical topics can provide a deeper understanding of data structures and algorithms?
-Mathematical topics such as Combinatorics, Probability, Discrete Math, and Discrete Structures can provide a deeper understanding of why certain data structures have different time complexities and how to prove the time complexity of algorithms.
Outlines
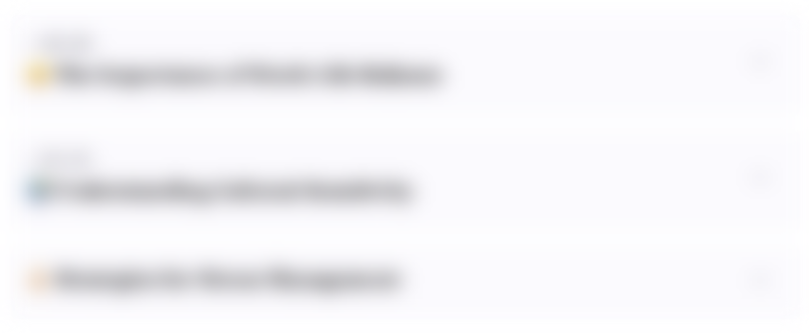
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
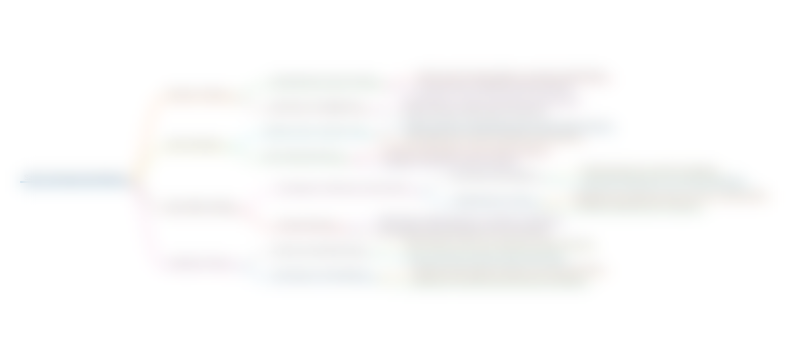
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
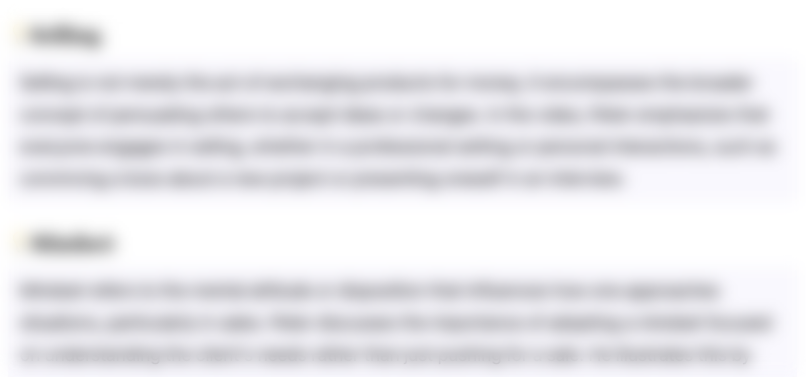
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
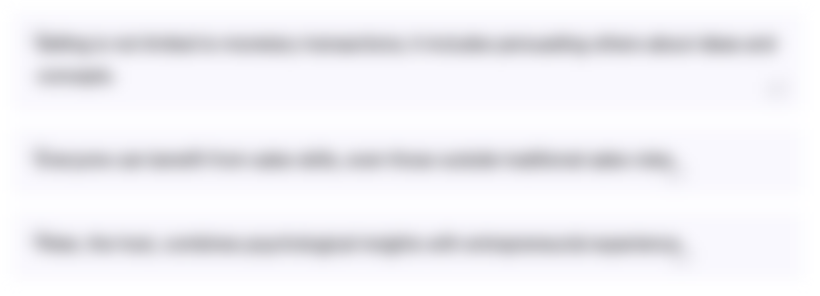
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
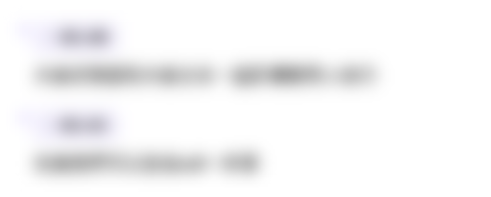
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
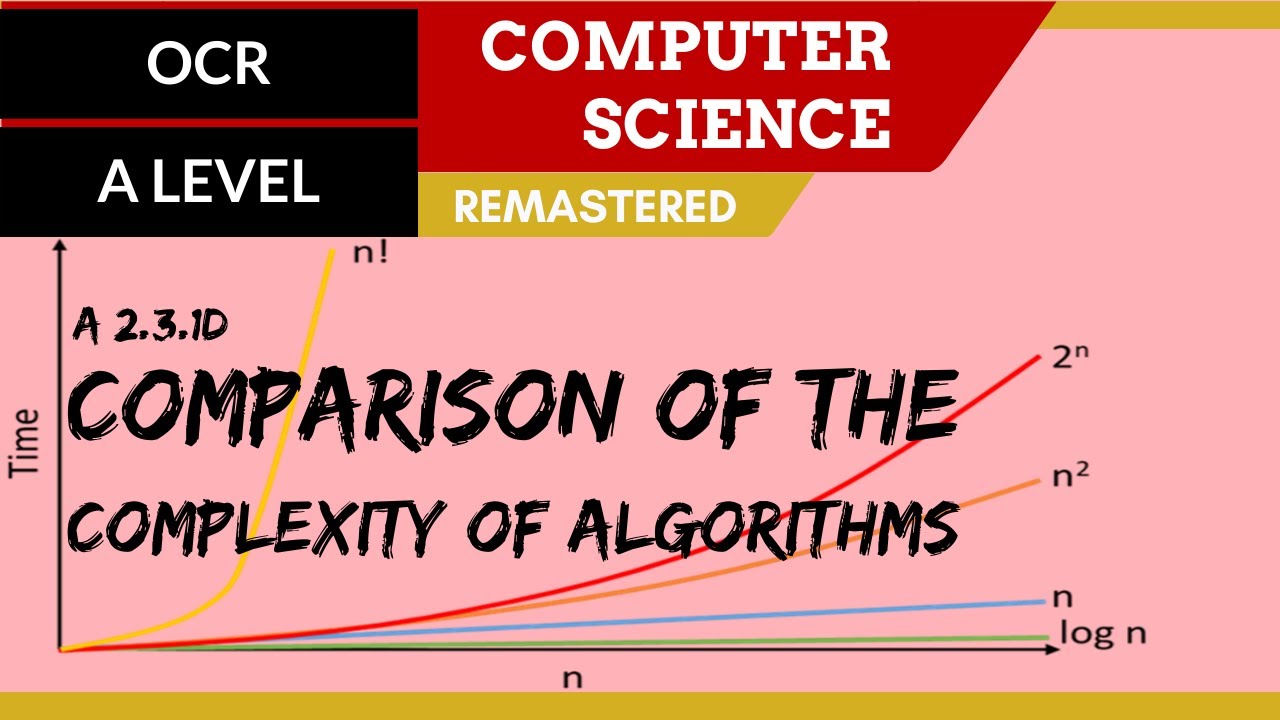
161. OCR A Level (H446) SLR26 - 2.3 Comparison of the complexity of algorithms

Data Structures and Algorithms in 15 Minutes
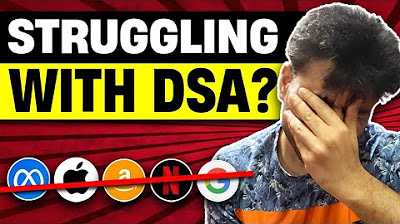
How to start DSA from scratch? Important Topics for Placements? Language to choose? DSA Syllabus A-Z

8 Data Structures Every Programmer Should Know
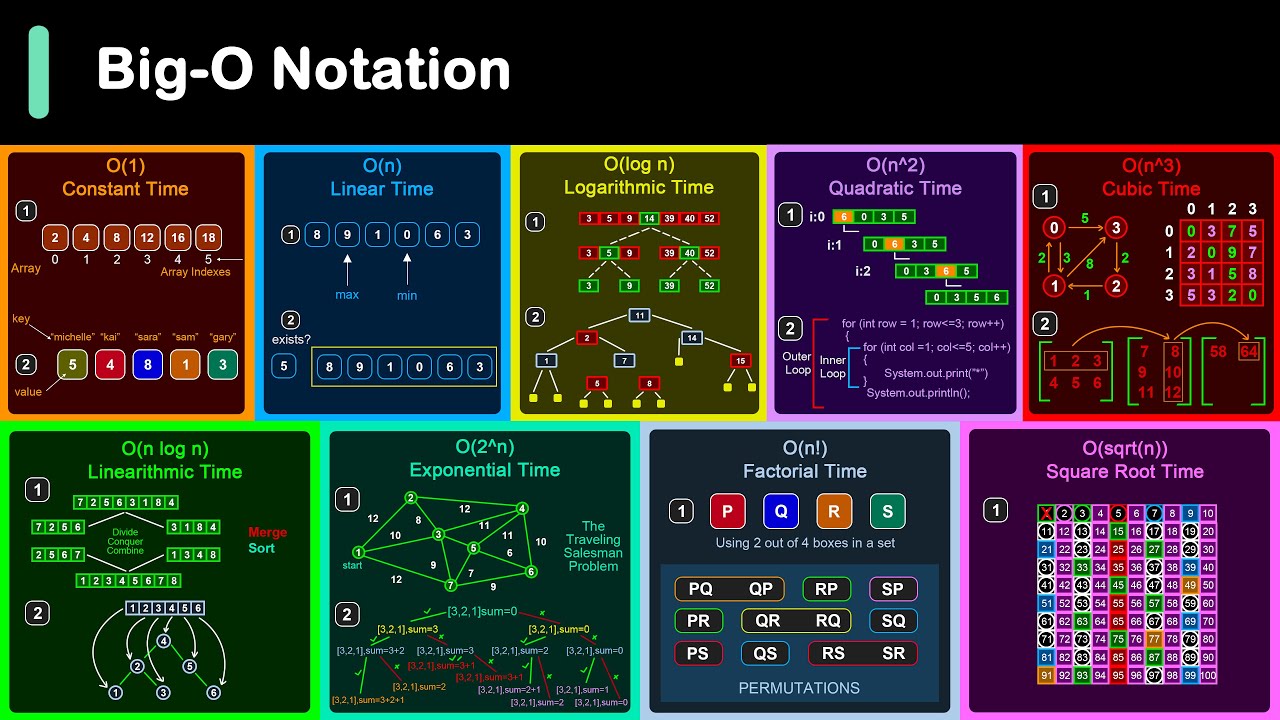
Big-O Notation in 3 Minutes

10 Key Data Structures We Use Every Day
5.0 / 5 (0 votes)