Programming Concepts - Design Patterns (Creational, Structural and Behavioral)
Summary
TLDRIn this video, Alex Forsyth introduces the concept of design patterns in computer science, drawing parallels with city planning. He explains how these patterns, categorized into creational, structural, and behavioral, can be applied to object-oriented programming to solve problems efficiently. Creational patterns focus on object instantiation, structural patterns on combining objects to form larger structures, and behavioral patterns on the interaction between objects. The video aims to provide a foundational understanding of these patterns, with individual patterns to be explored in future videos.
Takeaways
- 📚 The video discusses the concept of design patterns, popularized by the book 'Design Patterns: Elements of Reusable Object-Oriented Software', commonly referred to as the 'Gang of Four' book.
- 🏛️ Design patterns are categorized into three main types: creational, structural, and behavioral, each serving different purposes in object-oriented programming.
- 💧 An example of a creational pattern is the factory method, which provides a way to create objects without specifying the exact class of object that will be created.
- 🐅 The factory method can be used to create a common interface for creating different types of objects, such as various kinds of animals, without redefining properties and methods for each type.
- 🚢 Another example given is the use of a logistics interface object to handle different types of shipping operations, like shipping by boat or by truck, which illustrates the application of design patterns in real-world scenarios.
- 🧱 Structural patterns focus on composing structures by identifying simple ways to build complex systems out of objects that interact with one another.
- 🔄 Behavioral patterns are concerned with the interactions between objects, such as how a 'bottled water' object interacts with an 'Alex' object, affecting properties like thirst levels.
- 🔑 The video emphasizes the advantages of using design patterns in programming, such as reusability and the ability to solve common problems efficiently.
- 🔍 The speaker, Alex Forsyth, uses the analogy of city planning to explain the application of design patterns in object-oriented programming, suggesting a structured approach to problem-solving.
- 🎯 The video aims to introduce the concept of design patterns and their importance in programming, with a promise to cover individual patterns in more detail in future videos.
- 👋 The script concludes with a friendly sign-off, wishing the viewers a great day and encouraging them to enjoy the content.
Q & A
What is the main topic discussed in the video script?
-The main topic discussed in the video script is design patterns in computer science, specifically their application in object-oriented programming.
What is the origin of the term 'design patterns' in computer science?
-The term 'design patterns' in computer science was popularized by a book often referred to as the 'Gang of Four' book, which categorized different design patterns.
What are the three categories of design patterns mentioned in the script?
-The three categories of design patterns mentioned are creational, structural, and behavioral.
What is an example of a creational design pattern mentioned in the script?
-An example of a creational design pattern mentioned is the factory method, which provides a template for creating objects.
How does the factory method help in object creation?
-The factory method helps in object creation by providing an interface for creating objects, allowing for the creation of different versions of objects without redefining their properties and methods each time.
What is the purpose of structural design patterns?
-Structural design patterns are used to create new objects by combining existing objects, allowing for the building of larger structures from smaller components.
Can you provide an example of how structural design patterns work?
-An example of structural design patterns is assembling a bottle of water using separate objects for the plastic bottle, the water, and the cap, and then combining them to form a complete product.
What are behavioral design patterns concerned with?
-Behavioral design patterns are concerned with the interactions between objects, how they affect each other's properties and methods.
How do behavioral design patterns solve problems?
-Behavioral design patterns solve problems by defining how objects interact with each other, affecting their properties and methods, which can be used to model complex interactions.
What is an example of a behavioral design pattern scenario given in the script?
-An example given in the script is a 'bottled water' object interacting with an 'Alex' object, where drinking from the bottle affects both the water level in the bottle and Alex's 'thirst' property.
What is the significance of understanding design patterns in programming?
-Understanding design patterns in programming is significant as it allows developers to solve common problems more efficiently by reusing proven solutions, promoting better software design and maintainability.
Outlines
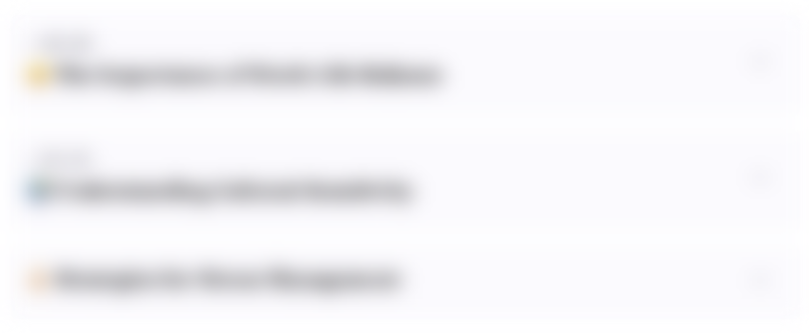
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
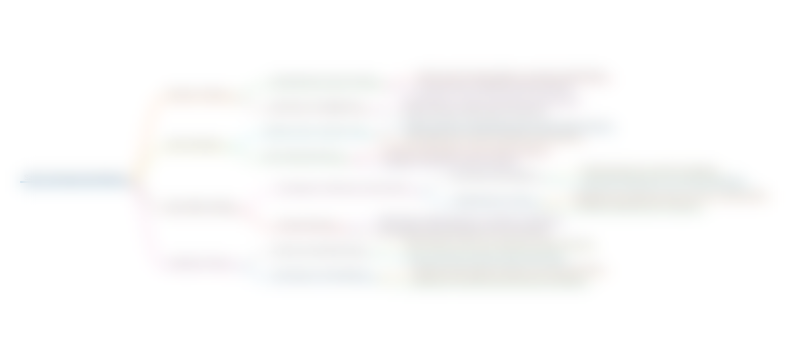
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
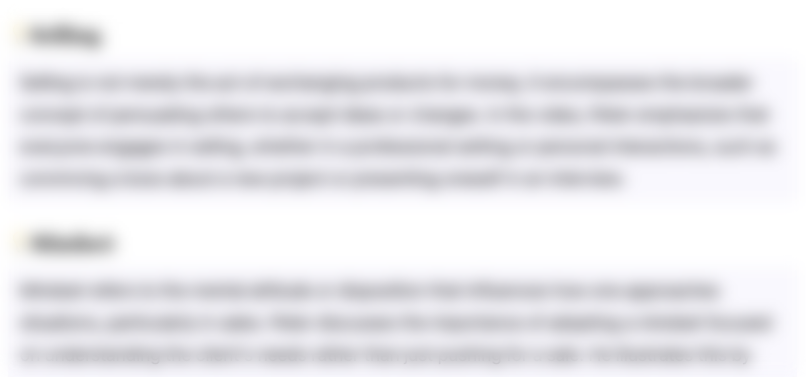
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
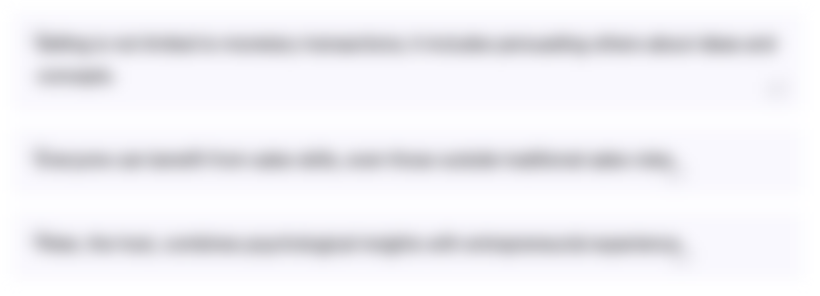
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
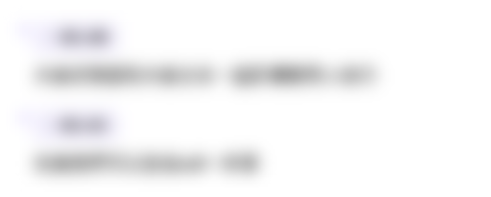
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
5.0 / 5 (0 votes)