90 - Orientação Objetos - Polimorfismo pt 01 - Introdução
Summary
TLDRThis tutorial introduces the concept of polymorphism in object-oriented programming using Java. It explains the creation of an abstract class for products, such as 'Computer' and 'Tomato,' each with tax calculation methods. The video walks through the process of defining product classes, implementing tax calculation, and organizing the code in separate packages for better structure. The instructor demonstrates the use of interfaces, abstract methods, and static methods to simplify the tax calculation process. The lesson prepares viewers to understand the core principles of polymorphism, setting the stage for more advanced discussions in future tutorials.
Takeaways
- 😀 Polymorphism allows objects of different types to be treated as instances of a common superclass, enabling flexibility in code design.
- 😀 Abstract classes like 'Produto' are used to define common properties and methods that must be implemented by subclasses.
- 😀 Interfaces, such as 'Taxavel', enforce a contract for subclasses to implement the method 'calcularImposto()', ensuring tax calculation consistency across product types.
- 😀 The 'Produto' class serves as a blueprint but cannot be instantiated directly; subclasses like 'Computador' and 'Tomate' provide specific implementations.
- 😀 Inheritance allows the 'Computador' and 'Tomate' classes to share common properties from 'Produto' while adding their own logic for tax calculation.
- 😀 The 'calcularImposto()' method is implemented in concrete subclasses to define tax calculations tailored to the specific product type, like 21% for computers and 6% for tomatoes.
- 😀 A service layer, such as 'CalculadoraImposto', separates business logic from domain objects, improving the organization and scalability of the code.
- 😀 Static methods can be used to perform actions like tax calculation without instantiating objects, making the code more efficient and straightforward.
- 😀 The method 'calcularImposto()' is used polymorphically, meaning it can handle different types of products (e.g., 'Computador' and 'Tomate') using the same method signature.
- 😀 Test classes create instances of 'Computador' and 'Tomate', calculate their taxes, and output the results, demonstrating the effectiveness of polymorphism in handling different types of data.
Q & A
What is the main topic discussed in the transcript?
-The main topic discussed in the transcript is 'polymorphism' in Java, specifically focusing on how polymorphism works in object-oriented programming and how it can be applied to real-world products like computers and tomatoes.
What is the purpose of creating an abstract class for products?
-The abstract class for products is created to ensure that all concrete product classes (like 'Computer' and 'Tomato') adhere to a common structure. This class defines the shared properties such as 'name' and 'value', and it ensures that all products must implement the method for calculating taxes.
Why is the 'calculateTax' method considered abstract in the 'Product' class?
-The 'calculateTax' method is abstract in the 'Product' class because the way tax is calculated can differ for each type of product (like computers or tomatoes). This method must be implemented in each subclass (e.g., Computer or Tomato), ensuring the correct tax logic for each product type.
What role does the 'Taxable' interface play in this example?
-The 'Taxable' interface defines the contract for any class that implements it, requiring the implementation of the 'calculateTax' method. It is used to ensure that any product, regardless of its specific type, has a way to calculate its tax.
How is the tax rate applied differently for a computer and a tomato?
-In the example, the tax rate for a computer is 21%, while the tax rate for a tomato is 6%. These rates are applied to the product's value to calculate the tax that needs to be paid.
What is the purpose of using the 'Service' package in the example?
-The 'Service' package is used to separate the business logic (in this case, tax calculation) from the domain model (Product). This separation helps maintain a clean structure and ensures that different concerns (like calculating taxes) are handled independently of the product's representation.
What is the advantage of using polymorphism in this example?
-Polymorphism allows different product types (e.g., Computer, Tomato) to be treated as instances of the common 'Product' class. This simplifies the code, as the tax calculation method can be called on any product, regardless of its specific type, and the appropriate method implementation will be invoked.
Why is it necessary to use both abstract classes and interfaces in this scenario?
-The abstract class 'Product' is used to define common properties and behavior, such as 'name' and 'value', while the 'Taxable' interface ensures that all products have a tax calculation method. This combination allows for both shared functionality and flexibility in implementation, adhering to the principles of object-oriented design.
What is the significance of using static methods in the tax calculation process?
-Using static methods in the 'TaxCalculator' class allows the calculation of taxes without needing to instantiate the class. This is useful when the tax calculation logic is not dependent on instance-specific data but rather on the provided product objects.
How does the example demonstrate the use of layers in a typical software architecture?
-The example demonstrates a multi-layer architecture with separate layers for the model (Product), business logic (Service), and visualization (test classes). This separation promotes modularity and maintainability in the software, following common practices in software design.
Outlines
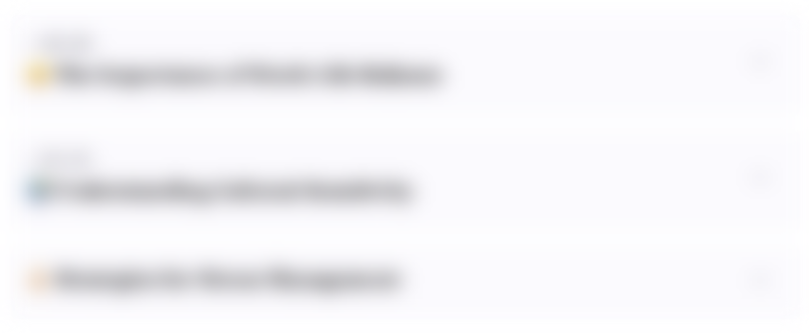
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
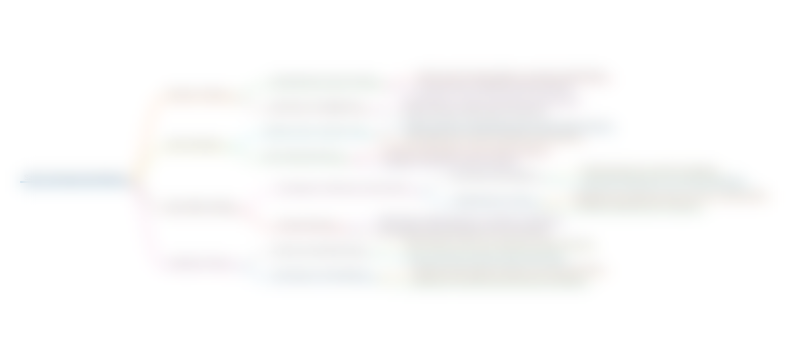
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
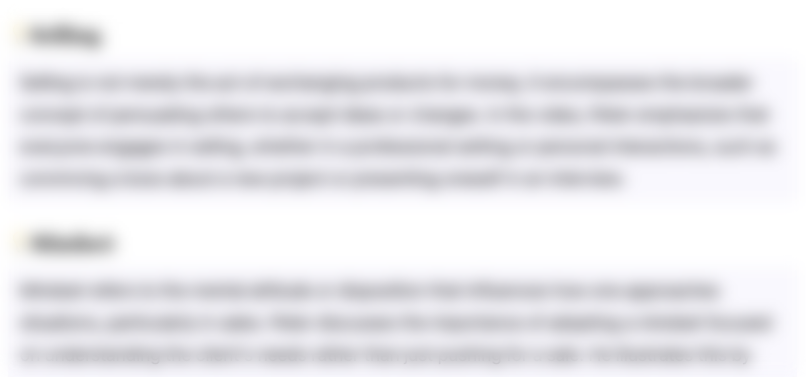
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
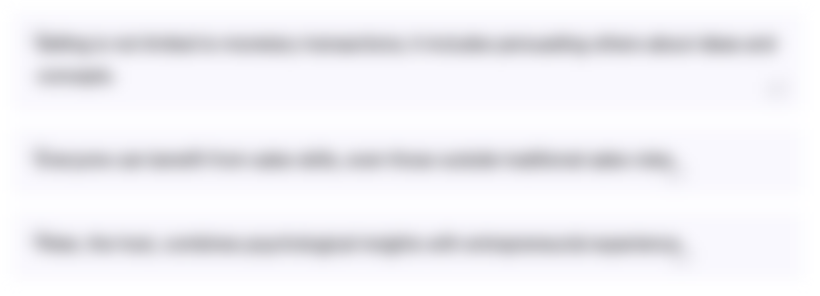
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
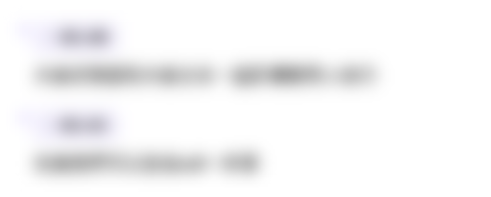
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
5.0 / 5 (0 votes)