One-to-Many and Many-to-Many Relationships in Clean Architecture
Summary
TLDRIn this tutorial, the domain layer of a movie rental system is implemented using Entity Framework. The video walks through the creation of entities like `Member`, `Rental`, and `Movie`, explaining how to model relationships such as one-to-many (Member to Rental) and many-to-many (Movie to Rental). Using Fluent API, the video shows how to configure these relationships and handle precision for decimal values. It also covers database context setup, migration for creating tables, and troubleshooting errors during the migration process. The tutorial concludes with the successful creation of database tables and hints at the next phase—building the application layer.
Takeaways
- 😀 The domain layer of an application focuses on handling domain-specific logic, such as managing entities and their relationships, independent of any other layers like infrastructure or application logic.
- 😀 A movie rental system requires entities like `Member`, `Rental`, and `Movie` to manage the business logic, with specific relationships defined between them.
- 😀 The relationship between `Member` and `Rental` is a one-to-many relationship, where a member can have multiple rentals, but each rental is tied to one member.
- 😀 The relationship between `Movie` and `Rental` is a many-to-many relationship. This is handled by introducing a join table called `MovieRental`, which links movies and rentals together.
- 😀 Fluent API in Entity Framework is used to configure entity relationships, allowing greater flexibility compared to data annotations.
- 😀 The `Member` class has properties such as `MemberId`, `FirstName`, `LastName`, and `Email`. It is linked to rentals via a one-to-many relationship.
- 😀 The `Rental` class includes properties like `RentalId`, `RentalDate`, `ExpiryDate`, and `TotalCost`, and is linked to both the member and movies.
- 😀 The `Movie` class has properties like `MovieId`, `Name`, `RentalCost`, and `RentalDurationInDays`. It is linked to rentals through the `MovieRental` join table.
- 😀 Entity relationships are configured using Fluent API within the `OnModelCreating` method, ensuring that the one-to-many and many-to-many relationships are correctly mapped in the database.
- 😀 Database migrations are used to generate the required database schema after defining the entities and relationships. Commands like `Add-Migration` and `Update-Database` are used to apply changes and create tables.
- 😀 Precision loss when dealing with decimal values (e.g., `TotalCost` and `RentalCost`) is handled in Fluent API by setting the column type to `decimal(18,2)` to ensure accurate representation.
Q & A
What is the purpose of the domain layer in the movie rental system?
-The domain layer handles the domain-specific logic of the application. In this case, it is responsible for managing entities like `Member`, `Rental`, and `Movie`, and defining their relationships and business rules within the system.
How does Entity Framework handle one-to-many relationships in the movie rental system?
-Entity Framework handles one-to-many relationships by linking a `Member` to multiple `Rentals` through a foreign key in the `Rental` table, which references the `Member` table's primary key.
Why does the script use Fluent API instead of Data Annotations for configuration?
-Fluent API is chosen because it offers more flexibility than Data Annotations, allowing more control over how the entities and relationships are mapped in the database, especially when dealing with complex relationships like many-to-many.
What is the role of the `MovieRental` table in the many-to-many relationship?
-The `MovieRental` table serves as a junction table in the many-to-many relationship between `Movie` and `Rental`. It stores the primary keys of both `Movie` and `Rental` to establish the relationship between them.
What challenge does Entity Framework face with many-to-many relationships, and how is it solved?
-Entity Framework does not directly handle many-to-many relationships without an intermediate table. To solve this, the script introduces a weak entity table (`MovieRental`) to link `Movie` and `Rental` through their primary keys.
How are `Member` and `Rental` linked in the domain model?
-The `Member` entity has a collection of `Rental` objects, establishing a one-to-many relationship. A `Rental` contains a foreign key (`MemberId`) that links it back to a `Member`.
What properties are included in the `Movie` entity, and why are they important?
-The `Movie` entity includes properties like `MovieId`, `MovieName`, `RentalCost`, and `RentalDuration`. These are essential for storing and managing details about each movie that can be rented in the system.
Why is the `decimal` type used for `RentalCost` and `TotalCost` properties, and what is the importance of precision?
-The `decimal` type is used for financial values such as `RentalCost` and `TotalCost` to ensure accurate representation of currency. Precision is important to avoid truncation or rounding errors, which could lead to incorrect financial calculations.
What is the significance of overriding the `OnModelCreating` method in the database context?
-Overriding the `OnModelCreating` method allows custom configuration of entity relationships using Fluent API. In this case, it is used to define how `Member`, `Rental`, and `Movie` entities are related and how their keys are set in the database.
What steps are involved in generating the database from the domain model?
-First, entities are defined and configured using Fluent API. Then, migrations are created using the Entity Framework CLI or Visual Studio, followed by applying the migration to the database to create the necessary tables and relationships.
Outlines
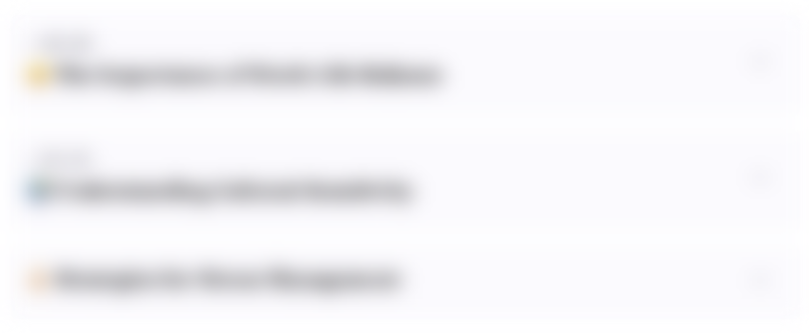
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
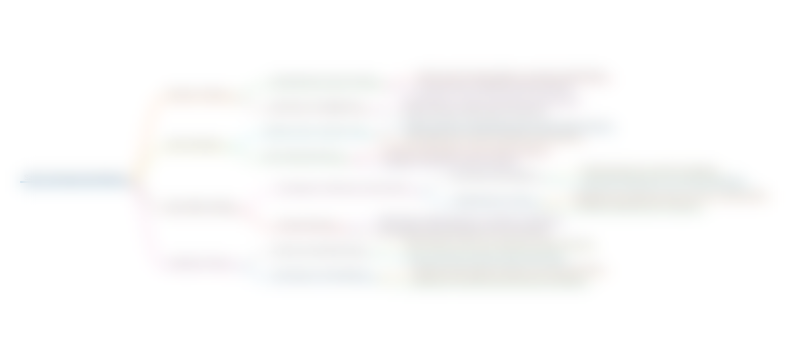
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
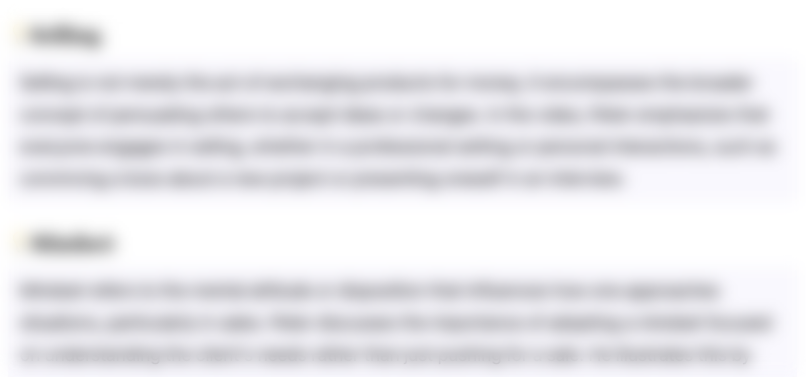
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
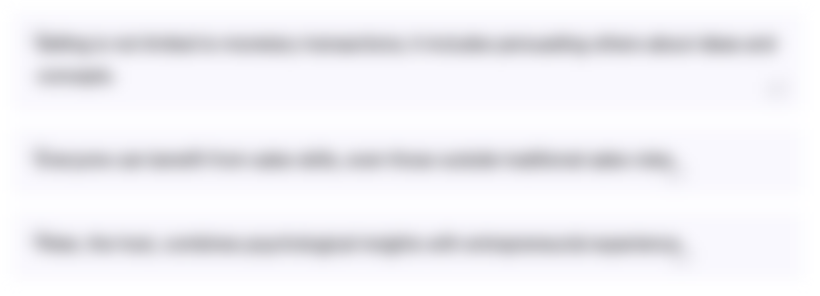
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
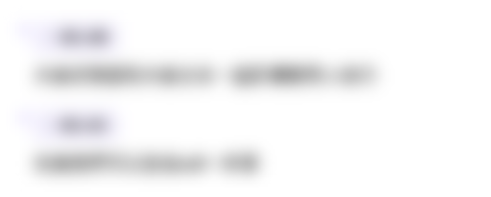
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
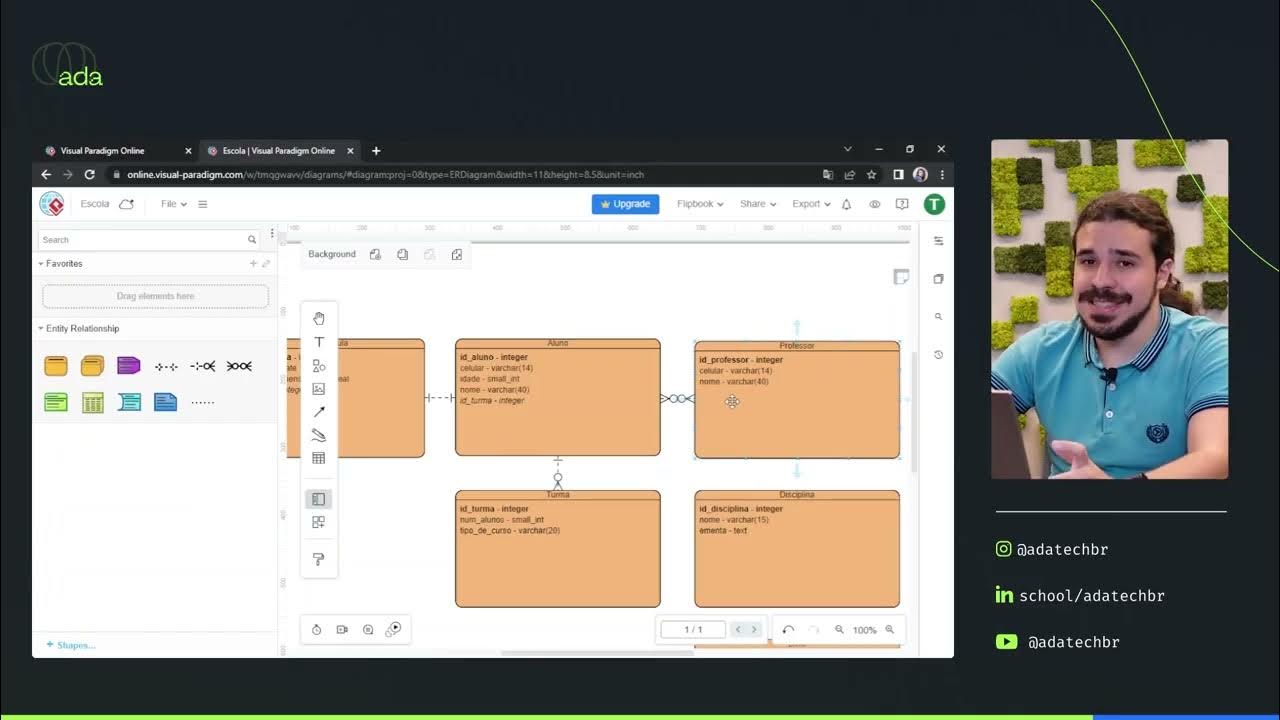
#M04A04 - MODELAGEM DE RELACIONAMENTOS
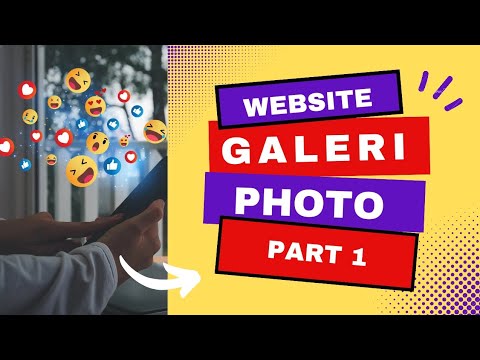
UKK RPL 2024 Website Galeri Photo Membuat Database menggunakan MariaDB xampp

Concept of Relationships in ER Diagram
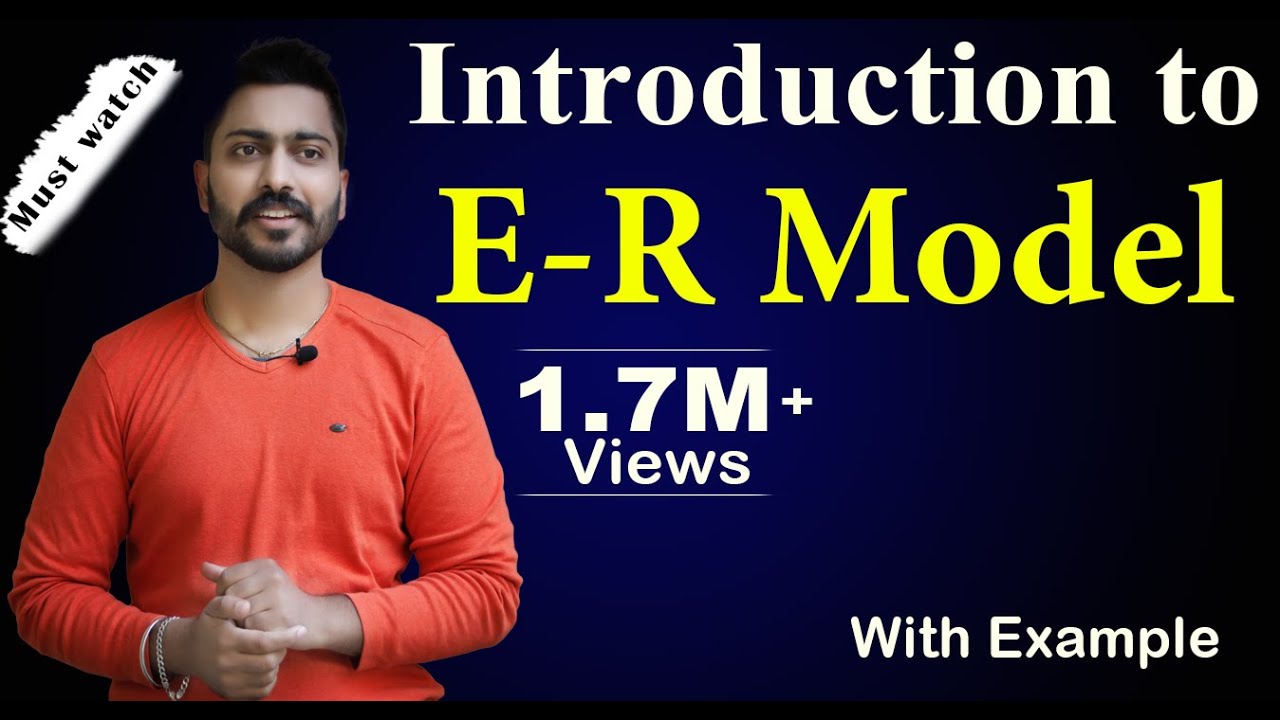
Lec-14: Introduction to ER model | ER Model क्या है
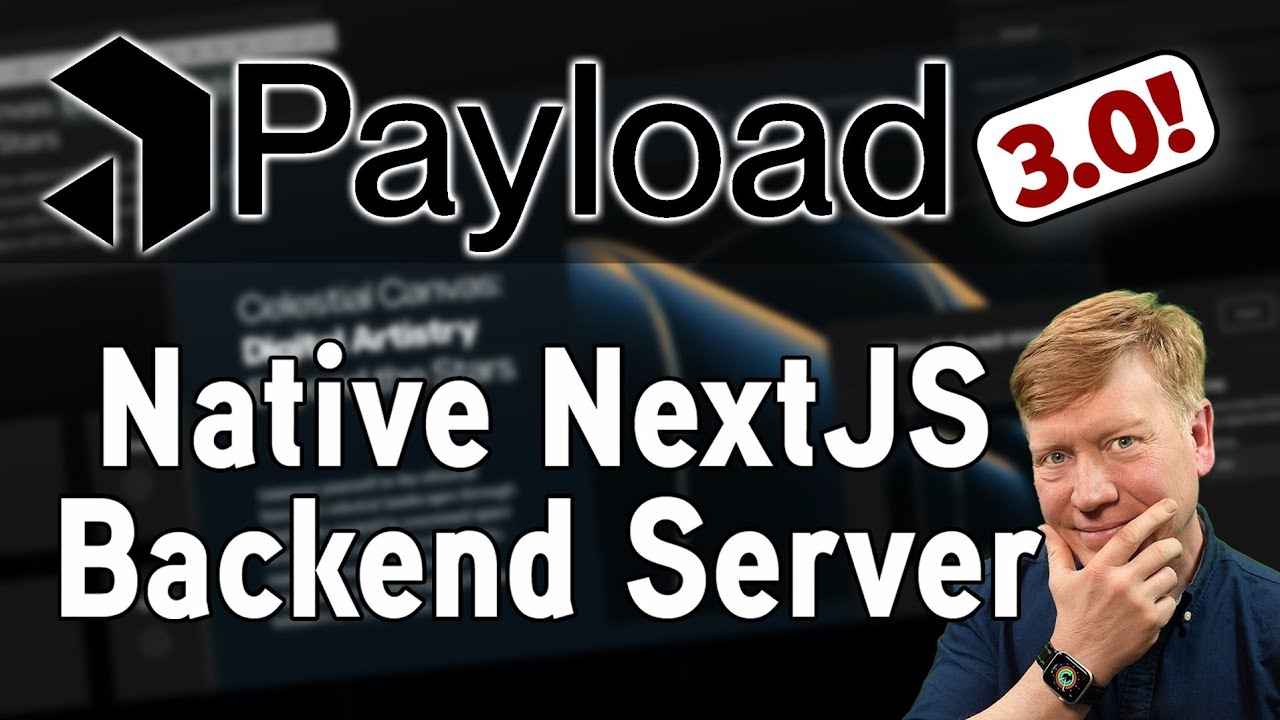
Payload: The Complete Backend for NextJS
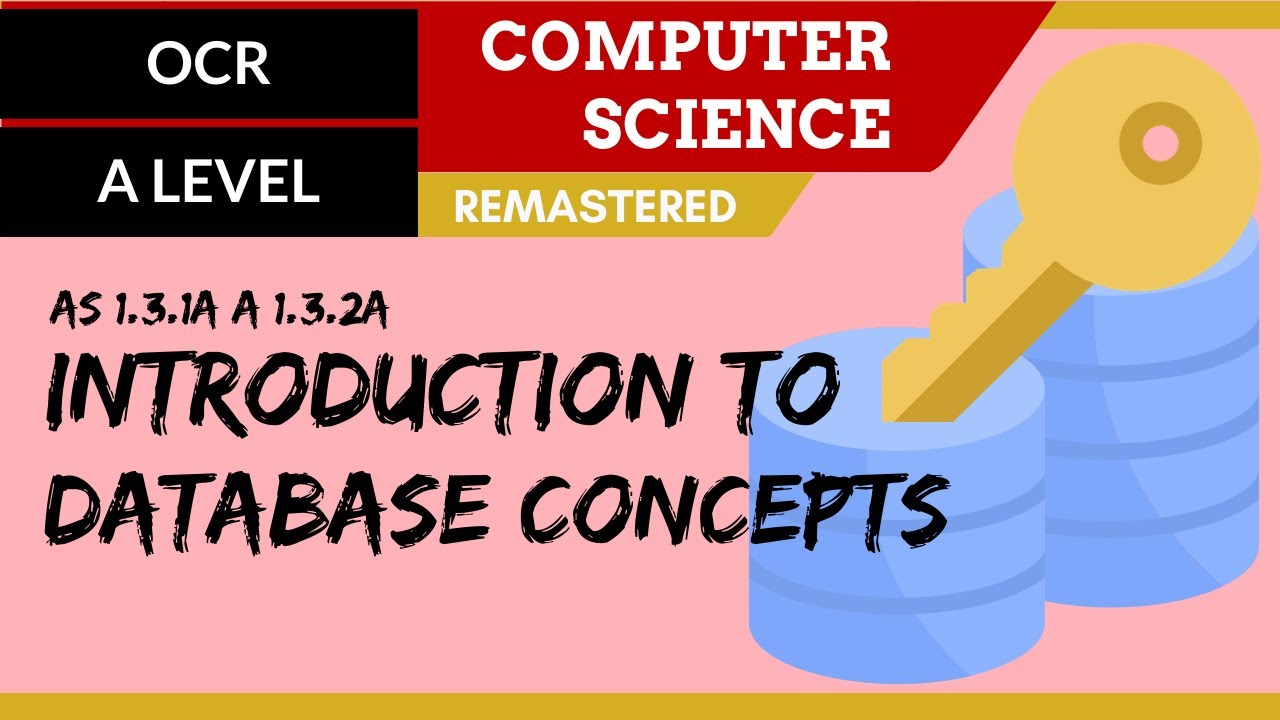
50. OCR A Level (H046-H446) SLR10 - 1.3 Introduction to database concepts
5.0 / 5 (0 votes)