Constructors in Java
Summary
TLDRIn this lecture, we explore the concept of constructors in Java, covering key topics like the role of constructors in object initialization, default constructors, and constructor overloading. The video explains how constructors must match the class name, lack return types, and are invoked using the 'new' operator. It delves into the default constructor, which takes no arguments and provides default values, and demonstrates how constructor overloading allows for multiple ways to initialize objects with varying parameters. Through clear code examples, the lecture showcases how constructors streamline object creation and initialization in Java.
Takeaways
- 😀 A constructor is a special method used to instantiate and initialize objects in object-oriented programming.
- 😀 Constructors must have the same name as the class they belong to.
- 😀 Constructors do not have a return type, distinguishing them from other methods.
- 😀 Constructors are invoked using the 'new' operator when creating objects.
- 😀 A default constructor is a constructor with no parameters and an empty body, used to create objects with default values.
- 😀 When no constructors are defined, Java automatically creates a default constructor.
- 😀 If a constructor is defined in the class, the default constructor is not automatically created by Java.
- 😀 Constructor overloading allows you to create multiple constructors with different parameter lists in the same class.
- 😀 A parameterized constructor can be used to initialize object attributes with specific values provided at the time of object creation.
- 😀 When using the default constructor, attributes are often initialized to default values (e.g., null for objects, 0 for numbers).
- 😀 Constructor overloading provides flexibility in creating objects, offering both default and customized initialization options.
Q & A
What is a constructor in object-oriented programming?
-A constructor is a special method used to instantiate and initialize objects of a class. It has the same name as the class and does not have a return type. It is called when an object is created, typically using the `new` operator.
What are the characteristics of a constructor?
-A constructor must have the same name as the class. It has no return type, not even `void`. Constructors are called using the `new` operator to initialize an object's attributes.
What is the difference between a default constructor and an overloaded constructor?
-A default constructor is a constructor that takes no parameters and has an empty body, used to create objects with default values. An overloaded constructor is one that can take parameters, allowing for custom initialization of an object.
Can you explain what happens when no constructor is defined in a class?
-If no constructor is explicitly defined in a class, Java automatically creates a default constructor with no parameters. This constructor initializes object attributes to default values (e.g., `null` for objects and `0` for numeric types).
What happens if you define a constructor in a class and no default constructor is provided?
-If you define a constructor with parameters, the default constructor is no longer automatically created by Java. If you still want to use a constructor with no parameters, you must explicitly define it.
How can you use a default constructor in a class with other constructors?
-To use a default constructor in a class that already has other constructors, you need to explicitly define it. This constructor would not take any parameters and would initialize attributes with default values.
What is constructor overloading and how does it work?
-Constructor overloading occurs when a class has multiple constructors, each with a different set of parameters. This allows objects of the class to be created with different initialization values based on which constructor is used.
What are the default values assigned by the default constructor in the example provided?
-In the example provided, the default constructor assigns a `null` value to the `center` attribute and `0` to the `radius` attribute of the `Circle` object.
How can we modify the default constructor to assign custom default values?
-You can modify the default constructor by manually assigning default values. For example, you can set the `center` to a new `Point(0, 0)` and the `radius` to `1.0` within the constructor body.
In the example, how does Java determine which constructor to use when creating an object?
-Java determines which constructor to use based on the number of arguments passed during object creation. If no arguments are passed, the default constructor is used; if arguments are passed, the constructor matching the number and type of arguments is invoked.
Outlines
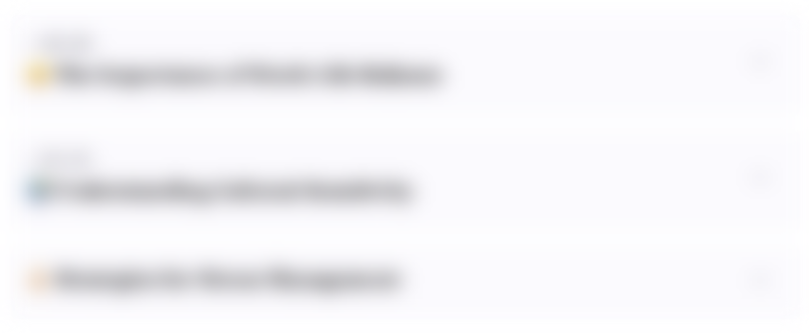
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
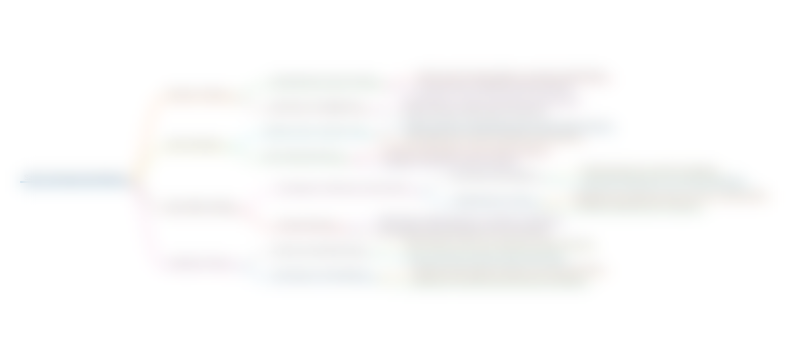
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
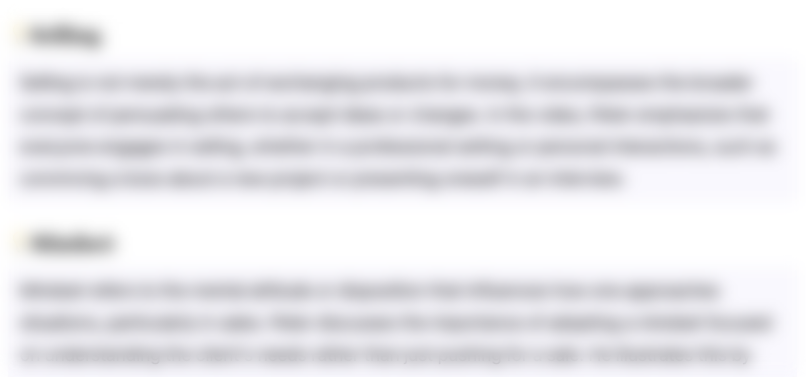
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
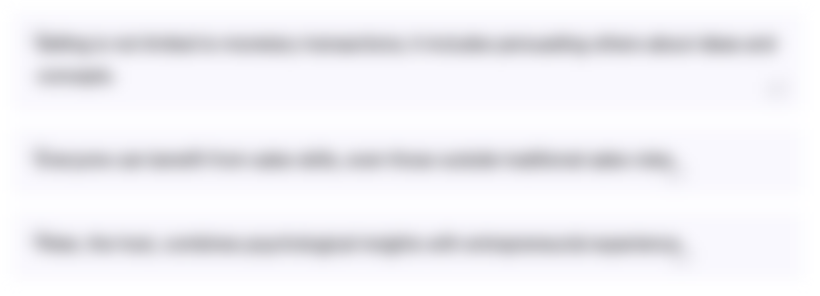
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
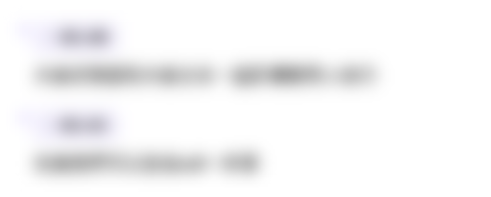
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
5.0 / 5 (0 votes)