Time & Space Complexity - Big O Notation - DSA Course in Python Lecture 1
Summary
TLDRIn this video, Greg explains key concepts of time and space complexity using Big O notation. He discusses the impact of different algorithms on performance, such as linear (O(n)) and quadratic (O(n^2)) time complexities, with practical examples like squaring numbers and finding pairs in an array. The video highlights how to visualize algorithm efficiency with graphs and explains how Big O represents worst-case scenarios. It also covers space complexity, including how using extra space or modifying the original array affects algorithm performance, providing a clear understanding of how to evaluate algorithm efficiency.
Takeaways
- 😀 Big O notation is used to measure the time and space complexity of algorithms, helping to understand their efficiency based on the input size.
- 😀 Time complexity is represented as Big O (e.g., O(n), O(n^2)), where 'n' refers to the number of elements or the input size.
- 😀 The example of squaring an array of numbers demonstrates O(n) time complexity, as each number in the array must be processed once.
- 😀 Generating all pairs of numbers from an array takes more time, resulting in O(n^2) time complexity because every element pairs with every other element.
- 😀 Time complexity can be visualized graphically, with O(n) showing a linear increase and O(n^2) representing a quadratic curve.
- 😀 A function with O(n^2) time complexity, such as generating all pairs, grows exponentially as the input size increases, making it significantly slower than O(n).
- 😀 Big O notation helps assess the worst-case scenario of an algorithm’s performance, indicating the maximum number of operations required.
- 😀 Space complexity measures how much memory an algorithm uses. O(n) space complexity indicates that the algorithm uses memory proportional to the input size.
- 😀 Algorithms that modify the original array or input data without allocating new space can have O(1) space complexity, meaning they use constant memory.
- 😀 Some algorithms have constant time complexity (O(1)), meaning they perform a fixed number of operations regardless of the input size (e.g., accessing the first element of an array).
- 😀 Constants and lower-order terms are typically discarded in Big O notation. For example, O(n^2 + 2n + 1) simplifies to O(n^2), as the highest degree term dominates the growth rate.
Q & A
What is Big O notation used for in programming?
-Big O notation is used to describe the time and space complexity of an algorithm. It helps measure how the runtime or memory usage of an algorithm increases as the size of the input grows.
In the example provided, what is the time complexity of the function that squares each number in an array?
-The time complexity of the function that squares each number in an array is Big O of n, or O(n), because it iterates through each element in the array once and performs a constant-time operation (squaring) on each.
How is the time complexity of the function that finds all pairs of numbers in an array different from the function that squares the numbers?
-The time complexity of the function that finds all pairs of numbers in an array is Big O of n^2, or O(n^2), because for each element in the array, the function must compare it with every other element, resulting in a nested loop. This leads to an overall quadratic growth in operations.
Why is the time complexity of the 'all pairs' function O(n^2)?
-The time complexity of the 'all pairs' function is O(n^2) because for each element in the array (n), the function performs another operation that involves iterating over most of the remaining elements, leading to a total of n * n operations.
What does it mean when we say an algorithm has constant time complexity, or O(1)?
-An algorithm with constant time complexity, O(1), means that the time to complete the operation does not depend on the size of the input. It will always take the same amount of time regardless of how large the array or input is.
What is an example of an algorithm with constant time complexity?
-An example of an algorithm with constant time complexity is array indexing. Accessing an element at a specific index in an array, such as 'a[0]', takes the same amount of time regardless of the size of the array.
How does space complexity relate to Big O notation, and what is an example in the provided script?
-Space complexity refers to the amount of memory an algorithm uses relative to the size of the input. In the provided script, squaring an array and storing the results in a new array would have a space complexity of O(n), because a new array of size n is created. However, modifying the original array in-place would result in O(1) space complexity, as no extra space is used.
Why do constants in Big O notation generally get omitted?
-Constants are omitted in Big O notation because they do not affect the growth rate of the algorithm as the input size increases. For example, if an algorithm has O(5n) time complexity, it is simplified to O(n), since the constant factor (5) does not change the fact that the algorithm grows linearly with the input size.
What is the difference between O(n) and O(n^2) in terms of algorithm performance?
-O(n) represents linear time complexity, meaning the time required grows directly proportional to the input size. O(n^2) represents quadratic time complexity, where the time grows much faster as the input size increases. A quadratic function is steeper and becomes significantly slower than a linear one for large input sizes.
Can Big O notation be used to compare different algorithms' performance, and how?
-Yes, Big O notation is commonly used to compare the efficiency of different algorithms. By expressing the time or space complexity in Big O terms, you can determine how an algorithm scales with increasing input size. For example, O(n) is generally faster than O(n^2) for large inputs, and O(1) is the most efficient in terms of time complexity.
Outlines
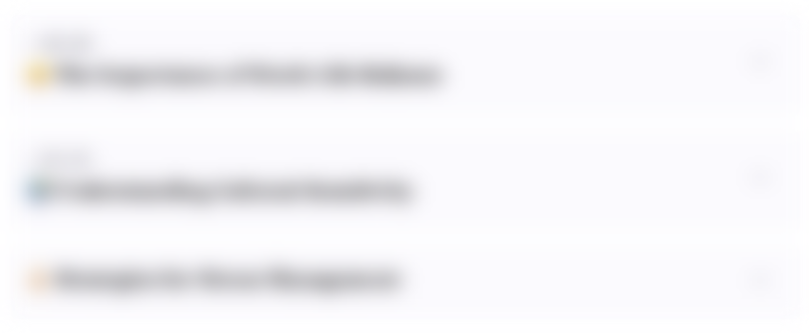
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
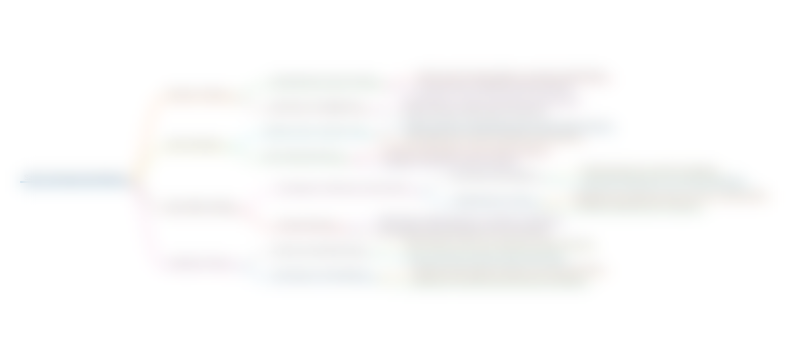
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
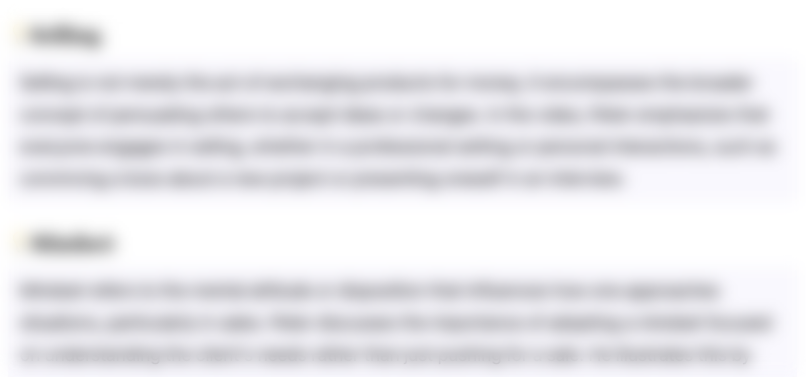
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
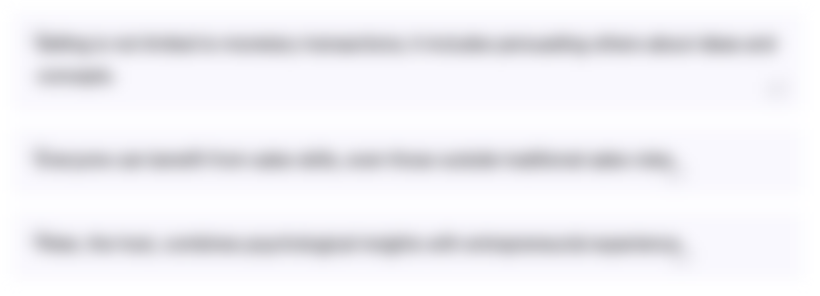
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
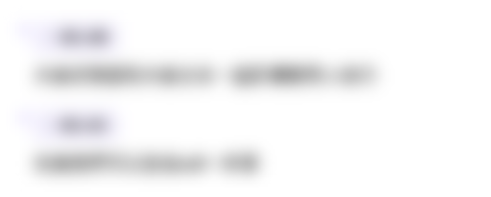
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführen5.0 / 5 (0 votes)